In AP Computer Science A, the concepts of objects and classes are foundational to understanding object-oriented programming (OOP). Objects are instances of classes, encapsulating attributes (data) and behaviors (methods) specific to the object. Classes serve as blueprints that define these attributes and behaviors, allowing for the creation of multiple objects with similar structures but individual states. This framework is crucial for developing scalable and maintainable software, making it a central topic in the curriculum and essential for mastering the course.
Learning Objectives
For the topic “Objects and Classes as Ways to Describe Instances, Attributes, and Behaviors” in AP Computer Science A, you should focus on mastering the concept of classes as blueprints for creating objects, each with unique instances. Understand how attributes define object properties and how methods enable object behaviors. Grasp the significance of constructors for initializing new objects. Learn about encapsulation to protect object data. Apply these concepts to create, manipulate, and interact with objects in programming scenarios, preparing you for both problem-solving and practical application questions on the exam.
“Objects and Classes as Ways to Describe Instances, Attributes, and Behaviors”
In object-oriented programming (OOP), which is a central concept in the AP Computer Science A curriculum, classes and objects are fundamental structures that help you organize and implement your code efficiently.
1. Understanding Classes and Objects
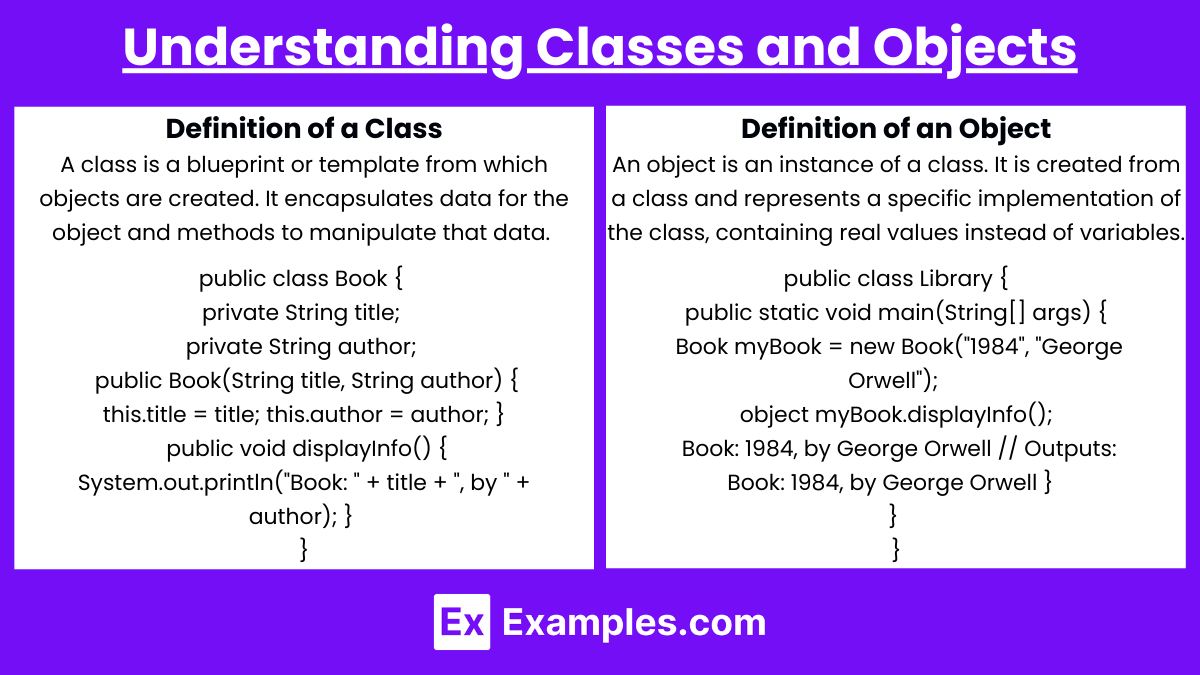
Definition of a Class: A class is a blueprint or template from which objects are created. It encapsulates data for the object and methods to manipulate that data. A class defines the types of data it can hold and the functions it can perform. A blueprint or template from which objects are created. It defines the data and the methods to manipulate that data.
Simple Example: ‘ Book ‘ Class and its Instance
public class Book {
// Attributes
private String title;
private String author;
// Constructor to initialize the Book object
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Method to display book information
public void displayInfo() {
System.out.println("Book: " + title + ", by " + author);
}
}
Definition of an Object: An object is an instance of a class. It is created from a class and represents a specific implementation of the class, containing real values instead of variables. An instance of a class. It represents a specific implementation of the class, containing actual values.
Creating and Using an Object: ‘ myBook ‘
Now, we create an object called myBook from the Book class, which will be a specific book with its own title and author.
public class Library {
public static void main(String[] args) {
// Creating an object of Book
Book myBook = new Book("1984", "George Orwell");
// Using the method of the Book
object myBook.displayInfo(); // Outputs: Book: 1984, by George Orwell
}
}
Attributes and Behaviors
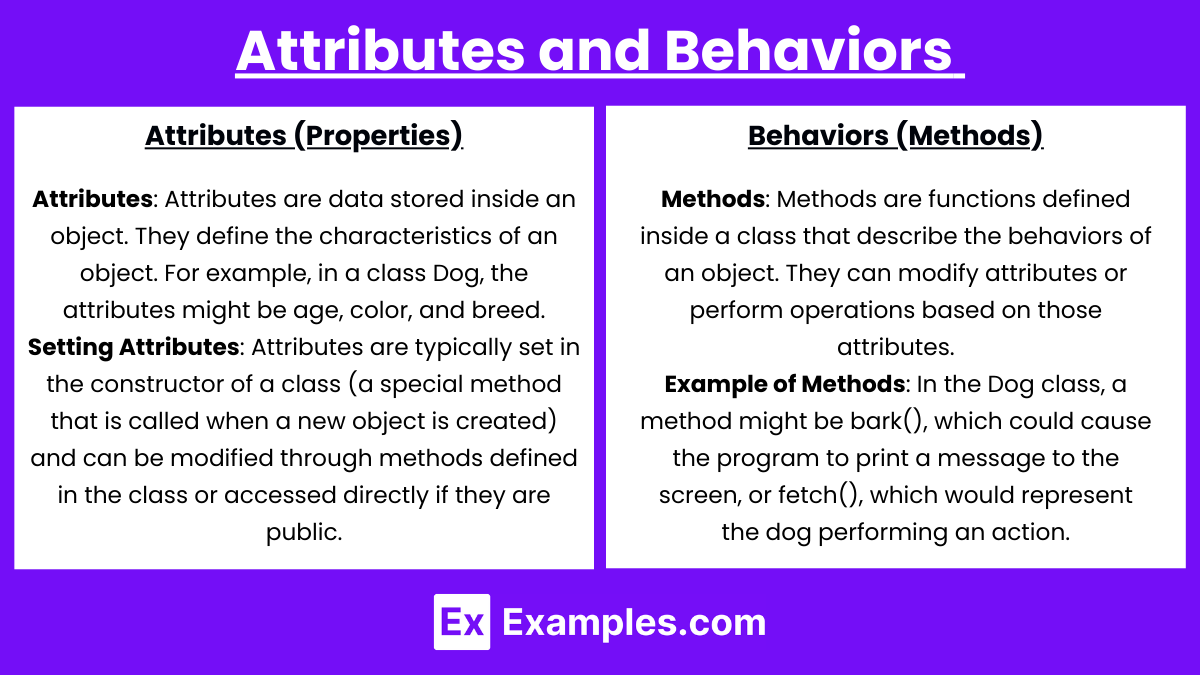
Attributes (Properties)
- Attributes: Attributes are data stored inside an object. They define the characteristics of an object. For example, in a class Dog, the attributes might be age, color, and breed.
- Setting Attributes: Attributes are typically set in the constructor of a class (a special method that is called when a new object is created) and can be modified through methods defined in the class or accessed directly if they are public.
Behaviors (Methods)
- Methods: Methods are functions defined inside a class that describe the behaviors of an object. They can modify attributes or perform operations based on those attributes.
- Example of Methods: In the Dog class, a method might be bark(), which could cause the program to print a message to the screen, or fetch(), which would represent the dog performing an action.
Defining Classes and Creating Objects
Syntax in Java: Java is typically used in AP Computer Science A for illustrating OOP concepts.
public class Car {
// Attributes
String color;
String make;
int year;
// Constructor
public Car(String color, String make, int year) {
this.color = color;
this.make = make;
this.year = year;
}
// Behaviors
void start() {
System.out.println("Car is starting");
}
void accelerate() {
System.out.println("Car is accelerating");
}
}
// Creating an object
Car myCar = new Car("Red", "Toyota", 2021);
myCar.start(); // Calling a behavior
Importance of Understanding Objects and Classes
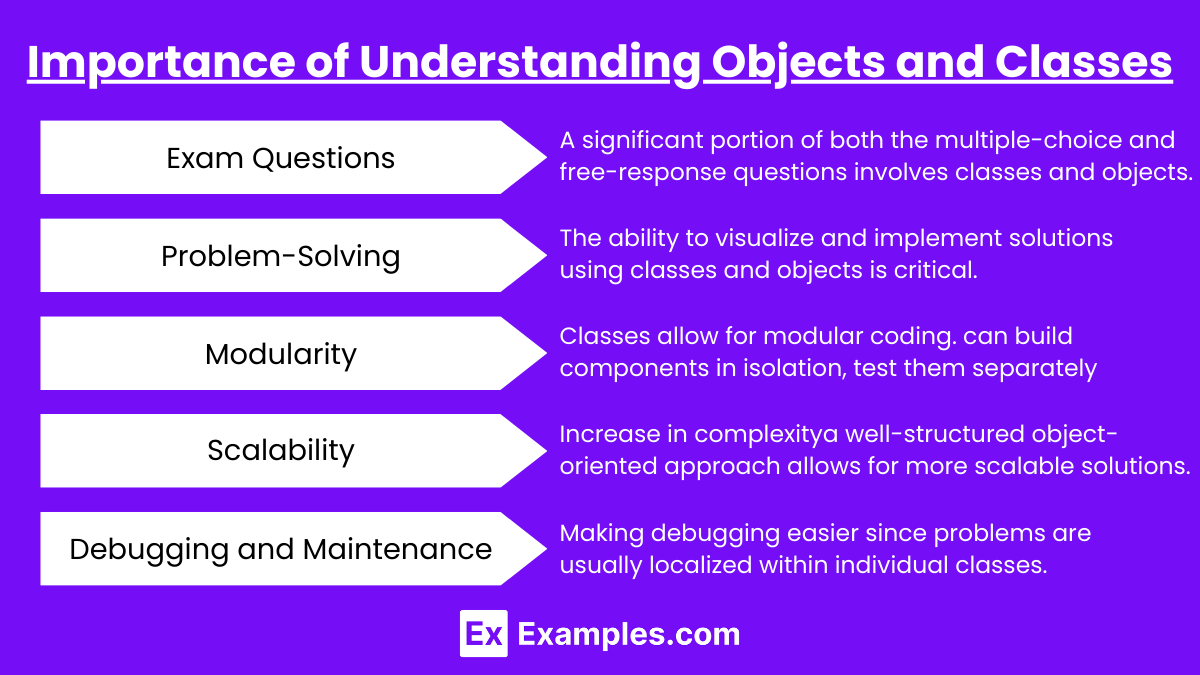
Central Role in Object-Oriented Programming (OOP)
Objects and classes form the core of object-oriented programming, a paradigm that organizes software design around data, or objects, rather than functions and logic. This approach mirrors how real-world interactions are often structured, making it a powerful way to model complex systems and processes in programming.
- Exam Questions: On the AP Computer Science A exam, a significant portion of both the multiple-choice and free-response questions involves classes and objects. Students are often asked to interpret, modify, or write new classes, demonstrating not just basic syntax but a deeper understanding of how objects interact within a system.
- Problem-Solving: The ability to visualize and implement solutions using classes and objects is critical. For instance, if tasked with creating a simulation of a school system, you would need to define classes such as Student, Teacher, and Classroom, each with its own attributes and behaviors.
- Modularity: Classes allow for modular coding. This means you can build components in isolation, test them separately, and then integrate them. This not only makes complex applications more manageable but also enhances code reusability.
- Scalability: As problems increase in complexity, a well-structured object-oriented approach allows for more scalable solutions. It’s easier to add new features or modify existing ones when the foundational structures (classes and objects) are well defined.
- Debugging and Maintenance: Classes encapsulate functionality, making debugging easier since problems are usually localized within individual classes. Maintenance becomes more straightforward as each class’s operations are independent of others, reducing the chance of unexpected bugs when changes are made.
Examples
Example 1: Bank Account Class
- Class: BankAccount
- Attributes: accountNumber, ownerName, balance
- Behaviors: deposit(amount), withdraw(amount), checkBalance()
- Description: The BankAccount class represents a bank account where ‘accountNumber’ identifies the account, ‘ownerName’ is the name of the account holder, and ‘balance’ shows the current funds available. The behaviors include depositing or withdrawing money with deposit(amount) and withdraw(amount), and checkBalance() to display the current balance.
Example 2: Car Class
- Class: Car
- Attributes: make, model, year, color
- Behaviors: start(), stop(), accelerate(speed), paint(newColor)
- Description: The Car class is used to represent a vehicle. Attributes like ‘make’, ‘model’, ‘year’, and ‘color’ define the car’s specifications. The behaviors include starting or stopping the car using start() and stop(), changing the car’s speed with accelerate(speed), and repainting the car using paint(newColor).
Example 3: Student Class
- Class: Student
- Attributes: studentID, name, gradeLevel, courses
- Behaviors: enroll(course), drop(course), updateGrade(course, grade)
- Description: In the Student class, ‘studentID’ uniquely identifies the student, ‘name’ is the student’s full name, ‘gradeLevel’ indicates their year in school, and ‘courses’ lists the classes they are taking. Behaviors include enrolling in new courses with enroll(course), dropping courses with drop(course), and updating grades for courses with updateGrade(course, grade).
Example 4: Smartphone Class
- Class: Smartphone
- Attributes: model, operatingSystem, storageCapacity, isUnlocked
- Behaviors: installApp(appName), makeCall(number), sendText(message, number)
- Description: The Smartphone class describes a mobile phone with attributes such as ‘model’, ‘operatingSystem’, ‘storageCapacity’, and whether it is ‘isUnlocked’. The behaviors include installing new applications with installApp(appName), making calls with makeCall(number), and sending text messages using sendText(message, number).
Example 5: Library Book Class
- Class: LibraryBook
- Attributes: ISBN, title, author, isAvailable
- Behaviors: checkOut(), returnBook(), reserve()
- Description: This class represents a book in a library. Attributes include ‘ISBN’ for the book identifier, ‘title’, ‘author’, and ‘isAvailable’ to indicate if the book is currently available for checkout. Behaviors consist of checking out the book with checkOut(), returning the book using returnBook(), and reserving the book if it’s currently loaned out with reserve().
Each example demonstrates how classes in object-oriented programming encapsulate both data (attributes) and procedures (behaviors) relevant to the objects they represent.
Multiple Choice Questions
Question 1
What does a constructor in a class primarily do?
A) It deletes an object of a class.
B) It initializes an object of a class with specific values.
C) It performs calculations when called.
D) It prints out the attributes of an object.
Correct Answer: B) It initializes an object of a class with specific values.
Explanation:
A constructor is a special method used to initialize objects. When a new object is created, the constructor sets the initial state of the object by assigning values to its attributes based on the parameters provided. This is essential for setting up an object when it is first created.
Question 2
Which of the following statements is true about encapsulation in object-oriented programming?
A) Encapsulation restricts direct access to some of an object’s components.
B) Encapsulation allows the attributes of a class to be accessed and modified directly.
C) Encapsulation eliminates the need for methods within a class.
D) Encapsulation only applies to methods, not attributes.
Correct Answer: A) Encapsulation restricts direct access to some of an object’s components.
Explanation:
Encapsulation is a fundamental concept in object-oriented programming that involves bundling the data (attributes) and methods that operate on the data into a single unit or class. It restricts direct access to some of the object’s components, which is usually done by making attributes private and providing public methods (getters and setters) to access and modify the values of these private attributes. This protects the integrity of the data and the object’s state.
Question 3
What is the relationship between a class and an object in object-oriented programming?
A) A class is a specific instance of an object.
B) An object is a template for a class.
C) A class is a generic template from which objects are created.
D) Objects and classes are unrelated.
Correct Answer: C) A class is a generic template from which objects are created.
Explanation:
In object-oriented programming, a class acts as a blueprint or template defining the nature of a future object. An object is then created as an instance of a class. This means that the class specifies the attributes and behaviors that its objects will have, but an actual object needs to be instantiated using the class blueprint for it to exist and operate within the program. This relationship is fundamental in understanding how object-oriented programming structures data and operations.