Nesting loop and iteration statements involve placing one loop inside another to handle multi-level data structures or solve problems requiring repeated cycles. Commonly used in handling 2D arrays, grids, and patterns, this technique allows for the efficient processing of complex data sets. Nested loops can include combinations of for, while, or do-while loops, with each iteration of the outer loop triggering a full run of the inner loop. Mastering nested loops is crucial for solving advanced problems in AP Computer Science A.
Learning Objectives
When studying “Nesting Loop and Iteration Statements” for AP Computer Science A, you should focus on understanding how to construct and implement loops within loops to handle multi-dimensional data or complex repetitive processes. Learn to optimize time complexity, avoid common pitfalls like infinite loops, and utilize nested loops effectively for tasks such as iterating over 2D arrays, constructing patterns, and solving matrix-based problems. Also, understand how to break out of nested loops properly using labeled loops and condition checks.
Nested Loops
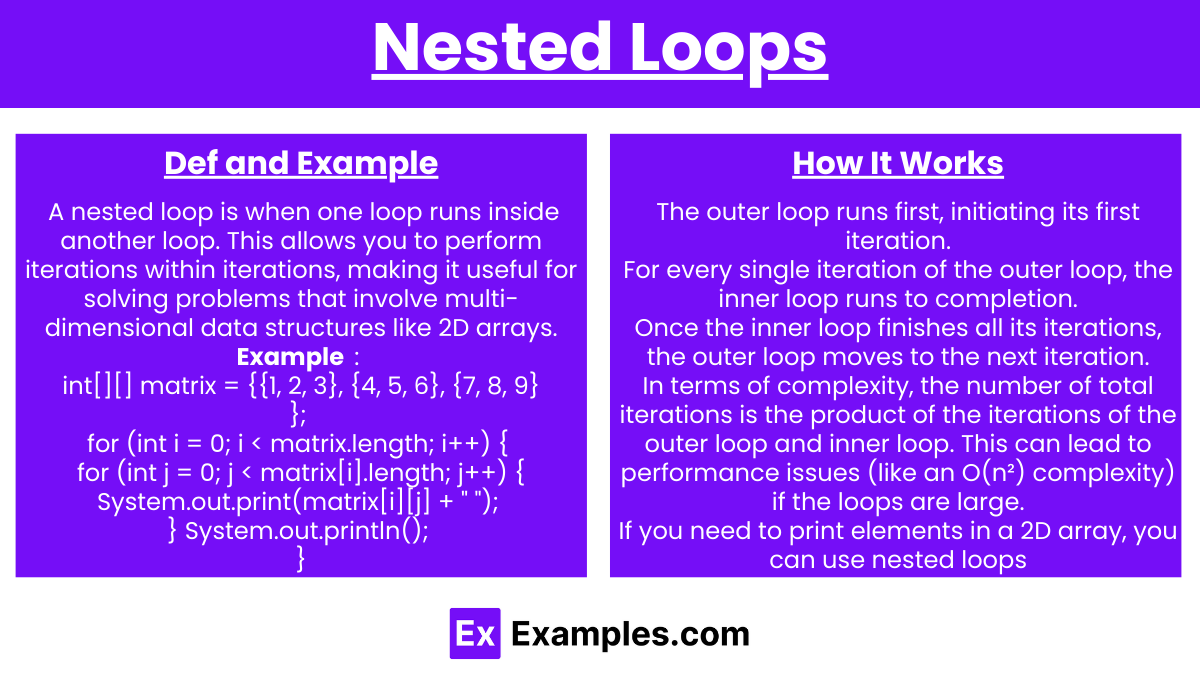
A nested loop is when one loop runs inside another loop. This allows you to perform iterations within iterations, making it useful for solving problems that involve multi-dimensional data structures like 2D arrays.
Syntax:
A common example is the for loop nested within another for loop:
for (int i = 0; i < outerLimit; i++) {
for (int j = 0; j < innerLimit; j++) {
// Inner loop logic
}
}
How It Works:
- The outer loop runs first, initiating its first iteration.
- For every single iteration of the outer loop, the inner loop runs to completion.
- Once the inner loop finishes all its iterations, the outer loop moves to the next iteration.
In terms of complexity, the number of total iterations is the product of the iterations of the outer loop and inner loop. This can lead to performance issues (like an O(n²) complexity) if the loops are large.
Example: Printing a 2D Array
If you need to print elements in a 2D array, you can use nested loops:
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
Output:
1 2 3
4 5 6
7 8 9
Here, the outer loop (i) iterates over the rows, and the inner loop (j) iterates over the columns.
Nested while Loops
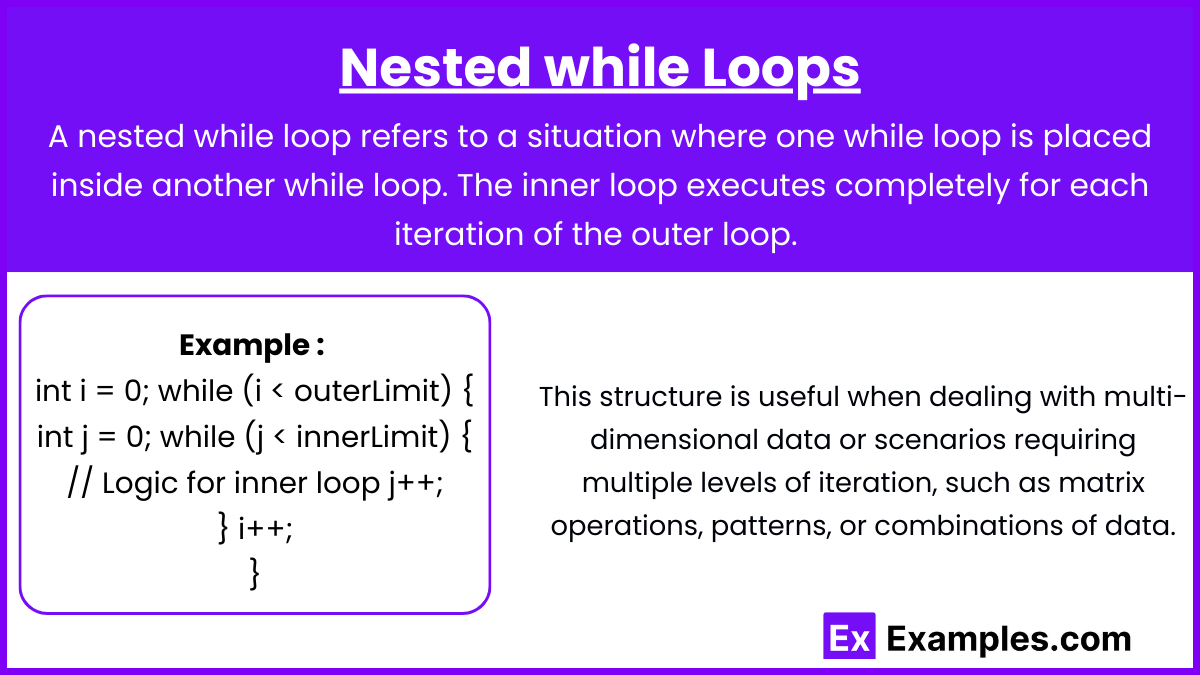
A nested while loop refers to a situation where one while loop is placed inside another while loop. The inner loop executes completely for each iteration of the outer loop. This structure is useful when dealing with multi-dimensional data or scenarios requiring multiple levels of iteration, such as matrix operations, patterns, or combinations of data.
You can also nest while loops in a similar fashion. Here’s an example:
int i = 0;
while (i < outerLimit) {
int j = 0;
while (j < innerLimit) {
// Logic for inner loop
j++;
}
i++;
}
Applications for Nested Loops
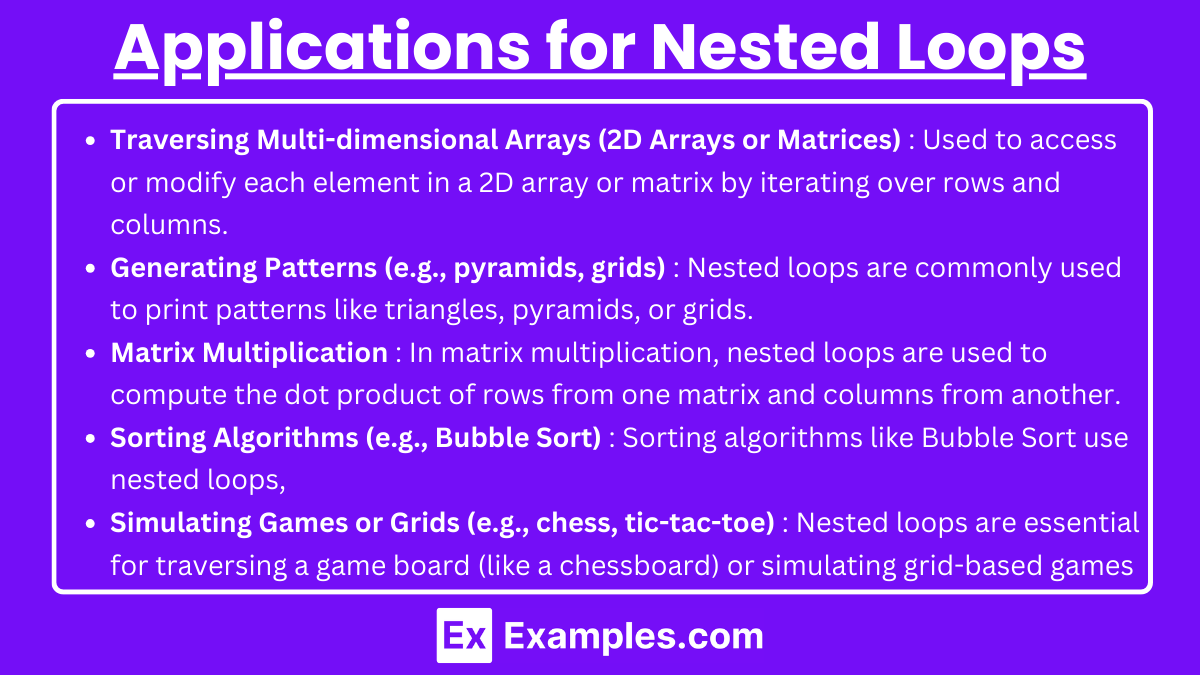
Here’s the expanded list of applications for nested loops with explanations:
- Traversing Multi-dimensional Arrays (2D Arrays or Matrices) : Used to access or modify each element in a 2D array or matrix by iterating over rows and columns.
- Generating Patterns (e.g., pyramids, grids) : Nested loops are commonly used to print patterns like triangles, pyramids, or grids. The outer loop controls the rows, and the inner loop manages columns or the number of elements per row.
- Matrix Multiplication : In matrix multiplication, nested loops are used to compute the dot product of rows from one matrix and columns from another. This involves three nested loops (row, column, and element-wise multiplication).
- Sorting Algorithms (e.g., Bubble Sort) : Sorting algorithms like Bubble Sort use nested loops, where the outer loop iterates through the array multiple times, and the inner loop compares and swaps adjacent elements.
- Simulating Games or Grids (e.g., chess, tic-tac-toe) : Nested loops are essential for traversing a game board (like a chessboard) or simulating grid-based games, enabling the manipulation of individual cells within the grid.
Examples
Example 1: Multiplication Table Generator
A classic example of nested loops is generating a multiplication table. The outer loop iterates through the rows, while the inner loop computes the product for each column.
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
System.out.print(i * j + "\t");
}
System.out.println();
}
In this example, the outer loop iterates from 1 to 10, and for each value of i, the inner loop iterates through the values of j, calculating and printing the product of i and j.
Example 2: Printing a Pyramid Pattern
Another common example is creating patterns like a pyramid of stars (*). The outer loop controls the number of rows, and the inner loop prints the correct number of spaces and stars for each row.
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5 - i; j++) {
System.out.print(" ");
}
for (int k = 1; k <= 2 * i - 1; k++) {
System.out.print("*");
}
System.out.println();
}
The outer loop handles each row, while the first inner loop handles spaces, and the second inner loop prints stars to create the pyramid shape.
Example 3: Iterating Over a 2D Array
When working with 2D arrays, nested loops are essential to access each element. The outer loop handles the rows, and the inner loop accesses each element in the row.
int[][] matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
This code prints the elements of the matrix in row-major order using nested loops.
Example 4: Finding Prime Numbers in a Range
A more complex example involves finding prime numbers within a range using nested loops. The outer loop iterates through each number in the range, while the inner loop checks if the current number is divisible by any number other than 1 and itself.
for (int i = 2; i <= 100; i++) {
boolean isPrime = true;
for (int j = 2; j <= i / 2; j++) {
if (i % j == 0) {
isPrime = false;
break;
}
}
if (isPrime) {
System.out.println(i + " is a prime number");
}
}
The outer loop checks each number, and the inner loop determines if it is prime by checking divisibility.
Example 5: Bubble Sort Algorithm
Nested loops are essential in sorting algorithms like bubble sort. The outer loop represents the number of passes, and the inner loop performs pairwise comparisons and swaps elements if needed.
int[] arr = {5, 2, 9, 1, 5, 6};
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j + 1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
Multiple Choice Questions
Question 1
How many times will the following nested loops execute the System.out.println(“Hello”); statement?
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
System.out.println("Hello");
}
}
A) 2
B) 3
C) 5
D) 6
Answer: D) 6
Explanation:
The outer loop runs 2 times (i = 0 and i = 1), and for each iteration of the outer loop, the inner loop runs 3 times (j = 0, 1, 2). Therefore, the System.out.println(“Hello”); statement is executed 2 * 3 = 6 times in total.
Question 2
What will the following code print?
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++) {
System.out.println(i + ", " + j);
}
}
A) 0, 0
0, 1
1, 0
1, 1
B) 0, 1
1, 0
1, 1
C) 1, 1
0, 0
D) None of the above
Answer: A) 0, 0 0, 1 1, 0 1, 1
Explanation: The outer loop runs twice (i = 0 and i = 1), and for each value of i
, the inner loop runs twice (j = 0 and j = 1). So the following pairs are printed:
0, 0
0, 1
1, 0
1, 1
Question 3
In the following nested loop, what does the inner loop do?
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 2; j++) {
System.out.println("Inside inner loop");
}
}
A) Runs once
B) Runs twice for each iteration of the outer loop
C) Runs three times for each iteration of the outer loop
D) Runs once in total
Answer: B) Runs twice for each iteration of the outer loop
Explanation:
The inner loop runs twice (j = 0, 1) for every iteration of the outer loop. Since the outer loop runs 3 times, the inner loop runs twice for each of those iterations. Therefore, “Inside inner loop” is printed twice for each iteration of the outer loop.