In AP Computer Science A, using comments effectively is essential for describing the functionality of code. Comments are non-executable lines that provide clarity about the purpose, logic, and expected outcomes of specific sections of code. They help both the programmer and others who may read the code later to understand its structure and logic. Well-placed comments enhance code readability, make debugging easier, and serve as documentation for the program’s behavior, making complex sections more manageable and maintaining consistency throughout the project.
Learning Objectives
For the topic “Using Comments to Describe the Functionality of Code” in AP Computer Science A, you should focus on understanding how to write clear, concise comments that explain the purpose and logic of your code. Learn to describe complex sections, variables, methods, and their expected behaviors to improve code readability and maintainability. Additionally, practice using comments to document code functionality for collaboration, debugging, and testing, ensuring that others can easily understand and work with your code.
Purpose of Comments
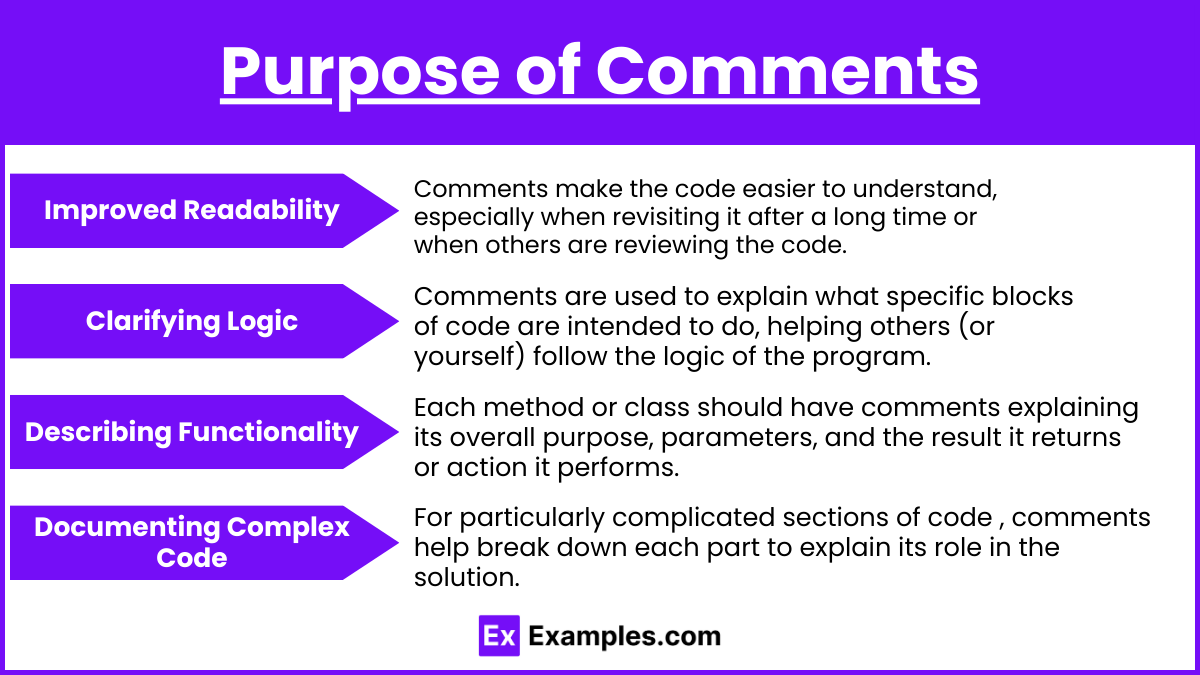
- Improved Readability: Comments make the code easier to understand, especially when revisiting it after a long time or when others are reviewing the code.
- Clarifying Logic: Comments are used to explain what specific blocks of code are intended to do, helping others (or yourself) follow the logic of the program.
- Describing Functionality: Each method or class should have comments explaining its overall purpose, parameters, and the result it returns or action it performs.
- Documenting Complex Code: For particularly complicated sections of code (such as nested loops, conditional statements, or recursive functions), comments help break down each part to explain its role in the solution.
Types of Comments
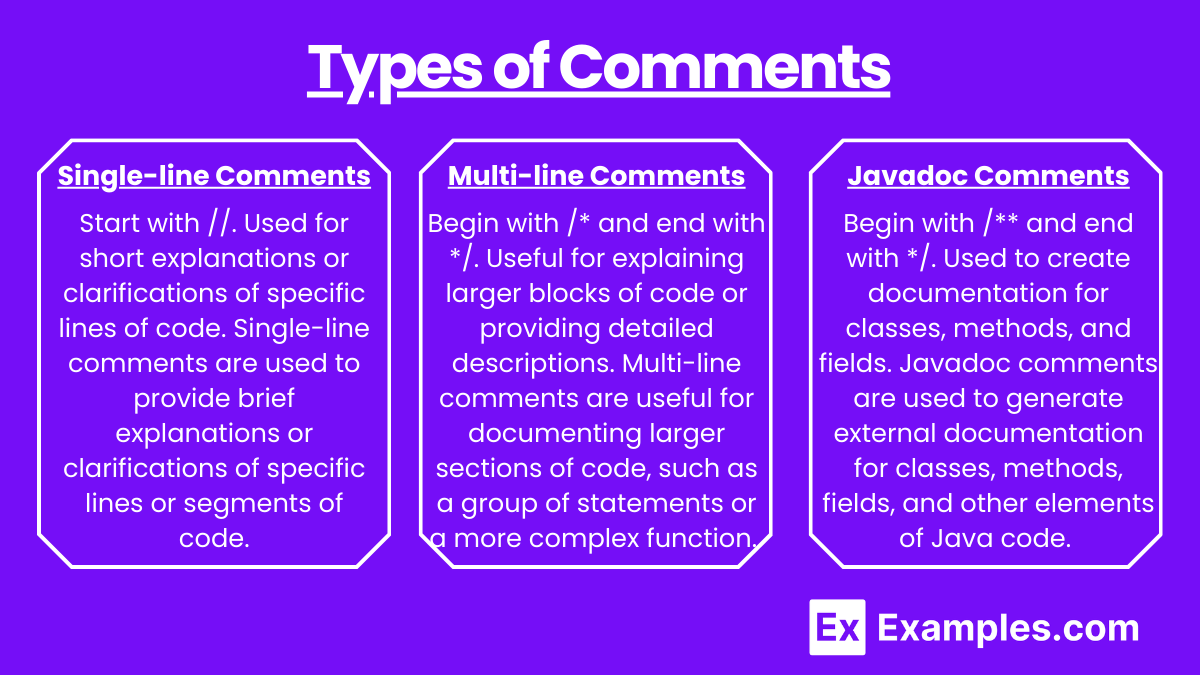
Single-line Comments: Start with //. Used for short explanations or clarifications of specific lines of code. Single-line comments are used to provide brief explanations or clarifications of specific lines or segments of code.
Example:
// This line calculates the area of a circle using the formula πr²
double area = Math.PI * radius * radius;
Multi-line Comments: Begin with /* and end with */. Useful for explaining larger blocks of code or providing detailed descriptions. Multi-line comments are useful for documenting larger sections of code, such as a group of statements or a more complex function. They can also be used to provide in-depth descriptions.
Example:
/*
* This method calculates the sum of the elements in an integer array.
* It takes an integer array as a parameter and returns the total sum of its elements.
* Each element in the array is added to the variable `sum` in a loop.
*/
public int sumArray(int[] array) {
int sum = 0;
for (int i = 0; i < array.length; i++) {
sum += array[i]; // Add each element's value to the running total
}
return sum;
}
Javadoc Comments: Begin with /** and end with */. Used to create documentation for classes, methods, and fields. Javadoc comments are used to generate external documentation for classes, methods, fields, and other elements of Java code. They are more formalized than single-line or multi-line comments and are often used in professional development to provide an API-style documentation for users of the code. Provide information about parameters (@param), return values (@return), and exceptions thrown (@throws).
Example :
/**
* Calculates the average of an array of numbers.
*
* @param numbers an array of integers to be averaged
* @return the average value of the numbers in the array
*/
public double calculateAverage(int[] numbers) {
int sum = 0;
for (int num : numbers) {
sum += num;
}
return (double) sum / numbers.length;
}
Best Practices for Using Comments
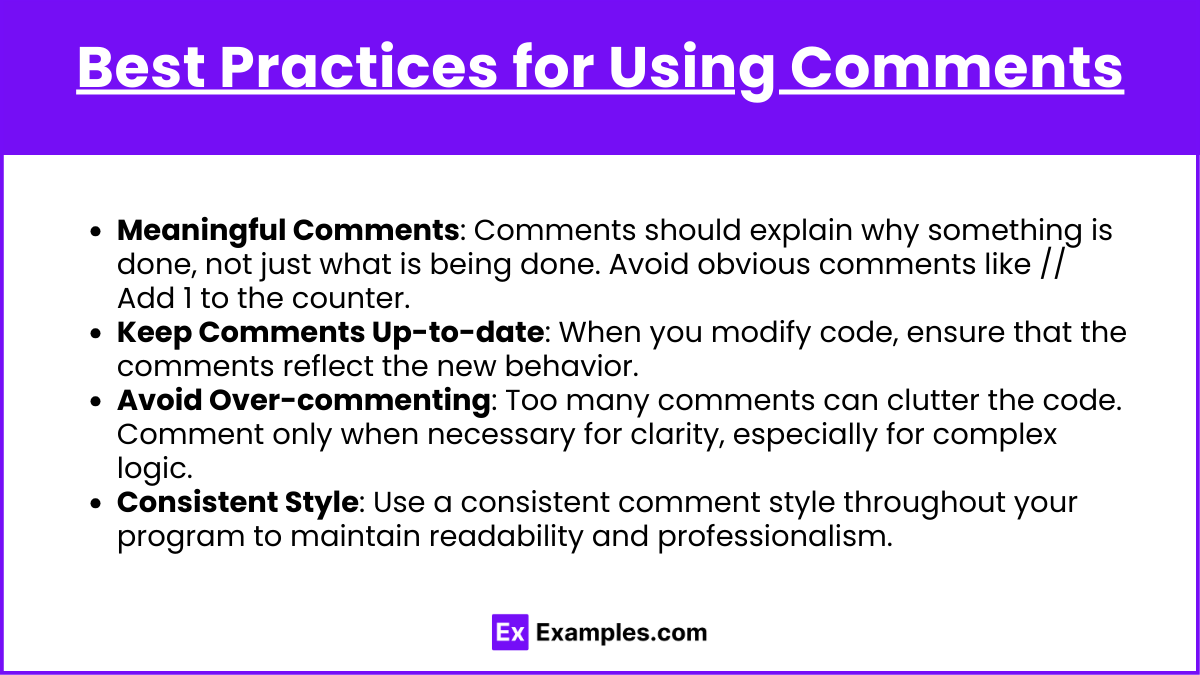
- Meaningful Comments: Comments should explain why something is done, not just what is being done. Avoid obvious comments like // Add 1 to the counter.
- Keep Comments Up-to-date: When you modify code, ensure that the comments reflect the new behavior.
- Avoid Over-commenting: Too many comments can clutter the code. Comment only when necessary for clarity, especially for complex logic.
- Consistent Style: Use a consistent comment style throughout your program to maintain readability and professionalism.
Examples
Example 1: Explaining Method Functionality
In a method that calculates the area of a rectangle, a comment at the beginning describes the purpose of the method and the parameters it takes. For instance:
// This method calculates the area of a rectangle.
// It takes two parameters: the length and width of the rectangle.
// The result is the product of length and width.
public double calculateArea(double length, double width) {
return length * width;
}
This kind of comment explains the purpose of the method and describes what the parameters represent, helping anyone reading the code understand the logic.
Example 2: Commenting on Loops and Conditions
In a loop that calculates the sum of even numbers, comments can be used to explain the logic behind the conditions inside the loop. For example:
// This loop iterates through the array.
// It checks if each number is even, and if it is, adds it to the sum.
int sum = 0;
for (int i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 == 0) {
sum += numbers[i]; // Add the even number to sum
}
}
The comments clarify the purpose of the loop and provide details on the condition inside, which helps in understanding the role of each line of code.
Example 3: Documenting Class-Level Comments
For complex classes, you can provide comments at the top to describe the class’s functionality. For instance:
// This class represents a Bank Account with basic operations.
// It includes methods to deposit, withdraw, and check the balance.
public class BankAccount {
private double balance;
// Constructor to initialize the account with a balance
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
}
A high-level comment like this introduces the class, summarizing its purpose and major functionality, which can be useful when reviewing the class as a whole.
Example 4 : Explaining Recursion in Code
Recursion can be tricky, and a comment explaining its purpose helps in understanding the flow. For example:
// This method calculates the factorial of a number using recursion.
// If the number is 0 or 1, it returns 1.
// Otherwise, it multiplies the number by the factorial of (number - 1).
public int factorial(int n) {
if (n <= 1) {
return 1; // Base case
} else {
return n * factorial(n - 1); // Recursive case
}
}
This comment helps the reader follow the recursive process and understand the base and recursive cases, which is vital for grasping how recursion works.
Example 5: Describing Complex Data Structures
When working with complex data structures like hash maps, comments can explain how they are used. For example:
// This method uses a HashMap to count the frequency of words in a sentence.
// The key is the word, and the value is the count of how many times it appears.
public Map<String, Integer> countWords(String sentence) {
Map<String, Integer> wordCount = new HashMap<>();
String[] words = sentence.split(" ");
for (String word : words) {
wordCount.put(word, wordCount.getOrDefault(word, 0) + 1); // Increment count
}
return wordCount;
}
This comment describes the functionality of the method and how the HashMap is utilized, making it easier for others to understand the purpose of each part of the code.
Multiple Choice Questions
Question 1
What is the primary purpose of adding comments to code?
A) To increase the size of the code for no reason
B) To help describe the functionality of the code and improve readability
C) To make the code harder to understand
D) To allow the code to run faster
Answer: B) To help describe the functionality of the code and improve readability
Explanation: The main purpose of comments in code is to explain what the code does, which makes it easier to understand. Comments are especially helpful for future reference or for other developers who may work on the code. Comments are not processed by the compiler, so they do not affect the execution speed or performance of the code.
Question 2
Which type of comment is most appropriate for documenting the overall functionality of a method in Java?
A) Single-line comments (//)
B) Multi-line comments (/* … */)
C) Javadoc comments (/** … */)
D) Inline comments
Answer: C) Javadoc comments (/** … */)
Explanation: Javadoc comments are specifically designed for documenting methods, classes, and fields in Java. They follow a particular syntax and can be processed to generate external documentation. Javadoc comments typically describe the purpose of the method, its parameters, return values, and any exceptions it might throw. Single-line or multi-line comments are not ideal for creating such detailed documentation.
Question 3
Which of the following is an example of a good comment practice?
A) Commenting every single line of code, including obvious operations
B) Writing comments only for complex sections of code
C) Writing comments that only describe what the code does, not why
D) Using comments to change the flow of program execution
Answer: B) Writing comments only for complex sections of code
Explanation: It is best practice to comment only when necessary, particularly for complex or non-obvious sections of code. Over-commenting, such as adding comments for every single line, can clutter the code and reduce readability. The best comments explain why something is being done rather than just stating what the code does, which can often be obvious from reading the code itself. Comments are not intended to change the flow of the program; they are for documentation purposes only.