In AP Computer Science A, creating loops to run an expression repeatedly until certain conditions are met is a fundamental skill. Loops allow you to automate repetitive tasks, control program flow, and manage data efficiently. Understanding how to construct and manage ‘ for ‘, ‘ while ‘, and ‘ do-while ‘ loops enables you to handle various programming challenges, from simple iteration to complex logic. Mastery of loops is crucial for solving problems effectively, optimizing code, and achieving high scores on the AP exam.
Learning Objectives
For the topic “Creating a Loop to Run an Expression Repeatedly Until Certain Conditions are Met” in AP Computer Science A, you should focus on mastering the syntax and application of ‘ for ‘, ‘ while ‘, and ‘ do-while ‘ loops. Understand how to use loop variables, conditions, and control statements like ‘ break ‘ and ‘ continue ‘. Practice identifying and avoiding common pitfalls, such as infinite loops and off-by-one errors. Apply loops to solve practical problems, ensuring you can efficiently control repetitive tasks in your programs.
Creating a Loop to Run an Expression Repeatedly Until Certain Conditions are Met
In programming, loops are essential for executing a block of code multiple times without redundancy. When you need a loop to run an expression repeatedly until a specific condition is met, you’ll typically use either a while loop or a do-while loop in Java. Let’s explore both in detail:
1. while Loop
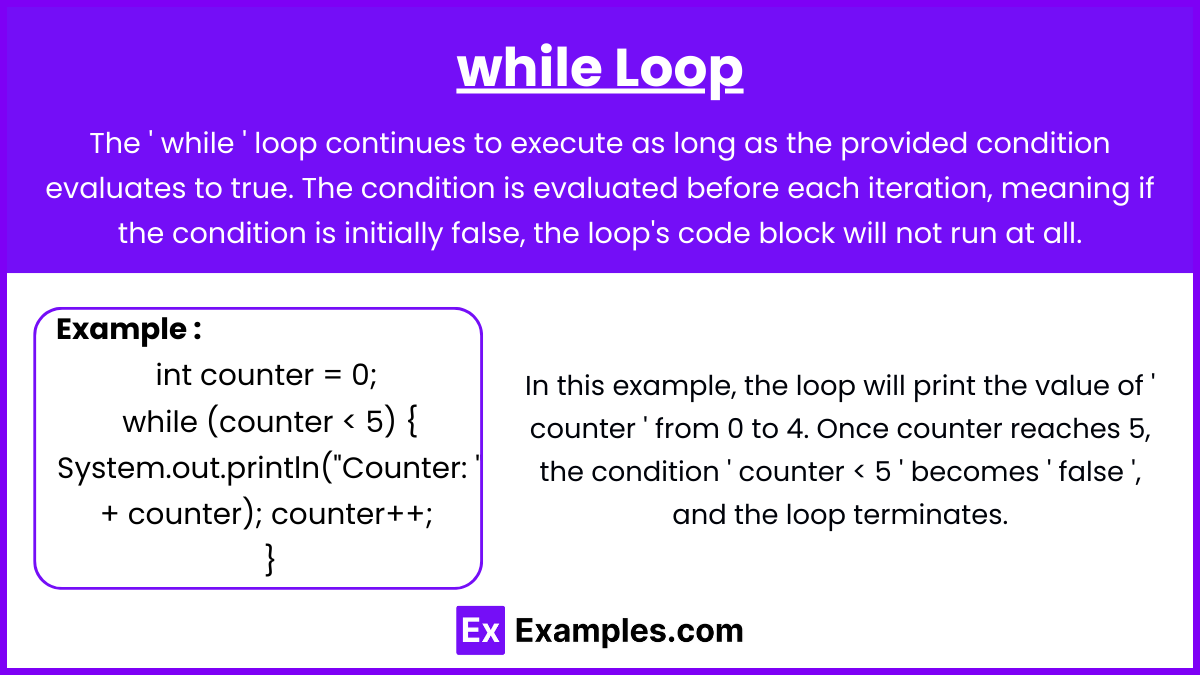
The ‘ while ‘ loop continues to execute as long as the provided condition evaluates to true. The condition is evaluated before each iteration, meaning if the condition is initially false, the loop’s code block will not run at all.
Syntax:
while (condition) {
// code to be executed
}
Example:
int counter = 0;
while (counter < 5) {
System.out.println("Counter: " + counter);
counter++;
}
In this example, the loop will print the value of ‘ counter ‘ from 0 to 4. Once counter reaches 5, the condition ‘ counter < 5 ‘ becomes ‘ false ‘, and the loop terminates.
2. do-while Loop
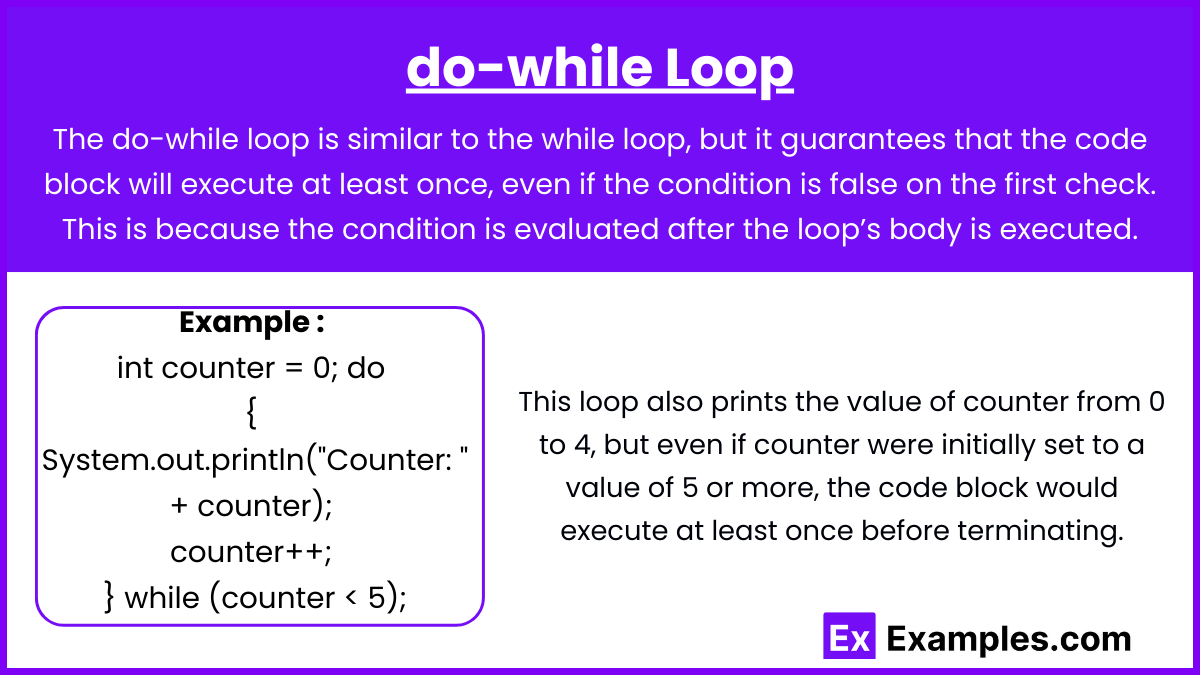
The do-while loop is similar to the while loop, but it guarantees that the code block will execute at least once, even if the condition is false on the first check. This is because the condition is evaluated after the loop’s body is executed.
Syntax:
do {
// code to be executed
} while (condition);
Example:
int counter = 0;
do {
System.out.println("Counter: " + counter);
counter++;
} while (counter < 5);
This loop also prints the value of counter from 0 to 4, but even if counter were initially set to a value of 5 or more, the code block would execute at least once before terminating.
3. for Loop (with condition)
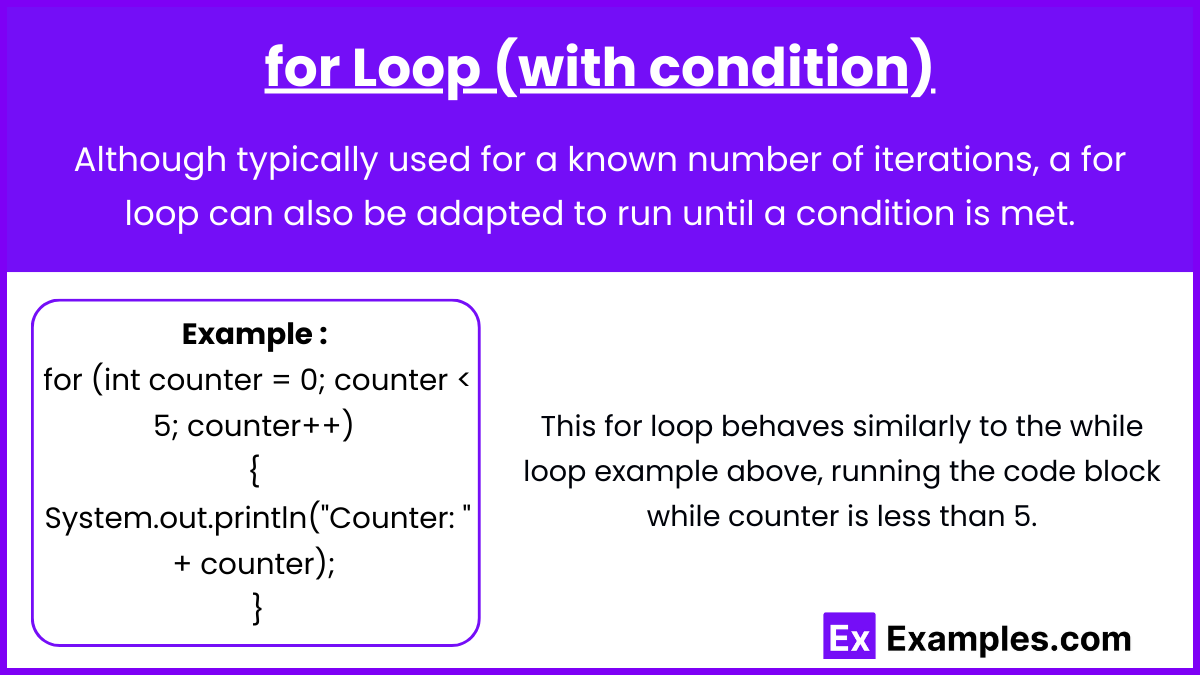
Although typically used for a known number of iterations, a for loop can also be adapted to run until a condition is met.
Syntax:
for (initialization; condition; update) {
// code to be executed
}
Example:
for (int counter = 0; counter < 5; counter++) {
System.out.println("Counter: " + counter);
}
This for loop behaves similarly to the while loop example above, running the code block while counter is less than 5.
4. Infinite Loops and Breaking Out
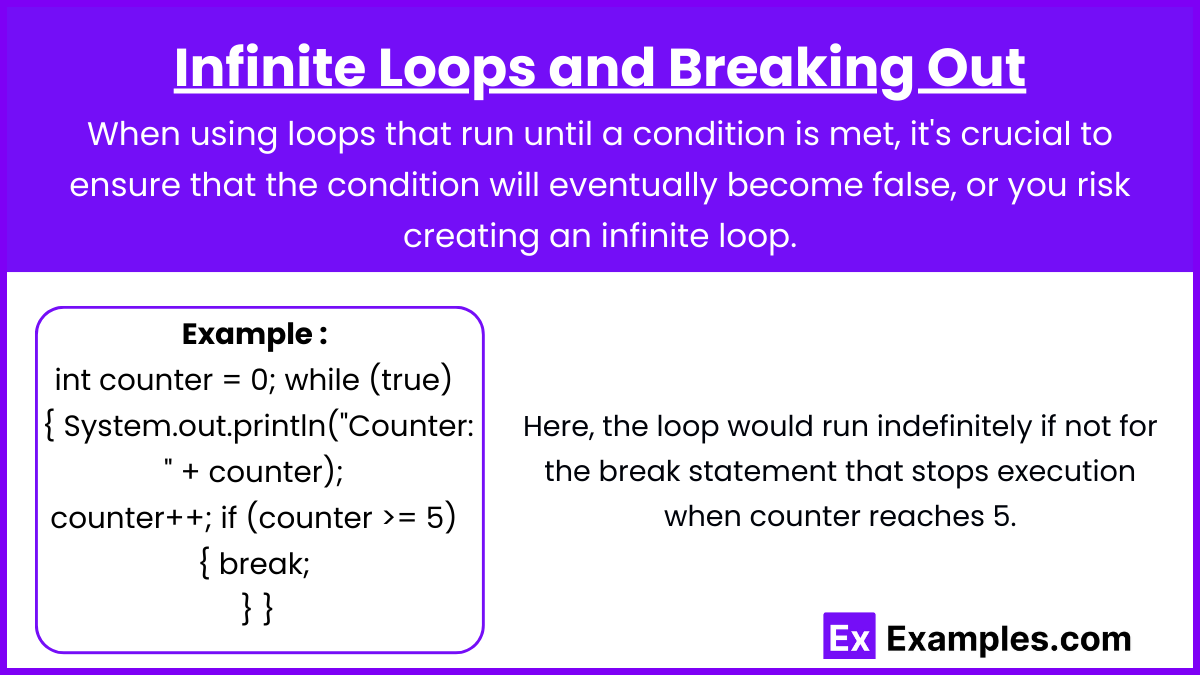
When using loops that run until a condition is met, it’s crucial to ensure that the condition will eventually become false, or you risk creating an infinite loop.
Example of an Infinite Loop:
while (true) {
// This loop runs forever unless there's a break
}
You can break out of a loop prematurely using the break statement when a certain condition inside the loop is met.
Example:
int counter = 0;
while (true) {
System.out.println("Counter: " + counter);
counter++;
if (counter >= 5) {
break;
}
}
Here, the loop would run indefinitely if not for the break statement that stops execution when counter reaches 5.
Controlling Loop Execution
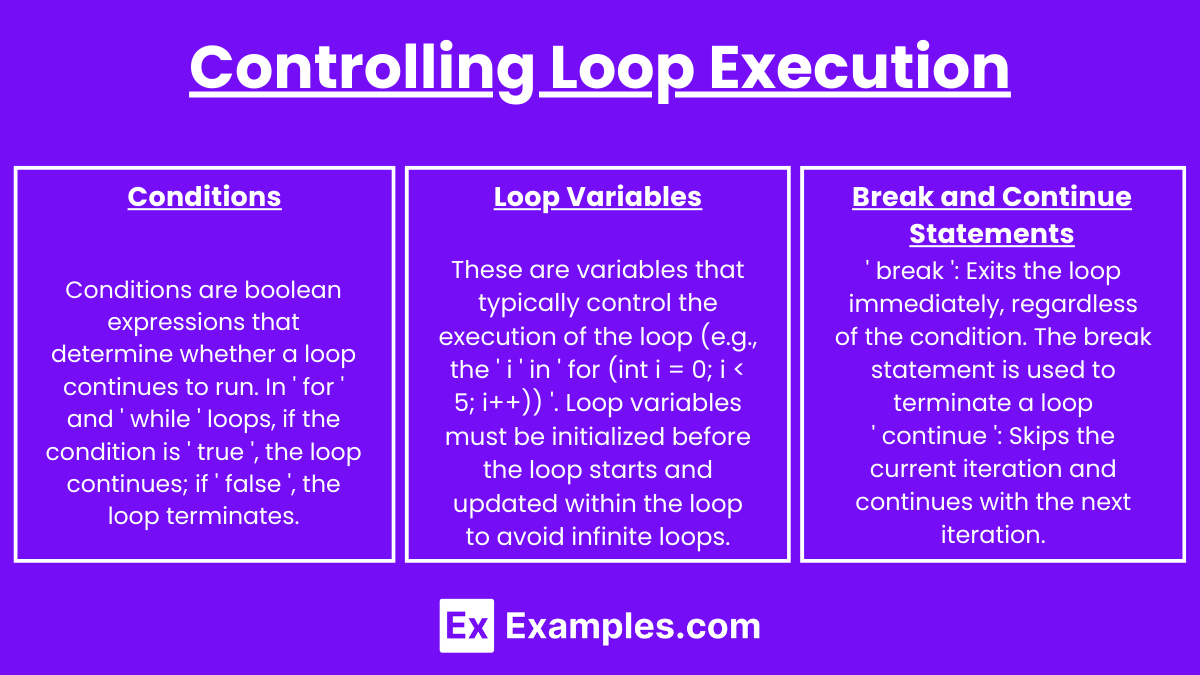
- Conditions:
- Conditions are boolean expressions that determine whether a loop continues to run. In ‘ for ‘ and ‘ while ‘ loops, if the condition is ‘ true ‘, the loop continues; if ‘ false ‘, the loop terminates.
- Loop Variables:
- These are variables that typically control the execution of the loop (e.g., the ‘ i ‘ in ‘ for (int i = 0; i < 5; i++)) ‘. Loop variables must be initialized before the loop starts and updated within the loop to avoid infinite loops.
- Break and Continue Statements:
- ‘ break ‘: Exits the loop immediately, regardless of the condition. The break statement is used to terminate a loop.
- ‘ continue ‘: Skips the current iteration and continues with the next iteration. The continue statement skips the current iteration of the loop and immediately jumps to the next iteration.
Examples
Example 1: Calculating Factorials
A common use of loops is to calculate the factorial of a number, which is the product of all positive integers up to that number. For instance, to compute 5!, you could use a for loop that multiplies numbers from 1 through 5. The loop runs until the counter reaches the number whose factorial is being calculated. This is useful in various mathematical and computer science applications where factorial calculations are required.
Example 2: Finding the Greatest Common Divisor (GCD)
The Euclidean algorithm for finding the GCD of two numbers involves repeatedly subtracting the smaller number from the larger one until the two numbers are equal, which is the GCD. This process can be efficiently implemented using a while loop, where the loop continues to execute as long as the two numbers differ, ensuring that the condition is met for the algorithm to stop and produce the correct result.
Example 3: User Input Validation
A loop can be used to repeatedly prompt the user for input until valid data is provided. For example, if a program requires the user to enter a number between 1 and 10, a while loop can keep asking for input until the user enters a value within the required range. This loop ensures that the program only proceeds when the input meets the specified condition, improving the program’s robustness and user experience.
Example 4: Simulating a Countdown Timer
Imagine a simple countdown timer that prints the numbers from 10 down to 1. A for loop or a while loop can be used here, with the loop decrementing the counter each time until it reaches zero. This loop continues to run until the condition of reaching zero is met, at which point the loop exits, and the countdown is complete.
Example 5: Searching in an Array
A loop can be used to search for a specific value in an array, iterating through each element until the value is found or the end of the array is reached. A for or while loop is typically employed, where the loop continues to run as long as the target value has not been found, ensuring that the search is comprehensive. This method is fundamental in algorithms like linear search, which is often taught in introductory computer science courses.
Multiple Choice Questions
Question 1
Which of the following loop types guarantees that the loop body will be executed at least once, regardless of the initial condition?
A) ‘ for ‘ loop
B) ‘ while ‘ loop
C) ‘ do-while ‘ loop
D) ‘ Infinite ‘ loop
Answer: C) ‘ do-while ‘ loop
Explanation: The ‘ do-while ‘ loop differs from ‘ for ‘ and ‘ while ‘ loops because it checks the condition after the loop body has executed. This ensures that the loop body runs at least once, even if the condition is initially false. In contrast, ‘ for ‘ and ‘ while ‘ loops check the condition before executing the loop body, so if the condition is false at the start, the loop body may not execute at all.
Question 2
What will be the output of the following code?
int count = 0;
while (count < 3) {
System.out.println("Count is: " + count);
count++;
}
A) Count is: 1
Count is: 2
Count is: 3
B) Count is: 0
Count is: 1
Count is: 2
C) Count is: 0
Count is: 1
Count is: 2
Count is: 3
D) Count is: 3
Count is: 2
Count is: 1
Answer: B) Count is: 0
Count is: 1
Count is: 2
Explanation: The loop starts with ‘ count ‘ initialized to 0. The condition ‘ count < 3 ‘ is checked before each iteration. Since the condition is true for ‘ count = 0 ‘, ‘ count = 1 ‘, and ‘ count = 2 ‘, the loop executes three times, printing ‘ Count is: 0 ‘, ‘ Count is: 1 ‘, and ‘ Count is: 2 ‘. After the third iteration, ‘ count ‘ becomes 3, which makes the condition false, and the loop terminates.
Question 3
Consider the following code snippet:
for (int i = 10; i > 0; i--) {
if (i == 5) {
break;
}
System.out.print(i + " ");
}
What will be the output of this code?
A) 10 9 8 7 6 5
B) 10 9 8 7 6
C) 10 9 8 7 6 5 4 3 2 1
D) 10 9 8 7 6 5 4 3 2
Answer: B) 10 9 8 7 6
Explanation: The ‘ for ‘ loop starts with ‘ i = 10 ‘ and decrements ‘ i ‘ by 1 in each iteration. The loop checks if ‘ i == 5 ‘ during each iteration. When ‘ i ‘ becomes 5, the ‘ break ‘ statement is executed, which immediately terminates the loop. Therefore, the output will be ‘ 10 9 8 7 6 ‘, and the loop stops before printing 5.