In Java programming for the AP Computer Science A exam, understanding how to call non-static void methods is essential. Non-static methods, unlike static methods, require an instance of their class to be invoked and can interact with the instance’s fields. Void methods are those that do not return any value, focusing instead on performing actions. This knowledge is foundational for managing behavior within objects, whether the methods require parameters to operate or not, enhancing both the code’s modularity and clarity.
Learning Objectives
For the topic “Calling Non-Static Void Methods With and Without Parameters” in AP Computer Science A, focus on understanding how to define non-static methods that do not return values, and how to invoke these methods using class instances. Learn to implement methods both with and without input parameters, recognize when to use each type, and appreciate how these methods interact with other parts of a class. Additionally, grasp the significance of method parameters in modifying the behavior of methods and their impact on a program’s functionality and readability.
Introduction to Non-Static Void Methods
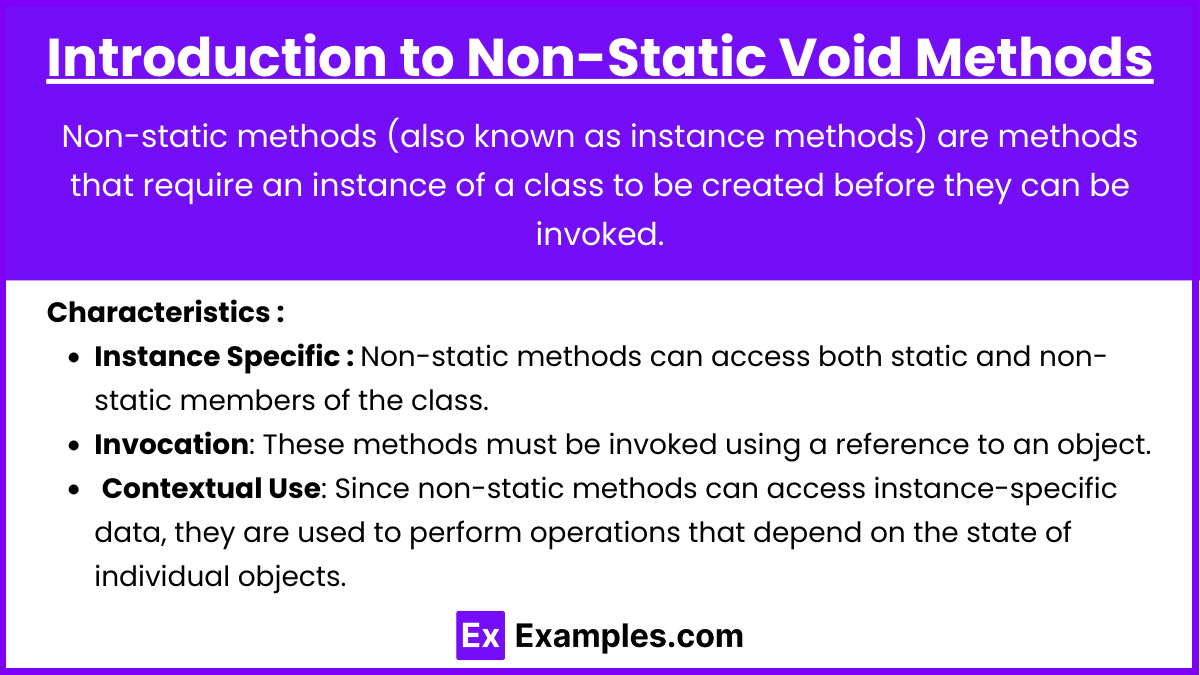
Non-static methods (also known as instance methods) are methods that require an instance of a class to be created before they can be invoked. These methods can manipulate instance variables that are non-static and can be accessed through the class instance.
Void methods are methods that do not return any value. Their main purpose is to perform an action rather than calculate and return data. When defining a void method, the keyword void is used in the method declaration.
Characteristics of Non-Static Methods
- Instance Specific: Non-static methods can access both static and non-static members of the class. However, their primary role is interacting with instance variables that belong to a specific object.
- Invocation: These methods must be invoked using a reference to an object. The syntax for calling a non-static method typically involves using the dot (.) operator on an object reference.
- Contextual Use: Since non-static methods can access instance-specific data, they are used to perform operations that depend on the state of individual objects.
Example of a Non-Static Void Method
Consider a class Car that has an instance method to start the car:
public class Car {
private boolean isRunning;
// Non-static void method
public void startEngine() {
isRunning = true;
System.out.println("Engine started!");
}
}
Calling Non-Static Void Methods Without Parameters
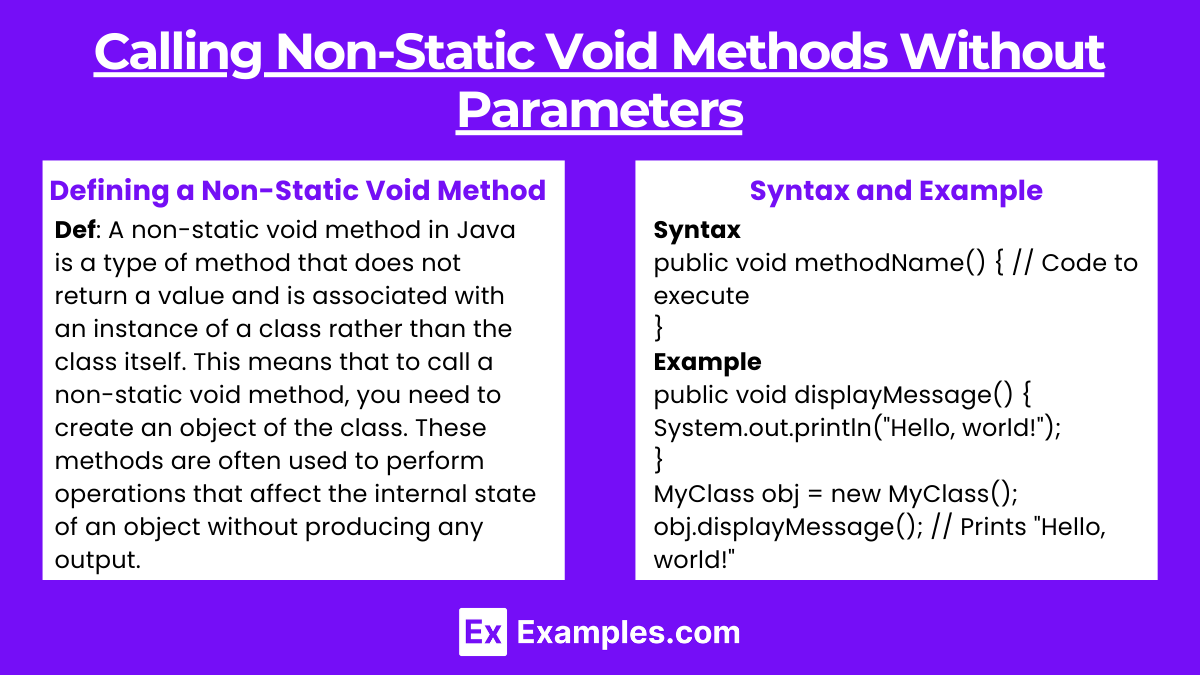
Defining a Non-Static Void Method: A non-static void method in Java is a type of method that does not return a value and is associated with an instance of a class rather than the class itself. This means that to call a non-static void method, you need to create an object of the class. These methods are often used to perform operations that affect the internal state of an object without producing any output. For instance, a method that updates the attributes of an object or initiates a process within the object would typically be defined as a non-static void method.
Syntax
public void methodName() {
// Code to execute
}
Example:
public void displayMessage() {
System.out.println("Hello, world!");
}
Creating an Instance:
Before you can call a non-static method, you must create an instance of the class.
Example:
MyClass obj = new MyClass();
Calling the Method:
Use the instance to call the method.
Example:
obj.displayMessage(); // Prints "Hello, world!"
Calling Non-Static Void Methods With Parameters
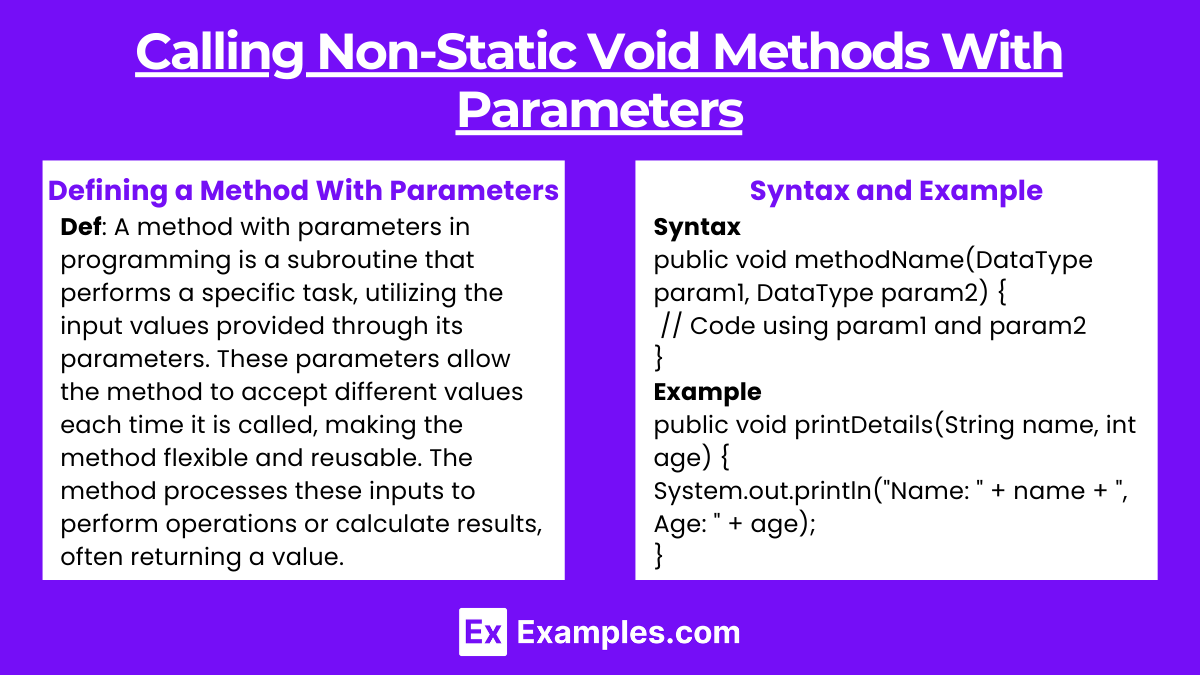
Defining a Method With Parameters:
Add parameters to the method definition to pass data to the method when called. A method with parameters in programming is a subroutine that performs a specific task, utilizing the input values provided through its parameters. These parameters allow the method to accept different values each time it is called, making the method flexible and reusable. The method processes these inputs to perform operations or calculate results, often returning a value. Parameters help customize the method’s execution context, enabling more dynamic interactions within a program’s code. For example, a method to calculate the area of a rectangle might accept parameters for length and width
Syntax:
public void methodName(DataType param1, DataType param2) {
// Code using param1 and param2
}
Example:
public void printDetails(String name, int age) {
System.out.println("Name: " + name + ", Age: " + age);
}
Calling the Method With Arguments:
Pass the arguments corresponding to the parameters when calling the method.
Example:
obj.printDetails("Alice", 30); // Prints "Name: Alice, Age: 30"
Examples
Example 1: Calling a Non-Static Void Method Without Parameters
Consider a class Robot that has a method to power on. The method powerOn does not take any parameters and simply prints a message indicating that the robot is powered on.
public class Robot {
public void powerOn() {
System.out.println("Robot is powered on.");
}
}
Robot myRobot = new Robot();
myRobot.powerOn(); // Output: Robot is powered on.
Example 2: Calling a Non-Static Void Method With One Parameter
In the Dog class, there is a method bark that takes one integer parameter representing the number of times the dog barks. This method prints a barking sound the specified number of times.
public class Dog {
public void bark(int times) {
for (int i = 0; i < times; i++) {
System.out.println("Bark!");
}
}
}
Dog myDog = new Dog();
myDog.bark(3); // Output: Bark! Bark! Bark!
Example 3: Calling a Non-Static Void Method With Multiple Parameters
In a Printer class, there is a method printDocument that accepts two parameters: the document name and the number of copies. It prints a message for each copy being printed.
public class Printer {
public void printDocument(String documentName, int copies) {
for (int i = 1; i <= copies; i++) {
System.out.println("Printing copy " + i + " of " + documentName);
}
}
}
Printer myPrinter = new Printer();
myPrinter.printDocument("Resume.pdf", 2); // Output: Printing copy 1 of Resume.pdf
// Printing copy 2 of Resume.pdf
Example 4: Calling a Non-Static Void Method Without Parameters to Modify an Instance Variable
The Light class has a method toggle that changes the state of a light from on to off or vice versa. This method doesn’t take any parameters and affects the instance variable isOn.
public class Light {
private boolean isOn = false;
public void toggle() {
isOn = !isOn;
System.out.println("Light is now " + (isOn ? "on" : "off"));
}
}
Light roomLight = new Light();
roomLight.toggle(); // Output: Light is now on
roomLight.toggle(); // Output: Light is now off
Example 5: Calling a Non-Static Void Method With Parameters to Modify State and Display Output
The Car class has a method updateFuelLevel that takes one parameter for the amount of fuel added. It updates the fuel level and prints the new level.
public class Car {
private int fuelLevel = 50; // Fuel level in percentage
public void updateFuelLevel(int newFuel) {
fuelLevel += newFuel;
System.out.println("Fuel level updated to: " + fuelLevel + "%");
}
}
Car myCar = new Car();
myCar.updateFuelLevel(20); // Output: Fuel level updated to: 70%
Multiple Choice Questions
Question 1
What is the primary purpose of a non-static void method in a Java class?
A) To return a value based on inputs
B) To perform an operation without returning a value
C) To modify the static fields of the class
D) To provide static utilities that can be accessed without creating an instance of the class
Correct Answer: B) To perform an operation without returning a value
Explanation:
Non-static void methods are designed to perform actions that do not return any value (denoted by the void keyword). These methods are instance methods, which means they work on the instance of a class and can manipulate instance variables. They are used for operations such as setting values, modifying the internal state of an object, or performing a task like printing output.
Question 2
Consider the following class definition:
public class Bicycle {
private int gear;
public void changeGear(int newGear) {
gear = newGear;
}
}
How would you correctly call the changeGear method on an instance of Bicycle?
A) ‘ Bicycle.changeGear(3); ‘
B) ‘ Bicycle myBike = new Bicycle(); myBike.changeGear(3); ‘
C) ‘ changeGear(3); ‘
D) ‘ new Bicycle().changeGear(); ‘
Correct Answer: B) ‘ Bicycle myBike = new Bicycle(); myBike.changeGear(3); ‘
Explanation:
Option B is correct because non-static methods require an instance of the class to be called. Here, a new instance of Bicycle named myBike is created, and then the changeGear method is called on this instance with the argument 3. This method call correctly uses the instance to access the non-static method, passing the necessary parameter to change the gear of the bicycle.
Question 3
What is the correct way to define a non-static void method in a Java class that takes no parameters and prints a message to the console?
A) ‘ public static void printMessage() { System.out.println(“Hello!”); } ‘
B) ‘ private void printMessage(String message) { System.out.println(message); } ‘
C) ‘ public void printMessage() { System.out.println(“Hello!”); } ‘
D) ‘ void printMessage(int number) { System.out.println(“Number: ” + number); } ‘
Correct Answer: C) ‘ public void printMessage() { System.out.println(“Hello!”); } ‘
Explanation:
Option C is the correct answer because it defines a public non-static void method that does not take any parameters and performs an operation (printing “Hello!” to the console). This definition allows the method to be called on instances of the class without requiring any arguments, adhering to the characteristics of non-static void methods that operate on an object’s state or perform actions without returning a value.