Traversing a 2D array using nested iteration statements is a key concept in AP Computer Science A. A 2D array consists of rows and columns, and each element can be accessed using two indices: one for the row and one for the column. This traversal involves using an outer loop to iterate over the rows and an inner loop to access each element in a row. Understanding this technique is essential for solving problems that involve performing operations on all elements of a 2D array efficiently.
Learning Objectives
When learning to traverse a 2D array using nested iteration statements for the AP Computer Science A exam, you should focus on understanding how to access and manipulate each element of a 2D array efficiently. Learn how nested loops interact, with the outer loop controlling rows and the inner loop controlling columns. Practice applying this concept to perform tasks such as summing elements, finding specific values, and iterating over both rectangular and jagged arrays, while ensuring optimal code structure and avoiding common errors like out-of-bound indices.
Traversing a 2D Array by Accessing the Elements Using Nested Iteration Statements
In a 2D array, each element is accessed through two indices: one for the row and one for the column. Traversing a 2D array means visiting each element in the array and performing some operation, often using nested loops. Here’s how you can traverse a 2D array using nested iteration statements.
Structure of a 2D Array
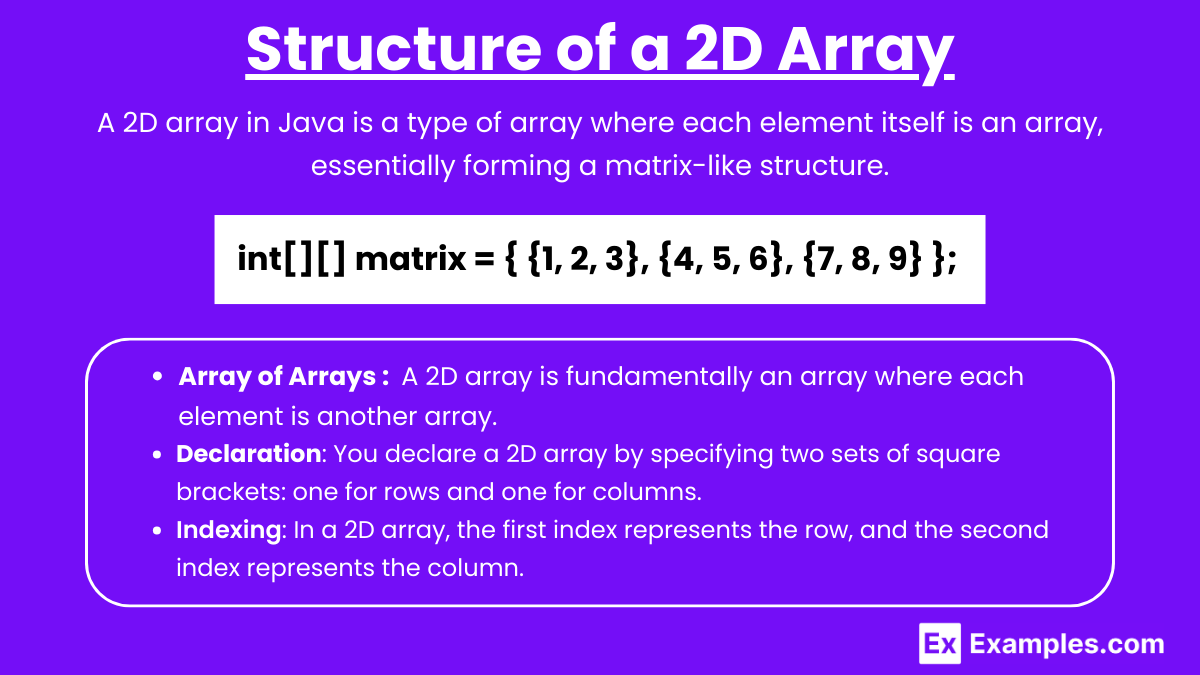
A 2D array in Java is a type of array where each element itself is an array, essentially forming a matrix-like structure. It allows you to store data in rows and columns, which can be especially useful for representing grid-based data such as tables, images, or matrices in mathematical operations. A 2D array in Java is essentially an array of arrays. You can visualize it as a table with rows and columns:
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Here, matrix has 3 rows and 3 columns.
Key Characteristics:
- Array of Arrays: A 2D array is fundamentally an array where each element is another array. This array can have different dimensions in both rows and columns, depending on how you define it.
- Declaration: You declare a 2D array by specifying two sets of square brackets: one for rows and one for columns.
- Indexing: In a 2D array, the first index represents the row, and the second index represents the column. For example, in matrix[2][1], the first index (2) refers to the third row, and the second index (1) refers to the second column.
Nested Loops for Traversing
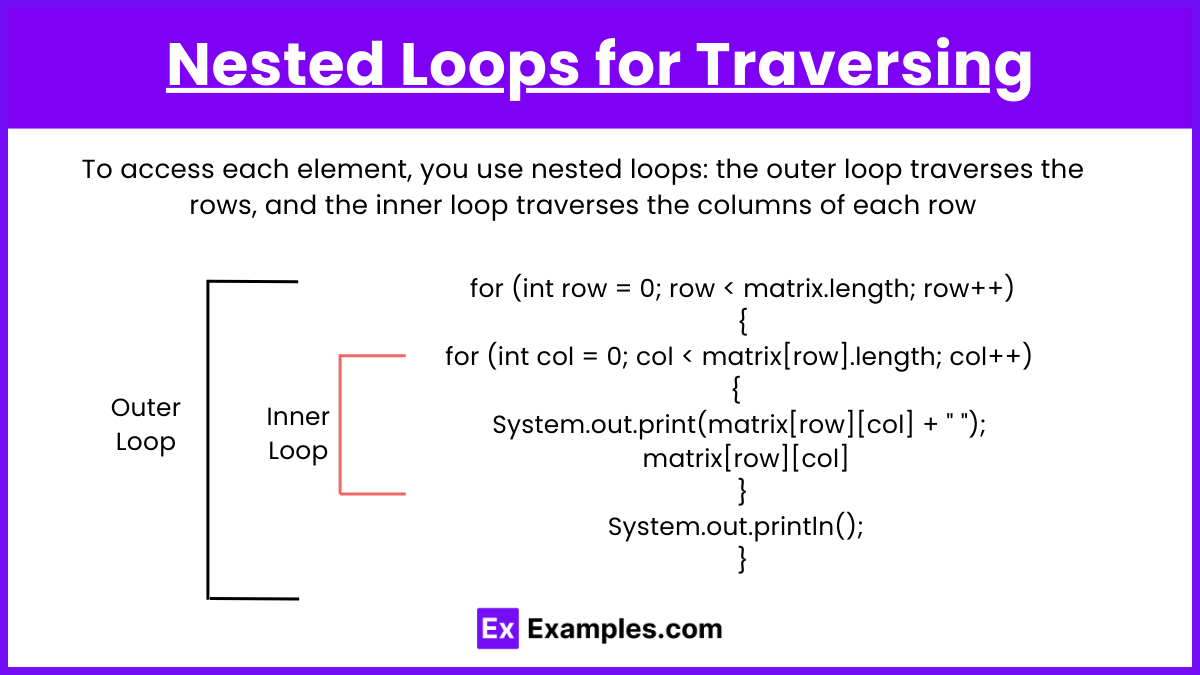
To access each element, you use nested loops: the outer loop traverses the rows, and the inner loop traverses the columns of each row.
for (int row = 0; row < matrix.length; row++) { // Outer loop for rows
for (int col = 0; col < matrix[row].length; col++) { // Inner loop for columns
System.out.print(matrix[row][col] + " "); // Access element at matrix[row][col]
}
System.out.println(); // Moves to the next line after each row
}
In the above code:
- The outer loop runs through each row of the 2D array.
- The inner loop accesses each element in the current row.
- The element at matrix[row][col] is accessed for each combination of row and column indices.
Important Points:
- Outer Loop (Rows): The outer loop (for (int row = 0; row < matrix.length; row++)) iterates over each row of the array. matrix.length gives the number of rows.
- Inner Loop (Columns): The inner loop (for (int col = 0; col < matrix[row].length; col++)) iterates over each element in a particular row. matrix[row].length gives the number of columns in that row, which is useful in case the 2D array has rows of unequal lengths (jagged array).
- Accessing Elements: Each element is accessed via matrix[row][col] where row represents the current row and col represents the current column.
Nested Iteration for Different Operations
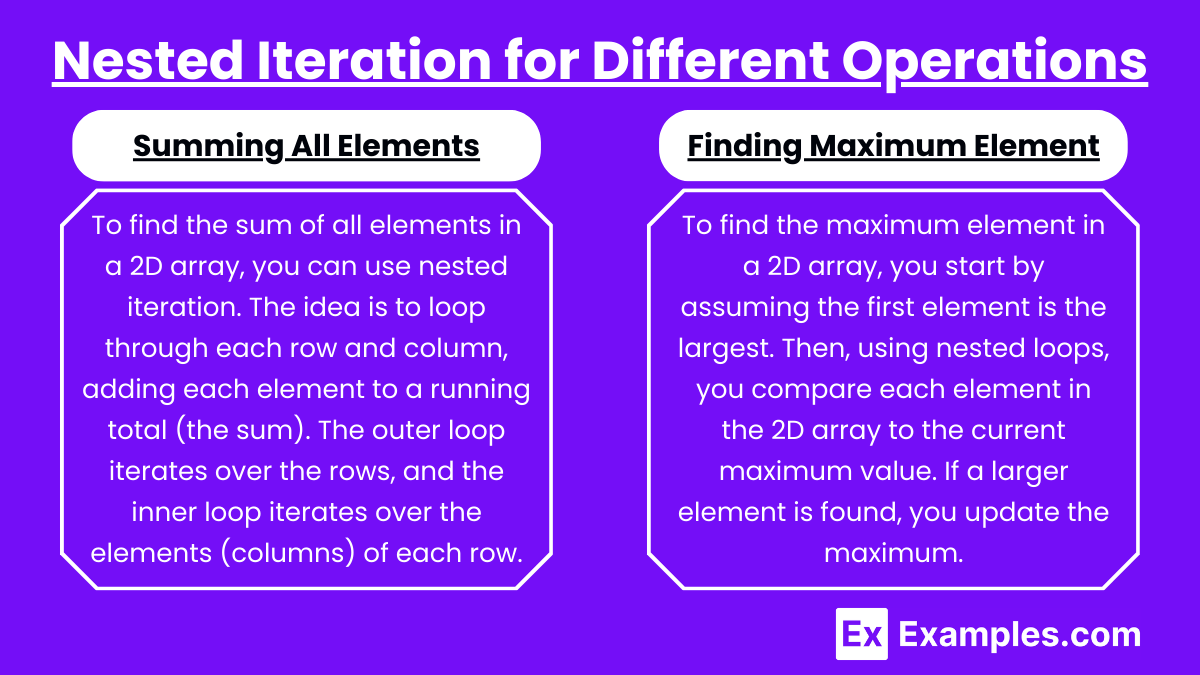
- Summing All Elements: To find the sum of all elements in a 2D array, you can use nested iteration. The idea is to loop through each row and column, adding each element to a running total (the sum). The outer loop iterates over the rows, and the inner loop iterates over the elements (columns) of each row.
int sum = 0;
for (int row = 0; row < matrix.length; row++) {
for (int col = 0; col < matrix[row].length; col++) {
sum += matrix[row][col];
}
}
System.out.println("Sum: " + sum);
- Finding Maximum Element: To find the maximum element in a 2D array, you start by assuming the first element is the largest. Then, using nested loops, you compare each element in the 2D array to the current maximum value. If a larger element is found, you update the maximum.
int max = matrix[0][0];
for (int row = 0; row < matrix.length; row++) {
for (int col = 0; col < matrix[row].length; col++) {
if (matrix[row][col] > max) {
max = matrix[row][col];
}
}
}
System.out.println("Max: " + max);
Key Considerations:
- Jagged Arrays: In some cases, arrays may have different lengths for each row. Always ensure you use matrix[row].length to avoid ArrayIndexOutOfBoundsException.
- Efficiency: Nested loops operate with a time complexity of O(n * m), where n is the number of rows and m is the number of columns.
- Enhanced for Loop: You can also use enhanced for loops for simplicity:
for (int[] row : matrix) {
for (int element : row) {
System.out.print(element + " ");
}
System.out.println();
}
Understanding and practicing this pattern will help you manage complex 2D array problems in the AP Computer Science A exam effectively.
Examples
Example 1: Counting Specific Elements in a 2D Array
Suppose you need to count how many times a specific value, such as 5, appears in a 2D array. Using nested loops, the outer loop iterates through the rows, while the inner loop checks each element in the columns. Each time the value 5 is found, a counter is incremented. This technique helps in efficiently counting occurrences in a 2D structure.
Example 2: Finding the Minimum Element in a 2D Array
To find the smallest element in a 2D array, start by assuming the first element is the smallest. The outer loop goes over each row, and the inner loop compares each element in the row to the current minimum value. If a smaller value is found, the minimum is updated. This is useful for identifying the least value in a grid of data, such as in temperature maps or game boards.
Example 3: alculating the Average of All Elements
If you need to calculate the average of all the elements in a 2D array, nested loops can be used to sum all the values, just like in the sum example above. After calculating the total sum, divide it by the total number of elements, which is the product of the number of rows and the number of columns in the array. This method is practical in calculating average values in data-heavy applications like image processing or sensor data analysis.
Example 4: Transposing a 2D Array
Transposing a 2D array means swapping its rows and columns. To achieve this, you need two nested loops: the outer loop traverses the rows, and the inner loop writes the values of each row as a column in a new array. This is common in mathematical operations, especially in linear algebra. For example, a matrix matrix[row][col] would be transposed to transposed[col][row].
Example 5: Rotating a 2D Array by 90 Degrees
A slightly more complex example is rotating a 2D array clockwise by 90 degrees. To rotate, nested loops are used to traverse the original array from left to right and store the elements in a new array such that the first row becomes the last column, the second row becomes the second last column, and so on. This operation is frequently used in game development or graphical applications, where rotations are needed for elements like game boards or image grids.
Multiple Choice Questions
Question 1
What will be the output of the following code?
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
for (int row = 0; row < matrix.length; row++) {
for (int col = 0; col < matrix[row].length; col++) {
System.out.print(matrix[row][col] + " ");
}
}
A) 1 2 3
B) 1 2 3 4 5 6 7 8 9
C) 123456789
D) 1 2 3 \n 4 5 6 \n 7 8 9
Answer: B) 1 2 3 4 5 6 7 8 9
Explanation: The code uses two nested loops to traverse the 2D array matrix. The outer loop iterates through the rows, and the inner loop iterates through the columns of each row. For each element matrix[row][col], the value is printed with a space in between. Since there is no newline (println) after the inner loop, all elements are printed in a single line, resulting in 1 2 3 4 5 6 7 8 9.
Question 2
Which of the following correctly describes the role of the inner loop when traversing a 2D array?
A) It iterates over the rows of the 2D array.
B) It accesses each element in a particular column.
C) It iterates over the columns of the current row.
D) It ensures each element is printed on a new line.
Answer: C) It iterates over the columns of the current row.
Explanation: The outer loop in a nested iteration structure iterates over the rows of the 2D array. The inner loop accesses each element of the current row by iterating over the columns in that row. So, the correct description of the inner loop is that it processes all the elements within a single row by iterating through each column of that row.
Question 3
What will happen if you use the following loop to traverse a 2D array matrix with 3 rows and 2 columns?
for (int row = 0; row <= matrix.length; row++) {
for (int col = 0; col < matrix[row].length; col++) {
System.out.print(matrix[row][col] + " ");
}
}
A) It will print the elements correctly.
B) It will throw an ArrayIndexOutOfBoundsException.
C) It will skip the last row and only print the first two rows.
D) It will print the elements in reverse order.
Answer: B) It will throw an ArrayIndexOutOfBoundsException.
Explanation: In the outer loop, the condition is row <= matrix.length, which is incorrect. The valid row indices for the 2D array are 0
to matrix.length – 1. However, row <= matrix.length allows the loop to iterate beyond the last valid index (i.e., it tries to access matrix[3] when the valid indices are 0, 1, and 2). This will result in an ArrayIndexOutOfBoundsException because there is no row at index 3. The correct condition should be row < matrix.length.