In the AP Computer Science A curriculum, mastering standard algorithms that utilize ArrayList traversals is essential. These algorithms encompass a range of tasks such as searching for elements, calculating sums, filtering based on specific conditions, and reversing the order of elements. Proficiency in these operations not only enhances problem-solving skills but also prepares students for complex programming challenges. By understanding and applying these algorithms, students can effectively manage and manipulate data collections, a critical skill for any aspiring computer scientist.
Learning Objectives
In preparing for the AP Computer Science A exam on the topic of “Standard Algorithms that Utilize ArrayList Traversals to Perform Functions,” you should aim to master several key objectives. Understand how to iterate over ArrayLists to perform calculations like sum, average, or finding minimum and maximum values. Learn to implement search algorithms to locate elements and conditions to filter data. Practice writing algorithms to reverse ArrayLists and recognize patterns in array manipulations. Gain proficiency in applying these concepts to solve practical programming problems efficiently, leveraging ArrayList traversals to manipulate and evaluate large data sets.
Standard Algorithms Utilizing ArrayList Traversals
Finding Maximum or Minimum
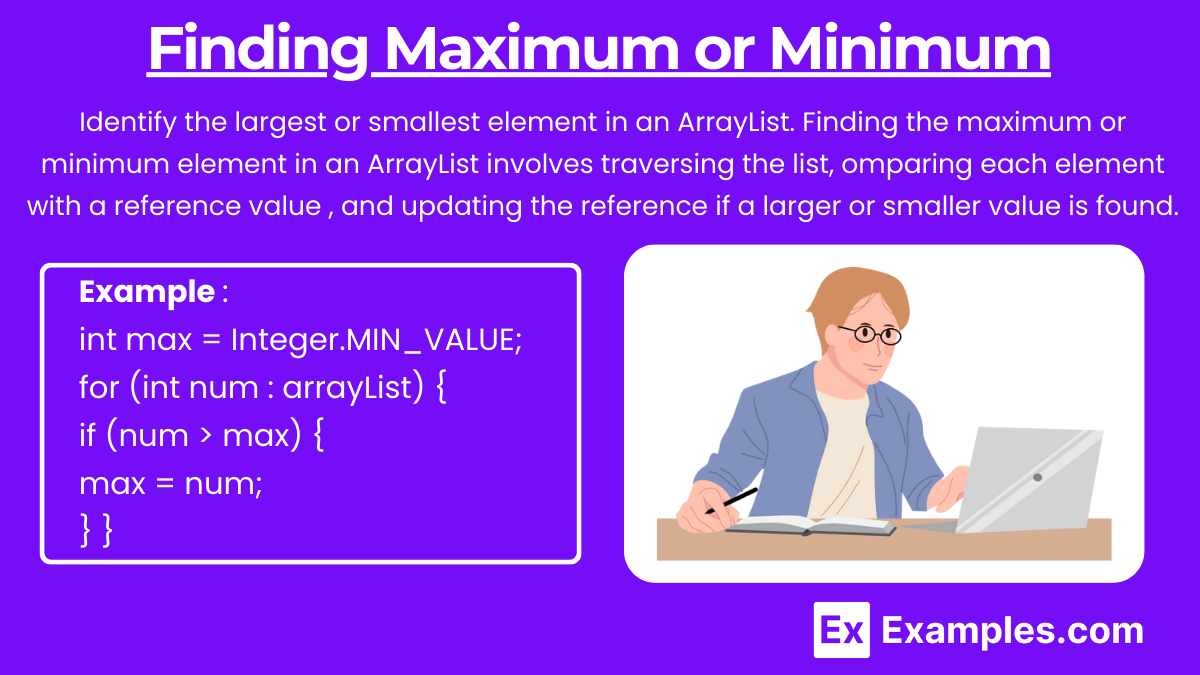
Identify the largest or smallest element in an ArrayList. Finding the maximum or minimum element in an ArrayList involves traversing the list, comparing each element with a reference value (initialized to the first element), and updating the reference if a larger (for maximum) or smaller (for minimum) value is found. This is a fundamental operation in many algorithms, such as finding the highest score, the largest number, or identifying the lowest value in a data set.
Process: Initialize a variable (max or min) to the value of the first element.Traverse the ArrayList using a loop.
Compare each element with max or min and update max or min accordingly. Maximum: If the current element is greater than the current max, update max to this element.Minimum: If the current element is smaller than the current min, update min to this element.
Example Code:
int max = Integer.MIN_VALUE;
for (int num : arrayList) {
if (num > max) {
max = num;
}
}
Search for an Element
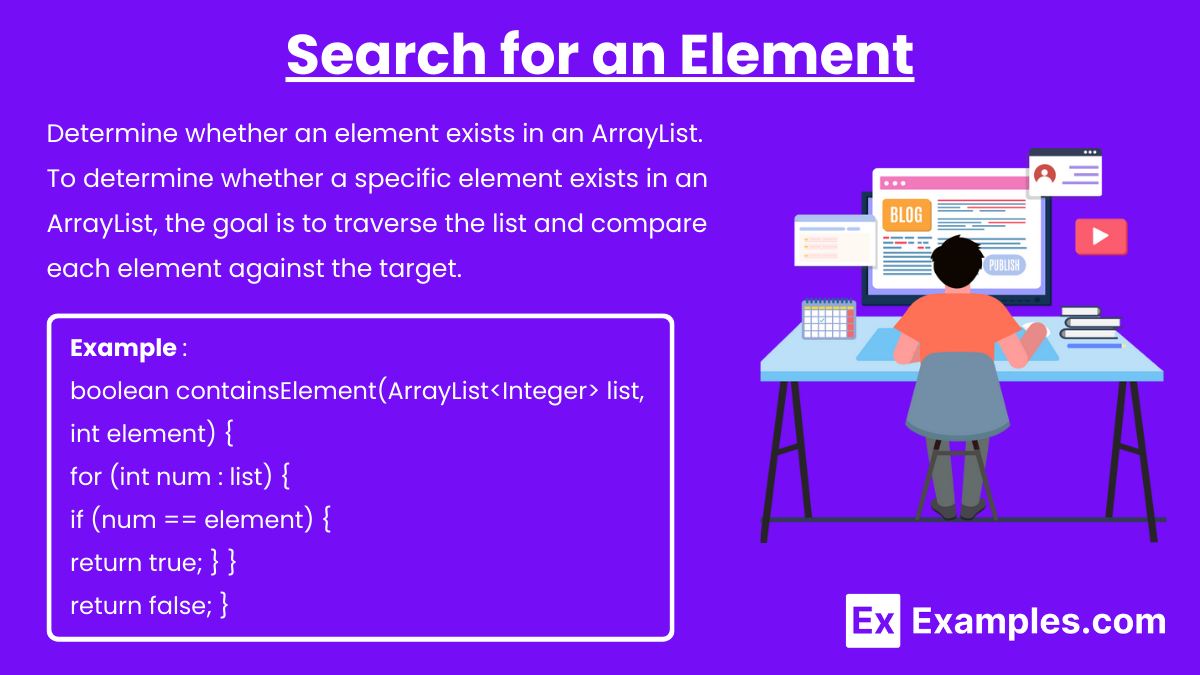
Objective: Determine whether an element exists in an ArrayList. To determine whether a specific element exists in an ArrayList, the goal is to traverse the list and compare each element against the target. The method should return a boolean value—true if the element is found, and false if it is not.
Process: Traverse the ArrayList using a loop. If the element is found, return true; otherwise, return false after the loop ends. Start at the beginning of the ArrayList and use a loop to examine each element in sequence.
Example Code:
boolean containsElement(ArrayList<Integer> list, int element) {
for (int num : list) {
if (num == element) {
return true;
}
}
return false;
}
Calculate Sum or Average
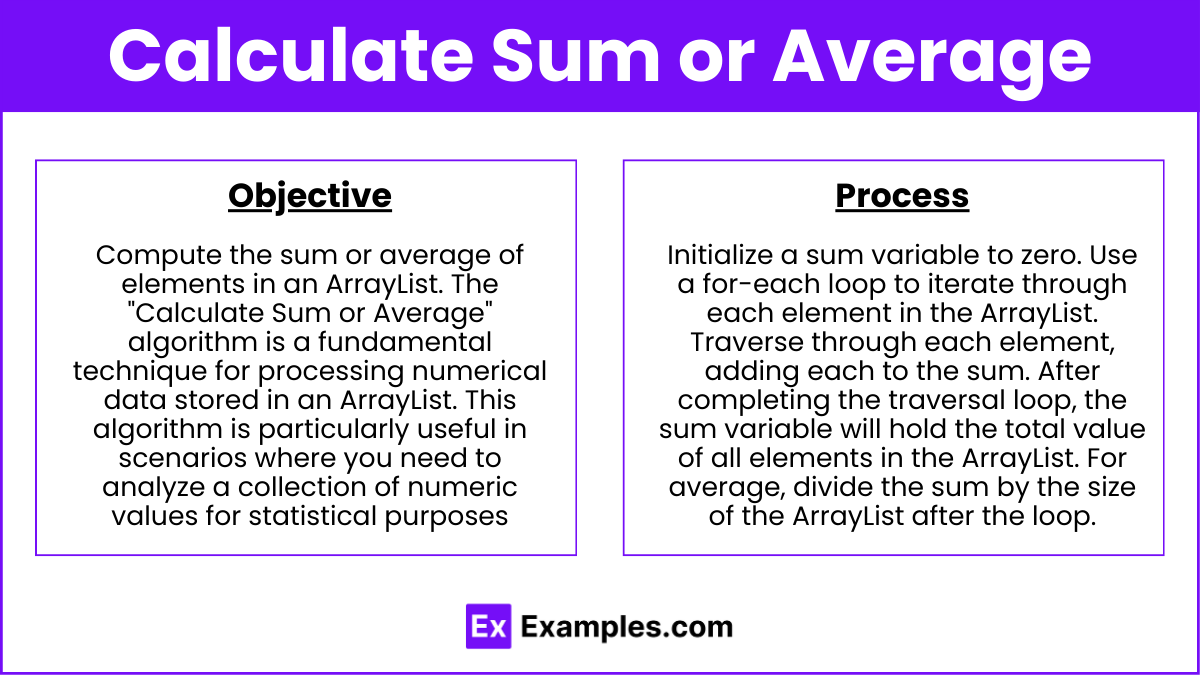
Objective: Compute the sum or average of elements in an ArrayList. The “Calculate Sum or Average” algorithm is a fundamental technique for processing numerical data stored in an ArrayList. This algorithm is particularly useful in scenarios where you need to analyze a collection of numeric values for statistical purposes, such as finding the total or average in sets of numbers.
Process: Initialize a sum variable to zero. Use a for-each loop to iterate through each element in the ArrayList. Traverse through each element, adding each to the sum. After completing the traversal loop, the sum variable will hold the total value of all elements in the ArrayList.For average, divide the sum by the size of the ArrayList after the loop.
Example Code:
int sum = 0;
for (int num : arrayList) {
sum += num;
}
int average = sum / arrayList.size();
Filtering Based on Condition
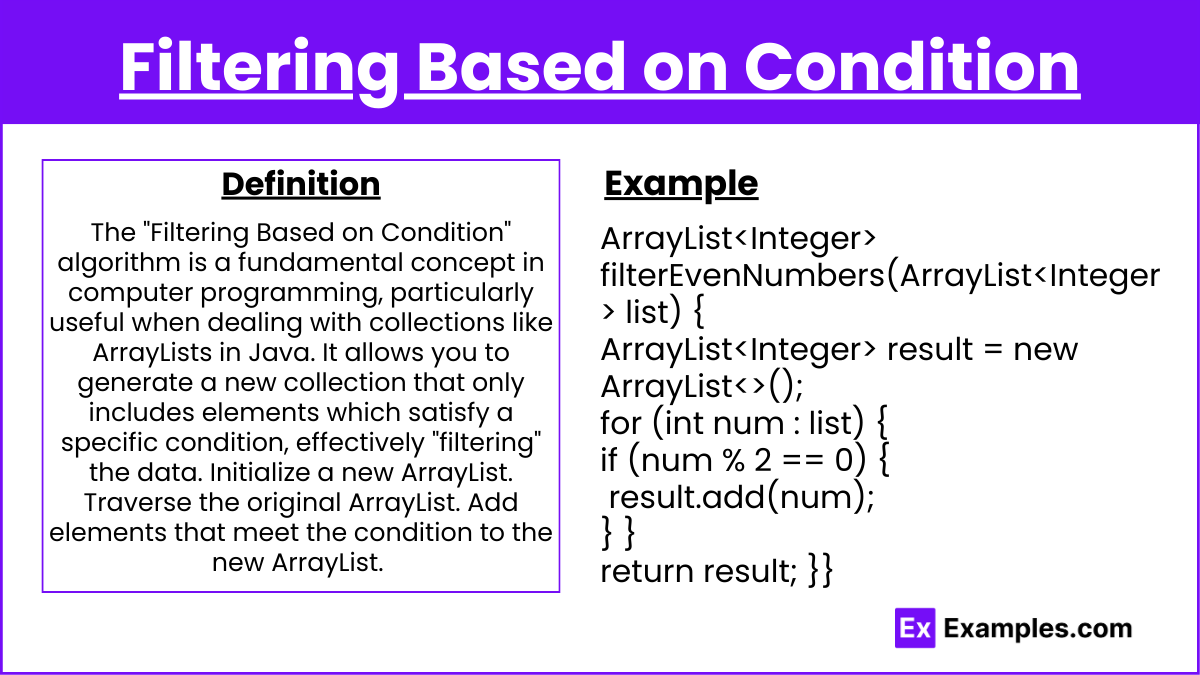
Objective: The “Filtering Based on Condition” algorithm is a fundamental concept in computer programming, particularly useful when dealing with collections like ArrayLists in Java. It allows you to generate a new collection that only includes elements which satisfy a specific condition, effectively “filtering” the data. Create a new ArrayList containing elements that meet a specific condition.
Process: Initialize a new ArrayList. Traverse the original ArrayList. Add elements that meet the condition to the new ArrayList. To create a new ArrayList that consists solely of elements from the original list that meet a predetermined condition.
Example Code:
ArrayList<Integer> filterEvenNumbers(ArrayList<Integer> list) {
ArrayList<Integer> result = new ArrayList<>();
for (int num : list) {
if (num % 2 == 0) {
result.add(num);
}
}
return result;
}
Reversing an ArrayList
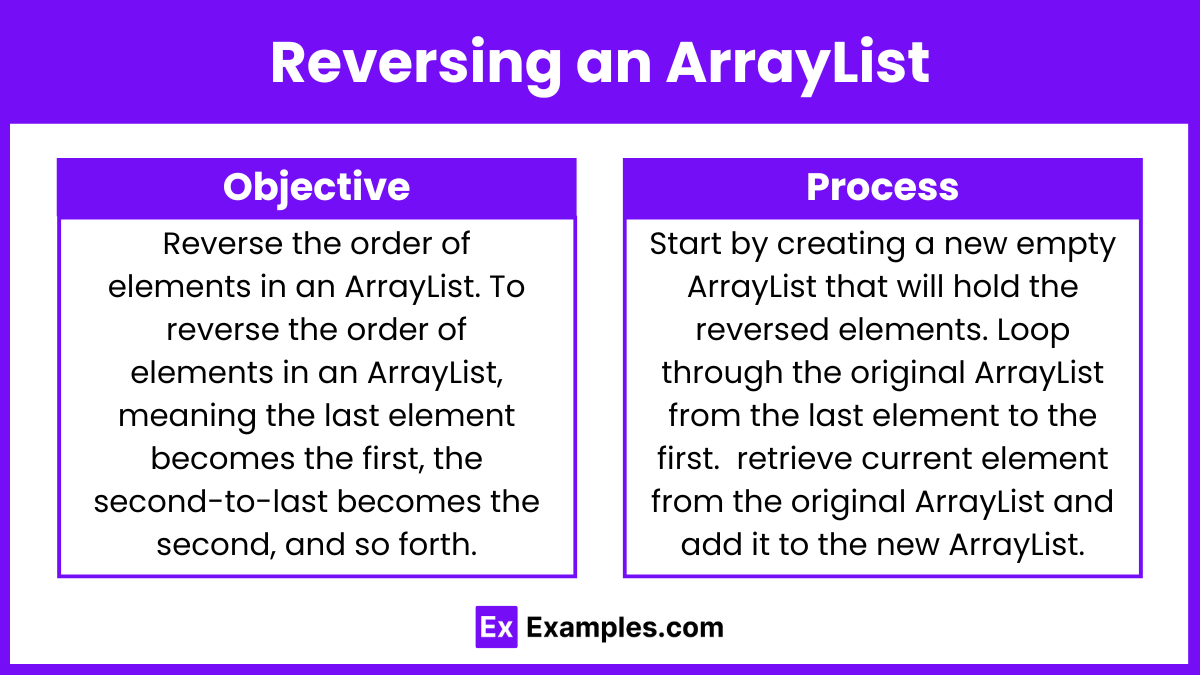
Objective: Reverse the order of elements in an ArrayList. To reverse the order of elements in an ArrayList, meaning the last element becomes the first, the second-to-last becomes the second, and so forth.
Process: Start by creating a new empty ArrayList that will hold the reversed elements. Loop through the original ArrayList from the last element to the first. During each iteration of the loop, retrieve the current element from the original ArrayList and add it to the new ArrayList.
Example Code
ArrayList<Integer> reverseArrayList(ArrayList<Integer> list) {
ArrayList<Integer> reversed = new ArrayList<>();
for (int i = list.size() - 1; i >= 0; i--) {
reversed.add(list.get(i));
}
return reversed;
}
Examples
Example 1: Sorting an ArrayList
This algorithm reorganizes the elements of an ArrayList into a specified order, either ascending or descending. The traversal involves comparing each element with others to determine their relative order. A common method for sorting in Java is using the Collections.sort() method, but implementing manual sorting like bubble sort or selection sort involves nested loops where each element is compared and possibly swapped until the entire list is ordered.
Example 2: Finding Duplicates
To find duplicates in an ArrayList, the algorithm traverses through the list, maintaining a separate collection (like a HashSet) to track elements that have already been seen. As the ArrayList is traversed, each element is checked against the set. If an element is already in the set, it is a duplicate; otherwise, it is added to the set. This helps in identifying all repeated elements in the ArrayList.
Example 3: Merging Two Sorted ArrayLists
This algorithm involves traversing two sorted ArrayLists simultaneously to merge them into a single sorted ArrayList. The process typically uses two indices tracking the current position in each list, comparing the elements at these positions, and adding the smaller (or larger, depending on desired order) element to a new ArrayList. This continues until all elements from both lists are exhausted.
Example 4: Reversing Elements in Place
Unlike creating a new ArrayList to hold reversed elements, this algorithm modifies the ArrayList in place. It involves traversing the ArrayList from both ends towards the center, swapping elements at the start index with those at the end index, incrementally moving towards the middle. This way, the ArrayList is reversed without needing additional storage.
Example 5: Counting Elements Matching a Condition
This function counts the number of elements in an ArrayList that meet a specific condition, such as being greater than a certain value or matching a string pattern. The ArrayList is traversed using a loop, and for each element, a condition check is performed. A counter is incremented each time an element satisfies the condition, resulting in the total count of matching elements after the traversal is complete.
Multiple Choice Questions
Question 1: Finding an Element
What does the following Java method do?
boolean findElement(ArrayList<String> list, String target) {
for (String item : list) {
if (item.equals(target)) {
return true;
}
}
return false;
}
A) Adds a target element to the list.
B) Returns true if the target element is in the list, otherwise false.
C) Counts how many times the target appears in the list.
D) Deletes the target element from the list.
Correct Answer: B
Explanation: This method traverses the entire ArrayList using a for-each loop. During each iteration, it checks if the current item is equal to the target. If it finds a match, it immediately returns true, indicating the presence of the target in the list. If the loop completes without finding the target, the method returns false. The method does not modify the list or count occurrences, only checks for existence.
Question 2: Calculating the Sum
What will the following method return when passed an ArrayList containing the elements [1, 2, 3, 4, 5]?
int calculateSum(ArrayList<Integer> numbers) {
int sum = 0;
for (int num : numbers) {
sum += num;
}
return sum;
}
A) 10
B) 15
C) 5
D) 20
Correct Answer: B
Explanation: This method calculates the sum of all elements in the ArrayList. It initializes a sum variable to zero and then iterates through each element in the ArrayList, adding each element to the sum. For the list [1, 2, 3, 4, 5], the sum would be 1+2+3+4+5=151+2+3+4+5=151+2+3+4+5=15.
Question 3: Filtering Elements
Which of the following methods correctly filters and returns only even numbers from an ArrayList of integers?
// Method A
ArrayList<Integer> filterEvens(ArrayList<Integer> list) {
ArrayList<Integer> evens = new ArrayList<>();
for (int num : list) {
if (num % 2 == 0) {
evens.add(num);
}
}
return evens;
}
// Method B
ArrayList<Integer> filterEvens(ArrayList<Integer> list) {
ArrayList<Integer> evens = new ArrayList<>();
for (int num : list) {
if (num % 2 != 0) {
evens.add(num);
}
}
return evens;
}
A) Method A
B) Method B
C) Both Method A and Method B
D) Neither Method A nor Method B
Correct Answer: A
Explanation: Method A is the correct implementation for filtering even numbers. It checks if each number in the ArrayList is divisible by 2 (i.e., num % 2 == 0). If true, the number is even, and it adds the number to the new ArrayList ‘evens’. Method B, on the other hand, incorrectly checks for odd numbers (i.e., num % 2 != 0) and would add only odd numbers to ‘evens’.