Standard algorithms that utilize array traversals are essential tools in programming, especially in AP Computer Science A. These algorithms involve iterating through arrays to perform various tasks, such as searching for specific elements, finding the minimum or maximum values, summing elements, or sorting arrays. By understanding how to efficiently traverse arrays, students can implement solutions to common problems, including linear search, binary search, bubble sort, and selection sort. Mastering these techniques enables students to develop more efficient and optimized code for a variety of challenges.
Learning Objectives
In studying “Standard Algorithms that Utilize Array Traversals to Perform Functions” for AP Computer Science A, you should learn how to efficiently traverse arrays using loops to access, modify, and analyze data. Focus on understanding and implementing common algorithms like linear search, binary search, summing elements, finding minimum/maximum, counting occurrences, and sorting arrays. Additionally, explore how to manipulate arrays by reversing or shifting elements. Practice applying these algorithms in different problem-solving contexts to reinforce your understanding and improve your coding efficiency.
Standard Algorithms that Utilize Array Traversals
In AP Computer Science A, understanding how to use standard algorithms with array traversals is key to solving problems efficiently. Here are the key concepts:
Traversing an Array
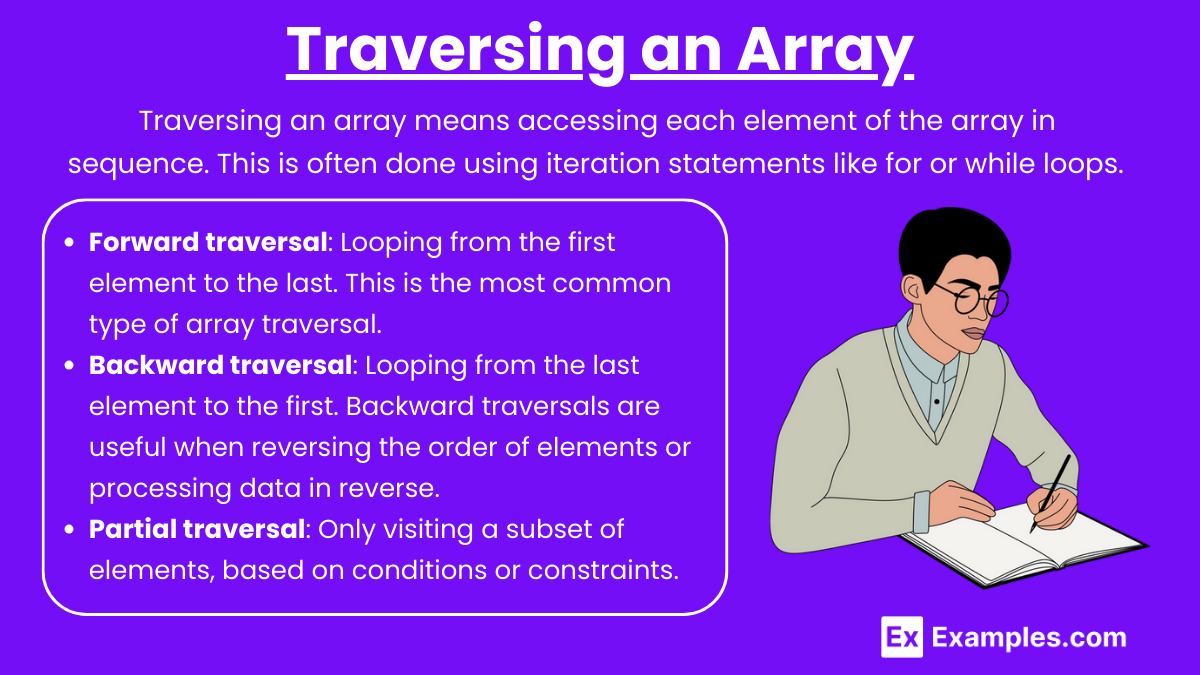
Traversing an array means accessing each element of the array in sequence. This is often done using iteration statements like for or while loops. Traversals are often implemented using iteration mechanisms like for, while, and enhanced for loops, depending on the scenario.Common traversal patterns include:
- Forward traversal: Looping from the first element to the last. This is the most common type of array traversal. In forward traversal, you begin at the first element (index 0) and move sequentially to the last element.
- Backward traversal: Looping from the last element to the first. Backward traversals are useful when reversing the order of elements or processing data in reverse.
- Partial traversal: Only visiting a subset of elements, based on conditions or constraints. This might be based on specific conditions or a range of indices.
Common Array Traversal Algorithms
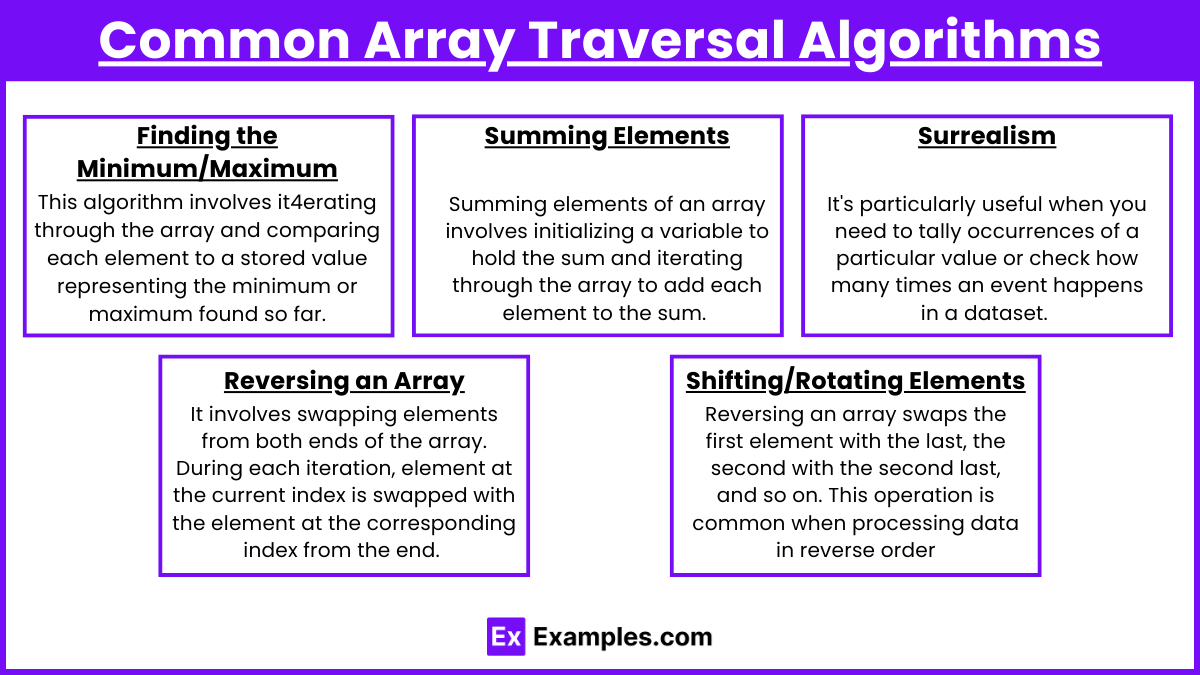
Several standard algorithms rely on traversing arrays:
Finding the Minimum/Maximum: This algorithm involves it4erating through the array and comparing each element to a stored value representing the minimum or maximum found so far. This is essential in problems that require identifying the smallest or largest value within a dataset. You iterate through the array and compare each element with a stored value for the minimum or maximum.
int min = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] < min) {
min = arr[i];
}
}
Similarly, the maximum value can be found by checking if each element is larger than the current maximum.
Summing Elements: Summing elements of an array involves initializing a variable to hold the sum and iterating through the array to add each element to the sum. This is useful for calculating totals, averages, or verifying integrity in numeric datasets. You can sum all the elements in an array by iterating over them.
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
Counting Specific Values: This algorithm counts how often a specific value appears in the array. It’s particularly useful when you need to tally occurrences of a particular value or check how many times an event happens in a dataset. To count how many times a particular value appears, you can traverse the array and increment a counter when the target value is encountered.
int count = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] == targetValue) {
count++;
}
}
Reversing an Array: Reversing an array involves swapping elements from both ends of the array. During each iteration, the element at the current index is swapped with the element at the corresponding index from the end. This process continues until the middle of the array is reached, ensuring that all elements have been reversed.
for (int i = 0; i < arr.length / 2; i++) {
int temp = arr[i];
arr[i] = arr[arr.length - 1 - i];
arr[arr.length - 1 - i] = temp;
}
Shifting/Rotating Elements: Reversing an array swaps the first element with the last, the second with the second last, and so on. This operation is common when processing data in reverse order or when reordering elements is required. You can shift or rotate elements by copying the last element to the first position or moving each element by one index.
int last = arr[arr.length - 1];
for (int i = arr.length - 1; i > 0; i--) {
arr[i] = arr[i - 1];
}
arr[0] = last;
Search Algorithms
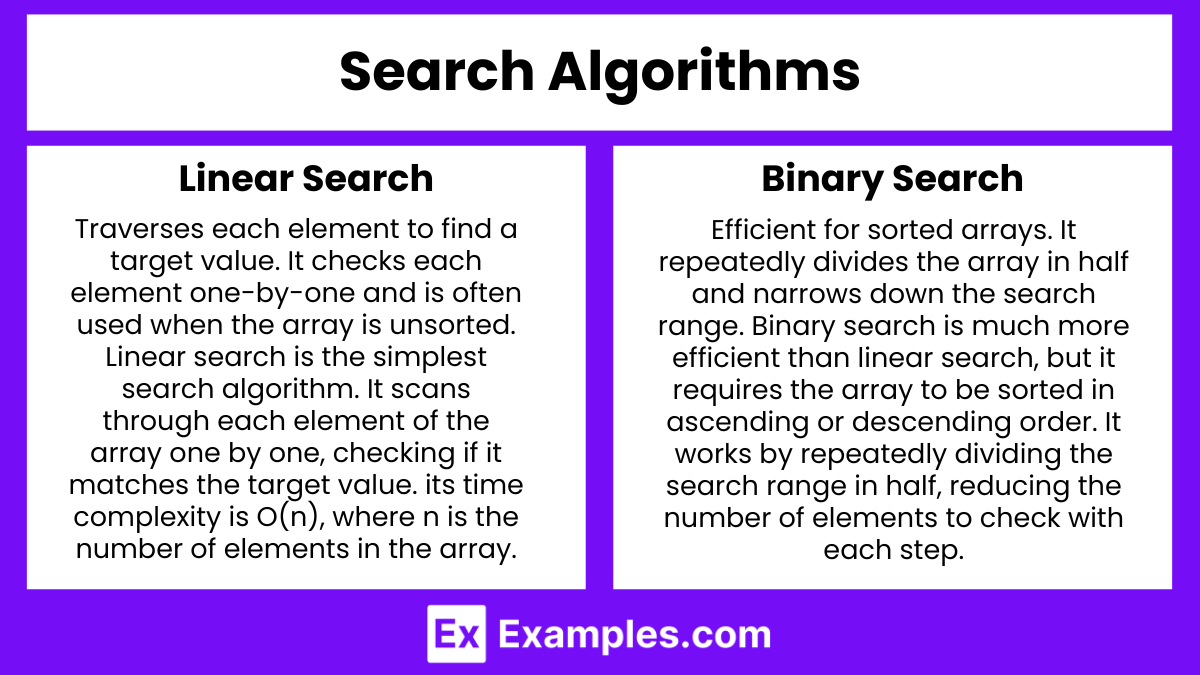
Linear Search: Traverses each element to find a target value. It checks each element one-by-one and is often used when the array is unsorted. Linear search is the simplest search algorithm. It scans through each element of the array one by one, checking if it matches the target value. its time complexity is O(n), where n is the number of elements in the array.
Since it doesn’t rely on the array being sorted, it works for both unsorted and sorted arrays.
boolean found = false;
for (int i = 0; i < arr.length; i++) {
if (arr[i] == targetValue) {
found = true;
break;
}
}
Binary Search: Efficient for sorted arrays. It repeatedly divides the array in half and narrows down the search range. Binary search is much more efficient than linear search, but it requires the array to be sorted in ascending or descending order. It works by repeatedly dividing the search range in half, reducing the number of elements to check with each step. The time complexity of binary search is O(logn), making it highly efficient for large datasets.
int binarySearch(int[] arr, int target) {
int low = 0;
int high = arr.length - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1;
}
Sorting Algorithms
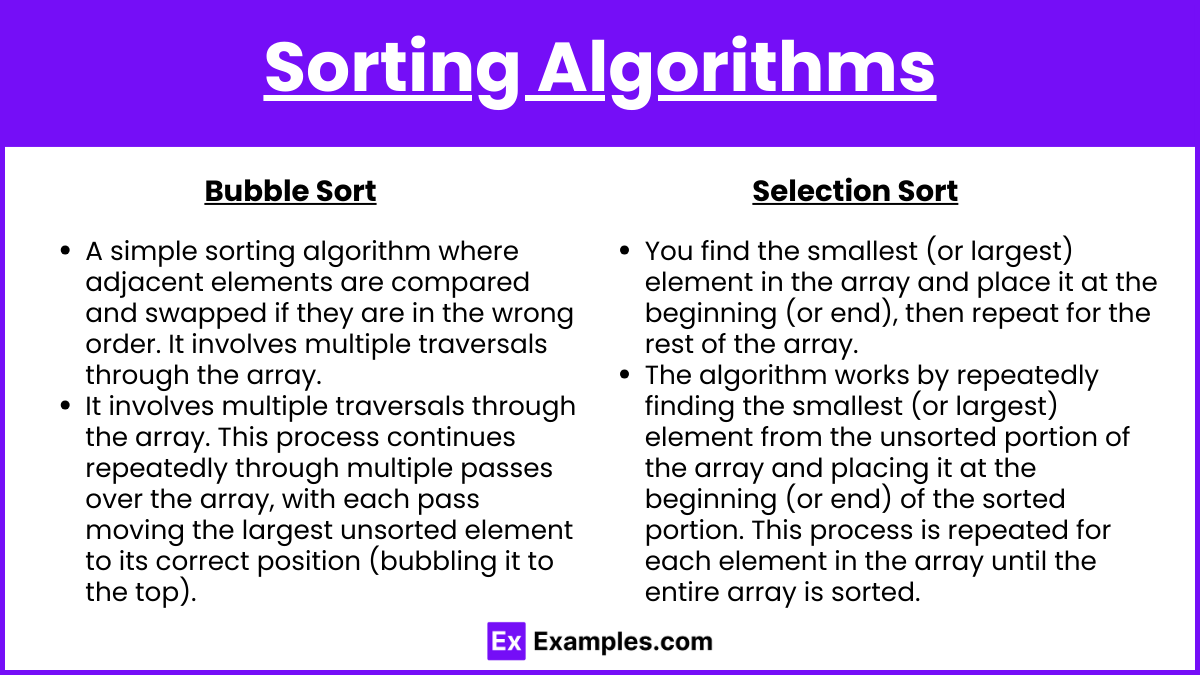
Bubble Sort: A simple sorting algorithm where adjacent elements are compared and swapped if they are in the wrong order. It involves multiple traversals through the array. This process continues repeatedly through multiple passes over the array, with each pass moving the largest unsorted element to its correct position (bubbling it to the top). After each traversal, the next largest element is positioned, so fewer comparisons are needed as the sorted section grows. The algorithm stops when no more swaps are necessary, indicating the array is sorted.
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - 1 - i; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
Selection Sort: You find the smallest (or largest) element in the array and place it at the beginning (or end), then repeat for the rest of the array.The algorithm works by repeatedly finding the smallest (or largest) element from the unsorted portion of the array and placing it at the beginning (or end) of the sorted portion. This process is repeated for each element in the array until the entire array is sorted. Specifically, Selection Sort maintains two subarrays: one that is sorted and one that remains unsorted.
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
Examples
Example 1. Finding the Minimum and Maximum
In this algorithm, the array is traversed to find the smallest or largest element. You maintain a variable to store the current minimum or maximum, starting with the first element. As you iterate through the array, each element is compared with the stored value, and if it’s smaller or larger (depending on the operation), the stored value is updated. By the end of the traversal, the variable holds the minimum or maximum value. This is useful in scenarios like finding the lowest price in a list of products or the highest score in a set of grades.
Example 2. Summing All Elements
This algorithm involves iterating through each element of the array and adding them together to produce a total sum. The sum is initialized to zero, and each element is added to the running total as the loop progresses. This operation is commonly used when calculating the total score in a game, the sum of all expenses in a budget, or any scenario where the accumulation of values is necessary. It also forms the basis for more complex algorithms, such as calculating averages or variances.
Example 3. Counting Occurrences of a Specific Value
In this algorithm, an array is traversed to count how many times a particular value appears. A counter variable is initialized to zero, and for each element in the array, the algorithm checks if it matches the target value. If it does, the counter is incremented. This is helpful for tasks such as counting the frequency of a particular grade, finding how many times a specific character appears in a string, or determining how many people in a dataset match certain criteria.
Example 4. Reversing an Array
This algorithm traverses the array from both ends simultaneously, swapping elements from the beginning with elements from the end until it reaches the middle. By using a simple swap operation, the original order of the array is reversed. Reversing arrays is important in various scenarios, such as reversing a sequence of steps in a pathfinding algorithm, processing data in reverse chronological order, or manipulating image data where pixel arrays need to be inverted.
Example 5. Linear Search
The linear search algorithm traverses the array from the first to the last element to find a target value. It checks each element in sequence, and if a match is found, it returns the index of the target. If the loop reaches the end of the array without finding the target, it indicates that the target isn’t present. This algorithm is straightforward and works on both sorted and unsorted arrays, making it useful in many everyday applications, such as searching for a specific item in a product catalog or checking for the presence of a value in a list of user inputs.
These algorithms highlight how array traversals are foundational in solving common programming tasks efficiently.
Multiple Choice Questions
Question 1
What is the time complexity of finding the maximum value in an unsorted array using a simple traversal algorithm?
A) O(logn)
B) O(1)
C) O(n)
D) O(n2)
Answer: C) O(n)
Explanation: Finding the maximum value in an unsorted array requires visiting each element at least once to compare it with the current maximum. This means that the algorithm must iterate over all nnn elements in the array. Therefore, the time complexity is O(n), as the algorithm performs a constant amount of work (comparison) for each element. The other time complexities:
- O(logn) is incorrect because logarithmic time is characteristic of algorithms that reduce the problem size at each step, like binary search.
- O(1) is incorrect because that refers to constant time, which would mean the operation does not depend on the size of the array.
- O(n2) is incorrect because it represents quadratic time, which is typical of more complex operations like nested loops, not a simple traversal.
Question 2
Which of the following best describes how a selection sort algorithm works using array traversal?
A) Traverse the array from end to start, placing the largest unsorted element at the end of the array.
B) Traverse the array, compare adjacent elements, and swap them if they are in the wrong order.
C) Traverse the array to find the minimum unsorted element and place it at the beginning of the array.
D) Traverse the array once, and the array is sorted.
Answer: C) Traverse the array to find the minimum unsorted element and place it at the beginning of the array.
Explanation: Selection sort works by dividing the array into a sorted and an unsorted section. In each iteration, the algorithm finds the minimum element from the unsorted portion and swaps it with the first unsorted element, thus growing the sorted portion one element at a time. This is repeated until the entire array is sorted.
- Option A describes a reverse traversal, which isn’t accurate for selection sort.
- Option B describes bubble sort, which compares and swaps adjacent elements.
- Option D is incorrect because selection sort requires multiple passes to sort the array fully, not just a single traversal.
Question 3
Which of the following statements is true about linear search?
A) Linear search is only efficient for sorted arrays.
B) Linear search checks all elements before concluding whether the target value is present or not.
C) Linear search can terminate early if the target value is found.
D) Linear search has a worst-case time complexity of O(logn).
Answer: C) Linear search can terminate early if the target value is found.
Explanation: Linear search sequentially checks each element in the array, but it can terminate early if the target value is found before reaching the end of the array. This improves the average-case performance, but the worst-case time complexity remains O(n), where nnn is the number of elements in the array.
- Option A is incorrect because linear search is typically used on unsorted arrays, although it works on both sorted and unsorted arrays.
- Option B is incorrect because linear search can terminate early as soon as it finds the target value, rather than checking all elements.
- Option D is incorrect because linear search has a worst-case time complexity of O(n), not O(logn), which is typical of binary search.