In AP Computer Science A, using common attributes and behaviors to group existing objects into superclasses is a key aspect of object-oriented programming (OOP). A superclass, also known as a parent class, serves as a blueprint that defines shared characteristics among related subclasses. This practice enhances code reusability, reduces redundancy, and promotes maintainability by allowing subclasses to inherit common fields and methods. Superclasses also enable polymorphism, where objects from different subclasses can be treated as instances of the superclass, improving code flexibility and scalability.
Learning Objectives
For the topic “Using Common Attributes and Behaviors to Group Existing Objects into Superclasses” in AP Computer Science A, you should focus on understanding how to define and implement superclasses to group shared attributes and methods among related subclasses. You will learn how inheritance promotes code reuse, how to override superclass methods in subclasses, and how polymorphism allows treating subclass objects as superclass types. Additionally, mastering abstract classes and methods will help in creating flexible designs that are extendable and maintainable across multiple related classes.
Using Common Attributes and Behaviors to Group Existing Objects into Superclasses
In object-oriented programming (OOP), a superclass, also known as a parent class or base class, serves as a general blueprint for related classes (called subclasses). Superclasses group shared attributes (fields) and behaviors (methods) that can be inherited by subclasses. This process is part of a concept called inheritance, which helps to reduce code duplication and make the code more modular.
Key Concepts
Attributes (Fields) in a Superclass
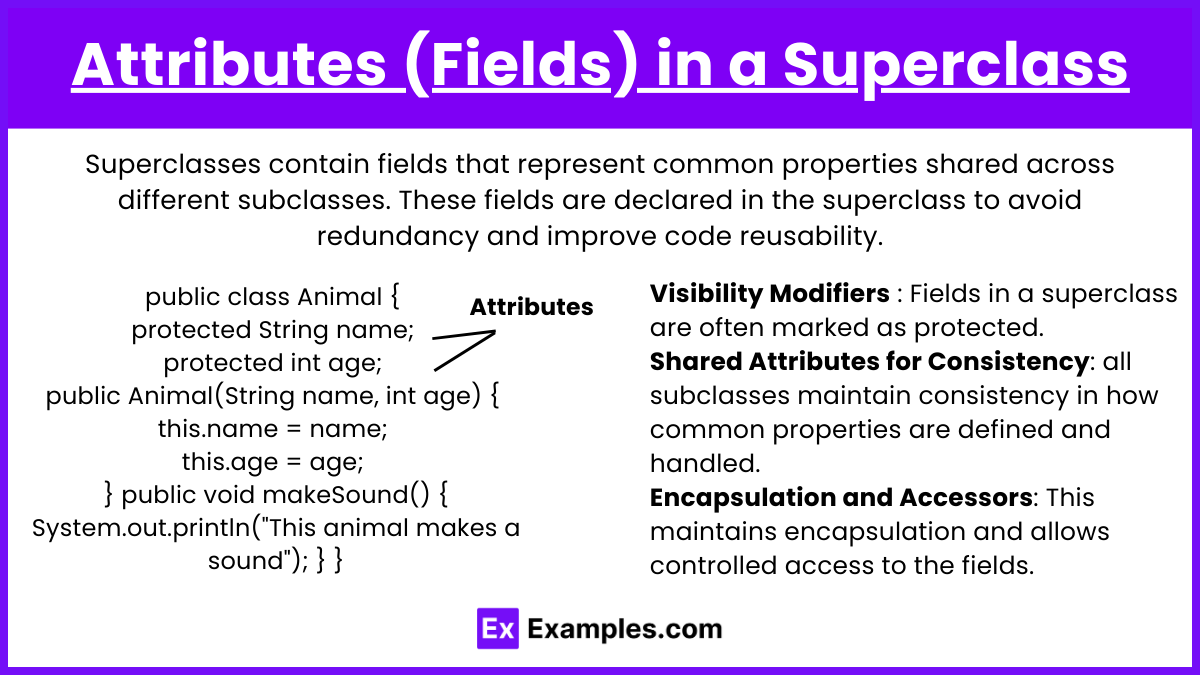
Superclasses contain fields that represent common properties shared across different subclasses. These fields are declared in the superclass to avoid redundancy and improve code reusability. For instance, if you have classes like Dog and Cat, they might both have a name and age attribute. These can be abstracted into a superclass called Animal.
Example:
public class Animal {
protected String name;
protected int age;
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
public void makeSound() {
System.out.println("This animal makes a sound");
}
}
Characteristics of Superclass Fields
- Visibility Modifiers : Fields in a superclass are often marked as protected. This allows the fields to be accessible by subclasses, while still maintaining some level of encapsulation (i.e., restricting access from unrelated classes).
- Shared Attributes for Consistency: In OOP, grouping shared attributes in a superclass ensures that all subclasses maintain consistency in how common properties are defined and handled. If the Animal superclass defines a name field, every animal will have a name attribute, regardless of whether it’s a dog, cat, or bird.
- Encapsulation and Accessors: Although fields can be declared protected for direct subclass access, a better practice is to keep fields private in the superclass and provide getter and setter methods. This maintains encapsulation and allows controlled access to the fields.
- Inheritance of Fields: When a subclass inherits from a superclass, it automatically gains all the fields of the superclass. This means that any subclass can utilize the common attributes, such as name and age, without redefining them.
Behaviors (Methods) in a Superclass
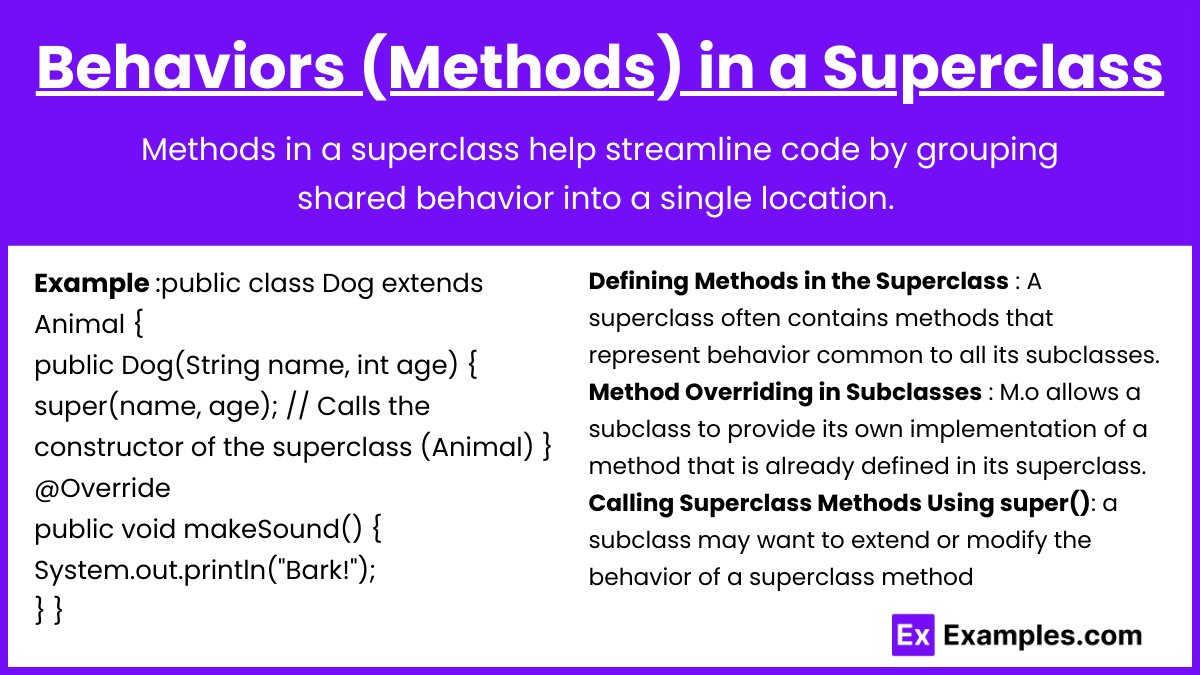
In object-oriented programming (OOP), a superclass not only contains common attributes but also defines methods (behaviors) that apply to all of its subclasses. Methods in a superclass help streamline code by grouping shared behavior into a single location. Subclasses can then either use the superclass’s methods as they are or override them to provide specific functionality tailored to that subclass. For example, all animals may have a makeSound() method, although the specific sound may differ. Subclasses can either use the superclass’s method or override it to provide specific behavior.
Example of overriding a method in a subclass:
public class Dog extends Animal {
public Dog(String name, int age) {
super(name, age); // Calls the constructor of the superclass (Animal)
}
@Override
public void makeSound() {
System.out.println("Bark!");
}
}
Concepts of Superclass Methods
- Defining Methods in the Superclass : A superclass often contains methods that represent behavior common to all its subclasses. These methods can be used directly by subclasses unless overridden.
- Method Overriding in Subclasses : Method overriding allows a subclass to provide its own implementation of a method that is already defined in its superclass. This is useful when subclasses share a method name and functionality, but the specific behavior should differ.
- Calling Superclass Methods Using super():In some cases, a subclass may want to extend or modify the behavior of a superclass method rather than completely replacing it. The super keyword can be used to call the superclass version of the method inside the overridden method.
- Abstract Methods in Superclasses: Sometimes, a superclass might define a method that should be implemented by all its subclasses, but the exact implementation will differ. In such cases, the method can be declared abstract in the superclass, which means it has no implementation in the superclass itself.
Grouping with Superclasses
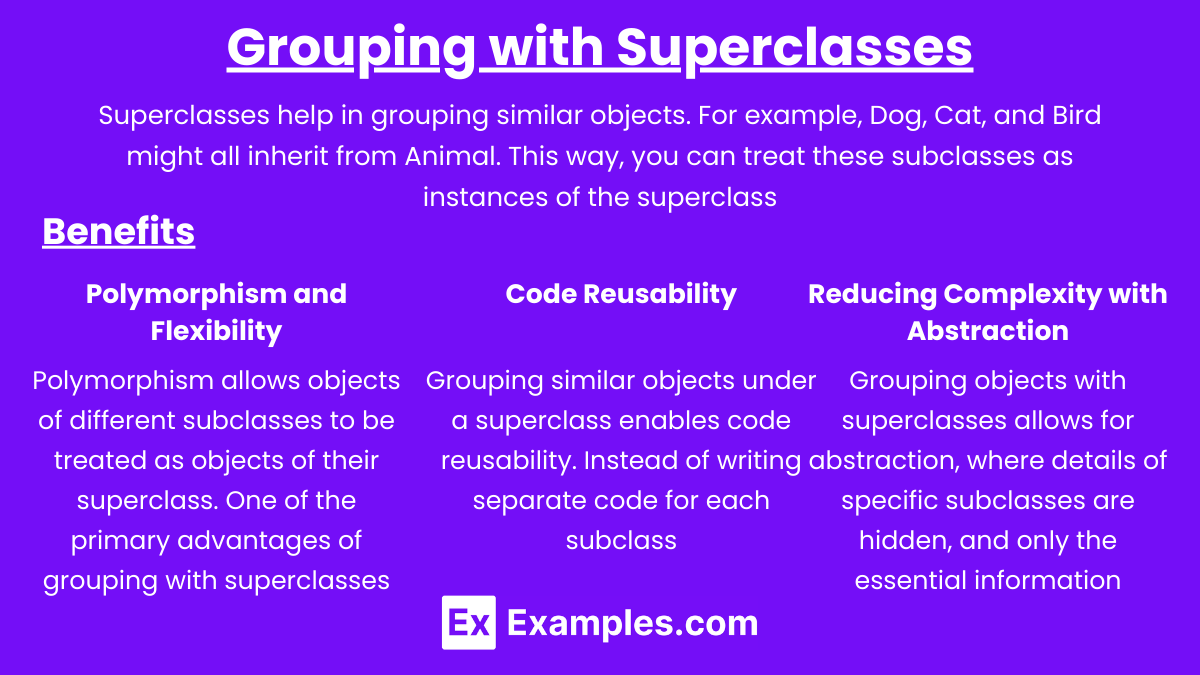
Superclasses help in grouping similar objects. For example, Dog, Cat, and Bird might all inherit from Animal. This way, you can treat these subclasses as instances of the superclass when needed, allowing you to write more flexible and generic code. Polymorphism allows objects of different subclasses (like Dog and Cat) to be handled as objects of their superclass (Animal).
For example:
Animal myPet = new Dog("Rex", 3); // Dog is treated as an Animal
myPet.makeSound(); // This will call the overridden method and print "Bark!"
Benefits of Grouping with Superclasses
- Polymorphism and Flexibility : One of the primary advantages of grouping with superclasses is polymorphism, which allows objects of different subclasses to be treated as objects of their superclass. This is extremely useful when writing generic code that can operate on different types of objects without needing to know their specific class.
- Code Reusability: Grouping similar objects under a superclass enables code reusability. Instead of writing separate code for each subclass, common behaviors and properties are written once in the superclass and inherited by all subclasses.
- Reducing Complexity with Abstraction: Grouping objects with superclasses allows for abstraction, where details of specific subclasses are hidden, and only the essential information (common attributes and behaviors) are exposed through the superclass.
Abstract Superclasses
Sometimes, a superclass is declared as abstract, meaning it cannot be instantiated directly. Instead, it provides a common structure for subclasses that implement specific details.
Example:
public abstract class Animal {
protected String name;
protected int age;
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
public abstract void makeSound(); // Abstract method to be implemented by subclasses
}
public class Cat extends Animal {
public Cat(String name, int age) {
super(name, age);
}
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
Examples
Example 1: Vehicles Superclass
A Vehicle superclass can be used to group common attributes like speed, fuelType, and behaviors like startEngine() and stopEngine(). Subclasses like Car, Truck, and Motorcycle inherit these attributes and methods, while each subclass can define specific behaviors, such as loadCargo() for a Truck or doWheelie() for a Motorcycle.
Example 2: Electronic Devices Superclass
The ElectronicDevice superclass can group common attributes such as brand, powerStatus, and behaviors like turnOn() and turnOff(). Subclasses such as Smartphone, Laptop, and Tablet can inherit these attributes and methods. Each subclass may have additional specific functionalities, like makeCall() in Smartphone or openLid() in Laptop.
Example 3: Employees Superclass
A Employee superclass can encapsulate common attributes like name, employeeId, and behaviors like calculateSalary() and takeLeave(). Subclasses such as Manager, Developer, and Designer inherit these fields and behaviors, while adding unique ones like approveProject() for Manager or writeCode() for Developer.
Example 4: Animals Superclass
The Animal superclass can group common attributes like age, weight, and behaviors such as eat() and sleep(). Subclasses like Bird, Fish, and Mammal inherit these attributes but implement behaviors differently, like fly() for Bird or swim() for Fish.
Example 5: Appliances Superclass
A HomeAppliance superclass can be created with common attributes like modelNumber, wattage, and methods such as turnOn() and turnOff(). Subclasses like WashingMachine, Refrigerator, and Microwave inherit these attributes and methods but can implement unique behaviors like startWashCycle() for WashingMachine or defrost() for Microwave.
Multiple Choice Questions
Question 1
What is the primary benefit of grouping common attributes and behaviors into a superclass in object-oriented programming?
A) It allows each subclass to have entirely different properties from other subclasses.
B) It promotes code reusability and reduces redundancy.
C) It forces all subclasses to use the exact same methods without customization.
D) It removes the need for method overriding in subclasses.
Correct Answer: B) It promotes code reusability and reduces redundancy.
Explanation: One of the main benefits of using superclasses is that common attributes and methods (behaviors) shared by subclasses can be defined in the superclass. This reduces redundancy by preventing repeated code in each subclass. Subclasses can inherit these common properties and behaviors, promoting code reusability. Options A, C, and D are incorrect because they misunderstand the concept of inheritance. Subclasses can have additional properties beyond the superclass (A), and they can override superclass methods to customize their behavior (C and D).
Question 2
Consider the following Java code:
public class Animal {
protected String name;
protected int age;
public void makeSound() {
System.out.println("This animal makes a sound");
}
}
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Bark!");
}
}
public class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow!");
}
}
What is the output when the following code is executed?
Animal myPet = new Dog();
myPet.makeSound();
A) This animal makes a sound
B) Meow!
C) Bark!
D) Compilation error
Correct Answer: C) Bark!
Explanation: In this example, myPet is declared as an Animal, but the object it refers to is a Dog. This is an example of polymorphism—even though myPet is of type Animal, the actual object is a Dog, and the overridden makeSound() method in the Dog class is called, producing the output “Bark!” The makeSound() method in the Animal class is overridden by both the Dog and Cat classes, so option A is incorrect. Option B is incorrect because myPet is a Dog, not a Cat. Option D is incorrect because the code compiles without any issues.
Question 3
Which of the following statements is true about abstract superclasses?
A) Abstract superclasses can be instantiated directly like regular classes.
B) Subclasses inheriting from an abstract superclass must provide implementations for all abstract methods.
C) Subclasses cannot override methods from an abstract superclass.
D) Abstract superclasses must contain only abstract methods.
Correct Answer: B) Subclasses inheriting from an abstract superclass must provide implementations for all abstract methods.
Explanation: An abstract superclass contains methods that may or may not be fully implemented. If a subclass inherits from an abstract superclass, it must provide concrete implementations for all the abstract methods that were not implemented in the superclass. Option A is incorrect because abstract classes cannot be instantiated directly. Option C is incorrect because subclasses can override methods (even concrete methods) from the abstract superclass. Option D is incorrect because an abstract superclass can contain both abstract and non-abstract (concrete) methods.