In AP Computer Science A, defining and overriding methods within subclasses and superclasses is essential for mastering object-oriented programming. A superclass defines common methods that can be inherited by multiple subclasses. Subclasses can either use these inherited methods or override them to provide more specific behavior, allowing for more tailored functionality. This process enables polymorphism, where a subclass method is dynamically called based on the object type at runtime, promoting code reusability and flexibility in managing complex systems and behaviors efficiently.
Learning Objectives
For the topic “Defining and Overriding Methods Within Subclasses and Superclasses” in AP Computer Science A, you should focus on understanding how to define methods in superclasses and subclasses, how to override methods to provide specific behavior, and the concept of polymorphism. Learn how to use the super keyword to invoke superclass methods, prevent overriding with final, and distinguish between method overloading and overriding. Grasp how abstract methods enforce subclass-specific implementations and recognize the role of access modifiers in inheritance.
Defining and Overriding Methods Within Subclasses and Superclasses for AP Computer Science A
Superclasses and Subclasses
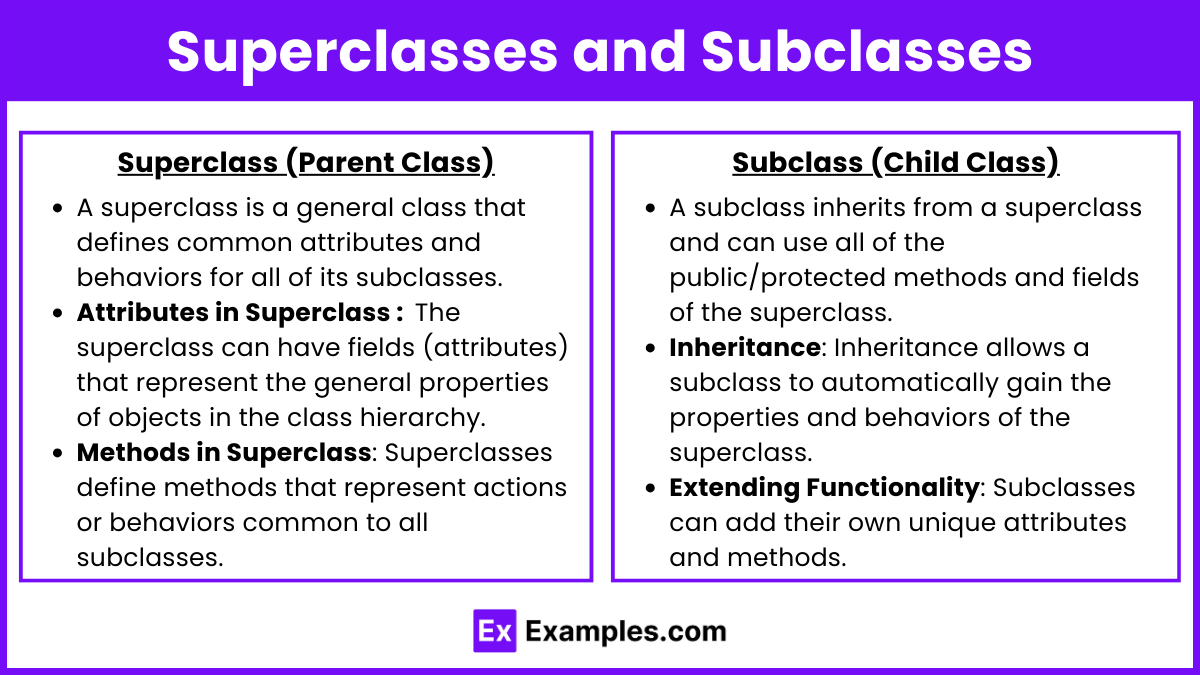
Superclass (Parent Class): A superclass is a general class that defines common attributes and behaviors for all of its subclasses. It represents the more generic version of objects. A superclass can be thought of as the generalized version of a class hierarchy, encapsulating common functionality.
- Attributes in Superclass: The superclass can have fields (attributes) that represent the general properties of objects in the class hierarchy. These fields are usually accessible to the subclass depending on their access modifiers (public, protected, or private).
- Methods in Superclass: Superclasses define methods that represent actions or behaviors common to all subclasses. These methods can be inherited by subclasses and can be directly used, or overridden (as described earlier) for more specialized behavior.
- Abstract Superclass: Sometimes, superclasses are abstract, meaning they cannot be instantiated on their own but serve as a blueprint for subclasses. Abstract superclasses may contain abstract methods, which must be implemented by subclasses.
Subclass (Child Class): A subclass inherits from a superclass and can use all of the public/protected methods and fields of the superclass. Additionally, it can define its own methods and fields, or even modify the inherited ones to provide specific functionality. The subclass may also override methods from the superclass to modify or extend their behavior.
- Inheritance: Inheritance allows a subclass to automatically gain the properties and behaviors of the superclass. This promotes code reuse, reducing redundancy.
- Extending Functionality: Subclasses can add their own unique attributes and methods. They can also override superclass methods to provide more specific functionality suited to the subclass’s purpose.
- Constructor Inheritance: While constructors are not inherited directly, a subclass can call the superclass’s constructor using super(), which is essential when the superclass constructor has parameters that must be initialized.
Method Definition
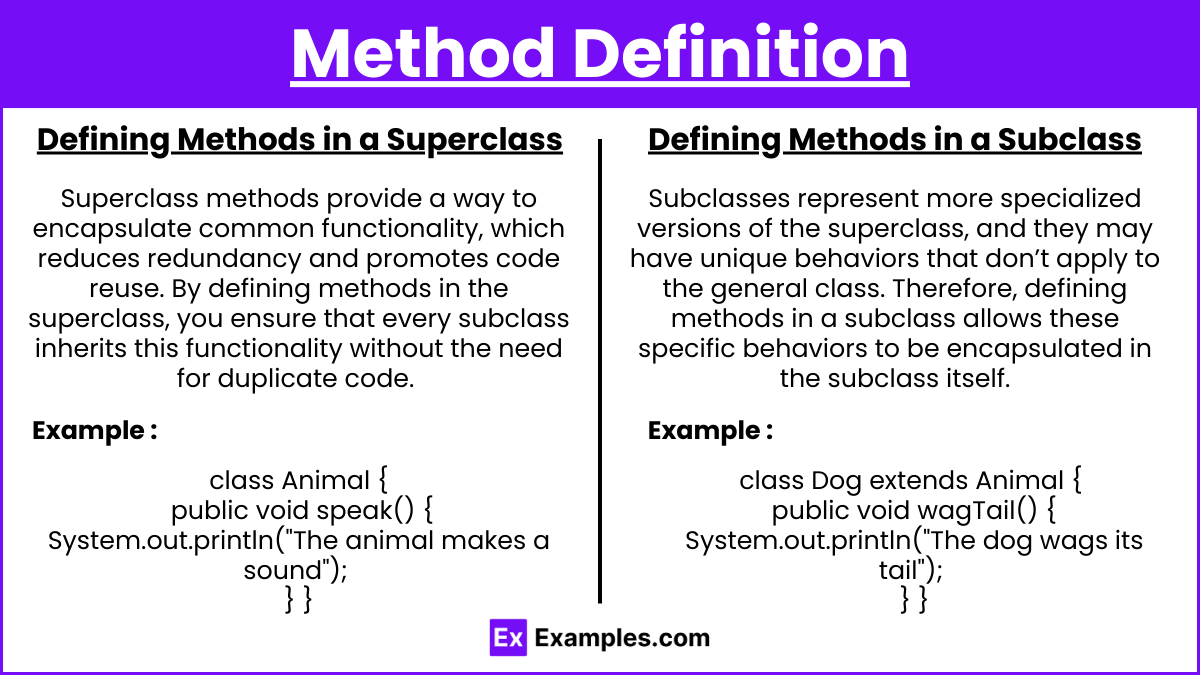
Defining Methods in a Superclass: Superclass methods provide a way to encapsulate common functionality, which reduces redundancy and promotes code reuse. By defining methods in the superclass, you ensure that every subclass inherits this functionality without the need for duplicate code. In a superclass, you define methods that will be common to all subclasses.
For example:
class Animal {
public void speak() {
System.out.println("The animal makes a sound");
}
}
In this example, speak() is a method defined in the superclass Animal and can be used by any subclass that inherits from Animal.
Defining Methods in a Subclass: Subclasses represent more specialized versions of the superclass, and they may have unique behaviors that don’t apply to the general class. Therefore, defining methods in a subclass allows these specific behaviors to be encapsulated in the subclass itself. Subclasses can define their own methods that are not present in the superclass.
For instance:
class Dog extends Animal {
public void wagTail() {
System.out.println("The dog wags its tail");
}
}
Here, Dog is a subclass of Animal and it defines a new method, wagTail(), which is not present in the superclass.
Method Overriding in Subclasses
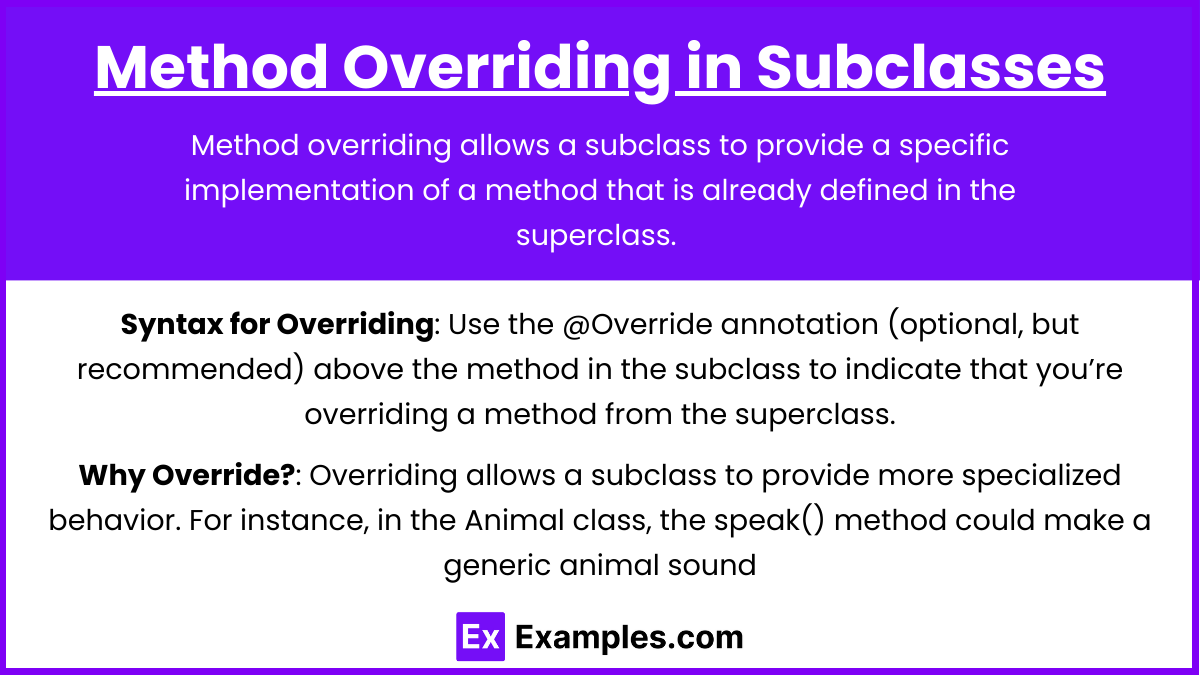
Method overriding allows a subclass to provide a specific implementation of a method that is already defined in the superclass. When a method is overridden, the subclass’s version of the method is used, even when the object is referred to as an instance of the superclass. Method overriding occurs when a subclass provides its own implementation of a method that is already defined in the superclass. This allows the subclass to offer more specific behavior. The overridden method in the subclass must have the same name, return type, and parameter list as the method in the superclass.
Syntax for Overriding: Use the @Override annotation (optional, but recommended) above the method in the subclass to indicate that you’re overriding a method from the superclass.The method signature must be exactly the same as the superclass method.
Example:
class Dog extends Animal {
@Override
public void speak() {
System.out.println("The dog barks");
}
}
Why Override?: Overriding allows a subclass to provide more specialized behavior. For instance, in the Animal class, the speak() method could make a generic animal sound, but in the Dog subclass, overriding allows the method to make a barking sound.
Polymorphism and Overriding
Polymorphism: A key concept related to method overriding is polymorphism, which allows one object to be treated as an instance of a parent class. Polymorphism enables dynamic method dispatch, where the correct overridden method in a subclass is called at runtime, even if the object is referenced by a superclass type. Polymorphism enables generic programming, allowing you to write more flexible and maintainable code. For instance, you can work with objects of different types through a common interface or superclass, without knowing the specific type at compile time.
Example:
Animal myDog = new Dog();
myDog.speak(); // Outputs: The dog barks
Here, even though myDog is declared as an Animal, at runtime, the speak() method of Dog is called due to method overriding.
Overloading vs. Overriding
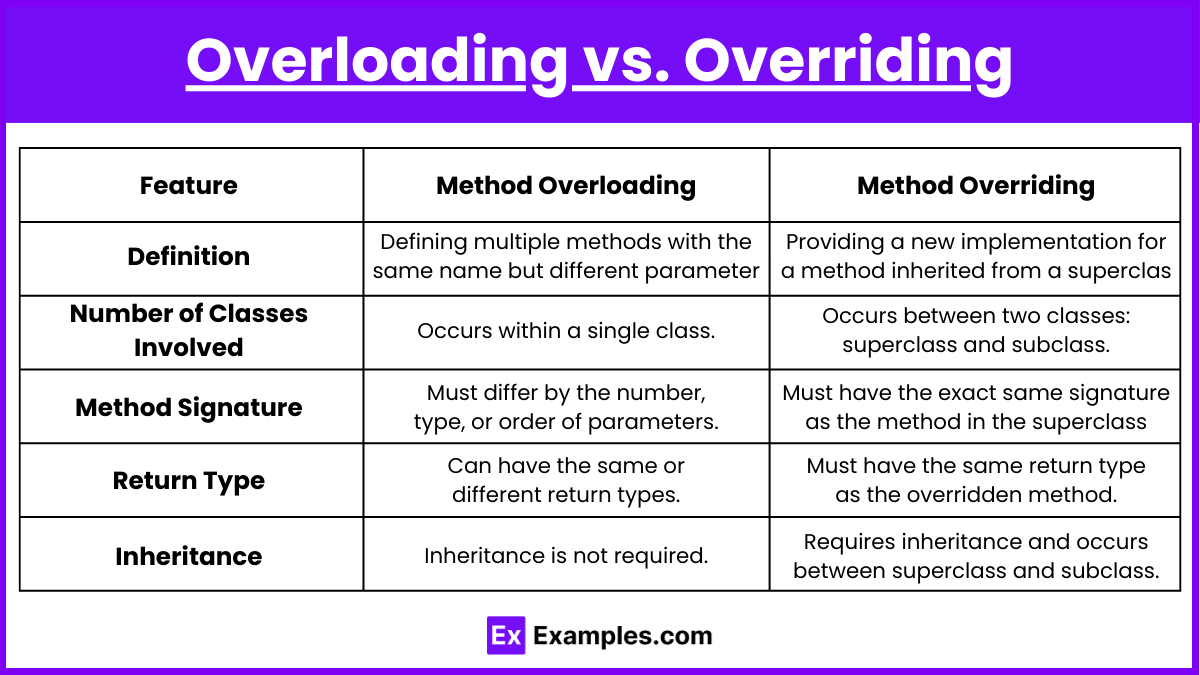
Feature | Method Overloading | Method Overriding |
---|---|---|
Definition | Defining multiple methods with the same name but different parameter lists within the same class. | Providing a new implementation for a method inherited from a superclass, while maintaining the same method signature. |
Number of Classes Involved | Occurs within a single class. | Occurs between two classes: superclass and subclass. |
Method Signature | Must differ by the number, type, or order of parameters. | Must have the exact same signature as the method in the superclass (same name, return type, and parameters). |
Return Type | Can have the same or different return types. | Must have the same return type as the overridden method. |
Inheritance | Inheritance is not required. | Requires inheritance and occurs between superclass and subclass. |
Use Case | Typically used when a class needs to perform similar tasks with different types of data or a different number of arguments. | Used when the subclass needs to provide a specific implementation for a method defined in the superclass |
Examples
Example 1: Animal and Dog Class
In this example, the superclass Animal defines a method speak() that prints a generic message, “The animal makes a sound.” In the subclass Dog, the speak() method is overridden to print “The dog barks.” This allows the Dog class to provide its specific behavior for the speak() method while still maintaining the structure defined by the Animal superclass.
Example 2: Vehicle and Car Class
The Vehicle class is the superclass that defines a method move() that prints “The vehicle moves.” The Car subclass overrides the move() method to print “The car drives on the road.” This is an example of a subclass overriding a method to specify its behavior for a general action like moving, making it more relevant to the type of vehicle.
Example 3: Shape and Circle Class
In the Shape superclass, a method draw() is defined that prints “Drawing a shape.” The subclass Circle overrides this method to print “Drawing a circle.” This example highlights how subclasses can customize the behavior of a method that is generic in the superclass, allowing each subclass to represent specific shapes.
Example 4: Employee and Manager Class
In the Employee superclass, a method work() is defined to print “The employee works.” The subclass Manager overrides the work() method to print “The manager oversees the team.” In this case, the subclass modifies the inherited behavior to reflect the different role of a manager compared to a general employee.
Example 5: Phone and Smartphone Class
In the Phone superclass, a method call() is defined to print “Making a call.” The subclass Smartphone overrides this method to print “Making a video call.” Here, the subclass builds on the general concept of calling from the superclass but introduces a more specific and modern behavior, reflecting the capabilities of a smartphone.
Multiple Choice Questions
Question 1
What happens if a subclass does not override a method from its superclass?
A. The method will not be available in the subclass.
B. The method will behave according to the implementation in the superclass.
C. The subclass will produce an error at runtime.
D. The method will become abstract in the subclass.
Answer: B. The method will behave according to the implementation in the superclass.
Explanation: If a subclass does not override a method from its superclass, the method remains available in the subclass and behaves exactly as it does in the superclass. This is the default behavior of inheritance in Java. Method overriding is only necessary when the subclass needs to change or customize the method’s behavior.
Question 2
Consider the following code snippet. What will be the output?
class Animal {
public void speak() {
System.out.println("The animal makes a sound");
}
}
class Dog extends Animal {
@Override
public void speak() {
System.out.println("The dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.speak();
}
}
A. The program will not compile because myDog is of type Animal.
B. The program will print “The animal makes a sound”.
C. The program will print “The dog barks”.
D. The program will produce a runtime error.
Answer: C. The program will print “The dog barks”.
Explanation: Although myDog is declared as an Animal, it is instantiated as a Dog. Due to polymorphism, Java calls the speak() method of the actual object type, which in this case is Dog. Since the Dog class overrides the speak() method, the program will print “The dog barks”.
Question 3
Which of the following correctly describes method overriding?
A. Overriding allows multiple methods with the same name but different parameter lists in the same class.
B. Overriding requires the method in the subclass to have a different name than the method in the superclass.
C. Overriding allows a subclass to provide its own implementation of a method that exists in its superclass.
D. Overriding is only possible if the method in the superclass is declared as private.
Answer: C. Overriding allows a subclass to provide its own implementation of a method that exists in its superclass.
Explanation: Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. The method must have the same name, return type, and parameters. Overriding does not involve multiple methods with different parameter lists (that’s method overloading) and cannot happen if the superclass method is private, as private methods are not inherited by subclasses.