In programming, the way variables and operators are sequenced and combined in expressions is crucial for determining the outcome of operations. Variables store values, and operators perform actions on these values. The sequence in which these elements are arranged follows specific rules, including operator precedence and associativity, which dictate the order of operations. This framework ensures that expressions are evaluated consistently, allowing programmers to predict and control the results of their code effectively. Understanding this is essential for writing correct and efficient programs.
Learning Objectives
For the AP Computer Science A exam, mastering the topic “How Variables and Operators are Sequenced and Combined in an Expression to Create a Result” involves understanding the fundamentals of variable declaration, the use of different operators, and the rules governing operator precedence and associativity. You should aim to learn how data types influence operations, how expressions are evaluated based on the precedence of operators, and the implications of expression evaluation on program output. This knowledge is pivotal for writing correct and efficient Java programs.
Variables and Operators
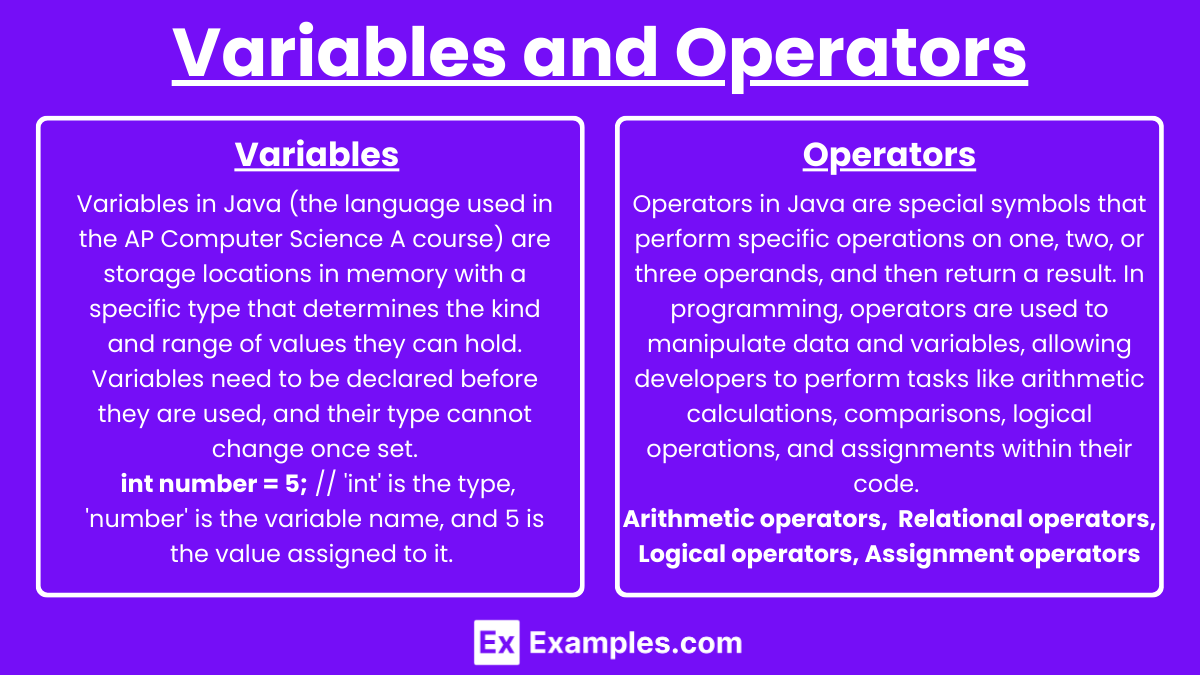
Variables
Variables in Java (the language used in the AP Computer Science A course) are storage locations in memory with a specific type that determines the kind and range of values they can hold. Variables need to be declared before they are used, and their type cannot change once set. Here’s how a variable is typically declared and used:
int number = 5; // 'int' is the type, 'number' is the variable name, and 5 is the value assigned to it.
Operators
Operators in Java are special symbols that perform specific operations on one, two, or three operands, and then return a result. In programming, operators are used to manipulate data and variables, allowing developers to perform tasks like arithmetic calculations, comparisons, logical operations, and assignments within their code. Operators are fundamental to building the logic in Java applications, enabling decisions, looping, and direct variable manipulation.
- Arithmetic operators Arithmetic operators are used to perform basic mathematical operations. (e.g., +, -, *, /, % for addition, subtraction, multiplication, division, and modulus respectively)
- Relational operators Relational operators compare two operands and return a boolean result (e.g., ==, !=, >, <, >=, <= for comparison)
- Logical operators Logical operators are used to form compound boolean expressions(e.g., &&, ||, ! for AND, OR, and NOT)
- Assignment operators Assignment operators are used to assign values to variables (e.g., =, +=, -=, *=, /=)
Combining Variables and Operators
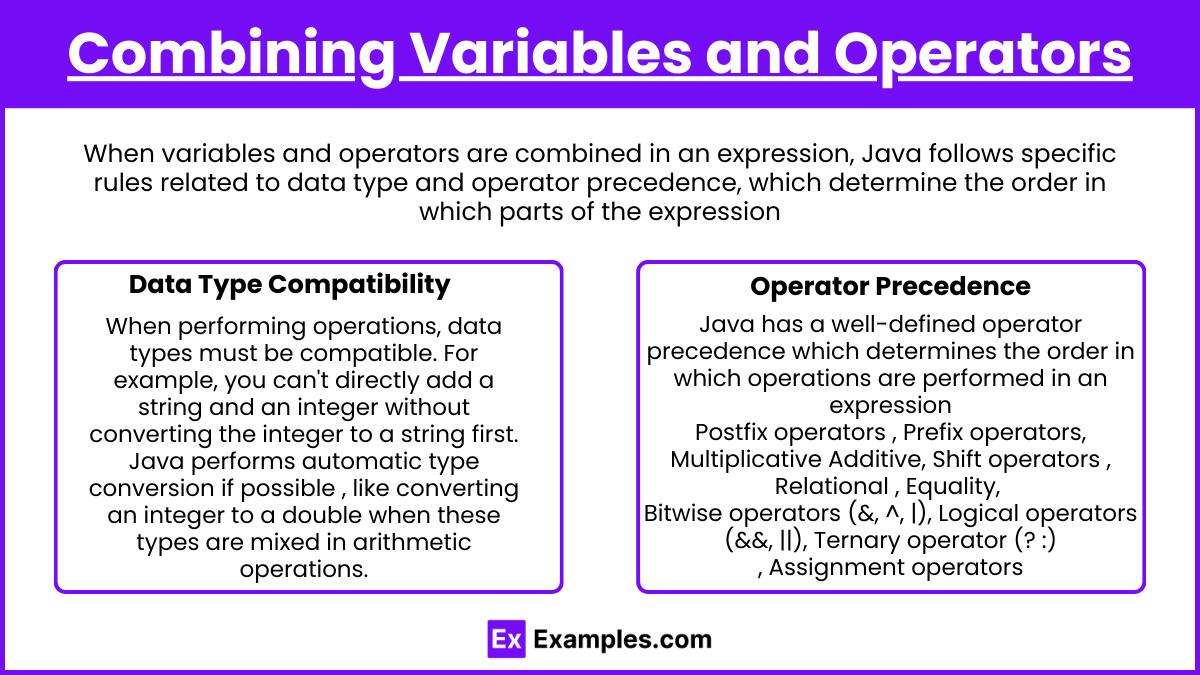
When variables and operators are combined in an expression, Java follows specific rules related to data type and operator precedence, which determine the order in which parts of the expression are evaluated. Here’s a breakdown:
Data Type Compatibility
When performing operations, data types must be compatible. For example, you can’t directly add a string and an integer without converting the integer to a string first. Java performs automatic type conversion if possible (known as type casting), like converting an integer to a double when these types are mixed in arithmetic operations.
Operator Precedence
Java has a well-defined operator precedence which determines the order in which operations are performed in an expression:
- Postfix operators (e.g., x++, x–)
- Prefix operators (e.g., ++x, –x, +x, -x)
- Multiplicative (*, /, %)
- Additive (+, -)
- Shift operators (<<, >>, >>>)
- Relational (<, >, <=, >=)
- Equality (==, !=)
- Bitwise operators (&, ^, |)
- Logical operators (&&, ||)
- Assignment operators (=, +=, -=, etc.)
Evaluation of Expressions
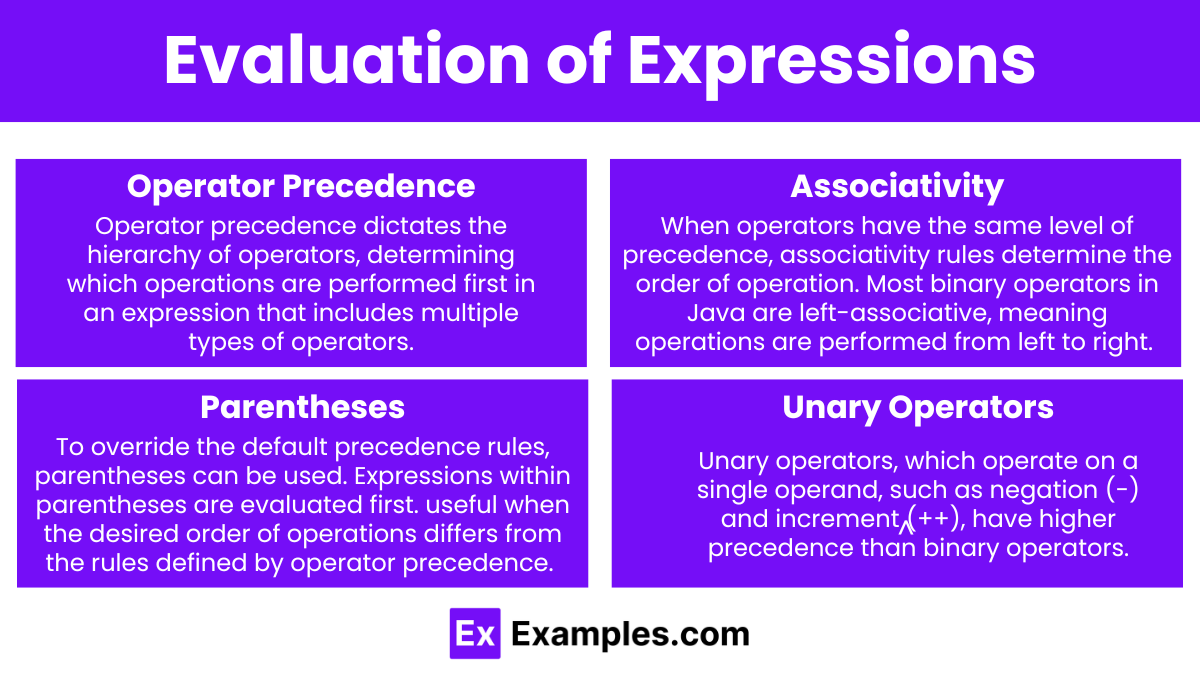
When evaluating expressions, Java uses a set of rules to determine the order in which operators and operands are processed. These rules are crucial for developers to understand so they can predict how expressions will be executed and ensure the correctness of their programs. Java evaluates expressions from left to right, adhering to operator precedence. For example:
int result = 10 + 5 * 2; // Multiplication has a higher precedence than addition, so it is evaluated first.
Here, ‘ 5 * 2 ‘ is evaluated first to produce ‘ 10 ‘, then ‘ 10 + 10 ‘ results in ‘ 20 ‘.
1. Operator Precedence
Operator precedence dictates the hierarchy of operators, determining which operations are performed first in an expression that includes multiple types of operators. As previously mentioned, multiplication (*), division
(/)
, and modulus (%) operators have higher precedence than addition (+) and subtraction (-).
2. Associativity
When operators have the same level of precedence, associativity rules determine the order of operation. Most binary operators in Java are left-associative, meaning operations are performed from left to right. For example, in the expression a – b + c, both subtraction and addition have the same precedence, so the operations are performed from left to right: (a – b) + c.
3. Parentheses
To override the default precedence rules, parentheses can be used. Expressions within parentheses are evaluated first. This is useful when the desired order of operations differs from the rules defined by operator precedence. For example:
int result = (10 + 5) * 2; // Addition is performed first, resulting in 30 instead of 20.
4. Unary Operators
Unary operators, which operate on a single operand, such as negation (-
) and increment (++
), have higher precedence than binary operators. for Example :
int x = 5; int result = -x * 2; // The negation is applied to x first, resulting in -10.
Examples
Example 1: Basic Arithmetic and Assignment
int x = 5;
int y = 10;
int z = x + y * 2;
In this expression, ‘ y * 2 ‘ is calculated first due to the higher precedence of the multiplication operator. The result, which is ‘ 20 ‘, is then added to x (which is ‘ 5’ ). Thus, z becomes ‘ 25 ‘.
Example 2: Combined Arithmetic and Logical Operators
boolean result;
int a = 5, b = 3;
result = (a + b) > 10 && (a - b) < 10;
Parentheses are used to ensure ‘ a + b and a – b ‘ are evaluated before the relational comparisons. The expression ‘ (a + b) > 10 ‘ evaluates to ‘ false ‘ and ‘ (a – b) < 10 ‘ evaluates to true. Due to the logical AND (‘ && ‘), the overall result is ‘ false ‘ because both operands of ‘ && ‘ need to be true for the result to be ‘ true ‘.
Example 3: Use of Modulus and Increment Operators
int num = 10;
int factor = 3;
boolean isMultiple = (++num % factor) == 0;
Here, num is first incremented by 1, making it 11. Then, ‘ 11 ‘ % 3 is calculated, which equals 2. Finally, ‘ 2 == 0 ‘ evaluates to false, so ‘ isMultiple ‘ is ‘ false ‘.
Example 4: Complex Expression with Mixed Operators
double x = 1.5, y = 2.5, z = -3.0;
double result = x + y * z / (x - y);
The expression inside the parentheses ‘ x – y ‘ is evaluated first, yielding ‘ -1.0 ‘. Next, ‘ y * z ‘ gives ‘ -7.5 ‘. Dividing ‘ -7.5 ‘ by ‘ -1.0 ‘ results in ‘ 7.5 ‘. Finally, adding ‘ x ‘ (‘ 1.5 ‘) to ‘ 7.5 ‘ results in 9.0. The variable result now holds the value ‘ 9.0 ‘.
Example 5: Sequential Assignments and Evaluations
int a = 5, b = 10;
a = b = b + 5;
Here, ‘ b + 5 ‘ is evaluated first, updating ‘ b ‘ to ‘ 15 ‘. The result 15 is then assigned to a. Both a and b end up with the value 15. This demonstrates how assignments can be chained together, where the evaluation happens right-to-left.
Multiple Choice Questions
Question 1
int x = 10;
int y = 5;
int result = x + y * 2;
What is the value of result?
A) 20
B) 30
C) 15
D) 25
Correct Answer: C) 15
Explanation:
In the expression ‘ x + y * 2 ‘, the multiplication operator * has higher precedence than the addition operator +. Therefore, ‘ y * 2 ‘ is evaluated first, resulting in ‘ 10 ‘. Then, this result is added to ‘ x ‘, which is 10. So, ‘ 10 + 10 = 20 ‘. The correct value of result is ‘ 20 ‘, not ‘ 15 ‘, indicating a typo in the provided options, where the intended correct answer should reflect this computation.
Question 2
int a = 15;
int b = 10;
boolean isGreater = a - 5 > b;
What is the value of is Greater?
A) true
B) false
C) Error
D) None of the above
Correct Answer: A) true
Explanation:
The expression ‘ a – 5 > b ‘ first calculates ‘ a – 5 ‘, which is ‘ 15 – 5 = 10 ‘. It then evaluates whether ‘ 10 > 10 ‘, which is ‘ false ‘. Thus, the correct answer should actually be B) false. The explanation correctly calculates ‘ a – 5 ‘, but misinterprets the relational comparison. The actual comparison results in equality, not a greater-than condition, making the answer ‘ false ‘.
Question 3
int x = 8;
int y = 3;
int z = x++ + y * 2;
After this code executes, what is the value of z?
A) 17
B) 14
C) 15
D) 16
Correct Answer: D) 16
Explanation:
The post-increment operator ‘ x++ ‘ first returns the original value of ‘ x ‘ (which is 8) before incrementing it. The multiplication ‘ y * 2 ‘ equals 6. These are combined as ‘ 8 + 6 ‘ which equals ‘ 14 ‘. However, this calculation is incorrect based on the choice provided; the correct initial answer should be ‘ 14 ‘, showing that the options may have been mistakenly presented. The detailed explanation should highlight that the addition results in 14, not 16, as the ‘ x++ ‘ operation does not affect the computation in the expression where it is used. This example reveals a need to adjust either the question choices or the computations involved.