In AP Computer Science A, a class defines the blueprint for creating objects, encapsulating data and behavior. The attributes (fields) of a class can be declared as public or private using access modifiers. Public attributes can be accessed directly from outside the class, while private attributes are restricted to the class itself. By using private attributes, developers ensure data integrity through encapsulation, allowing controlled access via public getter and setter methods. This design principle promotes organized, secure, and maintainable code in object-oriented programming.
Learning Objectives
In studying the makeup of a class and the distinction between public and private attributes for AP Computer Science A, you should learn how to define and implement a class, use access modifiers (public and private) to control access to attributes, and understand the importance of encapsulation in object-oriented programming. You should also be able to create and use getter and setter methods to manage private data, apply the concept of data integrity, and recognize the role of public and private methods within class structures.
A class in Java is a blueprint for creating objects, defining their attributes (variables) and behaviors (methods). Each class typically has:
Attributes (Fields/Instance Variables)
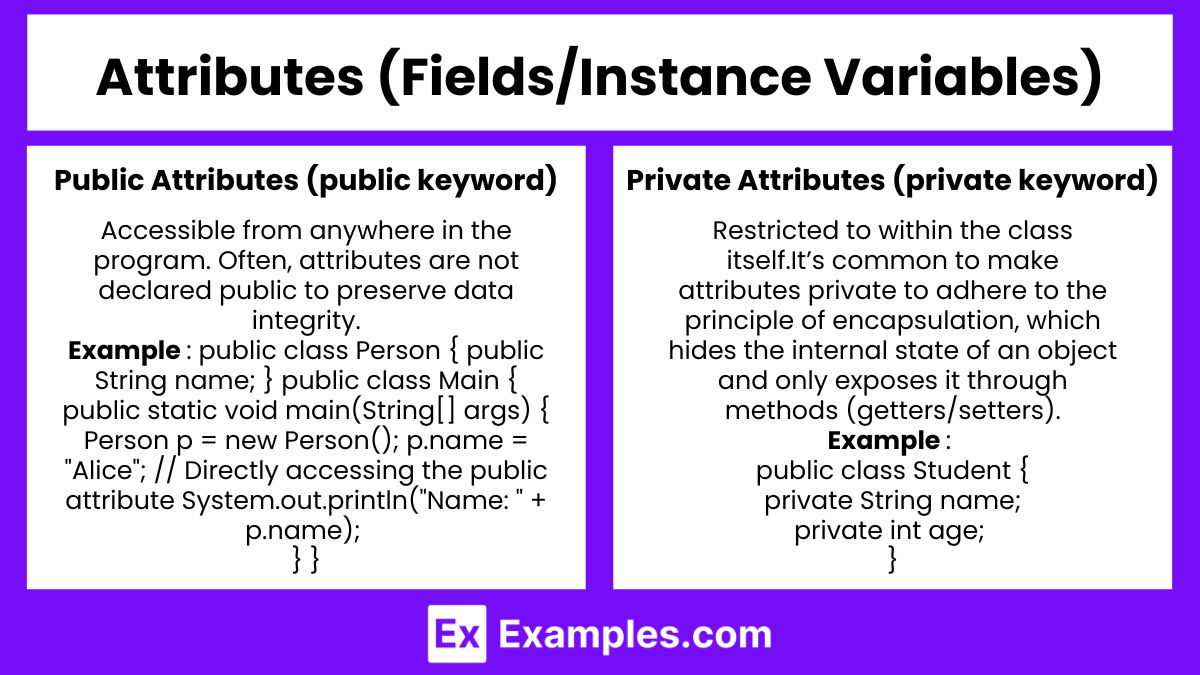
These represent the data or state of the object. Attributes can be of various data types, like int, double, or String. The access to these attributes is controlled using access modifiers:
- Public Attributes (public keyword):
- Accessible from anywhere in the program.
- Often, attributes are not declared public to preserve data integrity.
public class Person {
public String name;
}
public class Main {
public static void main(String[] args) {
Person p = new Person();
p.name = "Alice"; // Directly accessing the public attribute
System.out.println("Name: " + p.name);
}
}
- Private Attributes (private keyword):
- Restricted to within the class itself.It’s common to make attributes private to adhere to the principle of encapsulation, which hides the internal state of an object and only exposes it through methods (getters/setters).
public class Student {
private String name;
private int age;
}
In the example above, both name and age are private, meaning they cannot be accessed directly from outside the Student class.
Accessors (Getters) and Mutators (Setters)
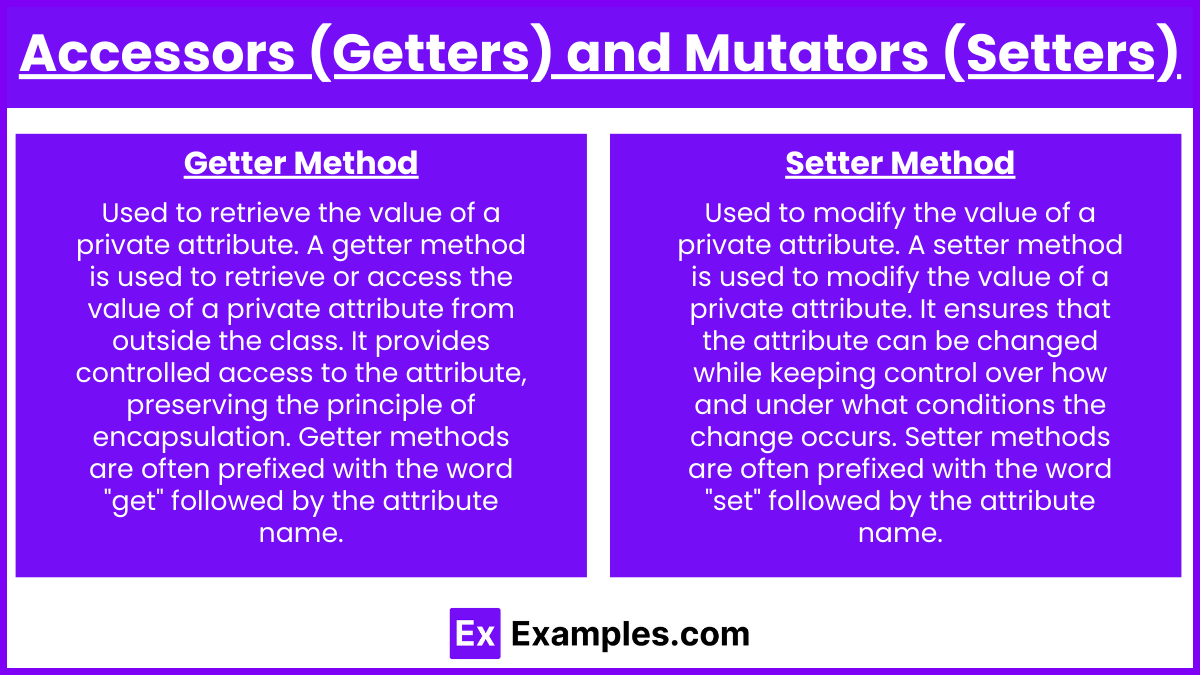
Since private attributes cannot be accessed directly, you use public methods to get and set the values of these private attributes.
Getter Method: Used to retrieve the value of a private attribute. A getter method is used to retrieve or access the value of a private attribute from outside the class. It provides controlled access to the attribute, preserving the principle of encapsulation. Getter methods are often prefixed with the word “get” followed by the attribute name.
public class Student {
private String name; // Private attribute
// Getter method
public String getName() {
return name; // Returns the value of the private attribute
}
}
Setter Method: Used to modify the value of a private attribute. A setter method is used to modify the value of a private attribute. It ensures that the attribute can be changed while keeping control over how and under what conditions the change occurs. Setter methods are often prefixed with the word “set” followed by the attribute name.
public class Student {
private String name; // Private attribute
// Setter method
public void setName(String newName) {
this.name = newName; // Updates the private attribute with the new value
}
}
Why Private Attributes?
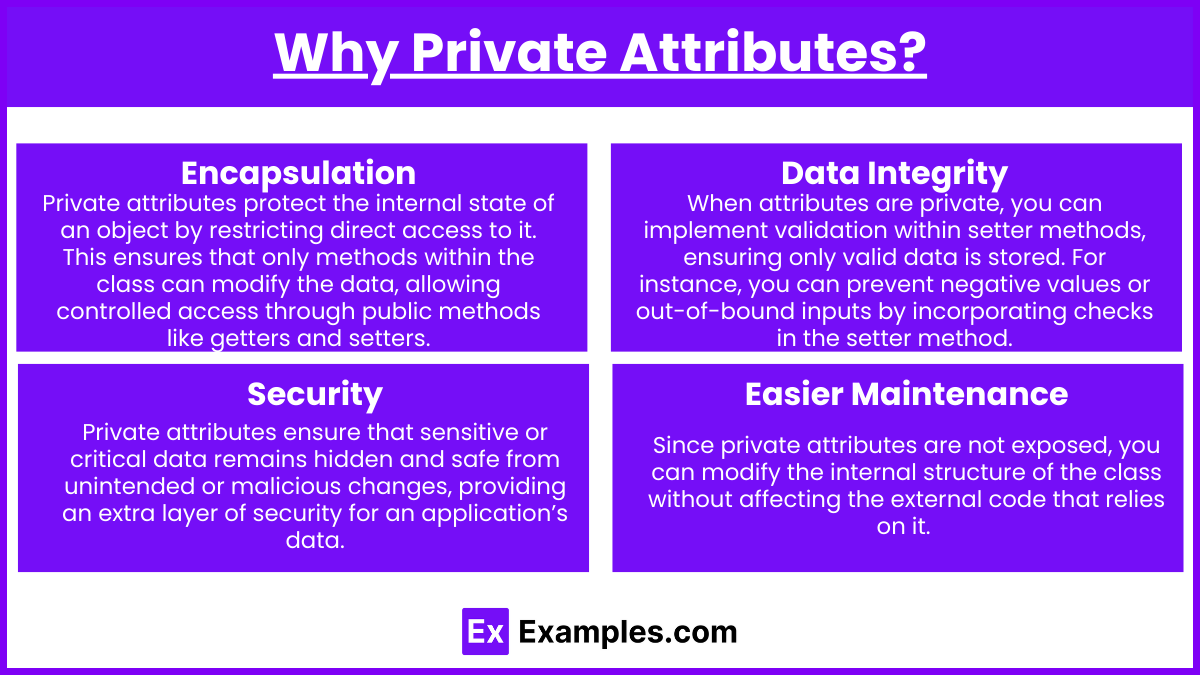
- Encapsulation: Private attributes protect the internal state of an object by restricting direct access to it. This ensures that only methods within the class can modify the data, allowing controlled access through public methods like getters and setters. This helps preserve the integrity of the data and the design of the class.
- Data Integrity: When attributes are private, you can implement validation within setter methods, ensuring only valid data is stored. For instance, you can prevent negative values or out-of-bound inputs by incorporating checks in the setter method. This prevents invalid or harmful modifications.
- Security: Private attributes ensure that sensitive or critical data remains hidden and safe from unintended or malicious changes, providing an extra layer of security for an application’s data.
- Easier Maintenance: Since private attributes are not exposed, you can modify the internal structure of the class without affecting the external code that relies on it. Changes to private data can be managed internally without requiring changes to the external code that uses the class.
Examples
Example 1 : Student Class Example
In a class representing students, the attributes like name, age, and studentID can be declared as private to ensure the integrity of the data. Public getter and setter methods can be provided to control access to these private attributes. For instance, a getName() method allows external classes to view the student’s name, while a setName(String name) method can be used to change the name. This approach encapsulates the data and provides controlled access.
Example 2: BankAccount Class Example
In a BankAccount class, attributes like accountNumber, balance, and owner are often made private to prevent direct modification from outside the class. The deposit() and withdraw() methods can be public, allowing controlled changes to the balance, while private attributes maintain the security and integrity of the account details. Additionally, providing public getter methods like getBalance() gives access to the balance without allowing direct modification.
Example 3: Car Class Example
For a Car class, attributes like make, model, and speed can be private to prevent external modification. Public methods like accelerate() or brake() can alter the car’s speed, ensuring that the speed attribute cannot be set to invalid values directly. For example, a setter method for speed might check that the speed is within a valid range before updating the attribute. This way, the class controls the valid behavior of the car’s properties.
Example 4: Employee Class Example
In an Employee class, attributes like salary, position, and employeeID are commonly private. Access to these attributes can be managed through public methods like getSalary() and setSalary(double salary). This ensures that the employee’s sensitive data, like salary, is not directly accessible or modifiable by external classes, thereby adhering to the principles of encapsulation.
Example 5: LibraryBook Class Example
A LibraryBook class might include attributes like title, author, and isAvailable. These attributes can be private, ensuring that they are only modified through public methods like checkoutBook() or returnBook(). For instance, the isAvailable attribute is private, and only the methods controlling the checkout and return process can modify its value, ensuring the correct availability status of the book at all times.
Multiple Choice Questions
Question 1
Which of the following is true about the private keyword when used with attributes in a Java class?
A) It allows the attribute to be accessed from anywhere in the program.
B) It restricts access to the attribute to methods within the same class.
C) It makes the attribute accessible to any class in the same package.
D) It prevents any class from accessing or modifying the attribute, including its own class.
Answer: B) It restricts access to the attribute to methods within the same class.
Explanation: The private keyword is used to restrict access to attributes so that they can only be accessed from within the same class. This helps in achieving encapsulation, one of the fundamental principles of object-oriented programming. The other options are incorrect:
- Option A is describing the behavior of the public keyword.
- Option C describes the behavior of the protected keyword, which allows access to other classes in the same package.
- Option D is incorrect because the class itself can still access and modify its private attributes.
Question 2
Why would a class use getter and setter methods for its private attributes?
A) To allow direct access to the private attributes from other classes.
B) To adhere to the principle of inheritance in object-oriented programming.
C) To allow controlled access and modification of private attributes.
D) To prevent the class from being instantiated.
Answer: C) To allow controlled access and modification of private attributes.
Explanation: Getter and setter methods are used to provide controlled access to private attributes of a class. The getter method retrieves the value of the attribute, while the setter method allows for modifying it. This approach maintains data integrity by adding a layer of control, ensuring that attributes are not altered directly but through defined methods that can validate input. The other options are incorrect:
- Option A is false because direct access to private attributes from other classes is not allowed.
- Option B refers to inheritance, which is not related to getters and setters.
- Option D is irrelevant, as getters and setters have no impact on whether a class can be instantiated.
Question 3
Given the following code, what will happen if another class tries to access the name attribute directly?
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
A) The name attribute will be accessed directly because it’s initialized in the constructor.
B) An error will occur because the name attribute is private and cannot be accessed directly.
C) The name attribute will be accessed directly since it has getter and setter methods.
D) The code will compile without errors, and the name attribute will be publicly accessible.
Answer: B) An error will occur because the name attribute is private and cannot be accessed directly.
Explanation: The name attribute in the Person class is marked as private, which means it cannot be accessed directly by any other class. If another class tries to access name (e.g., person.name), a compile-time error will occur. Access to name is only allowed through the public getter and setter methods (getName() and setName()), ensuring the principle of encapsulation. The other options are incorrect:
- Option A is wrong because initializing an attribute in a constructor does not change its access modifier.
- Option C is wrong because having getter and setter methods does not allow direct access to private attributes.
- Option D is incorrect because the private attribute remains inaccessible outside the class unless accessed through the provided methods.