In AP Computer Science A, understanding how to define an object’s behavior using methods is key to mastering object-oriented programming. Methods, both instance and static, are essential for manipulating and accessing data within classes. Particularly, static methods, exemplified by the Java Math class, perform tasks independent of class instances, offering universally accessible functionalities. This concept not only enhances modularity and reuse of code but also plays a crucial role in designing efficient and manageable software solutions.
Learning Objectives
For the AP Computer Science A Exam, focus on understanding how to define and utilize methods to dictate an object’s behavior in Java. Learn to differentiate between instance methods and static methods, and know when to use each. Grasp the concept of method overloading and how it enhances flexibility in programming. Familiarize yourself with the Java Math class, emphasizing its static methods for common mathematical operations. Practice implementing and invoking both types of methods to develop a strong foundation in object-oriented programming principles.
Defining Object Behavior with Methods
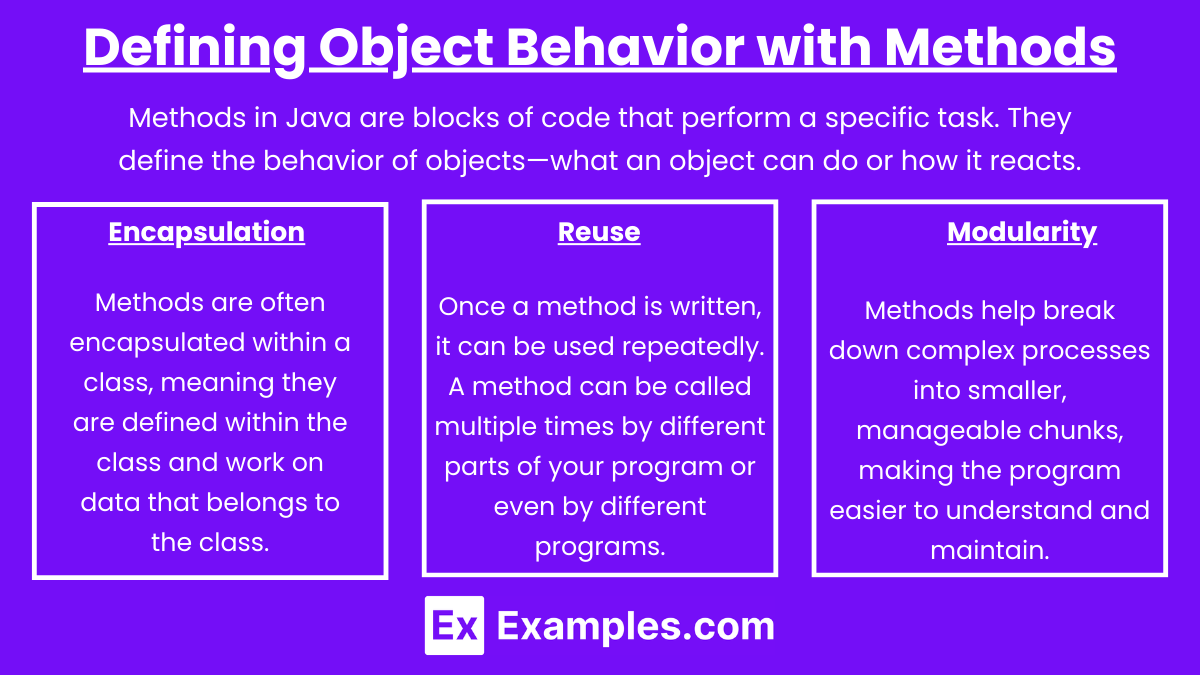
Methods in Java are blocks of code that perform a specific task. They define the behavior of objects—what an object can do or how it reacts. Here’s how methods contribute to object behavior:
- Encapsulation: Methods are often encapsulated within a class, meaning they are defined within the class and work on data that belongs to the class. This keeps the data safe from outside interference and misuse.
- Reuse: Once a method is written, it can be used repeatedly. A method can be called multiple times by different parts of your program or even by different programs.
- Modularity: Methods help break down complex processes into smaller, manageable chunks, making the program easier to understand and maintain.
Example:
public class Dog {
// Instance variables
private String name;
// Constructor
public Dog(String name) {
this.name = name;
}
// Method to describe barking behavior
public void bark() {
System.out.println(name + " barks.");
}
}
// Creating and using an object of Dog class
Dog myDog = new Dog("Rex");
myDog.bark(); // Output: Rex barks.
2. Static Methods
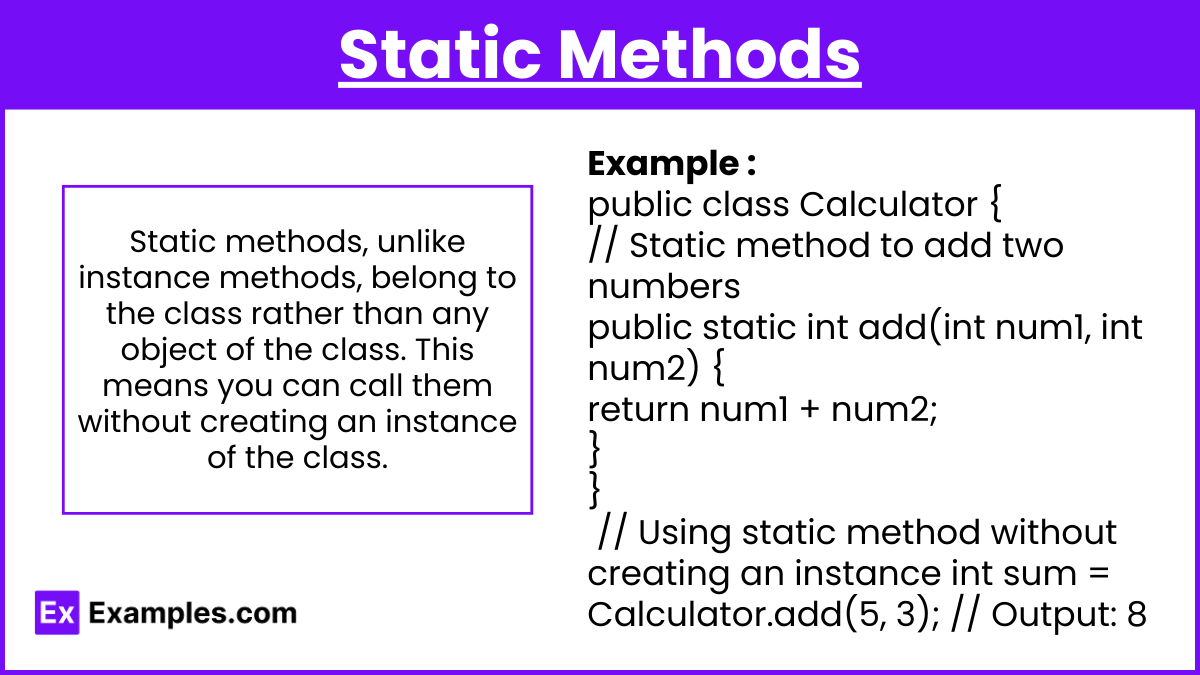
Static methods, unlike instance methods, belong to the class rather than any object of the class. This means you can call them without creating an instance of the class.
- Utility functions: Static methods are often used for operations that don’t require any data from instances of the class.
- Global access: Since static methods belong to the class, they can be accessed globally using the class name, which is useful for utility or helper methods.
Example:
public class Calculator {
// Static method to add two numbers
public static int add(int num1, int num2) {
return num1 + num2;
}
}
// Using static method without creating an instance
int sum = Calculator.add(5, 3); // Output: 8
3. The Math Class
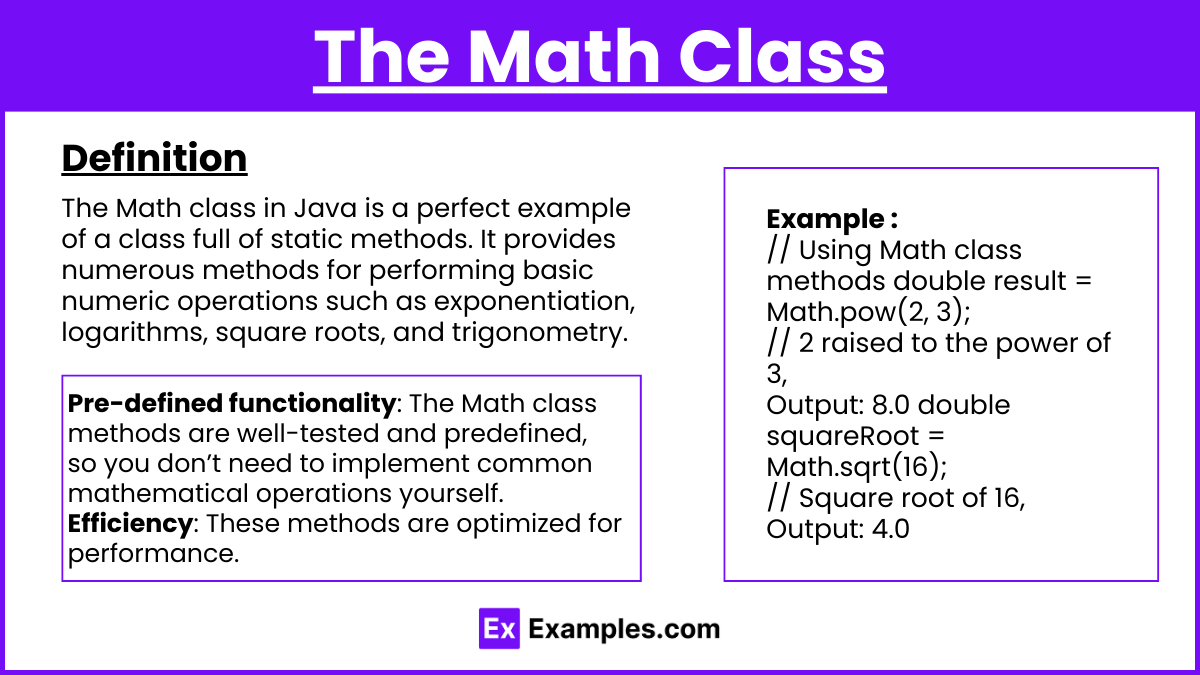
The Math class in Java is a perfect example of a class full of static methods. It provides numerous methods for performing basic numeric operations such as exponentiation, logarithms, square roots, and trigonometry.
- Pre-defined functionality: The Math class methods are well-tested and predefined, so you don’t need to implement common mathematical operations yourself.
- Efficiency: These methods are optimized for performance.
Example:
// Using Math class methods
double result = Math.pow(2, 3); // 2 raised to the power of 3, Output: 8.0
double squareRoot = Math.sqrt(16); // Square root of 16, Output: 4.0
Examples
Example 1: Vehicle Speed Calculation
A class Vehicle can include methods to manipulate or retrieve vehicle properties. For instance, a method calculateSpeed might determine the speed based on distance and time parameters. Additionally, a static method could be used to convert speed units from kilometers per hour to miles per hour.
public class Vehicle {
private double distanceTravelled; // in kilometers
private double timeTaken; // in hours
public Vehicle(double distance, double time) {
this.distanceTravelled = distance;
this.timeTaken = time;
}
public double calculateSpeed() {
return distanceTravelled / timeTaken;
}
public static double convertToMilesPerHour(double speedKmHr) {
return speedKmHr * 0.621371;
}
}
Vehicle car = new Vehicle(100, 1.5);
double speed = car.calculateSpeed(); // Output: 66.67 km/hr
double speedMph = Vehicle.convertToMilesPerHour(speed); // Output: 41.45 mph
Example 2: Bank Account Management
In a ‘ BankAccount ‘ class, methods like deposit and withdraw define how an account handles transactions. A static method could calculate interest on a given balance using a standard interest rate, encapsulating this common functionality.
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
balance -= amount;
}
public static double calculateInterest(double balance, double annualRate) {
return balance * annualRate / 100;
}
}
BankAccount account = new BankAccount(2000);
account.deposit(500); // New balance: 2500
double interest = BankAccount.calculateInterest(account.getBalance(), 5); // Annual interest: 125
Example 3: Temperature Converter
A class TemperatureConverter might include static methods to convert between Celsius and Fahrenheit. This allows easy temperature conversion without needing to instantiate the class.
public class TemperatureConverter {
public static double celsiusToFahrenheit(double celsius) {
return (celsius * 9/5) + 32;
}
public static double fahrenheitToCelsius(double fahrenheit) {
return (fahrenheit - 32) * 5/9;
}
}
double tempF = TemperatureConverter.celsiusToFahrenheit(25); // Output: 77.0 F
double tempC = TemperatureConverter.fahrenheitToCelsius(77); // Output: 25.0 C
Example 4: Circle Operations
A Circle class could define methods to compute the circumference and area. Here, static methods from the Math class are used to calculate these values based on the radius.
public class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
public double getCircumference() {
return 2 * Math.PI * radius;
}
}
Circle circle = new Circle(5);
double area = circle.getArea(); // Output: 78.54
double circumference = circle.getCircumference(); // Output: 31.42
Example 5: Random Number Generator
A utility class Randomizer might encapsulate the behavior of generating different types of random numbers. A static method could leverage Math.random() to generate a random integer within a specified range.
public class Randomizer {
public static int getRandomNumber(int min, int max) {
return (int) (Math.random() * (max - min + 1) + min);
}
}
int randomNumber = Randomizer.getRandomNumber(1, 100); // Random number between 1 and 100
Multiple Choice Questions
Question 1
What is the main characteristic of a static method in Java?
A) It must return a value.
B) It can be called without creating an instance of the class.
C) It can only access static variables.
D) It cannot use any method parameters.
Correct Answer: B) It can be called without creating an instance of the class.
Explanation: Static methods belong to the class, not to any instance of the class. This allows them to be called using the class name without needing to create an object of the class. While static methods can indeed access only static variables directly (choice C), this characteristic is not their main defining feature but rather a limitation. Choices A and D are incorrect because static methods can return void (i.e., no value), and they can use parameters just like instance methods.
Question 2
Which of the following is a correct use of the Math class in Java?
A) Math m = new Math();
B) double result = Math.sqrt(16.0);
C) Math.random(1, 50);
D) int result = Math.addExact(5, 10);
Correct Answer: B) double result = Math.sqrt(16.0);
Explanation: The Math class in Java contains static methods and does not have a public constructor, so it cannot be instantiated (choice A is incorrect). Choice B is correct because Math.sqrt() is a static method that calculates the square root of a number, and it is used correctly here. Choice C is incorrect because Math.random() does not take any parameters and only returns a double value between 0.0 and 1.0. Choice D uses a valid method, Math.addExact(), but the question prompt and the context imply basic operations like square root, not integer-specific methods, though technically this could also be seen as correct if not for the specific context of simpler Math class examples.
Question 3
Why would a developer use static methods in a utility class?
A) To allow for data modification in individual objects.
B) To use the methods without needing to instantiate the class.
C) Because static methods execute faster than instance methods.
D) To access non-static fields of the class.
Correct Answer: B) To use the methods without needing to instantiate the class.
Explanation: Static methods are often used in utility classes to provide functionality that does not depend on the state of an object instance, allowing the methods to be accessed directly by using the class name (choice B). This makes utility functions like mathematical calculations or conversions easily accessible throughout an application without the overhead of object creation. Choice A is incorrect as static methods cannot modify instance-specific data. Choice C is misleading; static methods do not inherently execute faster than instance methods—their performance benefit comes from not requiring an object instance. Choice D is incorrect because static methods cannot directly access non-static (instance) fields of a class.