onditional statements in Java are crucial for decision-making in programs, enabling code to respond dynamically to different inputs. By using if, else if, else, and switch statements, developers can create flexible, responsive applications that execute specific blocks of code based on evaluated conditions. This control structure is essential for handling diverse scenarios, such as validating user input, executing different algorithms, or managing game logic. Mastering conditional statements is vital for success in the AP Computer Science A exam and beyond in programming.
Learning Objectives
When studying “Using Conditional Statements to Execute Different Statements based on Input Values” for AP Computer Science A, you should focus on understanding how to construct and use ‘ if ‘, ‘ else if ‘, ‘ else ‘, and ‘ switch ‘ statements effectively. Learn to evaluate conditions using relational and logical operators, and understand the flow of control in nested and sequential conditionals. Practice writing code that handles various scenarios, ensuring you can make accurate decisions based on input values and execute the correct code block accordingly.
Using Conditional Statements to Execute Different Statements Based on Input Values
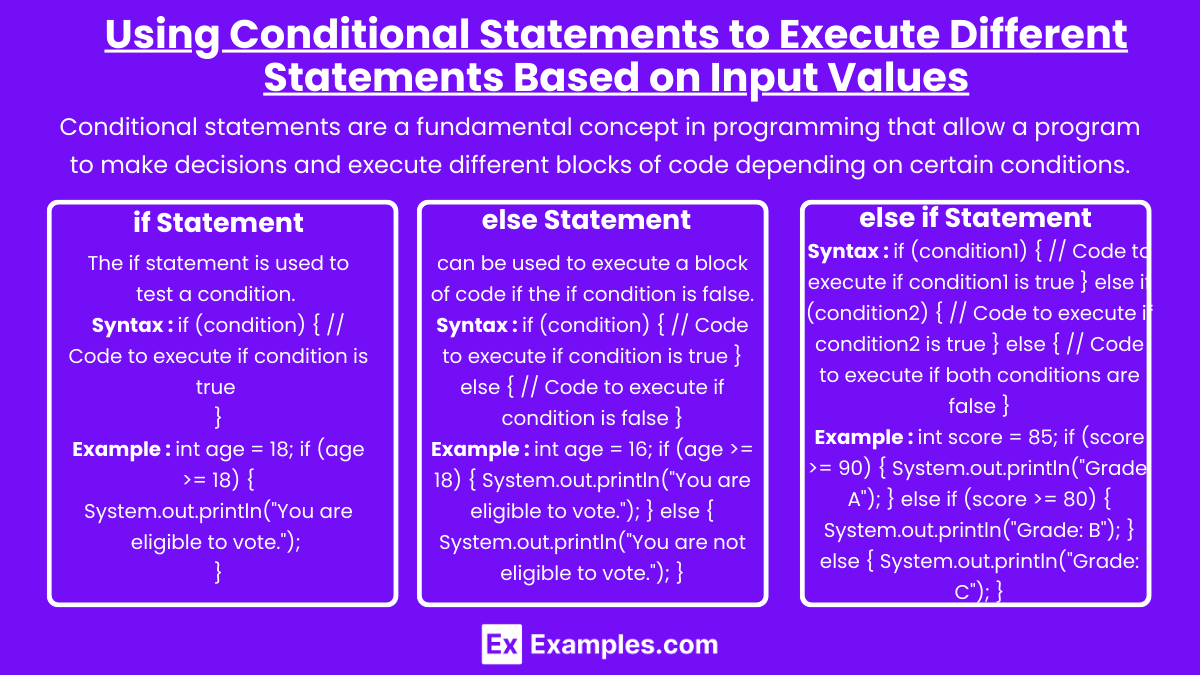
Conditional statements are a fundamental concept in programming that allow a program to make decisions and execute different blocks of code depending on certain conditions. In Java, which is the language used in the AP Computer Science A exam, the primary conditional statements are if, else if, else, and switch.
1. if Statement
The if statement is used to test a condition. If the condition evaluates to true, the code block inside the if statement is executed. If the condition is false, the program skips the if block.
Syntax:
if (condition) {
// Code to execute if condition is true
}
Example:
int age = 18;
if (age >= 18) {
System.out.println("You are eligible to vote.");
}
In this example, if age is 18 or greater, the program will print “You are eligible to vote.”
2. else Statement
The else statement can be used to execute a block of code if the if condition is false.
Syntax:
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Example:
int age = 16;
if (age >= 18) {
System.out.println("You are eligible to vote.");
} else {
System.out.println("You are not eligible to vote.");
}
In this example, since age is less than 18, the program will print “You are not eligible to vote.”
3. else if Statement
The ‘ else if ‘ statement allows you to test multiple conditions. It comes after an ‘ if ‘ statement and before an ‘ else ‘ statement.
Syntax:
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition2 is true
} else {
// Code to execute if both conditions are false
}
Example:
int score = 85;
if (score >= 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else {
System.out.println("Grade: C");
}
In this example, since score is 85, the program will print “Grade: B.”
switch and Nested Conditional Statements
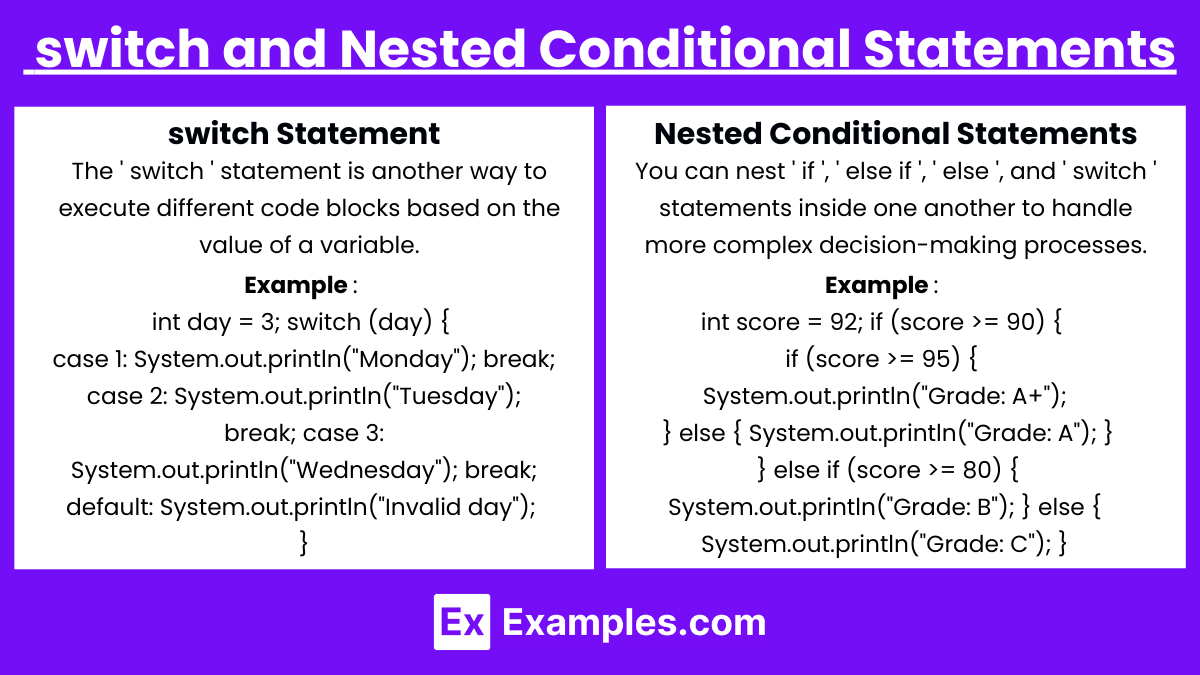
4. switch Statement
The ‘ switch ‘ statement is another way to execute different code blocks based on the value of a variable. It is generally used when you need to compare the same variable to multiple values.
Syntax:
switch (variable) {
case value1:
// Code to execute if variable == value1
break;
case value2:
// Code to execute if variable == value2
break;
// Additional cases...
default:
// Code to execute if variable doesn't match any case
}
Example:
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
default:
System.out.println("Invalid day");
}
In this example, since day is 3, the program will print “Wednesday.”
5. Nested Conditional Statements
You can nest ‘ if ‘, ‘ else if ‘, ‘ else ‘, and ‘ switch ‘ statements inside one another to handle more complex decision-making processes.
Example:
int score = 92;
if (score >= 90) {
if (score >= 95) {
System.out.println("Grade: A+");
} else {
System.out.println("Grade: A");
}
} else if (score >= 80) {
System.out.println("Grade: B");
} else {
System.out.println("Grade: C");
}
In this example, the program will print “Grade: A” because the score is 92, which is between 90 and 95.
Tips for the AP Exam
- Understand how to use each conditional statement and when it’s appropriate to use if-else, else if, or switch.
- Practice writing code that handles different input scenarios.
- Be aware of the common pitfalls, such as forgetting the break statement in a switch case or misplacing the curly braces {} in nested if statements.
- Remember that conditions are often based on relational operators (==, !=, <, >, <=, >=) and logical operators (&&, ||, !).
Examples
Example 1: Determining Voting Eligibility
Imagine a program that checks if a person is eligible to vote based on their age. The program first uses an if statement to see if the age is 18 or older. If the condition is true, it prints a message stating that the person is eligible to vote. If the age is less than 18, the else block is executed, printing a message that the person is not eligible to vote. This simple example shows how conditional statements can control the flow of a program based on user input.
Example 2: Grading System
Consider a grading system where a program assigns a letter grade based on a student’s score. Using an if-else if-else structure, the program checks if the score is 90 or above to assign an “A,” between 80 and 89 for a “B,” and between 70 and 79 for a “C.” If the score falls below 70, the program assigns a “D.” This example illustrates how multiple conditions can be evaluated to determine the appropriate response based on the input score.
Example 3: Menu Selection
In a restaurant ordering system, a program can use a switch statement to process a customer’s menu selection. The program checks the input value representing the selected menu item and executes different blocks of code to display the price or details of the selected item. If the input doesn’t match any case, the default block provides an error message or prompts the user to choose again. This is a practical use of conditional statements to manage user choices in applications.
Example 4: Password Strength Checker
A program that evaluates the strength of a password might use nested if statements to check various conditions such as length, inclusion of numbers, special characters, and uppercase letters. If all conditions are met, the program declares the password as “Strong.” If only some conditions are met, it might declare it as “Medium,” and if few or none are met, it declares the password as “Weak.” This example shows how multiple layers of conditional logic can be used to provide a more nuanced assessment based on input criteria.
Example 5: Discount Application
A retail program might use conditional statements to apply discounts based on the total purchase amount. For instance, an if-else structure could check if the total is over $100 to apply a 10% discount, between $50 and $100 for a 5% discount, and no discount for totals below $50. This conditional logic ensures that the appropriate discount is applied based on the input value, helping businesses automate pricing strategies efficiently.
Multiple Choice Questions
Question 1
What will be the output of the following code snippet?
int num = 10;
if (num < 10) {
System.out.println("Less than 10");
} else if (num == 10) {
System.out.println("Equal to 10");
} else {
System.out.println("Greater than 10");
}
A) Less than 10
B) Equal to 10
C) Greater than 10
D) No output
Correct Answer: B) Equal to 10
Explanation: In this code, the variable ‘ num ‘ is initialized to 10. The first ‘ if ‘ condition checks whether ‘ num < 10 ‘, which is false. The else if condition then checks whether ‘ num == 10 ‘, which is true. Therefore, the program will execute the ‘ System.out.println(“Equal to 10”); ‘ statement, and the output will be “Equal to 10.” The ‘ else ‘ block is not executed because the ‘ else if ‘ condition is true.
Question 2
Given the following code, what will be the output if the value of day is 5?
int day = 5;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
default:
System.out.println("Invalid day");
}
A) Monday
B) Friday
C) Invalid day
D) No output
Correct Answer: B) Friday
Explanation: In the ‘ switch ‘ statement, the value of ‘ day ‘ is 5. The program checks each case until it finds one that matches the value of ‘ day ‘. When it reaches ‘ case 5 ‘, it executes ‘ System.out.println(“Friday”); ‘ and then breaks out of the switch statement. Therefore, the output is “Friday.” The default case is not executed because a matching case was found.
Question 3
Consider the following code snippet. What will be the output?
int score = 78;
if (score >= 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else if (score >= 70) {
System.out.println("Grade: C");
} else {
System.out.println("Grade: F");
}
A) Grade: A
B) Grade: B
C) Grade: C
D) Grade: F
Correct Answer: C) Grade: C
Explanation: The variable score is set to 78. The program evaluates each condition in sequence:
- ‘ if (score >= 90) is false. ‘
- ‘ else if (score >= 80) is also false. ‘
- ‘ else if (score >= 70) is true. ‘
Since the third condition is true, the program executes ‘ System.out.println(“Grade: C”); ‘. No further conditions are evaluated, and the output is “Grade: C.” The ‘ else ‘ block is not executed because the condition score >= 70 is true.