In AP Computer Science A, executing recursive methods involves writing functions that call themselves to solve problems. Recursion breaks down complex tasks into simpler sub-tasks, utilizing base cases to stop further calls and recursive cases to reduce the problem. Understanding recursion is essential for solving problems like factorials, Fibonacci sequences, and search algorithms. Each call creates a new stack frame, which resolves once the base case is reached, allowing the program to backtrack and complete the task. Mastering recursion is key to efficient problem-solving.
Learning Objectives
When learning about executing recursive methods for AP Computer Science A, focus on understanding how recursion works by identifying base cases and recursive cases in methods. Learn how the call stack operates during recursion and the importance of a proper termination condition. You should be able to trace recursive calls, recognize when recursion is appropriate, and compare recursive and iterative solutions for efficiency. Additionally, practice applying recursion to solve common problems such as factorials, Fibonacci sequences, and search algorithms like binary search.
What are Recursive Methods ?
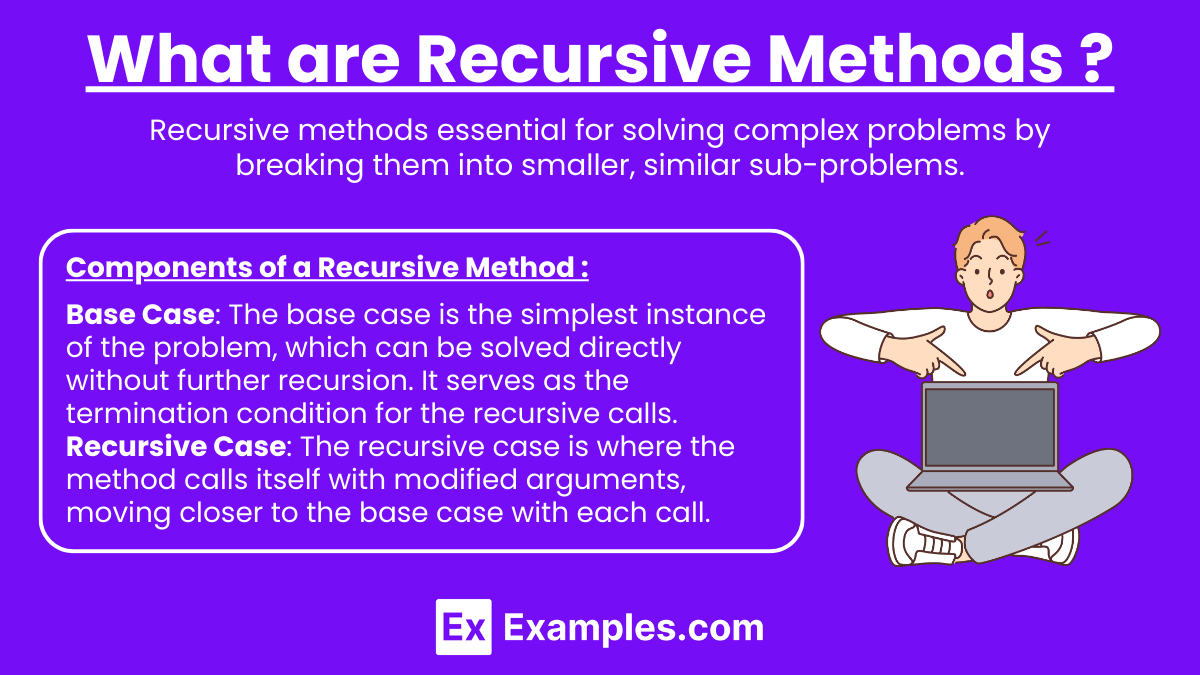
Recursive methods are a fundamental concept in computer science, essential for solving complex problems by breaking them into smaller, similar sub-problems. In recursive methods, a function calls itself repeatedly until it reaches a stopping condition, or base case. This approach is especially useful for problems that can be divided into identical sub-problems.
Components of a Recursive Method:
- Base Case: The base case is the simplest instance of the problem, which can be solved directly without further recursion. It serves as the termination condition for the recursive calls. Without a base case, the recursion would continue indefinitely, leading to a stack overflow.
- Recursive Case: The recursive case is where the method calls itself with modified arguments, moving closer to the base case with each call. It continues to break the problem down into smaller sub-problems until the base case is met.
Steps to Execute a Recursive Method
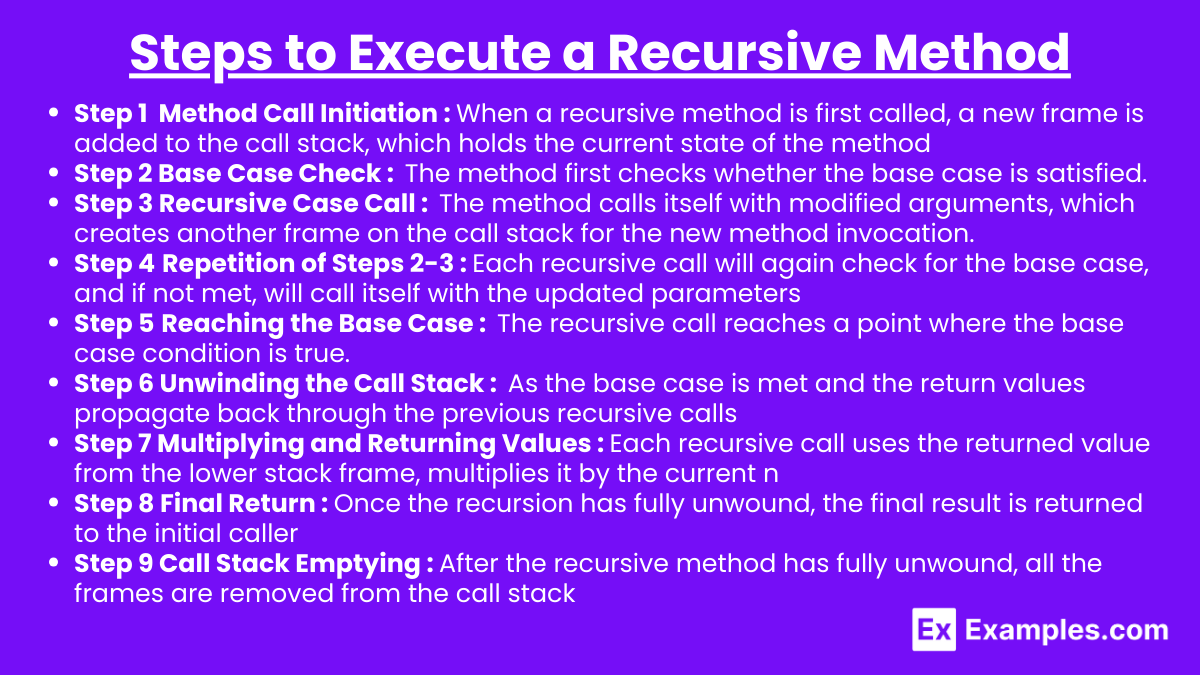
- Method Call Initiation:
- When a recursive method is first called, a new frame is added to the call stack, which holds the current state of the method (parameters, local variables, return address).
- Example: factorial(4) is called, a frame is created and added to the call stack, and the function checks whether n == 1.
- Base Case Check:
- The method first checks whether the base case is satisfied. If so, it returns the corresponding result and unwinds the recursion. If not, the method proceeds to the recursive case.
- For factorial(4), since n is not 1, the method moves to the recursive case and calculates 4 * factorial(3).
- Recursive Case Call:
- The method calls itself with modified arguments, which creates another frame on the call stack for the new method invocation.
- In the case of factorial(4), it calls factorial(3) and pushes it onto the call stack.
- Repetition of Steps 2-3:
- Each recursive call will again check for the base case, and if not met, will call itself with the updated parameters, thus continuing to add frames to the call stack.
- For factorial(3), the call checks n == 1 (false) and moves on to call factorial(2).
- Reaching the Base Case:
- Eventually, the recursive call reaches a point where the base case condition is true. For factorial, this is when n == 1.
- When factorial(1) is called, it meets the base case and returns 1 without making another recursive call. This return value starts the process of unwinding the call stack.
- Unwinding the Call Stack:
- As the base case is met and the return values propagate back through the previous recursive calls, the call stack begins to shrink.
- The result of factorial(1) (which is 1) is returned to the factorial(2) call, which multiplies it by 2 to give 2, and returns it to the factorial(3) call.
- Multiplying and Returning Values:
- Each recursive call uses the returned value from the lower stack frame, multiplies it by the current n, and then returns this new result up the call stack.
- This process continues:
- factorial(2) returns 2 to factorial(3), which calculates 3 * 2 = 6.
- factorial(3) returns 6 to factorial(4), which calculates 4 * 6 = 24.
- Final Return:
- Once the recursion has fully unwound, the final result is returned to the initial caller. In the case of factorial(4), the final result 24 is returned to the main method or point where factorial(4) was first invoked.
- Call Stack Emptying:
- After the recursive method has fully unwound, all the frames are removed from the call stack, and the stack returns to its state prior to the initial method invocation.
Key Considerations for Recursive Methods
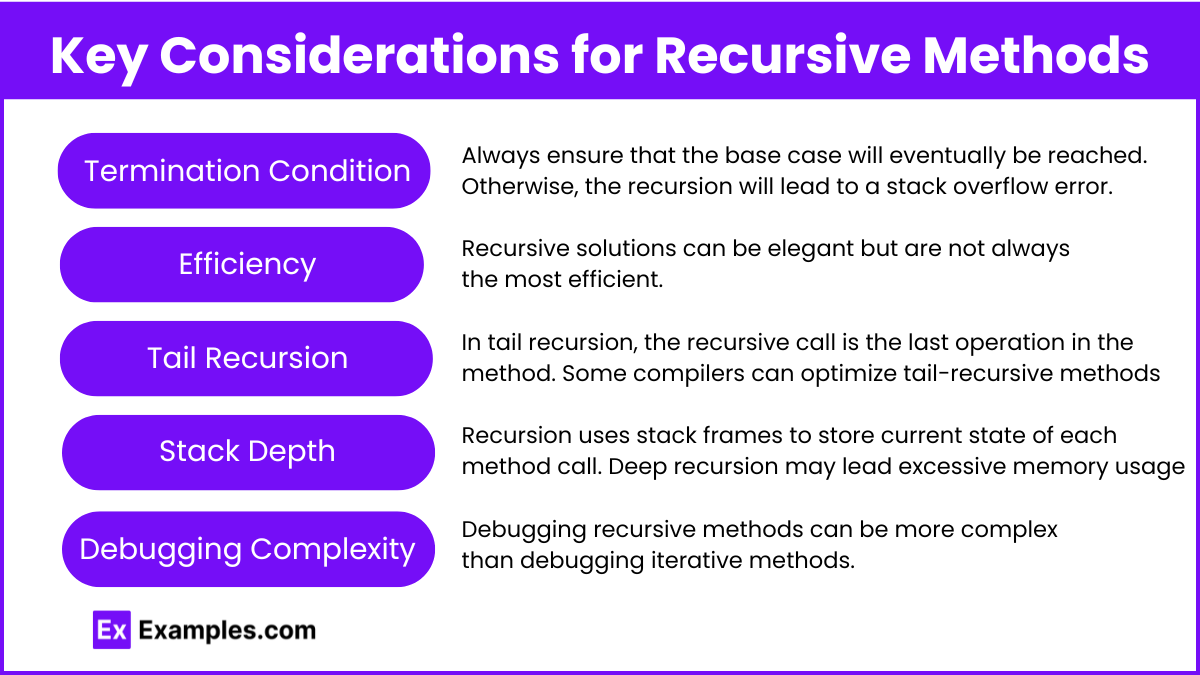
- Termination Condition: Always ensure that the base case will eventually be reached. Otherwise, the recursion will lead to a stack overflow error.
- Efficiency: Recursive solutions can be elegant but are not always the most efficient. Some problems have better iterative solutions to avoid excessive memory usage due to deep recursion.
- Tail Recursion: In tail recursion, the recursive call is the last operation in the method. Some compilers can optimize tail-recursive methods to avoid adding new frames to the call stack, improving performance.
- Stack Depth: Recursion uses stack frames to store the current state of each method call. Deep recursion may lead to excessive memory usage and eventually cause a stack overflow if the recursion depth exceeds the available stack space. Iterative methods may avoid this issue by not relying on the call stack.
- Debugging Complexity: Debugging recursive methods can be more complex than debugging iterative methods. Tracing recursive calls, especially with deep or mutually recursive functions, can be difficult. Using tools like print statements or debuggers with call stack inspection can help trace recursive execution.
Examples
Example 1: Factorial Calculation
A classic example of recursion is calculating the factorial of a number. The factorial of a number nnn, written as n!, is the product of all positive integers up to nnn. A recursive method solves this by multiplying nnn with the factorial of n−1, continuing until the base case of n = 1 is reached. For example, factorial(4) recursively calls factorial(3), factorial(2), and so on, until factorial(1) returns 1, at which point the results are multiplied to get 24.
Example 2: Fibonacci Sequence
The Fibonacci sequence is another common example where recursion shines. In the Fibonacci sequence, each number is the sum of the two preceding ones, starting from 0 and 1. The recursive function calls itself to compute the Fibonacci number at position nnn by summing the values of the function at n-1 and n-2, with the base case being n = 0 or n = 1. For instance, to find fibonacci(5), the function calls fibonacci(4) and fibonacci(3) recursively until reaching the base cases.
Example 3: Binary Search
Binary search is a highly efficient algorithm for finding an element in a sorted array. The recursive approach divides the array into halves and checks whether the middle element is the target. If not, the search continues in the left or right half based on the comparison. This recursive process repeats until the target is found or the array size becomes zero (base case). For example, a recursive binary search on an array of 10 elements would first check the middle and then either search the left half or the right half, significantly reducing the search space.
Example 4: Tower of Hanoi
The Tower of Hanoi is a mathematical puzzle that can be solved using recursion. The goal is to move a set of disks from one peg to another, following specific rules. The recursive solution involves moving smaller subsets of disks between pegs by calling the method recursively on smaller subsets, with the base case being a single disk. The recursive function handles multiple disks by moving n-1 disks from the source to the auxiliary peg, then moving the nnn-th disk to the destination, and finally moving the n-1 disks from the auxiliary peg to the destination.
Example 5: Tree Traversal (Binary Tree)
Recursion is an essential tool for traversing tree data structures, such as binary trees. In a binary tree, each node has up to two children. Recursive tree traversal methods, such as in-order, pre-order, or post-order, visit nodes recursively by first visiting the left subtree, then the node itself, and finally the right subtree (in in-order traversal). The base case occurs when the recursion reaches a leaf node (with no children), after which the recursion unwinds. This recursive approach efficiently handles the hierarchical nature of trees.
Multiple Choice Questions
Question 1
What is the purpose of the base case in a recursive method?
A) To ensure that the recursion continues indefinitely.
B) To provide a stopping condition to prevent infinite recursion.
C) To speed up the recursion process.
D) To make the recursion method consume less memory.
Correct Answer: B) To provide a stopping condition to prevent infinite recursion.
Explanation: The base case is critical in any recursive method because it provides a condition where the recursion stops. Without a base case, the method would continue calling itself indefinitely, eventually causing a stack overflow error. The base case defines the simplest instance of the problem that can be solved without further recursive calls, preventing infinite recursion.
Question 2
Consider the following recursive function:
public int sumOfNumbers(int n) {
if (n == 1) {
return 1;
} else {
return n + sumOfNumbers(n - 1);
}
}
What will be the output of the function call sumOfNumbers(4)?
A) 4
B) 10
C) 15
D) 24
Correct Answer: B) 10
Explanation: The function calculates the sum of integers from 1 to n
. Here’s how it works for sumOfNumbers(4):
- sumOfNumbers(4) = 4 + sumOfNumbers(3)
- sumOfNumbers(3) = 3 + sumOfNumbers(2)
- sumOfNumbers(2) = 2 + sumOfNumbers(1)
- sumOfNumbers(1) = 1 (Base case)
Thus:
- sumOfNumbers(2) = 2 + 1 = 3
- sumOfNumbers(3) = 3 + 3 = 6
- sumOfNumbers(4) = 4 + 6 = 10
So, the result of sumOfNumbers(4) is 10.
Question 3
Which of the following statements is true about recursion?
A) Recursive methods always execute faster than iterative methods.
B) Recursive methods can sometimes lead to stack overflow errors if not properly designed.
C) Recursive methods cannot be used to solve problems involving searching or sorting.
D) Every recursive method must call itself with the same argument in every recursive step.
Correct Answer: B) Recursive methods can sometimes lead to stack overflow errors if not properly designed.
Explanation: Recursive methods can indeed lead to stack overflow errors if they are not designed with a proper base case or if the recursion depth is too deep (i.e., too many recursive calls). Option A is incorrect because recursion does not always execute faster than iteration; in fact, recursion can be slower and consume more memory. Option C is incorrect because recursion is commonly used in algorithms like merge sort, quick sort, and binary search. Option D is incorrect because the argument passed in each recursive call often changes to move toward the base case.