Assignment operators in Java are fundamental tools for modifying values stored in variables. They enable efficient updates to variable states, which is crucial for controlling program flow and managing data. These operators include the basic assignment (‘ = ‘), as well as compound forms like ‘ += ‘, ‘ -= ‘, ‘ *= ‘,’ /= ‘, and ‘ %= ‘ which combine arithmetic operations with assignment. Mastery of these operators is essential for writing concise and effective code, especially in contexts like loops, conditionals, and data manipulation tasks found in the AP Computer Science A exam.
Learning Objectives
When studying the use of assignment operators to produce a value for the AP Computer Science A exam, focus on understanding the syntax and application of each operator, including ‘ = ‘, ‘ += ‘, ‘ -= ‘, ‘ *= ‘, ‘ /= ‘, and ‘ %= ‘. Learn how to efficiently update variable values, manage state changes within loops, and handle conditional logic operations. Practice implementing these operators in coding problems to enhance computational thinking and problem-solving skills, ensuring you can apply these concepts effectively in both straightforward and complex programming scenarios.
Using Assignment Operators to Produce a Value
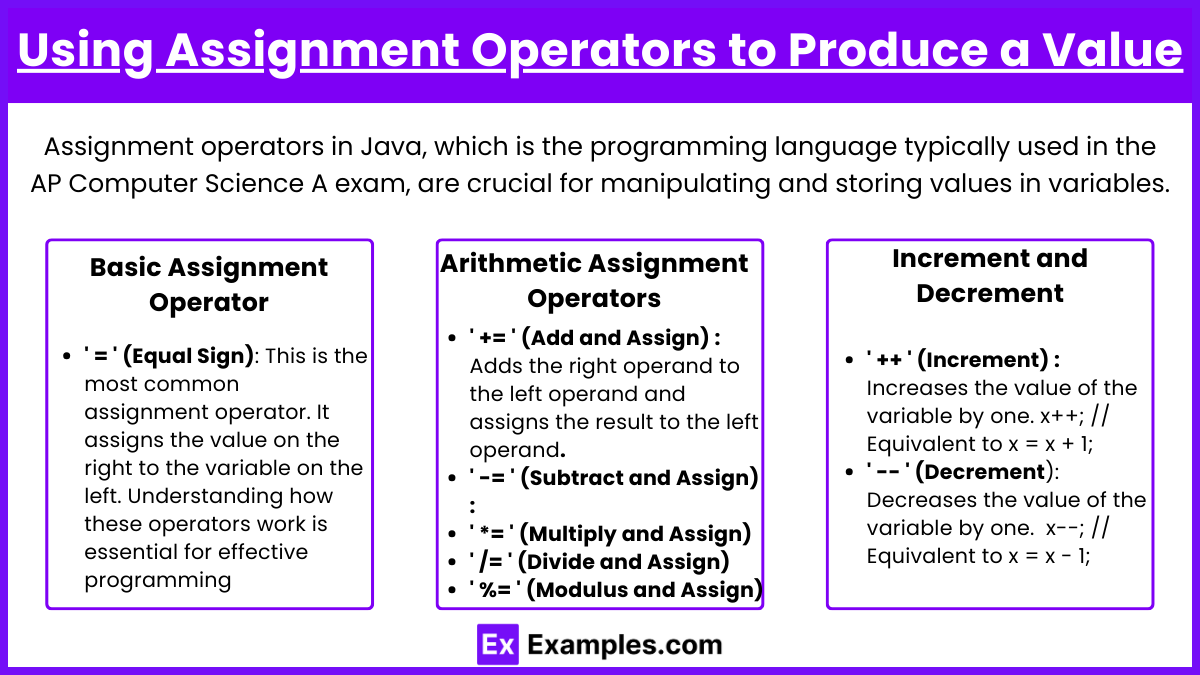
Assignment operators in Java, which is the programming language typically used in the AP Computer Science A exam, are crucial for manipulating and storing values in variables. Understanding how these operators work is essential for effective programming and problem-solving in the exam.
1. Basic Assignment Operator
‘ = ‘ (Equal Sign): This is the most common assignment operator. It assigns the value on the right to the variable on the left.
int x = 5; // Assigns 5 to variable x
2. Arithmetic Assignment Operators
These operators perform an arithmetic operation on the variable and then assign the result back to the variable.
‘ += ‘ (Add and Assign): Adds the right operand to the left operand and assigns the result to the left operand.
x += 3; // Equivalent to x = x + 3;
‘ -= ‘ (Subtract and Assign): Subtracts the right operand from the left operand and assigns the result to the left operand.
x -= 2; // Equivalent to x = x - 2;
‘ *= ‘ (Multiply and Assign): Multiplies the right operand with the left operand and assigns the result to the left operand.
x *= 4; // Equivalent to x = x * 4;
‘ /= ‘ (Divide and Assign): Divides the left operand by the right operand and assigns the result to the left operand.
ex /= 2; // Equivalent to x = x / 2;
‘ %= ‘ (Modulus and Assign): Computes the remainder of dividing the left operand by the right operand and assigns the result to the left operand.
x %= 3; // Equivalent to x = x % 3;
3. Increment and Decrement
These are unary operators that increase or decrease the value of a variable by one. They are particularly useful in loops and conditional operations.
‘ ++ ‘ (Increment): Increases the value of the variable by one.
x++; // Equivalent to x = x + 1;
‘ — ‘ (Decrement): Decreases the value of the variable by one.
x--; // Equivalent to x = x - 1;
Impact of Assignment Operators on Variable Scope and Lifetime
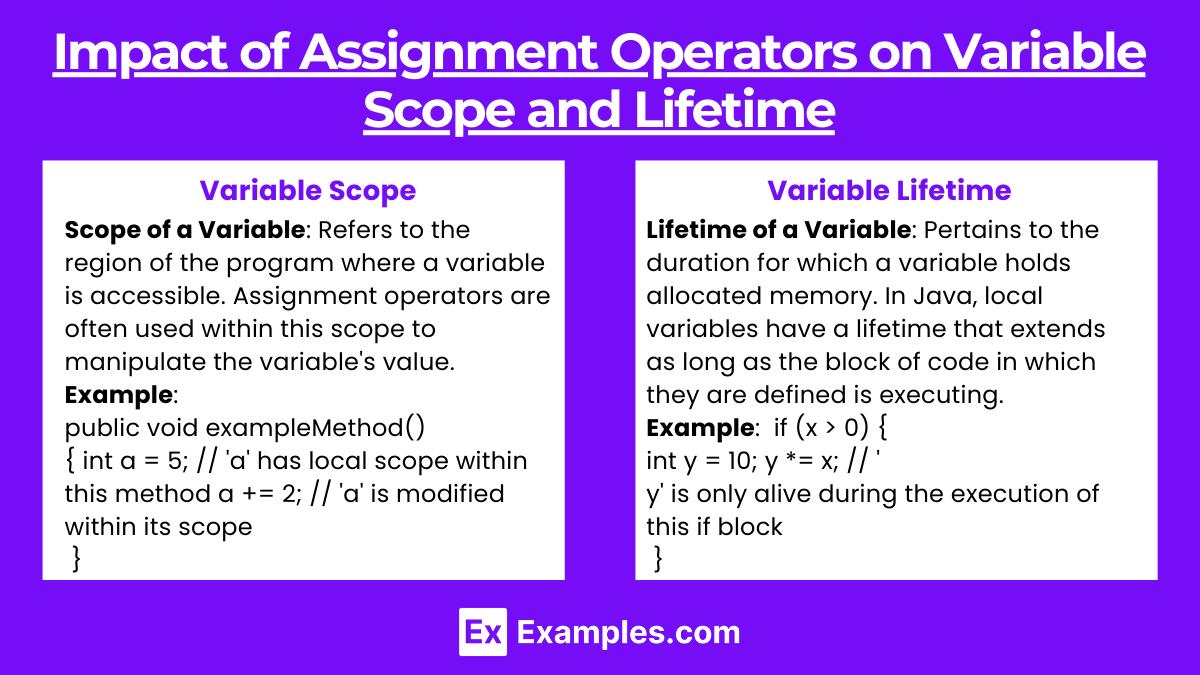
Assignment operators not only manage values within variables but also influence variable scope and lifetime—key concepts in Java that can significantly affect program behavior and memory management.
Variable Scope
Scope of a Variable: Refers to the region of the program where a variable is accessible. Assignment operators are often used within this scope to manipulate the variable’s value.
public void exampleMethod() {
int a = 5; // 'a' has local scope within this method
a += 2; // 'a' is modified within its scope
}
Here, ‘ a ‘ is only accessible within ‘ exampleMethod ‘, and any assignments or modifications to ‘ a ‘ are confined to this method.
Variable Lifetime
Lifetime of a Variable: Pertains to the duration for which a variable holds allocated memory. In Java, local variables have a lifetime that extends as long as the block of code in which they are defined is executing.
if (x > 0) {
int y = 10;
y *= x; // 'y' is only alive during the execution of this if block
}
Important Concepts to Understand
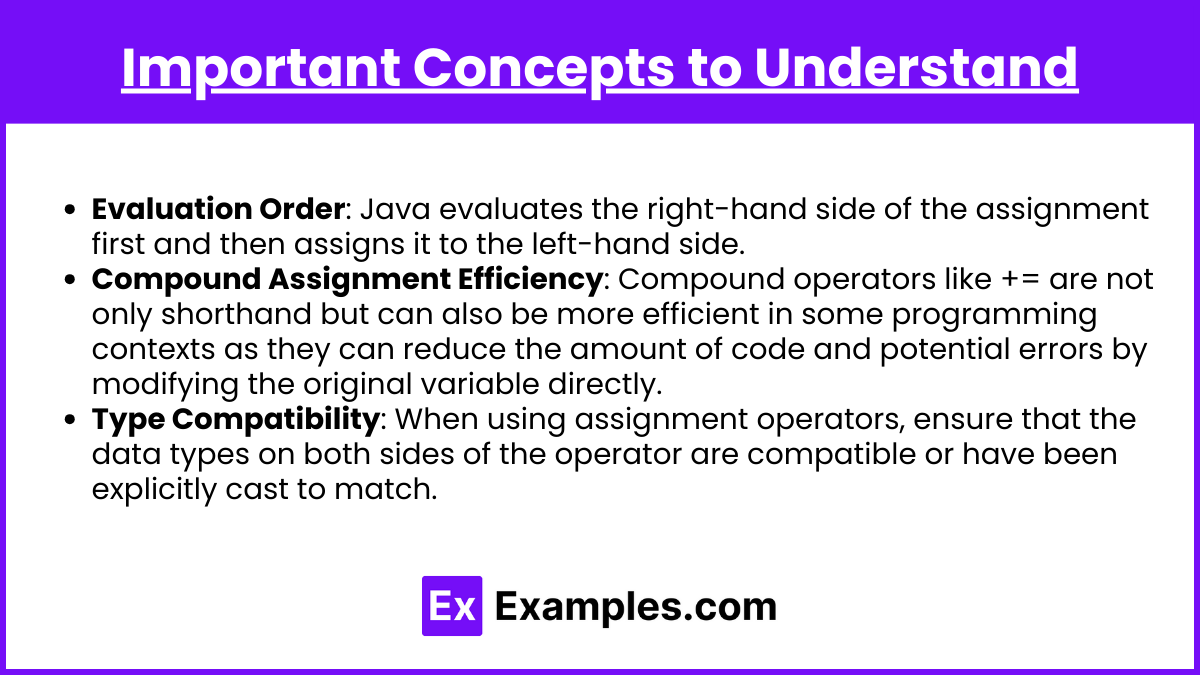
- Evaluation Order: Java evaluates the right-hand side of the assignment first and then assigns it to the left-hand side. This is crucial in understanding expressions like a = b = c = 5; where c is first assigned 5, then
b
is assigned the value of c, and finallya
is assigned the value of b. - Compound Assignment Efficiency: Compound operators like += are not only shorthand but can also be more efficient in some programming contexts as they can reduce the amount of code and potential errors by modifying the original variable directly.
- Type Compatibility: When using assignment operators, ensure that the data types on both sides of the operator are compatible or have been explicitly cast to match. For example, assigning a double to an int without casting will cause a compilation error.
Examples
Example 1: Incrementing a Counter
In this example, a counter variable ‘ count ‘ is incremented by 1 using the ‘ += ‘ operator. Initially set at 0, the ‘ count ‘ variable is updated to reflect each iteration in a loop or as an event count.
int count = 0;
count += 1; // Increment count by 1
Example 2: Calculating Total Cost
Here, a variable ‘ totalCost ‘ is used to accumulate costs from different items. As each item’s cost is computed or retrieved, it is added to ‘ totalCost ‘ using the ‘ += ‘ operator, making the code more readable and concise.
double totalCost = 0.0;
totalCost += 45.50; // Adding the cost of the first item
totalCost += 30.25; // Adding the cost of the second item
Example 3: Decrementing Stock Quantity
In inventory management, when an item is sold, the stock quantity needs to be decremented. The -= operator is used to subtract the sold quantity from the ‘ stockQuantity ‘ variable.
int stockQuantity = 50;
stockQuantity -= 5; // Decrementing by 5 units sold
Example 4: Applying Discount
To apply a discount percentage to a price, the ‘ *= ‘ operator can be used to adjust the original price by a discount factor. In this case, applying a 20% discount to ‘ originalPrice ‘ would be accomplished as follows:
double originalPrice = 100.0;
originalPrice *= 0.80; // Applying a 20% discount
Example 5: Normalizing a Value
When normalizing a value, such as scaling a score to be out of 100, the ‘ *= ‘ operator is handy. If ‘ score ‘ is out of 50, it can be normalized by multiplying by 2.
double score = 47;
score *= 2; // Normalizing to be out of 100
Multiple Choice Questions
Question 1
What is the value of ‘ x ‘ after the following code segment is executed?
int x = 10;
x += 5;
A) 5
B) 10
C) 15
D) 20
Correct Answer: C) 15
Explanation: The ‘ += ‘ operator adds the right-hand operand to the left-hand operand and assigns the result back to the left-hand operand. In this case, ‘ 5 ‘ is added to ‘ 10 ‘, and the result, ‘ 15 ‘, is stored back in ‘ x ‘.
Question 2
Consider the following code:
int a = 5;
int b = 10;
a *= b;
What is the final value of a?
A) 0
B) 5
C) 10
D) 50
Correct Answer: D) 50
Explanation: The ‘ *= ‘ operator multiplies the operand on the left by the operand on the right and assigns the result to the left operand. Here, ‘ a ‘ is multiplied by ‘ b ‘ (5 * 10), resulting in ‘ 50 ‘, which is then assigned back to ‘ a ‘.
Question 3
Which of the following statements correctly decreases the value of ‘ x ‘ by ‘ 2 ‘ and stores the new value in ‘ x ‘?
int x = 8;
A) ‘ x =- 2 ‘;
B) ‘ x -= 2 ‘;
C) ‘ x = x – 2 ‘;
D) Both B and C are correct.
Correct Answer: D) Both B and C are correct.
Explanation:
- Option B uses the ‘ -= ‘ operator, which subtracts the right-hand side value from the left-hand side value and assigns the result back to the left-hand side. ‘ x -= 2 ‘ is equivalent to ‘ x = x – 2 ‘.
- Option C directly uses the assignment operator ‘ = ‘ with the expression ‘ x – 2 ‘, achieving the same result.
- Option A is incorrect because ‘ x =- 2 ‘; would be parsed as ‘ x = -2 ‘, setting ‘ x ‘ to ‘ -2 ‘ rather than decreasing its current value by 2.
These questions cover basic to intermediate uses of assignment operators, helping to ensure that students understand both the syntax and the semantics of these operators.