Traversing an array by accessing its elements using iteration statements is a fundamental concept in AP Computer Science A. It involves systematically visiting each element in the array to perform operations like displaying, modifying, or processing data. This can be achieved using various iteration methods, such as for loops, enhanced for loops, and while loops. Understanding how to efficiently traverse arrays is essential for solving problems related to data manipulation and processing, making it a key skill for success in the AP Computer Science A Exam.
Learning Objectives
In learning how to traverse an array using iteration statements for the AP Computer Science A exam, you should focus on understanding how to access and process each element of an array using for, while, and enhanced for-each loops. Key skills include managing loop counters, avoiding out-of-bounds errors, and applying different loops for tasks like summing values, searching for specific elements, or modifying data. You should also practice solving problems that involve nested loops and array manipulation to reinforce these concepts.
Traversing an Array by Accessing the Elements Using Iteration Statements
In AP Computer Science A, traversing an array involves systematically accessing each element in the array to perform operations such as displaying, modifying, or processing the data. To do this, iteration statements like for loops and while loops are commonly used.
For Loop Iteration
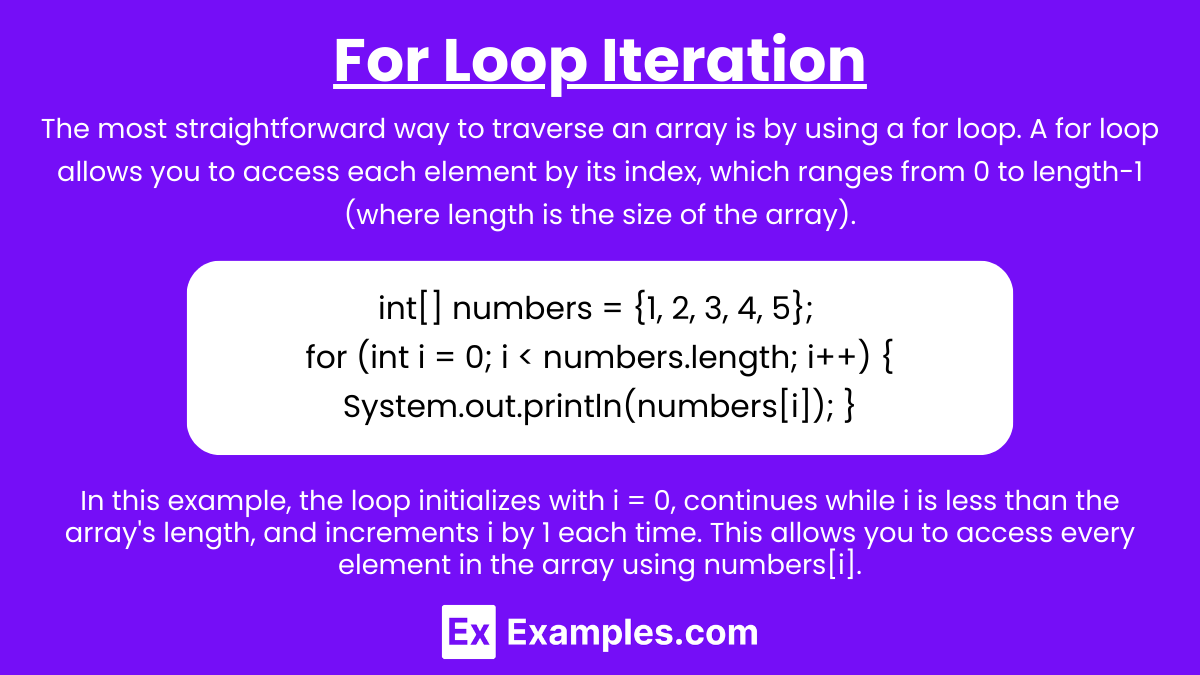
The most straightforward way to traverse an array is by using a for loop. A for loop allows you to access each element by its index, which ranges from 0 to length-1 (where length is the size of the array).
Example of traversing an array: In this example, the loop initializes with i = 0, continues while i is less than the array’s length, and increments i by 1 each time. This allows you to access every element in the array using numbers[i].
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
Enhanced For Loop (For-Each Loop)
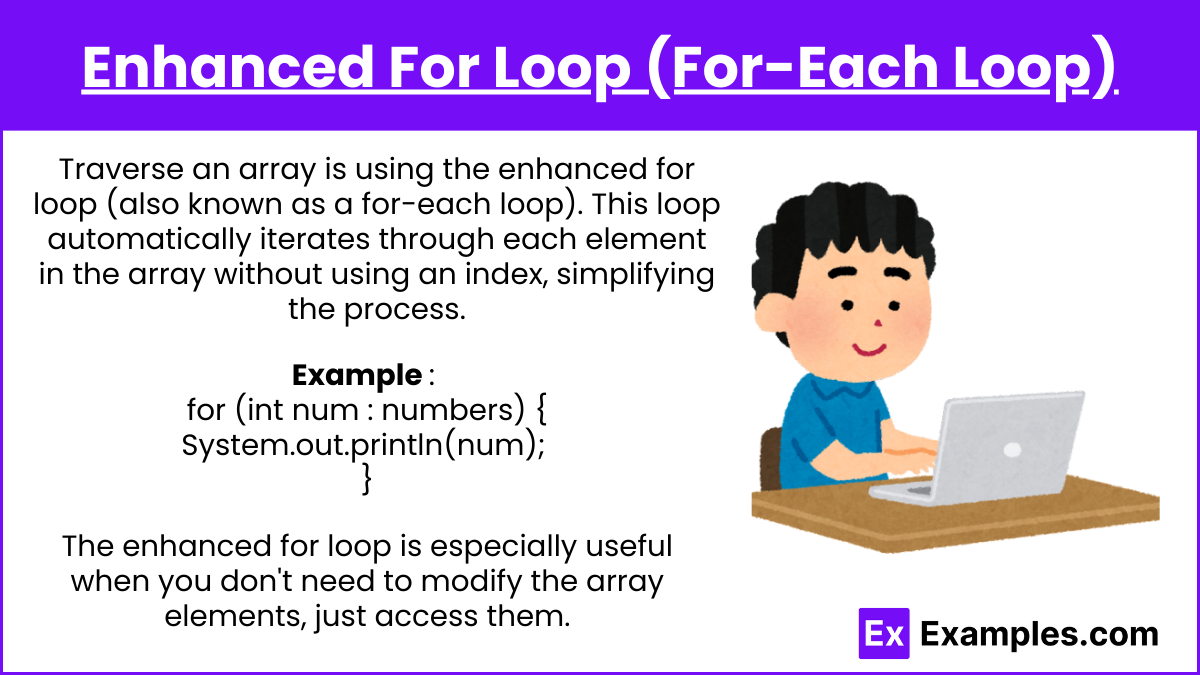
Another way to traverse an array is using the enhanced for loop (also known as a for-each loop). This loop automatically iterates through each element in the array without using an index, simplifying the process.
Example:
for (int num : numbers) {
System.out.println(num);
}
In this case, num represents each element in the numbers array during each iteration. The enhanced for loop is especially useful when you don’t need to modify the array elements, just access them.
While Loop Iteration
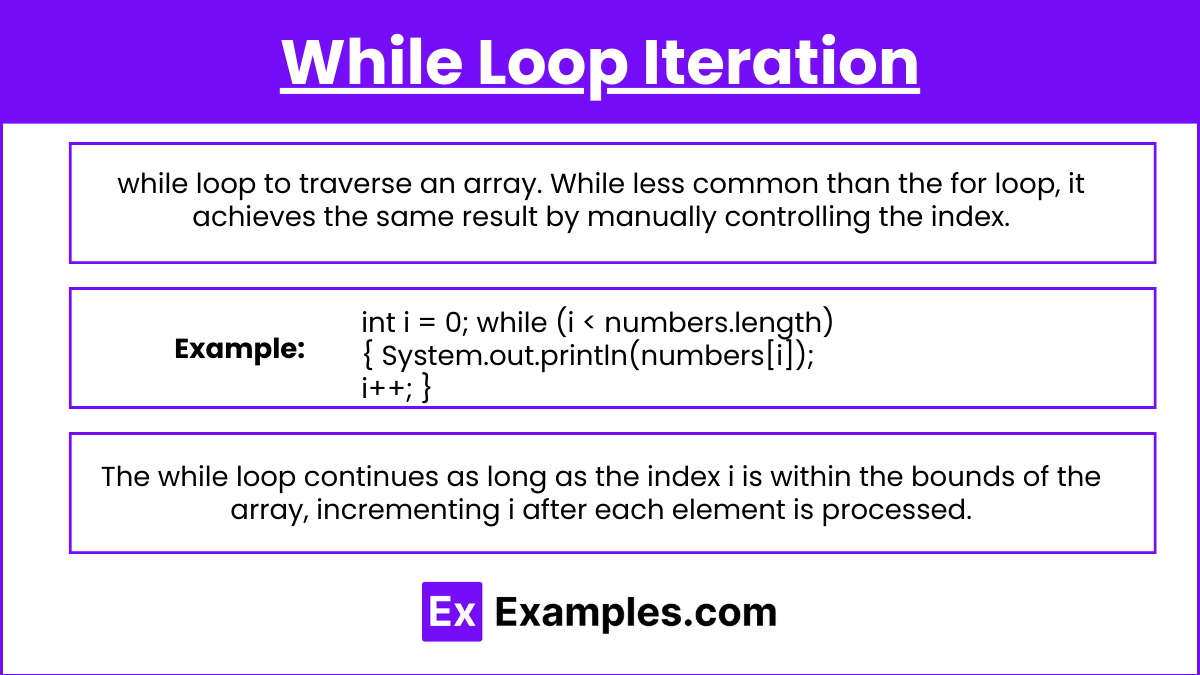
You can also use a while loop to traverse an array. While less common than the for loop, it achieves the same result by manually controlling the index.
Example:
int i = 0;
while (i < numbers.length) {
System.out.println(numbers[i]);
i++;
}
The while loop continues as long as the index i is within the bounds of the array, incrementing i after each element is processed.
Key Considerations for Iteration
- Array Length: Always ensure that the loop’s termination condition checks the correct array length (array.length). Accessing an index outside the array’s bounds results in an ArrayIndexOutOfBoundsException.
- Modifying Elements: If you’re modifying elements during traversal, use the standard for loop where you can reference the index to update specific elements.
- Nested Iteration: In some cases, arrays may contain other arrays (e.g., 2D arrays), where nested loops are necessary to access each element.
Examples
Example 1: Printing All Elements of an Integer Array Using a Standard For Loop
This is the most common way to traverse an array. For example, if you have an array of integers and want to print each element, you can use a for loop that starts from index 0
and continues until the length of the array. In each iteration, you access the element at the current index and print it.
int[] numbers = {10, 20, 30, 40, 50};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
Example 2: Summing All Elements of a Double Array Using an Enhanced For Loop
The enhanced for loop (also known as a for-each loop) allows you to traverse an array without explicitly using the index. This is useful when you don’t need to know the position of elements but want to process them, like summing the values of all elements in a double array.
double[] prices = {19.99, 9.99, 14.99, 29.99};
double total = 0;
for (double price : prices) {
total += price;
}
System.out.println("Total: " + total);
Example 3: Counting Occurrences of a Specific Element Using a While Loop
A while loop can also be used to traverse an array. In this example, the loop counts how many times a specific value appears in an array. You start with an index at 0 and increment it as long as it’s within the array’s bounds.
int[] scores = {85, 92, 75, 85, 88, 92, 85};
int target = 85;
int count = 0;
int i = 0;
while (i < scores.length) {
if (scores[i] == target) {
count++;
}
i++;
}
System.out.println("Occurrences of " + target + ": " + count);
Example 4: Reversing the Elements of a String Array Using a For Loop
You can traverse an array from the last element to the first by using a for loop that starts from the highest index (length-1) and decrements. This can be useful for reversing the order of elements in the array.
String[] words = {"apple", "banana", "cherry", "date"};
for (int i = words.length - 1; i >= 0; i--) {
System.out.println(words[i]);
}
Example 5: Modifying Array Elements Using Index-Based Access in a For Loop
Sometimes you need to traverse an array to modify its elements. For example, you can multiply every element in an integer array by 2 using a for loop that accesses each element by index, modifies it, and updates the value in the array.
int[] values = {2, 4, 6, 8, 10};
for (int i = 0; i < values.length; i++) {
values[i] = values[i] * 2;
}
for (int value : values) {
System.out.println(value);
}
Multiple Choice Questions
Question 1
What will the following code output?
int[] numbers = {2, 4, 6, 8, 10};
for (int i = 0; i < numbers.length; i++) {
System.out.print(numbers[i] + " ");
}
A) 2 4 6 8 10
B) 2 4 6 8
C) 10 8 6 4 2
D) 0 1 2 3 4
Answer: A) 2 4 6 8 10
Explanation: The code uses a standard for loop to traverse the numbers array from index 0 to numbers.length – 1 (which is 4
for this array). At each iteration, it prints the element at the current index followed by a space. Since the array numbers contains the elements {2, 4, 6, 8, 10}, the loop will print each element in order, resulting in the output: “2 4 6 8 10”. Option B is incorrect because it omits the last element, and option C is incorrect because the loop traverses from the first to the last element (not in reverse). Option D is completely unrelated to the content of the array.
Question 2
Which loop is most appropriate for traversing an array if you do not need to modify the array elements and do not need to know their index positions?
A) Standard for loop
B) while loop
C) Enhanced for loop (for-each)
D) Do-while loop
Answer: C) Enhanced for loop (for-each)
Explanation: The enhanced for loop, also known as the for-each loop, is ideal when you need to access each element of the array without modifying them or needing their index position. This loop iterates through each element of the array directly, simplifying code. The standard for loop (A) and while loop (B) are better when you need to access or modify elements using their index. The do-while loop (D) is rarely used for array traversal and is generally less efficient in this context.
Question 3
What will the following code output if the target element 3
is not found in the array?
int[] values = {1, 2, 4, 5};
int target = 3;
boolean found = false;
for (int i = 0; i < values.length; i++) {
if (values[i] == target) {
found = true;
break;
}
}
if (found) {
System.out.println("Element found");
} else {
System.out.println("Element not found");
}
A) Element found
B) Element not found
C) 3
D) Index out of bounds error
Answer: B) Element not found
Explanation: The code iterates through the values array searching for the target value 3
. Since the target value is not present in the array {1, 2, 4, 5}, the if condition inside the loop will never be true, and the variable found will remain false. After the loop completes, the code checks the found variable and prints “Element not found” because found was not updated to true. Option A is incorrect because the target value was not found, and option C is irrelevant. Option D is incorrect because the loop is correctly bounded within the array length, preventing an index out-of-bounds error.