In AP Computer Science A, understanding Application Programming Interfaces (APIs) and libraries is essential. APIs define how software components interact, serving as a blueprint for building applications. Libraries, collections of precompiled routines, streamline programming tasks by providing reusable code. By mastering these tools, students can efficiently create robust applications. Key Java libraries include java.util and java.math, which offer functionalities like data structures and mathematical operations, pivotal for excelling in the exam and future software development.
Learning Objectives
For the AP Computer Science A exam, you should focus on learning how to effectively utilize Java APIs and libraries. This includes understanding how to import and use library classes and methods, creating and manipulating objects using these libraries, and applying static methods appropriately. It’s essential to become proficient in reading and interpreting API documentation to leverage existing libraries for solving complex problems. Additionally, practice coding with common libraries, such as those for collections and utilities, to build a strong practical understanding of their applications in real-world programming scenarios.
Definitions of API and Libraries
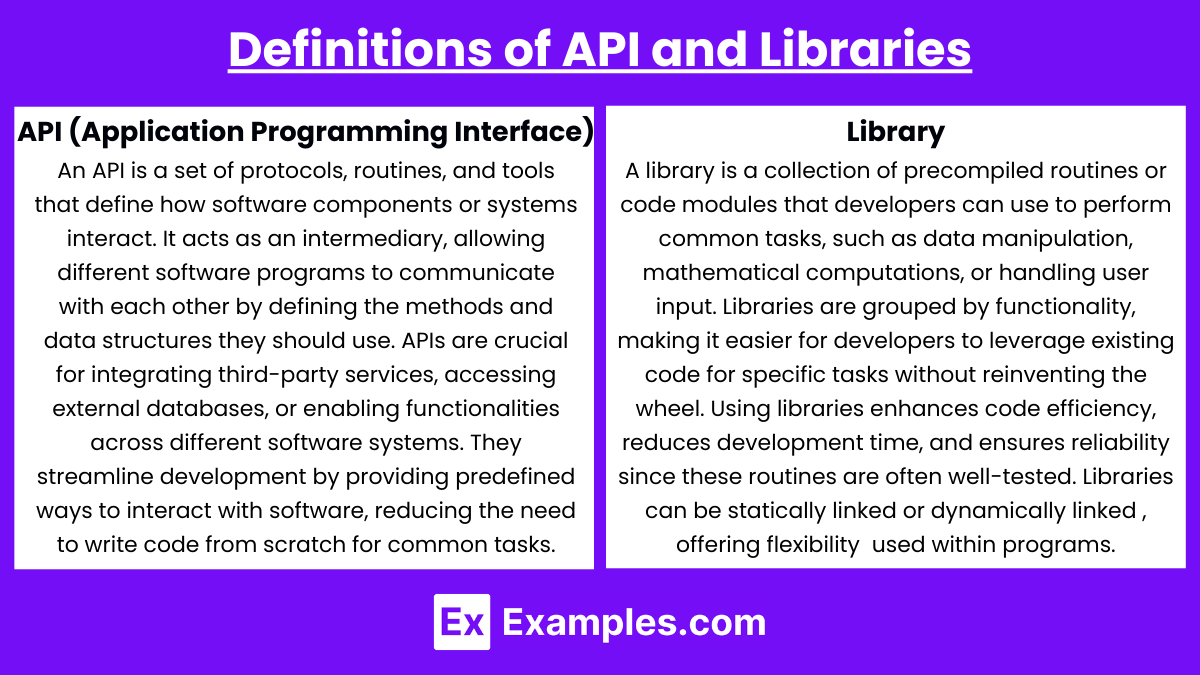
API (Application Programming Interface): An API is a set of protocols, routines, and tools that define how software components or systems interact. It acts as an intermediary, allowing different software programs to communicate with each other by defining the methods and data structures they should use. APIs are crucial for integrating third-party services, accessing external databases, or enabling functionalities across different software systems. They streamline development by providing predefined ways to interact with software, reducing the need to write code from scratch for common tasks.
Library: A library is a collection of precompiled routines or code modules that developers can use to perform common tasks, such as data manipulation, mathematical computations, or handling user input. Libraries are grouped by functionality, making it easier for developers to leverage existing code for specific tasks without reinventing the wheel. Using libraries enhances code efficiency, reduces development time, and ensures reliability since these routines are often well-tested. Libraries can be statically linked (included at compile-time) or dynamically linked (loaded at runtime), offering flexibility in how they are used within programs.
Using APIs and Libraries in Java
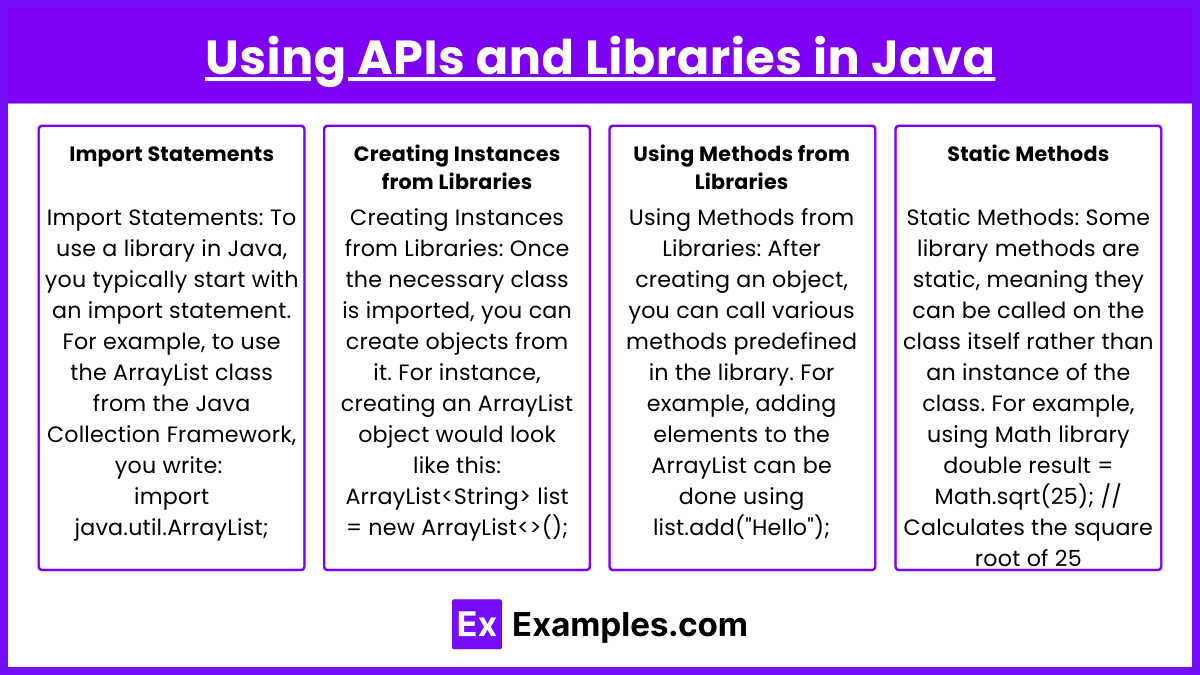
In AP Computer Science A, the focus is primarily on Java libraries. Key points include:
Import Statements: To use a library in Java, you typically start with an import statement. For example, to use the ArrayList class from the Java Collection Framework, you write:
import java.util.ArrayList;
Creating Instances from Libraries: Once the necessary class is imported, you can create objects from it. For instance, creating an ArrayList object would look like this:
ArrayList<String> list = new ArrayList<>();
Using Methods from Libraries: After creating an object, you can call various methods predefined in the library. For example, adding elements to the ArrayList can be done using:
list.add("Hello");
Static Methods: Some library methods are static, meaning they can be called on the class itself rather than an instance of the class. For example, using Math library:
double result = Math.sqrt(25); // Calculates the square root of 25
Why APIs and Libraries are Important
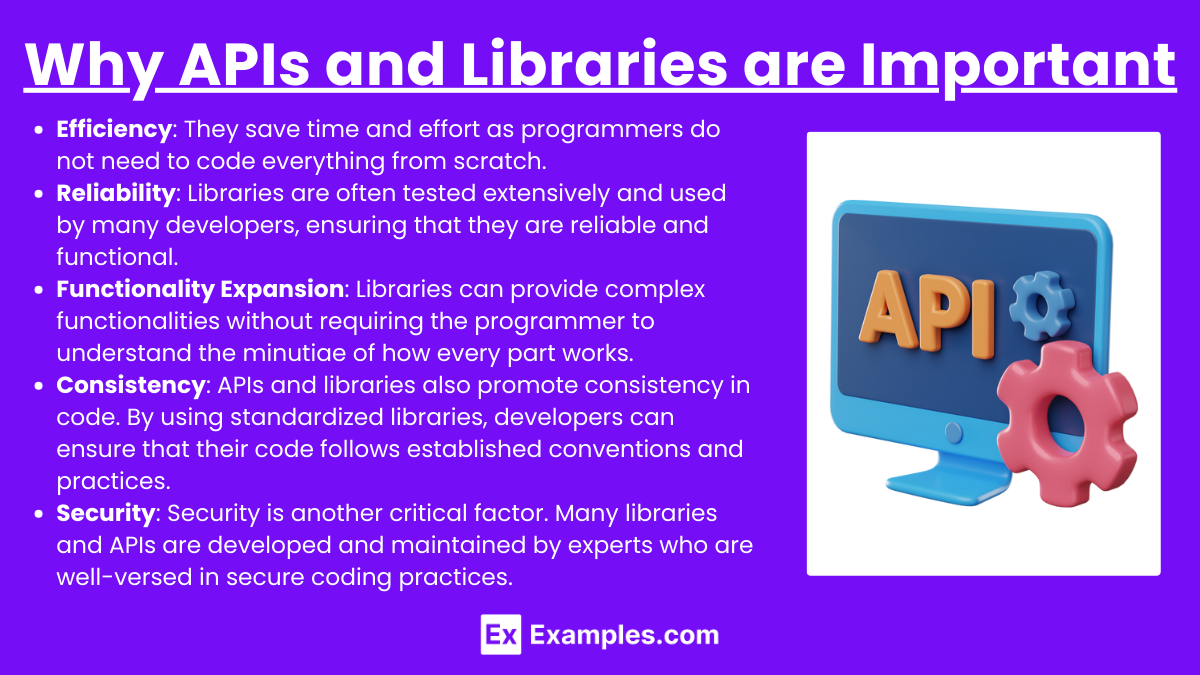
- Efficiency: They save time and effort as programmers do not need to code everything from scratch.
- Reliability: Libraries are often tested extensively and used by many developers, ensuring that they are reliable and functional.
- Functionality Expansion: Libraries can provide complex functionalities without requiring the programmer to understand the minutiae of how every part works.
- Consistency: APIs and libraries also promote consistency in code. By using standardized libraries, developers can ensure that their code follows established conventions and practices. This consistency makes the code easier to understand, maintain, and debug, especially when working in teams.
- Security: Security is another critical factor. Many libraries and APIs are developed and maintained by experts who are well-versed in secure coding practices. By using these tools, developers can enhance the security of their applications, relying on the robust security features provided by these libraries.
Examples
Example 1: Java Collections Framework
The Java Collections Framework provides a set of classes and interfaces for handling groups of objects. For instance, to manage a list of items dynamically, you can use the ‘ ArrayList ‘ class from the ‘ java.util package ‘. This is initiated by importing the class with import ‘ java.util.ArrayList; ‘ and then creating an instance of ‘ ArrayList ‘ to add, remove, and access elements efficiently. It’s a powerful example of how libraries simplify data management in applications.
Example 2: Java File Handling
The Java API includes robust support for file input and output through its java.io package. For example, to read text from a file, you can use the ‘ FileReader ‘ and ‘ BufferedReader ‘ classes. By importing these classes and creating appropriate instances, developers can read lines of text from a file, demonstrating how APIs facilitate complex file operations without extensive coding.
Example 3: Java Networking API
Java provides extensive support for network operations through its java.net package. This includes classes like ‘ Socket ‘ and ‘ ServerSocket ‘ for implementing TCP network protocols. By using these classes, a programmer can create client-server applications to communicate over the network, showcasing the use of APIs in facilitating network communications.
Example 4: Java Swing for GUI Development
Java Swing, part of the Java Foundation Classes (JFC), provides a rich set of APIs for building graphical user interfaces (GUIs). Classes like ‘ JFrame ‘, ‘ JButton ‘, and ‘ JTextField ‘ allow developers to create windows, buttons, and text fields, respectively. Importing and utilizing these classes from the ‘ javax.swing ‘ package enables the creation of user-friendly interfaces with various interactive elements.
Example 5: Java Math Library
The ‘ java.math ‘ package includes classes such as ‘ BigInteger ‘ and ‘ BigDecimal ‘ for performing operations on large integers and decimal numbers with high precision, which are crucial for applications requiring accurate mathematical calculations. This is particularly useful in banking and financial software to ensure precision in calculations. By importing these classes, programmers can handle large-scale arithmetic operations more efficiently than using primitive data types.
Multiple Choice Questions
Question 1
What is the purpose of the ‘ import ‘ statement in Java?
A) To load classes from Java’s API and libraries
B) To declare methods within a class
C) To increase the speed of program compilation
D) To document code
Correct Answer: A) To load classes from Java’s API and libraries
Explanation: The import statement in Java is used to bring in specific classes and entire packages from Java’s extensive set of libraries, allowing developers to use pre-built classes and methods in their programs. This eliminates the need for writing all code from scratch and leverages powerful, pre-tested functionality provided by Java. Options B, C, and D are incorrect as they describe other aspects unrelated to the functionality of the import statement.
Question 2
Which Java package is commonly used for reading and writing files?
A) ‘ java.util ‘
B) ‘ java.net ‘
C) ‘ java.io ‘
D) ‘ java.awt ‘
Correct Answer: C) java.io
Explanation: The ‘ java.io ‘ package in Java is specifically designed for input and output, including reading from and writing to files. It provides various classes such as ‘ FileReader ‘, ‘ FileWriter ‘, ‘ BufferedReader ‘, and ‘ BufferedWriter ‘ to handle file operations efficiently. Option A is for utility classes like collections, option B is for network operations, and option D is for abstract window toolkit (GUI components), making them incorrect in this context.
Question 3
Which of the following is NOT a valid reason to use libraries in Java programming?
A) To reduce code redundancy
B) To improve program performance
C) To avoid learning basic programming concepts
D) To access pre-built functionality
Correct Answer: C) To avoid learning basic programming concepts
Explanation: Libraries are used in programming to reduce code redundancy, access pre-built functionality, and potentially improve program performance by using optimized code. However, they are not intended to serve as a substitute for learning the fundamental concepts of programming. Using libraries without understanding the basics can lead to poor coding practices and dependency on specific libraries without comprehending underlying principles. Options A, B, and D are benefits of using libraries and thus incorrect choices for this question.