In AP Computer Science A, building on conditional statements to create multiple possible outcomes is a fundamental concept that allows programs to make decisions based on varying inputs and conditions. By using structures like ‘ if ‘, ‘ else if ‘, and ‘ else ‘, programmers can design logic that handles a wide range of scenarios, ensuring the program responds appropriately under different circumstances. Mastering these conditional constructs is crucial for developing complex, dynamic applications, and is essential for success on the AP Computer Science A exam.
Learning Objectives
When studying “Building on Conditional Statements to Create Multiple Possible Outcomes” for the AP Computer Science A exam, you should focus on mastering the ability to design and implement complex decision-making structures using ‘ if ‘, ‘ else if ‘, and ‘ else ‘ statements. Learn to create clear, logically ordered code that handles multiple scenarios effectively. Additionally, practice optimizing conditional logic for readability and performance, ensuring you can apply these skills to solve real-world problems efficiently and correctly in your exam.
Building on Conditional Statements to Create Multiple Possible Outcomes
In programming, conditional statements allow you to execute certain pieces of code based on whether a condition is true or false. When you’re building on conditional statements to create multiple possible outcomes, you’re typically working with a series of if, else if, and else statements. Each of these provides a different pathway that the program can follow depending on the value of certain variables or expressions.
1. Basic Structure of Conditional Statements
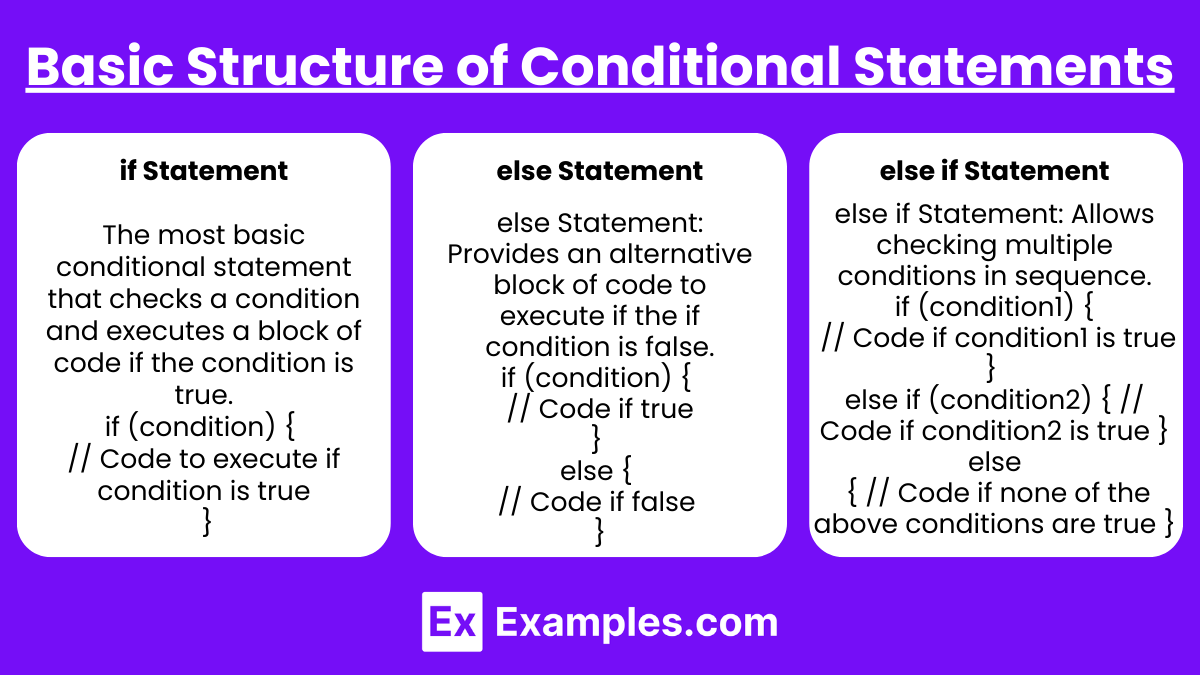
if Statement: The most basic conditional statement that checks a condition and executes a block of code if the condition is true.
if (condition) {
// Code to execute if condition is true
}
else Statement: Provides an alternative block of code to execute if the if condition is false.
if (condition) {
// Code if true
} else {
// Code if false
}
else if Statement: Allows checking multiple conditions in sequence. If the first condition is false, the next else if condition is evaluated.
if (condition1) {
// Code if condition1 is true
} else if (condition2) {
// Code if condition2 is true
} else {
// Code if none of the above conditions are true
}
2. Creating Multiple Possible Outcomes
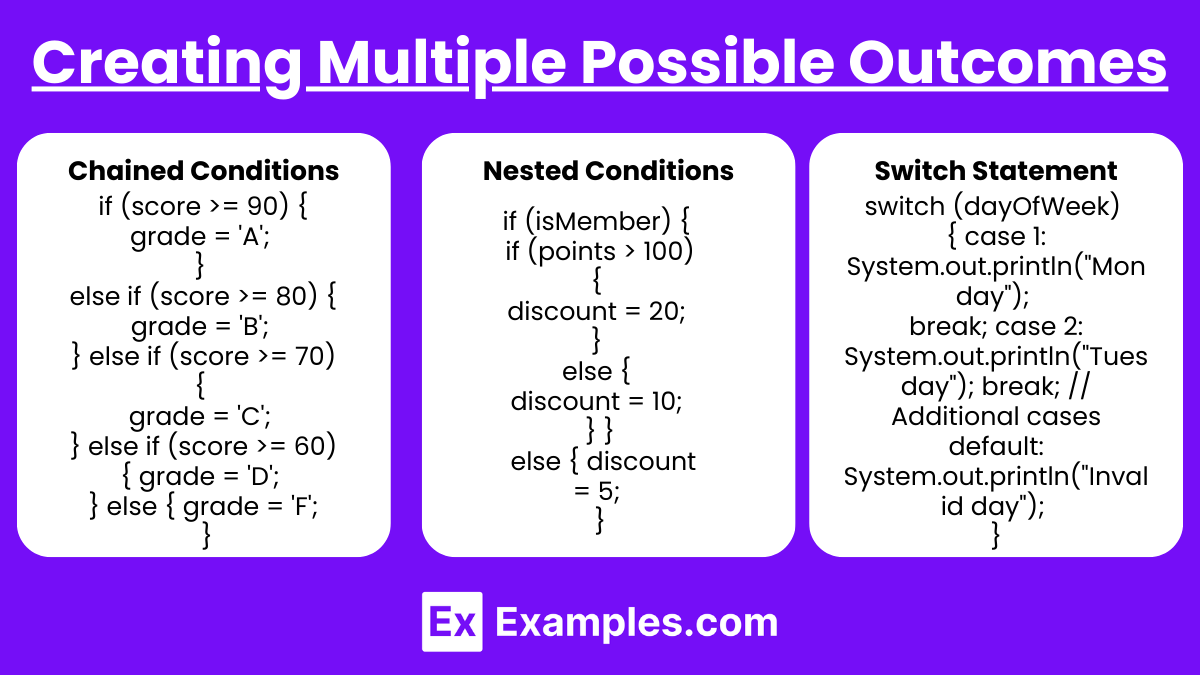
Chained Conditions: You can chain several else if statements to handle multiple scenarios. This is useful when you want to categorize outcomes based on ranges or distinct values.
if (score >= 90) {
grade = 'A';
} else if (score >= 80) {
grade = 'B';
} else if (score >= 70) {
grade = 'C';
} else if (score >= 60) {
grade = 'D';
} else {
grade = 'F';
}
Nested Conditions: Sometimes, conditions depend on previous outcomes, leading to nested if-else blocks.
if (isMember) {
if (points > 100) {
discount = 20;
} else {
discount = 10;
}
} else {
discount = 5;
}
Switch Statement: An alternative to multiple else if statements when comparing a single variable against many possible values. It’s typically used with discrete values rather than ranges.
switch (dayOfWeek) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// Additional cases
default:
System.out.println("Invalid day");
}
3. Best Practices for Conditional Statements
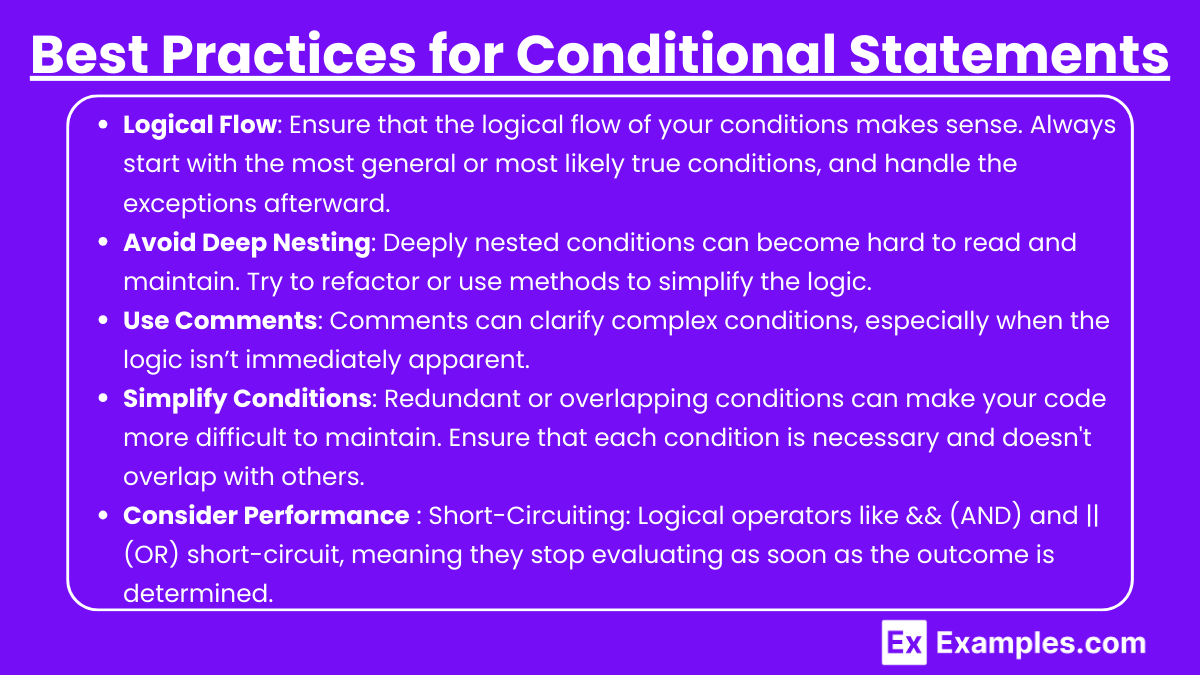
- Logical Flow: Ensure that the logical flow of your conditions makes sense. Always start with the most general or most likely true conditions, and handle the exceptions afterward.
- Avoid Deep Nesting: Deeply nested conditions can become hard to read and maintain. Try to refactor or use methods to simplify the logic.
- Use Comments: Comments can clarify complex conditions, especially when the logic isn’t immediately apparent.
- Simplify Conditions: Redundant or overlapping conditions can make your code more difficult to maintain. Ensure that each condition is necessary and doesn’t overlap with others. Combine or refactor conditions where possible to streamline the logic.
- Consider Performance : Short-Circuiting: Logical operators like && (AND) and || (OR) short-circuit, meaning they stop evaluating as soon as the outcome is determined. Utilize this to optimize your conditions for performance.
Examples
Example 1: Grading System
Imagine you are tasked with developing a grading system for a school. The system needs to assign a letter grade based on a student’s score. You would use a series of ‘ if-else ‘ statements to check the score against various thresholds. For instance, if the score is 90 or above, the grade is ‘A’. If it’s between 80 and 89, the grade is ‘B’, and so on, down to ‘F’ for scores below 60. This approach allows you to categorize the score into different outcomes effectively.
Example 2: Online Store Discount Calculation
Consider an online store that offers discounts based on the total purchase amount and whether the customer is a member. Using nested conditional statements, the program can check ‘ if ‘ the customer is a member and then apply different discount rates depending on the total amount spent. For example, a member spending over $100 might receive a 20% discount, while a non-member spending the same amount might only receive 10%. This scenario requires combining membership status and purchase amount to determine the final discount.
Example 3: Traffic Light Control System
A traffic light control system might use conditional statements to manage the flow of vehicles at an intersection. The system checks the current state of the light (e.g., green, yellow, red) and the time elapsed in that state. Based on these conditions, it determines the next state. For instance, if the light is green and has been green for more than 30 seconds, the system might change it to yellow. If it’s yellow for 5 seconds, it changes to red, and so on. This logic ensures smooth and orderly traffic management.
Example 4: User Authentication
In a user authentication system, the program might use conditional statements to handle various outcomes based on the user’s input. For example, if the username is correct but the password is incorrect, the system might prompt the user to retry. If both are incorrect, it could lock the account after a certain number of attempts. If the username and password are correct, the system grants access. This approach allows the system to handle different scenarios (correct/incorrect input) and respond appropriately.
Example 5 : Game Scoring System
In a video game, you might use conditional statements to determine the player’s level or rank based on their score or achievements. For instance, ‘ if ‘ a player’s score is between 0 and 1000, they remain at the beginner level. ‘ If ‘ the score is between 1001 and 5000, they advance to the intermediate level, and a score above 5000 might elevate them to the expert level. Additionally, special achievements or milestones might unlock bonuses or extra levels. This system creates a dynamic experience where multiple outcomes depend on the player’s performance.
Multiple Choice Questions
Question 1
Which of the following best describes the purpose of using else if in a conditional structure?
A) To provide an alternative action if the if condition is false and there are no other conditions.
B) To check multiple conditions sequentially, allowing different outcomes based on different criteria.
C) To execute a block of code only if all preceding conditions are false.
D) To terminate the program if a specific condition is met.
Answer: B) To check multiple conditions sequentially, allowing different outcomes based on different criteria.
Explanation: The else if statement is used when there are multiple possible conditions that need to be checked one after the other. If the first if condition is false, the program moves on to evaluate the else if condition, and so on. This structure allows the program to create multiple possible outcomes, each depending on the specific condition that is true.
Question 2
Consider the following code snippet:
int score = 85;
String grade;
if (score >= 90) {
grade = "A";
} else if (score >= 80) {
grade = "B";
} else if (score >= 70) {
grade = "C";
} else if (score >= 60) {
grade = "D";
} else {
grade = "F";
}
What will be the value of grade after this code executes?
A) “A”
B) “B”
C) “C”
D) “D”
Answer: B) “B”
Explanation: The variable score is set to 85. The program checks the conditions sequentially:
- The first condition score >= 90 is false.
- The second condition score >= 80 is true, so the code assigns “B” to the variable grade.
Since the correct condition is found, the subsequent else if statements are not evaluated. Therefore, the value of grade will be “B”.
Question 3
What is the advantage of using a ‘ switch ‘ statement instead of multiple ‘ else if ‘ statements when working with conditional logic?
A) A ‘ switch ‘ statement is faster in all cases compared to else if.
B) A ‘ switch ‘ statement allows for more complex conditions than else if.
C) A ‘ switch ‘ statement is more readable and efficient when dealing with a single variable with multiple possible discrete values.
D) A ‘ switch ‘ statement automatically handles all possible conditions without needing default cases.
Answer: C) A ‘ switch ‘ statement is more readable and efficient when dealing with a single variable with multiple possible discrete values.
Explanation: A ‘ switch ‘ statement is particularly useful when you have a single variable and need to compare it against multiple discrete values. It improves readability and efficiency by eliminating the need for multiple ‘ else if ‘ statements. Each case within a ‘ switch ‘ is clearly separated, making the logic easier to follow. However, it is not inherently faster in all cases, and it doesn’t handle complex conditions better than ‘ else if ‘. Also, a switch statement doesn’t automatically handle all conditions unless a default case is provided to cover any unhandled cases.