In AP Computer Science A, traversing an ArrayList involves accessing each element to perform operations or retrieve data. This is typically done using iteration statements such as traditional for loops, enhanced for-each loops, or the Iterator interface. These methods allow efficient element access in sequential order, either by index or through direct element references. Mastering different traversal techniques is crucial for problem-solving, manipulating data, and writing efficient programs, as ArrayList structures are frequently used in AP exam questions and coding challenges.
Learning Objectives
When studying “Traversing an ArrayList by Accessing the Elements Using Iteration Statements” for AP Computer Science A, you should learn how to effectively iterate through an ArrayList using traditional for loops, enhanced for-each loops, and iterators. Focus on understanding when to use each type of loop, how to access or modify elements within the list, and how to avoid common errors like ConcurrentModificationException or IndexOutOfBoundsException. Master these techniques to write efficient, error-free code for processing collections of data.
Using a for Loop
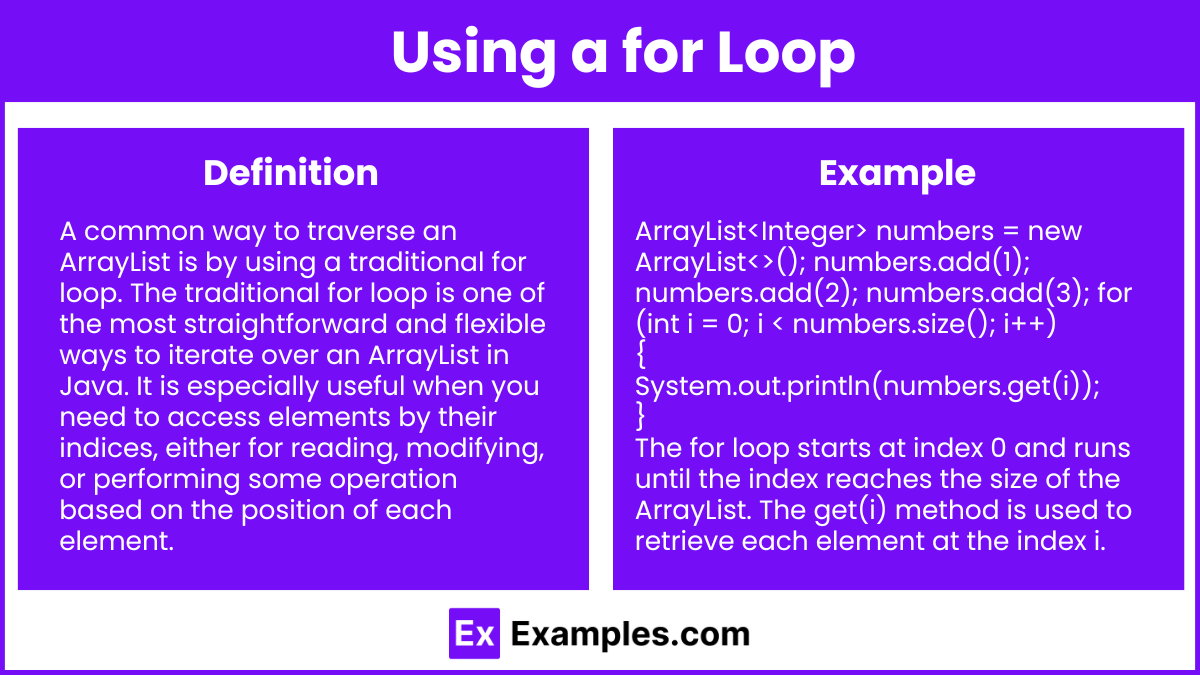
A common way to traverse an ArrayList is by using a traditional for loop. The traditional for loop is one of the most straightforward and flexible ways to iterate over an ArrayList in Java. It is especially useful when you need to access elements by their indices, either for reading, modifying, or performing some operation based on the position of each element.This allows you to access each element by its index, which can be useful if you need to modify or reference specific indices.
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
for (int i = 0; i < numbers.size(); i++) {
System.out.println(numbers.get(i));
}
- Explanation: The for loop starts at index 0 and runs until the index reaches the size of the ArrayList. The get(i) method is used to retrieve each element at the index i.
Enhanced for-each Loop
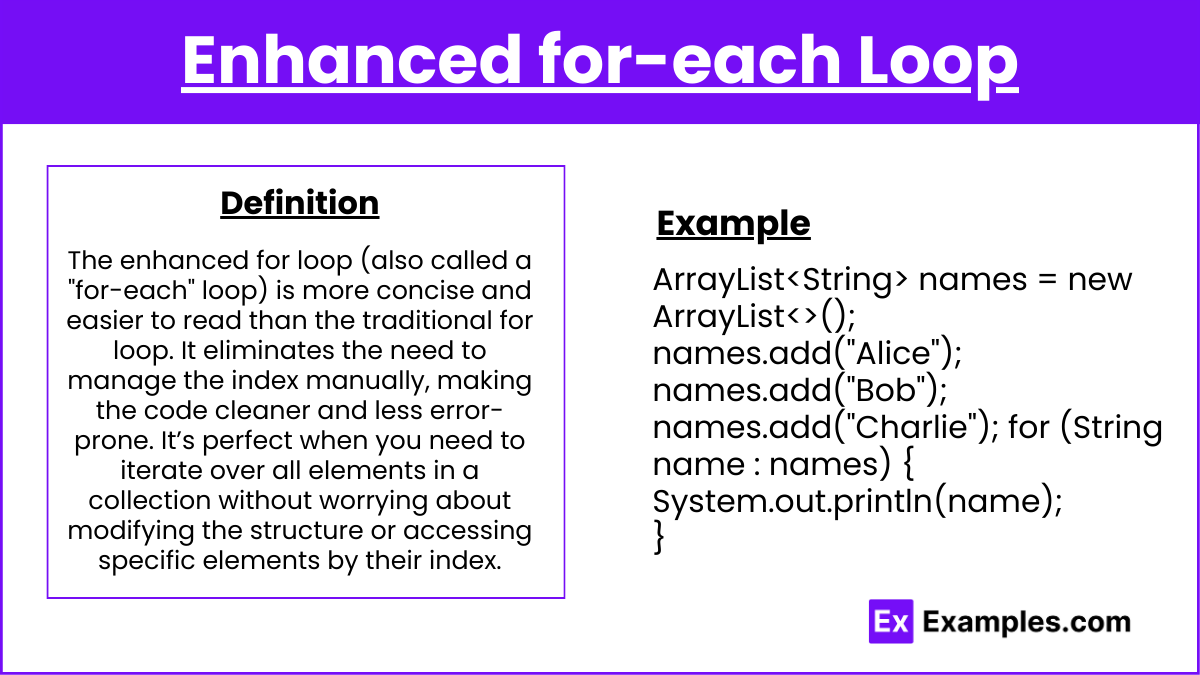
The enhanced for loop (also called a “for-each” loop) is more concise and easier to read than the traditional for loop. It eliminates the need to manage the index manually, making the code cleaner and less error-prone. It’s perfect when you need to iterate over all elements in a collection without worrying about modifying the structure or accessing specific elements by their index.It automatically iterates through all elements without using an index.
ArrayList<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
for (String name : names) {
System.out.println(name);
}
- Explanation: In this example, the for-each loop iterates through the ArrayList directly, and each element is temporarily assigned to the variable name. This method is ideal when you don’t need to modify the ArrayList or access specific indices.
Using an Iterator
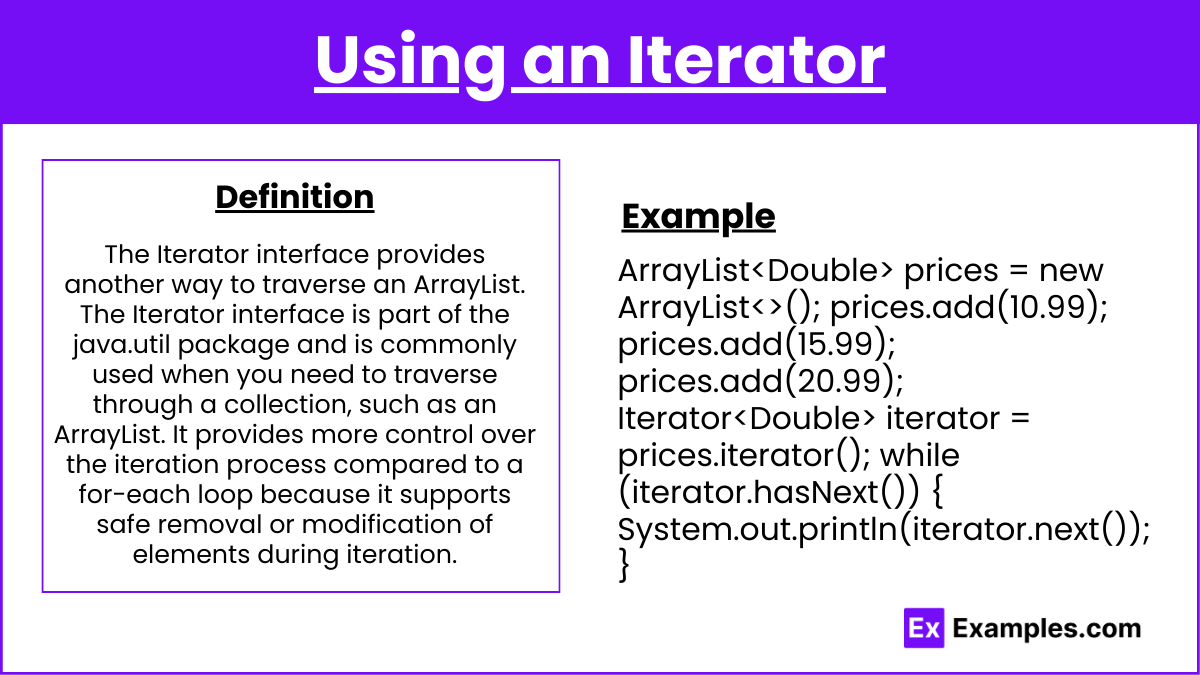
The Iterator interface provides another way to traverse an ArrayList. The Iterator interface is part of the java.util package and is commonly used when you need to traverse through a collection, such as an ArrayList. It provides more control over the iteration process compared to a for-each loop because it supports safe removal or modification of elements during iteration. It is useful when you need to modify the ArrayList while iterating through it (e.g., removing elements).
ArrayList<Double> prices = new ArrayList<>();
prices.add(10.99);
prices.add(15.99);
prices.add(20.99);
Iterator<Double> iterator = prices.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
- Explanation: The hasNext() method checks if there are more elements to iterate over, and next() retrieves the next element. This method is more flexible than the for-each loop because it allows for removal or modification of elements during iteration.
Using a forEach() Method
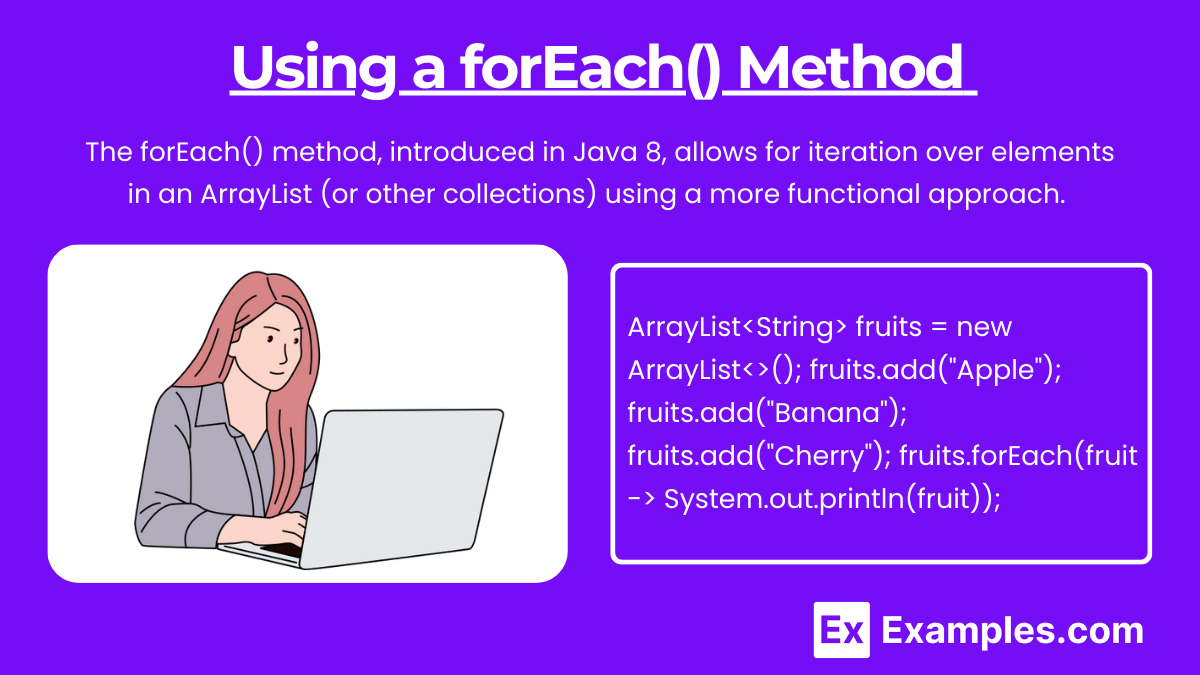
The forEach() method, introduced in Java 8, allows for iteration over elements in an ArrayList (or other collections) using a more functional approach. This method takes a Consumer functional interface as a parameter, which is typically represented as a lambda expression or method reference. The Consumer interface accepts a single input and performs an operation, typically without returning a value. This method is concise and functional in style.
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
fruits.forEach(fruit -> System.out.println(fruit));
- Explanation: The forEach() method takes a lambda expression that defines the action to perform on each element in the ArrayList. This method is concise and leverages functional programming paradigms.
Examples
Example 1: Using a Basic for Loop to Access and Modify Elements
In a scenario where you need to iterate through an ArrayList and modify certain elements, a traditional for loop provides access to each element’s index. For instance, an ArrayList of integers can be traversed to double the value of each element:
ArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4));
for (int i = 0; i < numbers.size(); i++) {
numbers.set(i, numbers.get(i) * 2);
}
Example 2: Using an Enhanced for-each Loop to Print Elements
The for-each loop simplifies accessing each element in an ArrayList without using an index. For example, if you have a list of strings representing fruit names, you can print each element
ArrayList<String> fruits = new ArrayList<>(Arrays.asList("Apple", "Banana", "Cherry"));
for (String fruit : fruits) {
System.out.println(fruit);
}
Example 3: Using an Iterator to Traverse and Remove Elements
Iterators provide a robust way to traverse ArrayList elements, especially when removing elements during iteration. For example, if you want to remove all elements less than 10 from an ArrayList of integers, the following code is ideal:
ArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(5, 10, 15, 20));
Iterator<Integer> iterator = numbers.iterator();
while (iterator.hasNext()) {
if (iterator.next() < 10) {
iterator.remove();
}
}
Example 4: Using the forEach() Method with a Lambda Expression
Java 8 introduced the forEach() method, which allows you to traverse the list using a lambda expression. This functional style can be used to print each element of an ArrayList:
ArrayList<String> animals = new ArrayList<>(Arrays.asList("Cat", "Dog", "Elephant"));
animals.forEach(animal -> System.out.println(animal));
Example 5: Using a for Loop to Calculate the Sum of All Elements
If you need to sum up all the elements in an ArrayList of numbers, a for loop allows you to accumulate the sum by accessing each element via its index:
ArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4));
int sum = 0;
for (int i = 0; i < numbers.size(); i++) {
sum += numbers.get(i);
}
System.out.println("Sum: " + sum);
Each example demonstrates a practical way to traverse an ArrayList using different iteration techniques, making your code adaptable to various situations.
Multiple Choice Questions
Question 1
Which of the following methods would you use to iterate through all elements of an ArrayList in Java?
A) for (int i = 0; i < arrayList.size(); i++)
B) for (int element : arrayList)
C) while (iterator.hasNext())
D) All of the above
Answer: D) All of the above
Explanation:
- Option A: A traditional for loop can be used to iterate through an ArrayList using an index, from 0 to the size of the list minus one.
- Option B: An enhanced for loop (or “for-each” loop) can also be used to iterate through each element of the ArrayList.
- Option C: A while loop combined with an iterator can traverse the ArrayList, checking if the next element exists (iterator.hasNext()). Thus, all of the methods listed are valid for traversing an ArrayList, making D the correct answer.
Question 2
What will be the output of the following code?
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
for (String fruit : fruits) {
System.out.print(fruit + " ");
}
A) Apple Banana Orange
B) Orange Banana Apple
C) Apple Orange Banana
D) Banana Orange Apple
Answer: A) Apple Banana Orange
Explanation: The enhanced for loop (for (String fruit : fruits)) iterates over the ArrayList in the order the elements were added. Since “Apple”, “Banana”, and “Orange” were added in this order, the loop will print them in the same sequence. Therefore, the output will be “Apple Banana Orange”.
Question 3
Consider the following code snippet:
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
for (int i = 0; i < numbers.size(); i++) {
if (numbers.get(i) % 2 == 0) {
numbers.remove(i);
}
}
What will be the contents of the numbers ArrayList after the loop execution?
A) [1, 2, 3]
B) [1, 3]
C) [2, 3]
D) [1, 3]
Answer: D) [1, 3]
Explanation: When traversing an ArrayList and removing elements inside a for loop, the loop’s index management becomes tricky. In this code, when the element 2 is found (at index 1), it is removed. After removal, the elements shift left, meaning the element 3 moves to index 1. However, the loop increments the index, skipping the new element at index 1 (3), and the loop terminates. Thus, only 1 and 3 remain in the ArrayList. Therefore, the correct answer is D) [1, 3].