In AP Computer Science A, defining the behaviors of an object is essential for creating functional and efficient programs. This is done through non-void, void, and static methods. Void methods perform actions without returning values, non-void methods return specific values to the caller, and static methods belong to the class rather than an instance, making them useful for general-purpose tasks. Understanding how to implement and use these methods effectively is key to mastering object-oriented programming concepts and excelling in the exam.
Learning Objectives
For the topic “Defining Behaviors of an Object Using non-void, void, and Static Methods,” in AP Computer Science A, you should focus on learning how to design methods that perform specific actions (void methods), return values (non-void methods), and handle tasks without needing object instances (static methods). Understand how to use return types and parameters to structure method behavior, and apply these concepts to solve programming problems. Additionally, practice distinguishing when to use instance methods versus static methods to optimize code functionality in object-oriented programming.
In AP Computer Science A, understanding how to define the behaviors of an object using non-void, void, and static methods is critical for writing robust and efficient code. Here’s a detailed breakdown of each type of method and how it defines an object’s behavior:
Void Methods
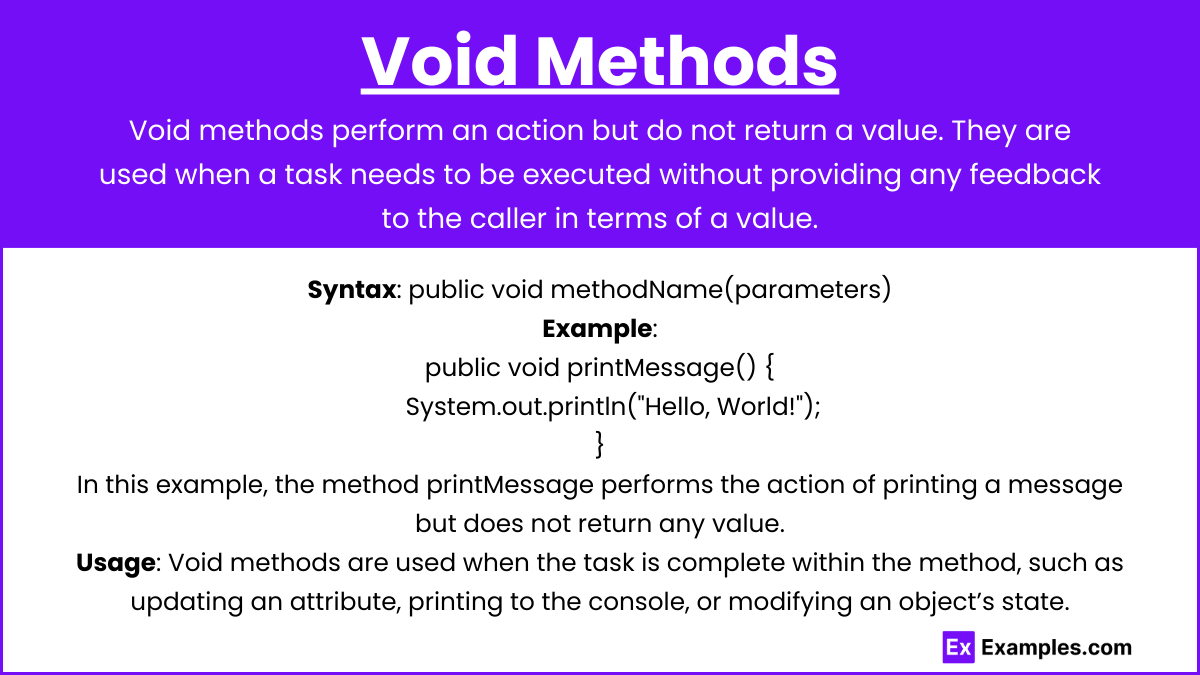
Definition: Void methods perform an action but do not return a value. They are used when a task needs to be executed without providing any feedback to the caller in terms of a value.
Syntax: public void methodName(parameters)
Example:
public void printMessage() {
System.out.println("Hello, World!");
}
In this example, the method printMessage performs the action of printing a message but does not return any value.
Usage: Void methods are used when the task is complete within the method, such as updating an attribute, printing to the console, or modifying an object’s state.
Non-Void Methods
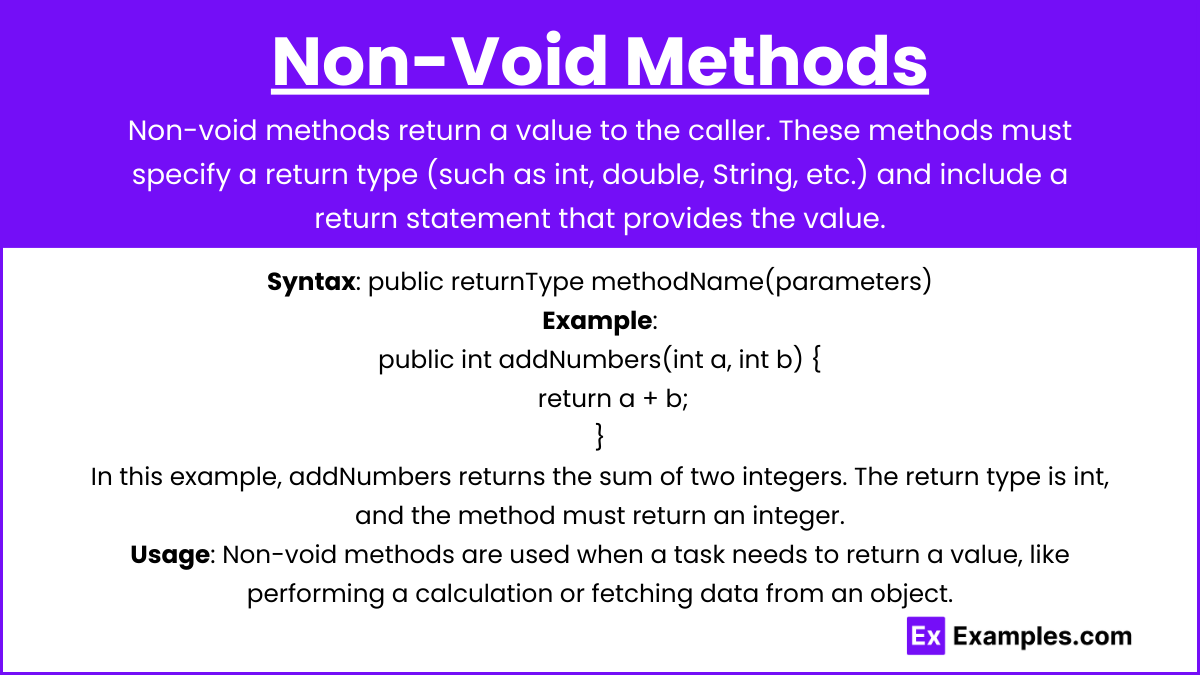
Definition: Non-void methods return a value to the caller. These methods must specify a return type (such as int, double, String, etc.) and include a return statement that provides the value.
Syntax: public returnType methodName(parameters)
Example:
public int addNumbers(int a, int b) {
return a + b;
}
In this example, addNumbers returns the sum of two integers. The return type is int, and the method must return an integer.
Usage: Non-void methods are used when a task needs to return a value, like performing a calculation or fetching data from an object.
Static Methods
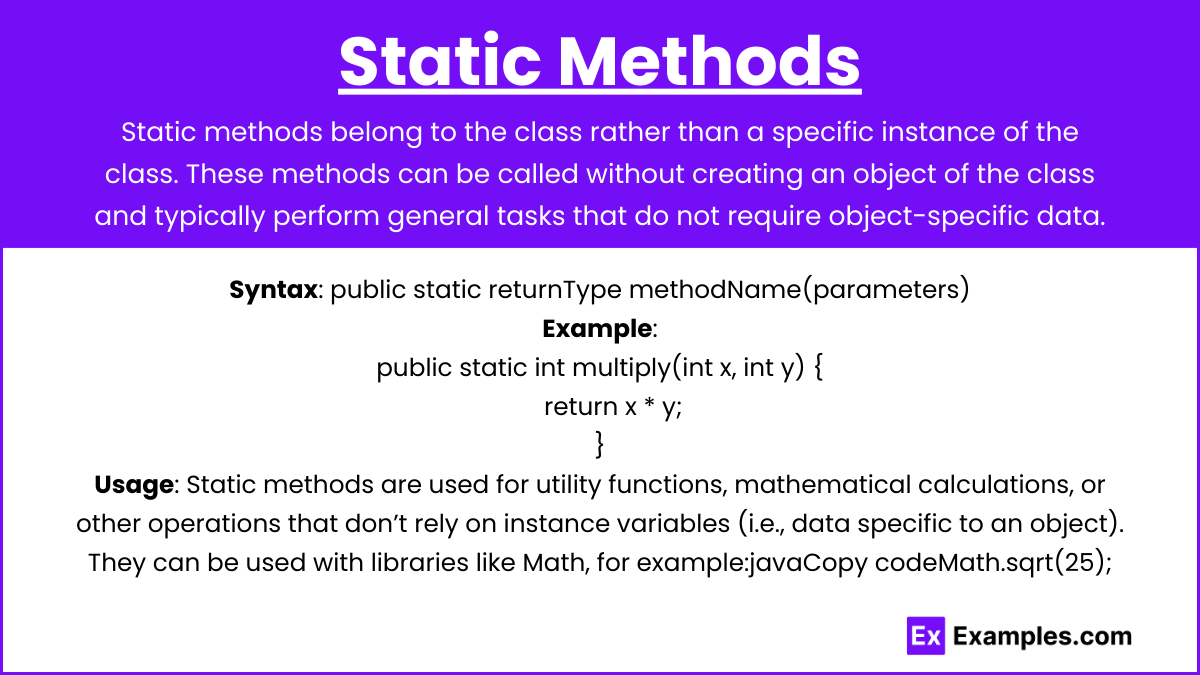
Definition: Static methods belong to the class rather than a specific instance of the class. These methods can be called without creating an object of the class and typically perform general tasks that do not require object-specific data.
Syntax: public static returnType methodName(parameters)
Example:
public static int multiply(int x, int y) {
return x * y;
}
In this example, multiply is a static method that multiplies two integers. It can be called using the class name, e.g., ClassName.multiply(5, 3).
Usage: Static methods are used for utility functions, mathematical calculations, or other operations that don’t rely on instance variables (i.e., data specific to an object). They can be used with libraries like Math, for example:javaCopy codeMath.sqrt(25);
Examples
Example 1: Void Method: Updating Object State
Consider a BankAccount class where you define a void method deposit to update the balance of an account. The method doesn’t return anything; it simply modifies the object’s state. For example:
public void deposit(double amount) {
balance += amount;
}
This deposit method adjusts the balance attribute of the BankAccount object but does not return any value to the caller.
Example 2: Non-Void Method: Returning Object Information
In the same BankAccount class, you can have a non-void method getBalance that returns the current balance. This method allows the caller to retrieve the account’s balance:
public double getBalance() {
return balance;
}
The method returns a double representing the balance, allowing external access to the object’s information without modifying it.
Example 3: Static Method: Utility Function for Interest Calculation
You can create a static method in the BankAccount class to calculate interest rates for different account types, without needing to access an object’s instance variables. For example:
public static double calculateInterest(double balance, double rate) {
return balance * rate;
}
This static method can be called directly using the class name (e.g., BankAccount.calculateInterest(1000, 0.05)), making it useful for general-purpose calculations not tied to a specific object.
Example 4: Void Method: Displaying Object Details
A void method displayAccountInfo in the BankAccount class could be used to print details of the account to the console. The method would perform the action of displaying information but not return any values:
public void displayAccountInfo() {
System.out.println("Account Number: " + accountNumber);
System.out.println("Balance: " + balance);
}
This method defines the behavior of presenting the object’s details without altering or returning any information.
Example 5: Non-Void Method: Returning a Boolean Value
Suppose you want a method that checks if a BankAccount has enough funds for a withdrawal. A non-void method hasSufficientFunds could return a boolean (true or false) indicating if the withdrawal is possible:
public boolean hasSufficientFunds(double withdrawalAmount) {
return balance >= withdrawalAmount;
}
This method returns a boolean, defining a behavior that allows the object to report its ability to perform an action (withdrawal) based on its state.
Multiple Choice Questions
Question 1:
Which of the following best describes a void method?
A) A method that returns a value of any type
B) A method that performs an action but does not return any value
C) A method that is called using the class name without creating an object
D) A method that always returns a boolean value
Answer: B) A method that performs an action but does not return any value
Explanation: A void method is one that performs an action but does not return any value to the caller. For example, a method that prints something to the console or modifies an object’s state without returning anything is considered a void method. In contrast, a non-void method returns a specific value (e.g., int, boolean, etc.), while static methods (which can also be void or non-void) are associated with the class, not an instance.
Question 2:
Which of the following is true about static methods?
A) Static methods can only be used with void return types
B) Static methods can access instance variables directly
C) Static methods belong to the class and can be called without creating an object
D) Static methods can only be called within the class they are defined in
Answer: C) Static methods belong to the class and can be called without creating an object
Explanation: Static methods are class-level methods that can be invoked using the class name without needing to create an instance of the class. They cannot access instance variables (non-static variables) directly, because instance variables belong to a specific object. Instead, static methods are often used for utility or helper functions that don’t rely on the state of an object. The statement that static methods can only be called within the class they are defined in is incorrect—they can be called from outside the class using the class name.
Question 3:
What will happen if a non-void method does not include a return statement?
A) The program will run but give incorrect output
B) The method will automatically return 0
C) The program will not compile
D) The method will return null
Answer: C) The program will not compile
Explanation: A non-void method is required to return a value of the type specified in the method signature. If the method does not include a return statement, the program will not compile because Java expects a value to be returned. The absence of a return statement in a non-void method violates this requirement, resulting in a compilation error. Void methods, on the other hand, do not need a return statement, unless used as return; to simply exit the method early.