In AP Computer Science A, setting an object’s attributes using constructors is essential for initializing an object’s state when it is created. Constructors are special methods in a class used to assign values to the object’s attributes, ensuring that it starts with valid data. Constructors can be parameterized or default, allowing flexibility in how objects are instantiated. By utilizing constructors, programmers can ensure consistent and logical initialization of objects, which is a fundamental aspect of object-oriented programming (OOP) principles and key to building efficient applications.
Learning Objectives
When learning about setting an object’s attributes using constructors for AP Computer Science A, you should focus on understanding how constructors initialize an object’s state by assigning values to its attributes. This includes mastering the syntax for both default and parameterized constructors, using the this keyword for clarity, and overloading constructors to offer flexibility in object creation. Additionally, you should learn how constructors ensure that objects are created in a valid state and how constructor overloading allows for multiple ways of instantiating objects.
Key Concepts of Setting an Object’s Attributes Using Constructors
What is a Constructor?
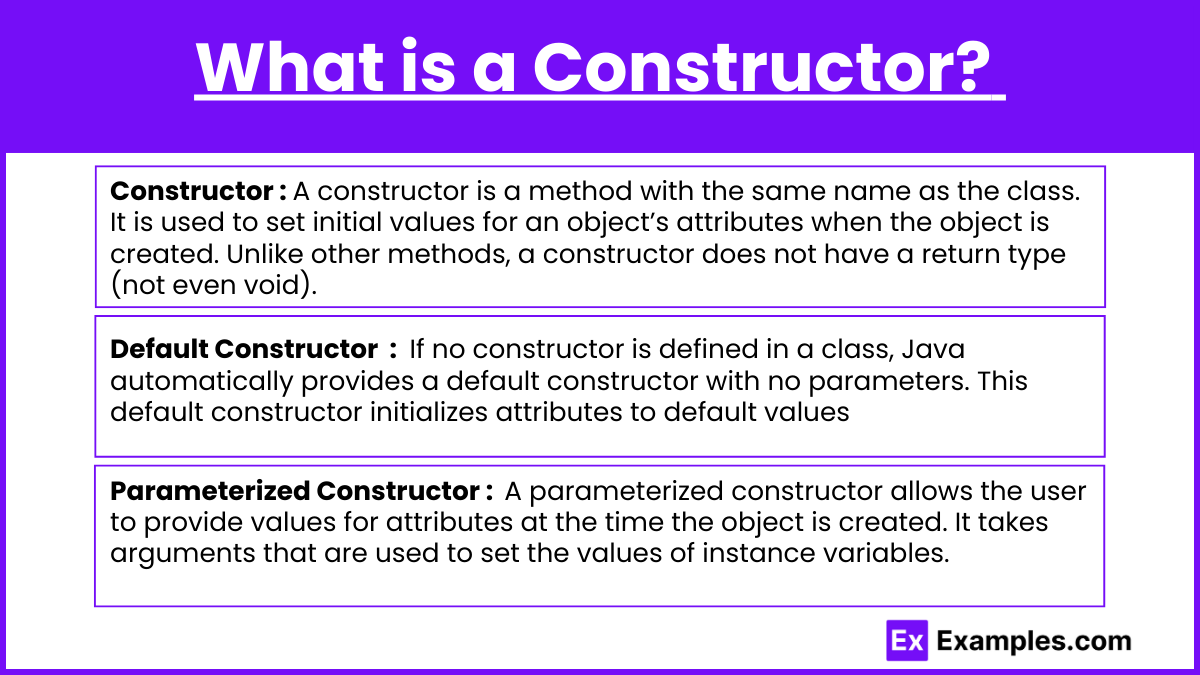
A constructor is a method with the same name as the class. It is used to set initial values for an object’s attributes when the object is created. Unlike other methods, a constructor does not have a return type (not even void).
Default Constructor:
If no constructor is defined in a class, Java automatically provides a default constructor with no parameters. This default constructor initializes attributes to default values (e.g., 0 for integers, null for objects, and false for booleans).
Example:
public class Student {
int age;
String name;
// Default constructor (automatically provided if no constructor is defined)
public Student() {
// Initializes to default values: age = 0, name = null
}
}
Parameterized Constructor:
A parameterized constructor allows the user to provide values for attributes at the time the object is created. It takes arguments that are used to set the values of instance variables.
Example:
public class Student {
int age;
String name;
// Parameterized constructor
public Student(int studentAge, String studentName) {
age = studentAge;
name = studentName;
}
}
// Creating a new object with specific values
Student student1 = new Student(18, "Alice");
Here, the Student class has a constructor that accepts age and name parameters, setting these values for the object’s attributes.
Constructor Overloading
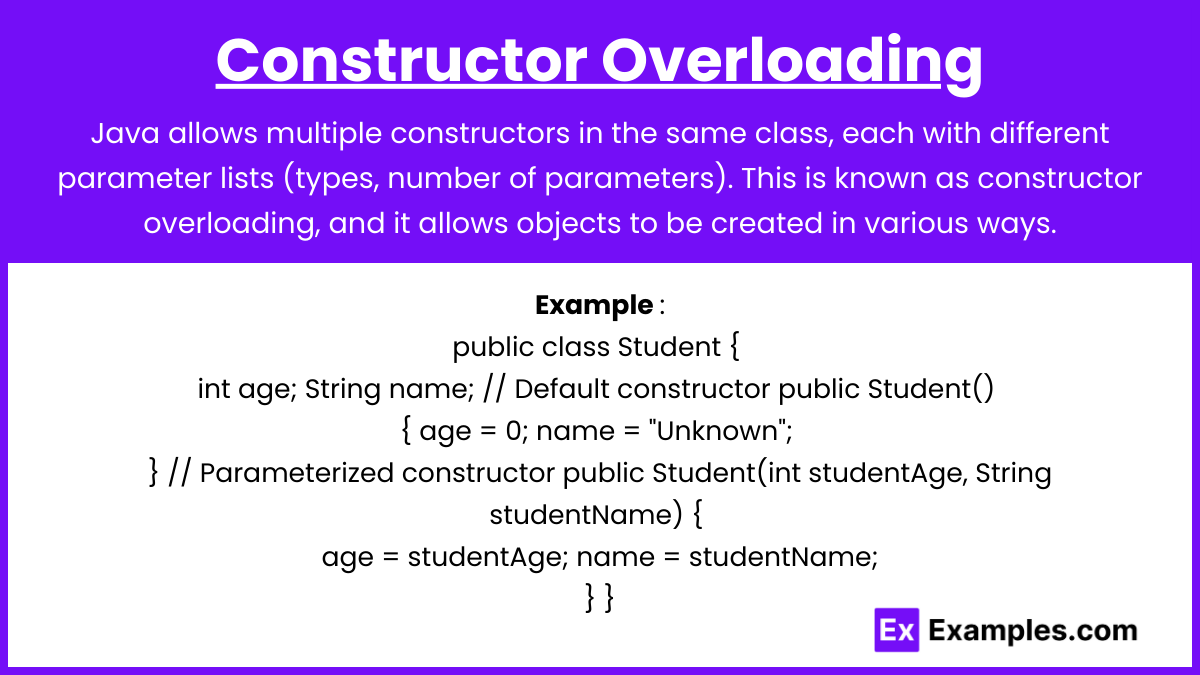
Java allows multiple constructors in the same class, each with different parameter lists (types, number of parameters). This is known as constructor overloading, and it allows objects to be created in various ways.
Example:
public class Student {
int age;
String name;
// Default constructor
public Student() {
age = 0;
name = "Unknown";
}
// Parameterized constructor
public Student(int studentAge, String studentName) {
age = studentAge;
name = studentName;
}
}
// Using different constructors
Student student1 = new Student(); // Uses default constructor
Student student2 = new Student(18, "Alice"); // Uses parameterized constructor
this Keyword
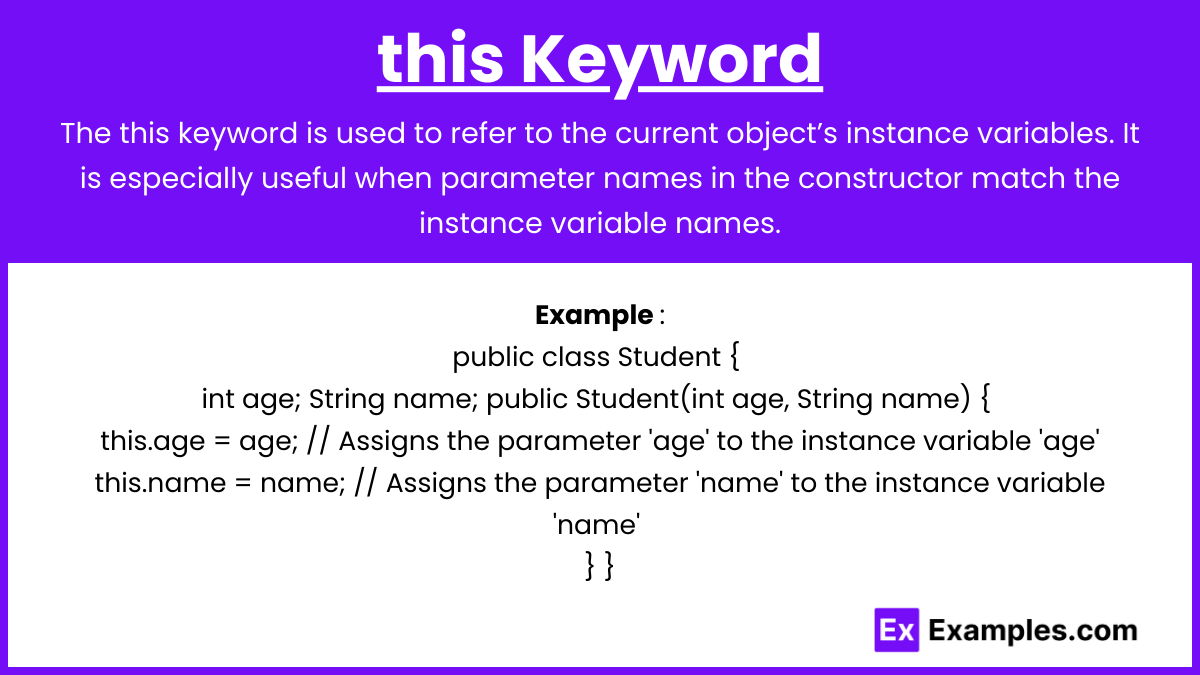
The this keyword is used to refer to the current object’s instance variables. It is especially useful when parameter names in the constructor match the instance variable names.
Example:
public class Student {
int age;
String name;
public Student(int age, String name) {
this.age = age; // Assigns the parameter 'age' to the instance variable 'age'
this.name = name; // Assigns the parameter 'name' to the instance variable 'name'
}
}
Setting Default Values Using Constructors
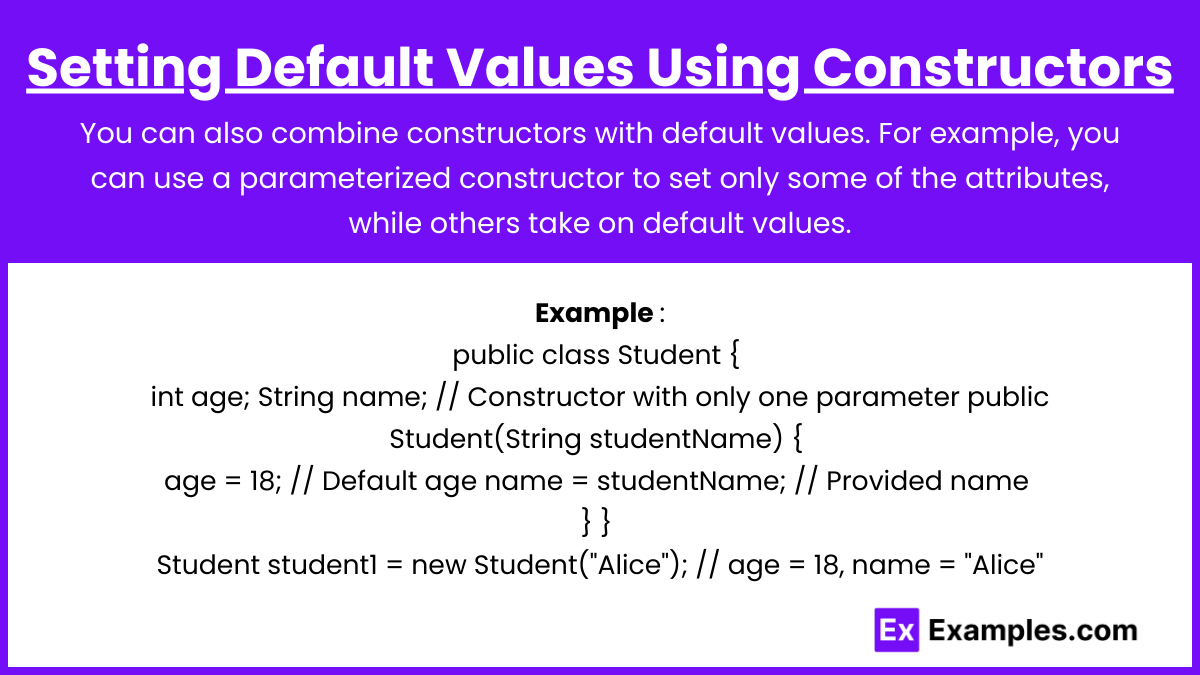
You can also combine constructors with default values. For example, you can use a parameterized constructor to set only some of the attributes, while others take on default values.
Example:
public class Student {
int age;
String name;
// Constructor with only one parameter
public Student(String studentName) {
age = 18; // Default age
name = studentName; // Provided name
}
}
Student student1 = new Student("Alice"); // age = 18, name = "Alice"
Examples
Example 1: Employee Class
In an Employee class, the constructor initializes the employee’s name, id, and salary. For instance, a parameterized constructor can be used to set the employee’s name to “John”, id to 101, and salary to 50000. This ensures that every time an Employee object is created, the attributes are properly initialized with specific values.
Example 2: Car Class
Consider a Car class with attributes like make, model, and year. A constructor can accept these parameters and assign them to the instance variables. When creating a Car object like Car myCar = new Car(“Toyota”, “Corolla”, 2021);, the attributes are initialized to “Toyota” for the make, “Corolla” for the model, and 2021 for the year.
Example 3: Book Class
In a Book class, the constructor might set attributes such as title, author, and price. Using a constructor like public Book(String title, String author, double price), a new Book object is created with specific values passed by the user, ensuring each book is properly described with its title, author’s name, and cost.
Example 4: Point Class
For a Point class that represents a point in a 2D space with x and y coordinates, a constructor can initialize these values. When creating a Point object such as Point p1 = new Point(5, 10);, the x and y coordinates are set to 5 and 10, respectively, making sure the object starts with defined coordinates.
Example 5: Person Class
A Person class might have attributes like name and age. By using a constructor like Person(String name, int age), you can set these attributes during object creation, such as Person person1 = new Person(“Alice”, 25);. This sets the name to “Alice” and age to 25, ensuring the object has valid attribute values.
Multiple Choice Questions
Question 1
What is the primary purpose of a constructor in a Java class?
A) To perform mathematical operations within the class
B) To initialize an object’s attributes when it is created
C) To return a specific value from a class method
D) To create static variables for the class
Answer: B) To initialize an object’s attributes when it is created
Explanation: A constructor is used to set up the initial state of an object by initializing its attributes. When an object is instantiated, the constructor is automatically called, ensuring that its fields are set with default or user-specified values. Constructors do not perform mathematical operations (Option A), return specific values (Option C), or create static variables (Option D). Static variables belong to the class, not individual objects, and are handled differently.
Question 2
Consider the following code snippet. What will be the value of age and name after creating the object Student student = new Student(20, “John”);?
public class Student {
int age;
String name;
public Student(int a, String n) {
this.age = a;
this.name = n;
}
}
A) age = 0, name = null
B) age = 20, name = “John”
C) age = 20, name = null
D) age = 0, name = “John”
Answer: B) age = 20, name = “John”
Explanation: In the constructor Student(int a, String n), the this keyword is used to assign the values passed as parameters (a and n) to the instance variables age and name. When the object Student student = new Student(20, “John”); is created, the values 20 and “John” are passed to the constructor, and this.age = a and this.name = n assign these values to the instance variables. Therefore, the age attribute will be set to 20, and the name attribute will be set to “John.”
Question 3
Which of the following statements about constructors is incorrect?
A) A constructor has the same name as the class.
B) Constructors can have parameters.
C) Constructors must have a return type.
D) If no constructor is provided, a default constructor is automatically created.
Answer: C) Constructors must have a return type.
Explanation: Option C is the incorrect statement because constructors do not have a return type, not even void. Constructors are designed to initialize an object, not to return values. Option A is correct, as constructors must have the same name as the class. Option B is also correct since constructors can take parameters (parameterized constructors). Option D is correct because if no constructor is defined, Java automatically provides a default constructor that initializes attributes to default values (e.g., 0, null, false).