“Creating the Same Value Using Equivalent Boolean Expressions” is a crucial topic in AP Computer Science A, focusing on transforming Boolean expressions to achieve the same logical outcome using different forms. This skill is essential for optimizing code, improving readability, and reducing complexity in programming. By understanding and applying logical equivalences like De Morgan’s Laws, double negation, and distributive properties, students can simplify conditions and enhance their problem-solving abilities, leading to more efficient and maintainable code in various programming scenarios.
Learning Objectives
When studying “Creating the Same Value Using Equivalent Boolean Expressions” for AP Computer Science A, you should focus on mastering the ability to recognize and apply logical equivalences such as De Morgan’s Laws, simplifying Boolean expressions, and refactoring code to improve clarity and efficiency. You should also aim to identify and eliminate redundant conditions, ensuring your code is both efficient and easy to maintain. Understanding these concepts is crucial for writing optimized and correct Boolean logic in programming.
Using Equivalent Boolean Expressions
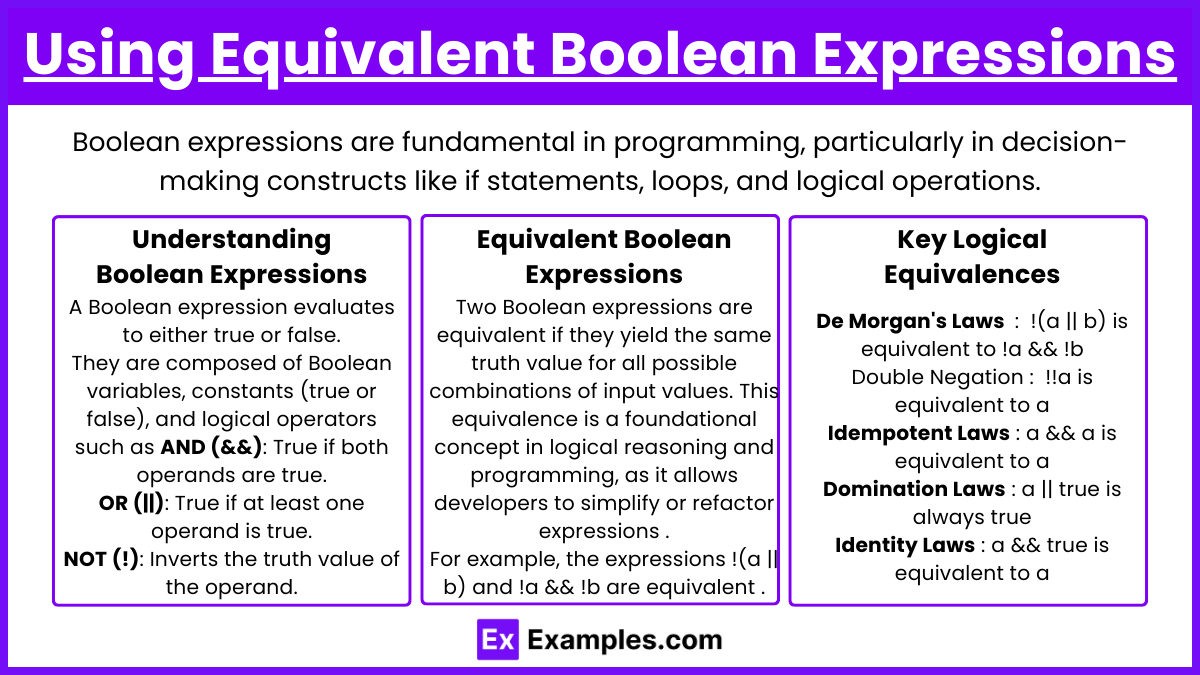
Boolean expressions are fundamental in programming, particularly in decision-making constructs like if statements, loops, and logical operations. Understanding how to create equivalent Boolean expressions can improve code readability, efficiency, and correctness.
1. Understanding Boolean Expressions
- A Boolean expression evaluates to either true or false.
- They are composed of Boolean variables, constants (true or false), and logical operators such as:
- AND (&&): True if both operands are true.
- OR (||): True if at least one operand is true.
- NOT (!): Inverts the truth value of the operand.
2. Equivalent Boolean Expressions
- Two Boolean expressions are equivalent if they yield the same truth value for all possible combinations of input values. This equivalence is a foundational concept in logical reasoning and programming, as it allows developers to simplify or refactor expressions without changing the outcome of the logic.
- For example, the expressions !(a || b) and !a && !b are equivalent due to De Morgan’s Laws. For instance, consider the expression ‘ !(a || b) ‘ and its equivalent ‘ !a && !b ‘. According to De Morgan’s Laws, these two expressions will always yield the same result.
3. Key Logical Equivalences
- De Morgan’s Laws:
- !(a || b) is equivalent to !a && !b
- !(a && b) is equivalent to !a || !b
- Double Negation:
- !!a is equivalent to a
- Idempotent Laws:
- a && a is equivalent to a
- a || a is equivalent to a
- Domination Laws:
- a || true is always true
- a && false is always false
- Identity Laws:
- a && true is equivalent to a
- a || false is equivalent to a
- Distributive Laws:
- a && (b || c) is equivalent to (a && b) || (a && c)
- a || (b && c) is equivalent to (a || b) && (a || c)
Application in Coding
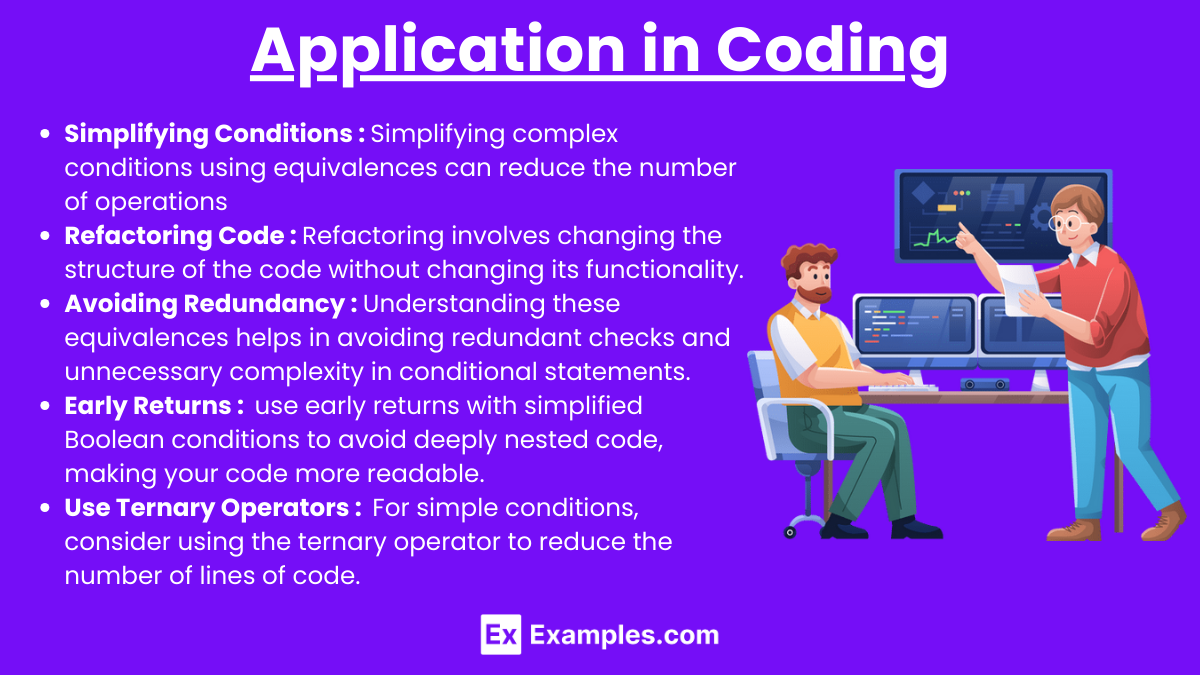
- Simplifying Conditions:
- Simplifying complex conditions using equivalences can reduce the number of operations and make the code more efficient.
- Refactoring Code:
- Refactoring involves changing the structure of the code without changing its functionality. Using equivalent Boolean expressions can help in refactoring conditions for better clarity or performance.
- Avoiding Redundancy:
- Understanding these equivalences helps in avoiding redundant checks and unnecessary complexity in conditional statements.
- Early Returns:
- In methods, use early returns with simplified Boolean conditions to avoid deeply nested code, making your code more readable.
- Use Ternary Operators:
- For simple conditions, consider using the ternary operator to reduce the number of lines of code.
Examples
Example 1: De Morgan’s Law Application
Consider the expression ‘ !(a || b) ‘. According to De Morgan’s Law, this expression can be rewritten as ‘ !a && !b ‘. Both expressions yield the same result because De Morgan’s Law states that the negation of a disjunction (||) is equivalent to the conjunction (&&) of the negations. For example, if ‘ a ‘ is ‘ true ‘ and ‘ b ‘ is ‘ false ‘, the original expression ‘ !(true || false) ‘ evaluates to ‘ false ‘, and the equivalent expression ‘ !true && !false ‘ also evaluates to ‘ false ‘.
Example 2: Simplifying Nested Negations:
An expression like ‘ !!x ‘ is equivalent to ‘ x ‘. The double negation cancels out, making the expression equivalent to its original value. For instance, if ‘ x ‘ is ‘ true ‘, then ‘ !!true ‘ simplifies to ‘ true ‘. This simplification is useful in scenarios where complex logic might introduce unnecessary negations, and recognizing this equivalence helps in simplifying Boolean conditions.
Example 3: Combining Conditions
Consider the expression ‘ (x > 5 && y > 5) || (x > 5 && z > 5) ‘. This can be simplified to ‘ x > 5 ‘ && ‘ (y > 5 || z > 5) ‘. Both expressions are equivalent because they evaluate to ‘ true ‘ under the same conditions: when ‘ x ‘ is greater than 5, and at least one of ‘ y ‘ or ‘ z ‘ is also greater than 5. This equivalence demonstrates how you can factor out common conditions to reduce redundancy and improve clarity.
Example 4: Distributing AND Over OR
The expression ‘ a && (b || c) ‘ is equivalent to ‘ (a && b) || (a && c) ‘. This distributive property allows you to expand a condition into a form that might be easier to evaluate in certain contexts. For example, if ‘ a ‘ is ‘ true ‘, and either ‘ b ‘ or ‘ c ‘ is ‘ true ‘, both forms will evaluate to ‘ true ‘. This equivalence is particularly useful when refactoring code to make logical expressions more transparent.
Example 5: Simplifying Always-True Conditions
The expression ‘ x || !x ‘ is always ‘ true ‘ because for any Boolean value of ‘ x ‘, either ‘ x ‘ or its negation ‘ !x ‘ will be ‘ true ‘. This can be simplified to the constant ‘ true ‘. Understanding that such expressions are equivalent to a constant value can help in optimizing code by eliminating unnecessary condition checks, leading to more efficient execution paths.
Multiple Choice Questions
Question 1
Which of the following expressions is equivalent to ‘ !(a && b) ‘?
A) ‘ !a || !b ‘
B) ‘ !a && !b ‘
C) ‘ a || b ‘
D) ‘ a && !b ‘
Answer: A) ‘ !a || !b ‘
Explanation: The expression ‘ !(a && b) ‘ can be transformed using De Morgan’s Law. De Morgan’s Law states that the negation of a conjunction (&&) is equivalent to the disjunction (||) of the negations. Therefore, ‘ !(a && b) ‘ is equivalent to ‘ !a || !b ‘. This equivalence is useful in simplifying Boolean expressions, especially when refactoring code to make it clearer or more efficient.
Question 2
What is the simplified equivalent of the expression ‘ (x > 10 && y > 5) || (x > 10 && z < 3) ‘?
A) ‘ x > 10 && (y > 5 || z < 3) ‘
B) ‘ x > 10 && (y > 5 && z < 3) ‘
C) ‘ (x > 10 || y > 5) && (x > 10 || z < 3) ‘
D) ‘ x > 10 || (y > 5 && z < 3) ‘
Answer: A) ‘ x > 10 && (y > 5 || z < 3) ‘
Explanation: The given expression can be simplified by factoring out the common condition ‘ x > 10 ‘. The original expression is ‘ (x > 10 && y > 5) || (x > 10 && z < 3) ‘. Factoring out ‘ x > 10 ‘ gives ‘ x > 10 && (y > 5 || z < 3) ‘. This simplification reduces redundancy and makes the logic more straightforward without altering the meaning of the expression.
Question 3
Which of the following expressions is always ‘ true ‘ regardless of the value of ‘ x ‘?
A) ‘ x || !x ‘
B) ‘ x && !x ‘
C) ‘ !x || !x ‘
D) ‘ x && x ‘
Answer: A) ‘ x || !x ‘
Explanation: The expression ‘ x || !x ‘ is always ‘ true ‘ because, for any Boolean value of ‘ x ‘, either ‘ x ‘ is ‘ true ‘ or its negation ‘ !x ‘ is ‘ true ‘. This is an example of a tautology in Boolean logic, where the expression is true under all possible conditions. Understanding this can help in recognizing when conditions are redundant or can be simplified in programming logic.