In AP Computer Science A, understanding constructors is crucial for object creation in Java. Constructors are special methods called when a new object is instantiated, initializing it with default or specific values. Default constructors don’t take parameters and usually set fields to predetermined values, while parameterized constructors allow for the customization of these values during object creation. This distinction is key for writing flexible and efficient code, as constructors determine how objects are initialized and managed in a program.
Learning Objectives
When studying “Creating Objects by Calling Constructors with and without Parameters” for AP Computer Science A, you should aim to understand how constructors initialize objects in Java, both with default values (no-argument constructors) and specific values (parameterized constructors). Focus on learning how to define and use constructors, the role of the ‘ this ‘ keyword, and how constructor overloading allows for flexibility in object creation. Additionally, practice writing classes that demonstrate these concepts, ensuring you can apply them effectively in code to manage object state.
What is a Constructor?
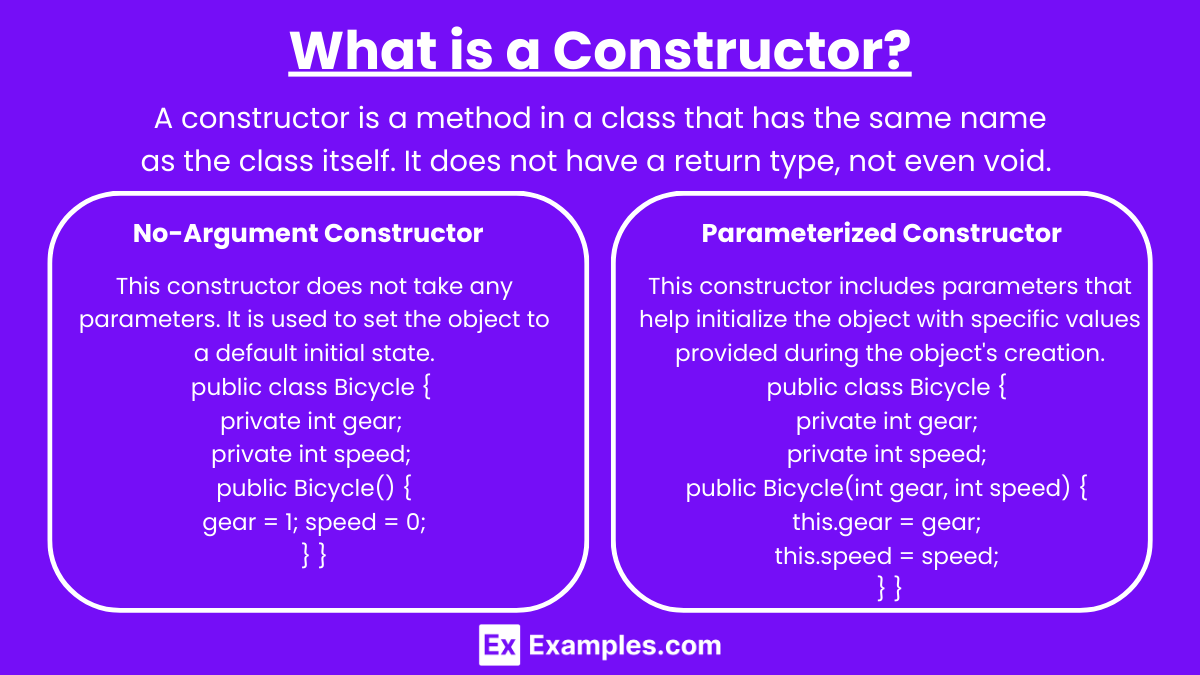
A constructor is a method in a class that has the same name as the class itself. It does not have a return type, not even void. The primary role of a constructor is to initialize the object’s state by setting initial values for the attributes of the object.
Types of Constructors
- No-Argument Constructor: This constructor does not take any parameters. It is used to set the object to a default initial state. If no constructor is explicitly defined in a class, Java automatically provides a default no-argument constructor that does nothing but call the superclass’s no-argument constructor. However, you can define your own no-argument constructor to set up initial values or perform actions when the object is created.
Example :
public class Bicycle {
private int gear;
private int speed;
// No-argument constructor
public Bicycle() {
gear = 1;
speed = 0;
}
}
- Parameterized Constructor: This constructor includes parameters that help initialize the object with specific values provided during the object’s creation. Parameterized constructors enhance the flexibility of object creation by allowing initialization with specified values.
Example :
public class Bicycle {
private int gear;
private int speed;
// Parameterized constructor
public Bicycle(int gear, int speed) {
this.gear = gear;
this.speed = speed;
}
}
Calling Constructors with and without Parameters
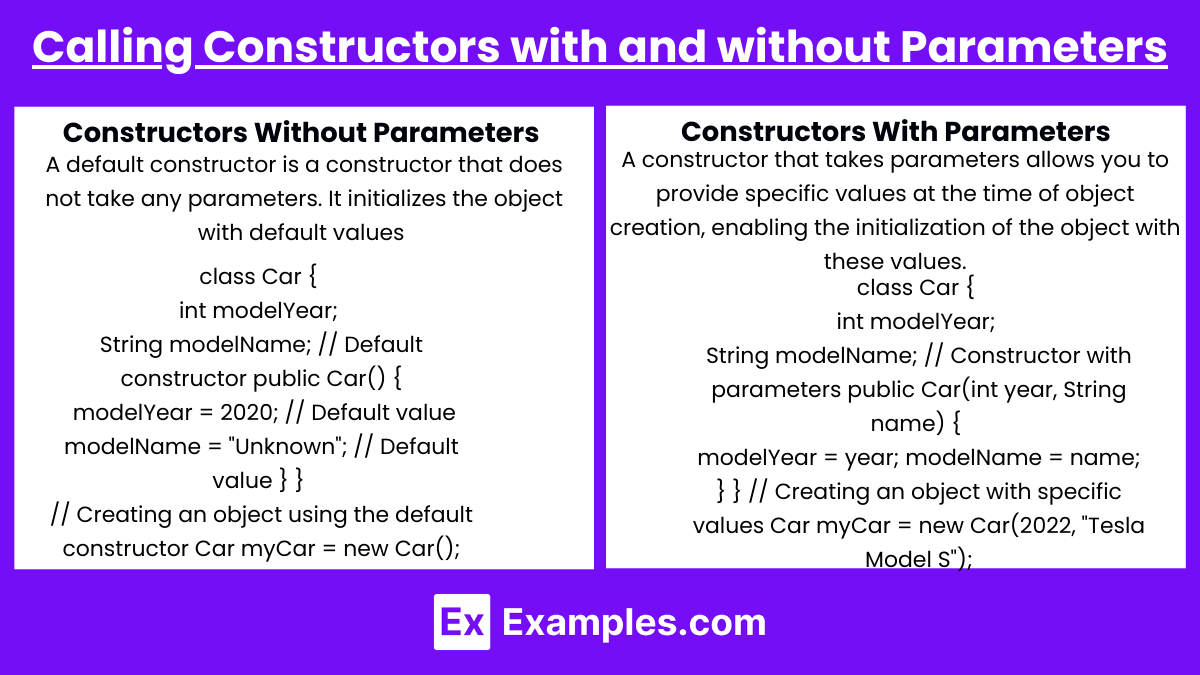
Constructors Without Parameters (Default Constructors)
- Definition: A default constructor is a constructor that does not take any parameters. It initializes the object with default values (e.g., null for objects, 0 for integers, false for boolean).
- Example:
class Car {
int modelYear;
String modelName;
// Default constructor
public Car() {
modelYear = 2020; // Default value
modelName = "Unknown"; // Default value
}
}
// Creating an object using the default constructor
Car myCar = new Car();
Constructors With Parameters
- Definition: A constructor that takes parameters allows you to provide specific values at the time of object creation, enabling the initialization of the object with these values.
- Example:
class Car {
int modelYear;
String modelName;
// Constructor with parameters
public Car(int year, String name) {
modelYear = year;
modelName = name;
}
}
// Creating an object with specific values
Car myCar = new Car(2022, "Tesla Model S");
Examples
Example 1: Constructor with Parameters
class Rectangle {
int width;
int height;
// Constructor with parameters
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
}
// Creating a rectangle object with specific dimensions
Rectangle myRectangle = new Rectangle(50, 100);
Here, the Rectangle class has a constructor that takes two parameters: width and height. This allows for the creation of a rectangle object with specific dimensions, demonstrated by the creation of myRectangle with a width of 50 and height of 100.
Example 2: Multiple Constructors (Overloading)
class Student {
String name;
int age;
// Default constructor
public Student() {
name = "Unknown";
age = 0;
}
// Constructor with parameters
public Student(String name, int age) {
this.name = name;
this.age = age;
}
}
// Creating student objects using both constructors
Student unknownStudent = new Student();
Student knownStudent = new Student("Alice", 21);
In this example, the Student class features two constructors: a default constructor and a parameterized one. This demonstrates constructor overloading, allowing the creation of student objects either with default values or specific properties.
Example 3: Complex Object with Multiple Data Types
class Smartphone {
String model;
int storage;
boolean isWaterResistant;
// Constructor with parameters
public Smartphone(String model, int storage, boolean isWaterResistant) {
this.model = model;
this.storage = storage;
this.isWaterResistant = isWaterResistant;
}
}
// Creating a smartphone object with specific features
Smartphone myPhone = new Smartphone("Galaxy S21", 256, true);
The Smartphone class includes a constructor with multiple parameters of different types (String, int, boolean), enabling the initialization of a new smartphone object with a specified model, storage capacity, and water-resistant feature. The creation of myPhone shows how diverse attributes can be initialized at the time of object creation.
Example 4: Constructor with Default Values and Parameters
class Laptop {
String brand;
int ram;
boolean hasSSD;
// Constructor with parameters and default values
public Laptop(String brand, int ram) {
this.brand = brand;
this.ram = ram;
this.hasSSD = true; // Default to true
}
}
// Creating a laptop object with specified brand and
RAM Laptop myLaptop = new Laptop("Dell", 16);
This example illustrates the use of a constructor with parameters for brand and ram, while setting a default value for the hasSSD property. When myLaptop is created, it is initialized with the specified brand “Dell” and 16 GB of RAM, and it automatically has an SSD.
Example 5: Nested Object Initialization
class Engine {
int horsepower;
// Constructor for Engine
public Engine(int horsepower) {
this.horsepower = horsepower;
}
}
class Car {
String make;
Engine engine;
// Constructor with nested object
public Car(String make, int engineHorsepower) {
this.make = make;
this.engine = new Engine(engineHorsepower); // Creating a new Engine object within the Car constructor
}
}
// Creating a car object with a nested engine object
Car myCar = new Car("Toyota", 268);
In this example, the Car class has a constructor that not only initializes the make of the car but also creates a new Engine object with specified horsepower. The myCar object is thus initialized with a “Toyota” make and an engine of 268 horsepower, demonstrating how constructors can be used to create complex objects with nested object properties.
Multiple Choice Questions
Question 1
What will happen if you try to instantiate an object using a constructor that does not exist in the class definition?
A) A default constructor will be used automatically.
B) A runtime exception will be thrown.
C) A compile-time error will occur.
D) The object will be created with null values for all its fields.
Correct Answer: C) A compile-time error will occur.
Explanation: If you try to create an object using a constructor that has not been defined in the class, Java will not be able to find a matching constructor to call and will therefore produce a compile-time error. Java does not automatically provide a default constructor if any constructors are explicitly defined in the class.
Question 2
Consider the following class definition:
class Vehicle {
String type;
int year;
public Vehicle(String type) {
this.type = type;
}
}
What is the correct way to create a new ‘ Vehicle ‘ object?
A) ‘ Vehicle myVehicle = new Vehicle(); ‘
B) ‘ Vehicle myVehicle = new Vehicle(“Car”); ‘
C) ‘ Vehicle myVehicle = new Vehicle(2015); ‘
D) ‘ Vehicle myVehicle = new Vehicle(“Car”, 2015); ‘
Correct Answer: B) Vehicle myVehicle = new Vehicle(“Car”);
Explanation: The only constructor defined in the Vehicle class requires a single String argument for the type of the vehicle. The correct way to instantiate a Vehicle object with this class definition is to provide a string argument, as shown in option B. Options A, C, and D either do not provide the necessary string argument or provide incorrect or extra arguments that do not match any constructor in the class.
Question 3
Which of the following statements about constructors in Java is true?
A) Constructors can return values.
B) Constructors must have the same name as the class.
C) A class can only have one constructor.
D) Constructors can only initialize fields.
Correct Answer: B) Constructors must have the same name as the class.
Explanation: In Java, constructors must have the same name as the class in which they are defined and cannot have a return type, not even ‘ void ‘. This is how Java distinguishes a constructor from a method. A class can have multiple constructors (constructor overloading) as long as each constructor has a different parameter list, and constructors can do more than just initialize fields; they can also execute any code needed during the object’s initialization (though initializing fields is their primary purpose). Thus, option B is the only true statement.