In AP Computer Science A, mastering the use of relational operators to find Boolean values is essential. These operators, such as >, <, >=, <=, ==, and !=, compare values and return a Boolean result—either true or false. Understanding how to manipulate these results with logical operators like &&, ||, and ! is crucial for controlling program flow, making decisions, and validating conditions. This skill is foundational for problem-solving and programming, preparing students to tackle complex scenarios effectively in their coursework and exams.
Learning Objectives
For the topic “Finding Boolean Values with Expressions Involving Relational Operators” in AP Computer Science A, you should aim to master the usage of relational operators (>, <, >=, <=, ==, !=) to compare numeric and character data types. Understand how to construct Boolean expressions and evaluate their truth values in different contexts. Develop skills to apply these operators in control structures such as if statements and loops, enhancing decision-making capabilities in programming. Grasp the integration of relational with logical operators (&&, ||, !) to formulate complex conditions essential for dynamic and conditional program flows.
Finding Boolean Values with Expressions
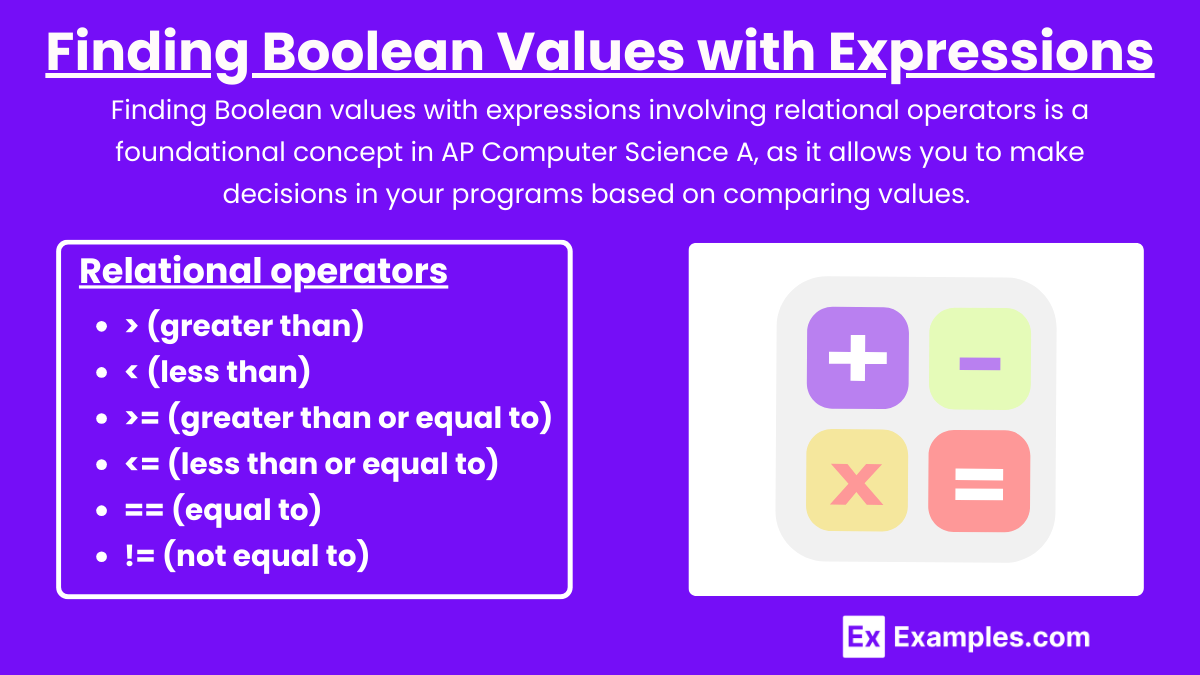
Finding Boolean values with expressions involving relational operators is a foundational concept in AP Computer Science A, as it allows you to make decisions in your programs based on comparing values. Here’s an in-depth look at how this works:
Relational Operators
Relational operators are used to compare two values. Here are the common relational operators in Java, which is the language typically used in AP Computer Science A:
- > (greater than)
- < (less than)
- >= (greater than or equal to)
- <= (less than or equal to)
- == (equal to)
- != (not equal to)
Each of these operators evaluates to a Boolean value (true or false) depending on the outcome of the comparison.
Using Relational Operators
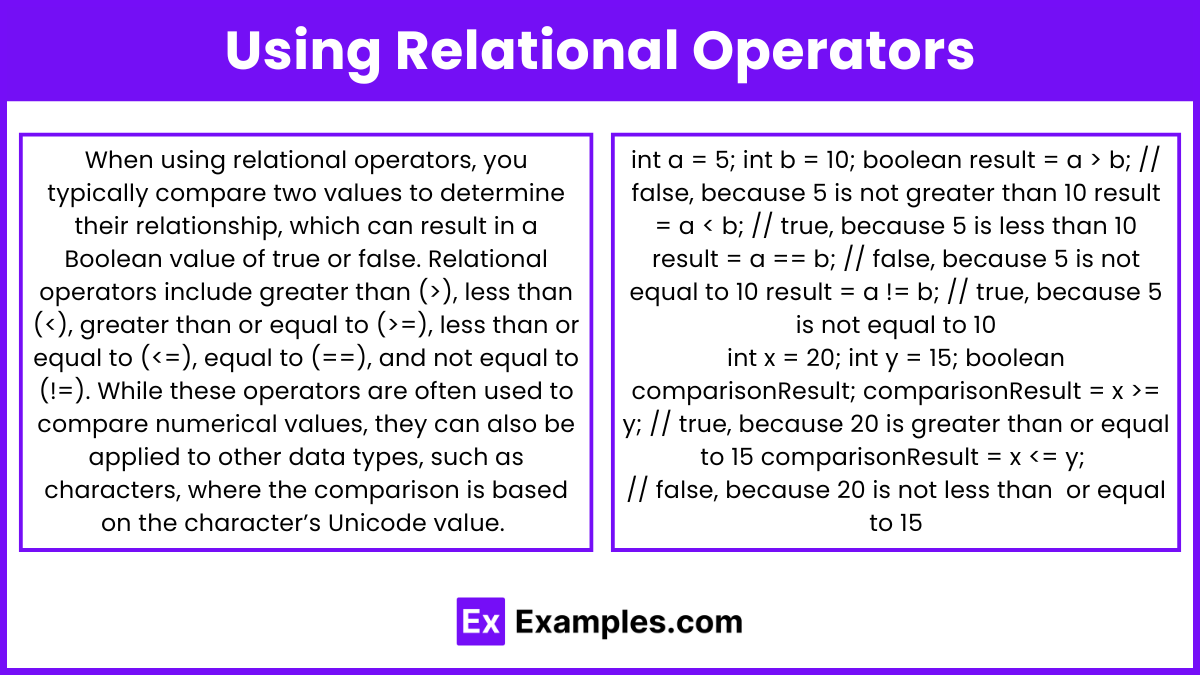
When using relational operators, you typically compare two values to determine their relationship, which can result in a Boolean value of true or false. Relational operators include greater than (>), less than (<), greater than or equal to (>=), less than or equal to (<=), equal to (==), and not equal to (!=). While these operators are often used to compare numerical values, they can also be applied to other data types, such as characters, where the comparison is based on the character’s Unicode value. For example, the expression ‘a’ < ‘b’ evaluates to true because the Unicode value of ‘a’ is less than that of ‘b’. In programming, relational operators are commonly used in conditional statements like if-else to control the flow of execution based on specific conditions. Here are some examples:
int a = 5;
int b = 10;
boolean result = a > b; // false, because 5 is not greater than 10
result = a < b; // true, because 5 is less than 10
result = a == b; // false, because 5 is not equal to 10
result = a != b; // true, because 5 is not equal to 10
int num = 100;
comparisonResult = num != 100; // false, because num is equal to 100
int x = 20;
int y = 15;
boolean comparisonResult;
comparisonResult = x >= y; // true, because 20 is greater than or equal to 15
comparisonResult = x <= y; // false, because 20 is not less than or equal to 15
Combining with Logical Operators
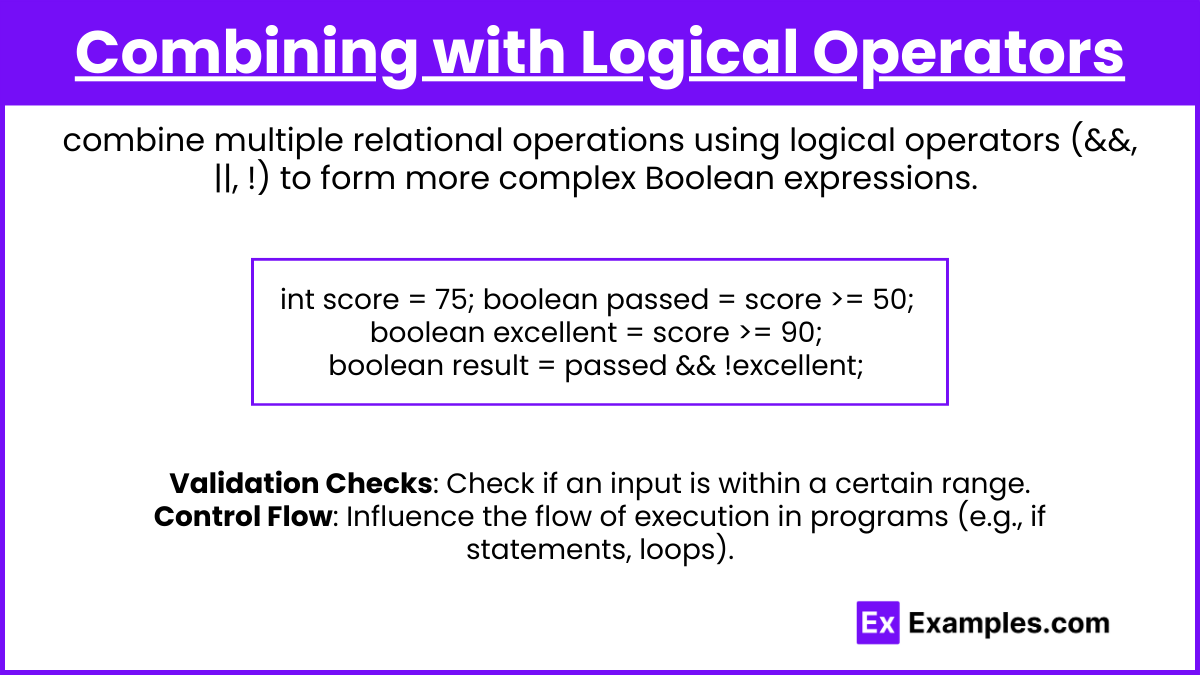
You can combine multiple relational operations using logical operators (&&, ||, !) to form more complex Boolean expressions. For example:
int score = 75;
boolean passed = score >= 50;
boolean excellent = score >= 90;
boolean result = passed && !excellent; // true if score is 50 or more but less than 90
- Validation Checks: Check if an input is within a certain range.
- Control Flow: Influence the flow of execution in programs (e.g., if statements, loops).
Importance in Programming
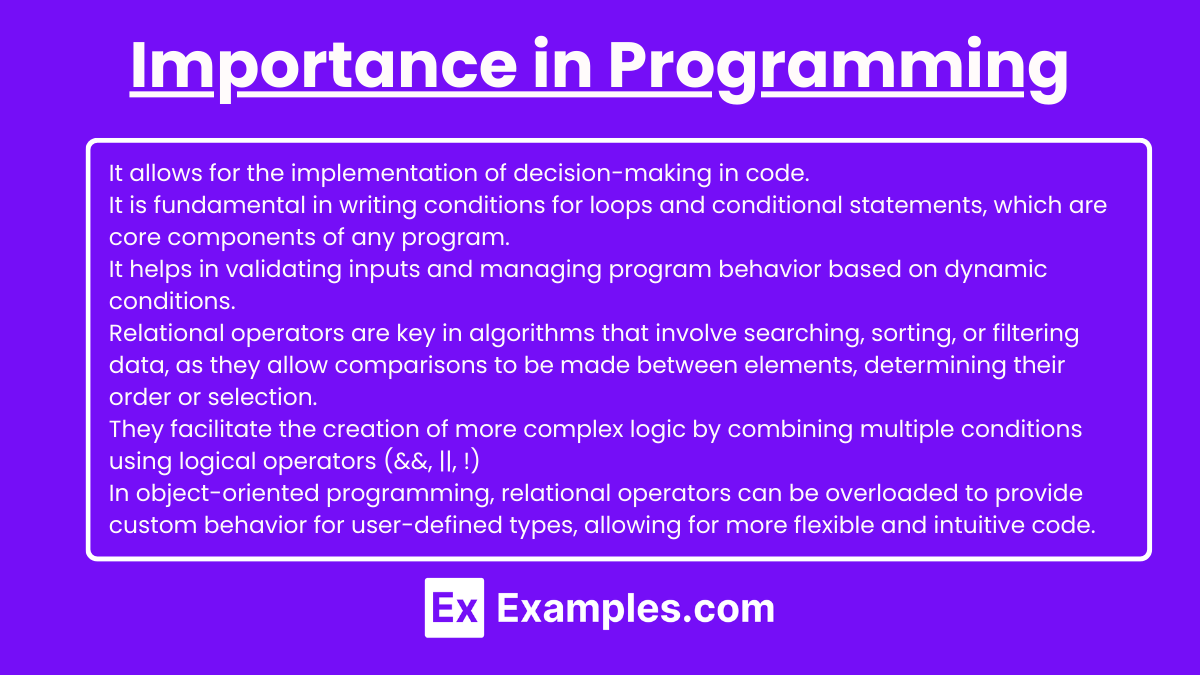
Understanding how to find and use Boolean values with relational operators is crucial because:
- It allows for the implementation of decision-making in code.
- It is fundamental in writing conditions for loops and conditional statements, which are core components of any program.
- It helps in validating inputs and managing program behavior based on dynamic conditions.
- Relational operators are key in algorithms that involve searching, sorting, or filtering data, as they allow comparisons to be made between elements, determining their order or selection.
- They facilitate the creation of more complex logic by combining multiple conditions using logical operators (&&, ||, !), which enhances a program’s ability to handle intricate decision-making scenarios.
- In object-oriented programming, relational operators can be overloaded to provide custom behavior for user-defined types, allowing for more flexible and intuitive code.
Examples
Example 1: Age Verification
To check if a user is eligible for signing up on a platform that requires users to be at least 18 years old, you can use the following expression:
int userAge = 17;
boolean isEligible = userAge >= 18;
Example 2: Score Classification
In a grading system, determining if a student has passed based on their score could involve:
int score = 85;
boolean isPass = score >= 50;
boolean isExcellent = score >= 90;
Here, isPass checks if the score is 50 or more using >=, resulting in true for a score of 85, while isExcellent checks for scores of 90 or above, resulting in false.
Example 3: Temperature Control
To ensure that a room’s temperature is within a comfortable range (say between 68°F and 72°F), the following expression can be used:
double roomTemperature = 70.5;
boolean isComfortable = (roomTemperature >= 68) && (roomTemperature <= 72);
This expression combines >= and <= operators with a logical AND (&&) to verify if the temperature is within the desired range, returning true.
Example 4: Discount Eligibility
For a special sale, customers purchasing more than 5 items receive a discount. This can be checked using:
int itemsPurchased = 7;
boolean getsDiscount = itemsPurchased > 5;
The > operator is used here to determine if the number of items purchased is greater than 5, yielding true for 7 items.
Example 5: Access Control
To control access to a secure area, a security system might check if an access code is exactly correct:
int enteredCode = 1234;
int correctCode = 1234;
boolean hasAccess = enteredCode == correctCode;
The == operator compares the entered code against the correct code, returning true when the user inputs the correct code.
Multiple Choice Questions
Question 1
What is the result of the following expression if ‘ a = 15 ‘ and ‘ b = 10 ‘?
boolean result = (a > b) && (a == 15);
A) ‘ true ‘
B) ‘ false ‘
C) ‘ null ‘
D) None of the above
Correct Answer: A) ‘ true ‘
Explanation: The expression consists of two conditions: ‘ a > b ‘ and ‘ a == 15 ‘. Given ‘ a = 15 ‘ and ‘ b = 10 ‘, the first condition ‘ a > b ‘ evaluates to true because 15 is indeed greater than 10. The second condition ‘ a == 15 ‘ also evaluates to true because a is equal to 15. Since both conditions are true, the logical AND operator (&&) between them also results in true.
Question 2
Evaluate the Boolean expression below when ‘ x = 5 ‘:
boolean check = (x < 10) || (x > 20);
A) ‘ true ‘
B) ‘ false ‘
C) ‘ null ‘
D) Compilation error
Correct Answer: A) true
Explanation:The expression contains two parts connected by a logical OR operator (||): x < 10 and x > 20. For x = 5, x < 10 is true because 5 is less than 10. The second part, x > 20, is false since 5 is not greater than 20. Since the logical OR operator returns true if at least one of the operands is true, the overall result of the expression is true.
Question 3
Which of the following expressions will return false when m = 7 and n = 7?
A) m == n
B) m != n
C) m < n
D) m <= n
Correct Answer: B) m != n
Explanation:
- A) m == n: Evaluates to true because m and n are equal (both are 7).
- B) m != n: Evaluates to false because m is not different from n; they are equal.
- C) m < n: Evaluates to false because m is not less than n; they are the same.
- D) m <= n: Evaluates to true because m is less than or equal to n.
The correct choice is B) because m != n is the only expression that would return false under the given conditions, aligning with the question’s request.