In the AP Computer Science A course, mastering the use of class libraries is essential, particularly with the ‘ Integer ‘ and ‘ Double ‘ classes from the java.lang package. These classes wrap the primitive data types ‘ int ‘ and ‘ double ‘ in objects, offering methods for data manipulation, conversion, and comparison. Understanding these libraries facilitates handling numeric data within Java programs, enhancing both functionality and efficiency. Familiarity with these classes, including autoboxing and unboxing, is crucial for achieving high scores on the exam.
Learning Objectives
When studying “Utilizing Class Libraries, Including ‘ Integer ‘ and ‘ Double ‘ ” for the AP Computer Science A exam, focus on mastering how to use the Integer and Double classes from the ‘ java.lang package ‘. Learn to parse strings into integers and doubles, handle numeric conversions, and implement utility methods for comparisons and value manipulation. Understand autoboxing and unboxing to seamlessly convert between primitive types and their wrapper class objects. Practice applying these methods in coding exercises to solidify your comprehension and ability to use these classes effectively in various programming scenarios.
Class Libraries
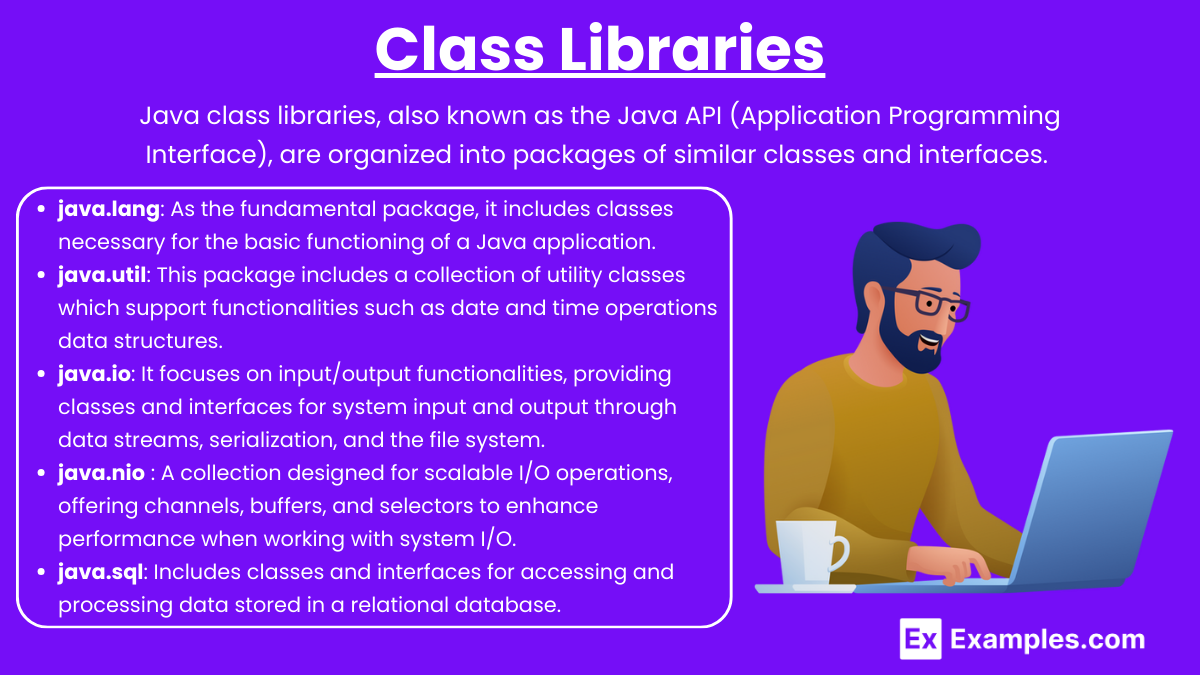
Class libraries in Java are extensive collections of pre-written classes and interfaces that provide a vast array of functionalities, from basic data manipulation to complex network operations. The java.lang package, which is automatically imported into every Java program, contains classes fundamental to the language, including Integer and Double.
Java class libraries, also known as the Java API (Application Programming Interface), are organized into packages of similar classes and interfaces. These packages provide a comprehensive framework that supports a wide range of applications. Here are some key packages:
- java.lang: As the fundamental package, it includes classes necessary for the basic functioning of a Java application. This package contains classes like String, Math, System, and wrapper classes such as Integer and Double, which are essential for data manipulation.
- java.util: This package includes a collection of utility classes which support functionalities such as date and time operations (Date, Calendar), data structures (ArrayList, HashMap, HashSet), and miscellaneous utility classes (Scanner, Random).
- java.io: It focuses on input/output functionalities, providing classes and interfaces for system input and output through data streams, serialization, and the file system.
- java.nio (New Input/Output): A collection designed for scalable I/O operations, offering channels, buffers, and selectors to enhance performance when working with system I/O.
- java.sql: Includes classes and interfaces for accessing and processing data stored in a relational database.
Utilizing the Integer and Double Classes
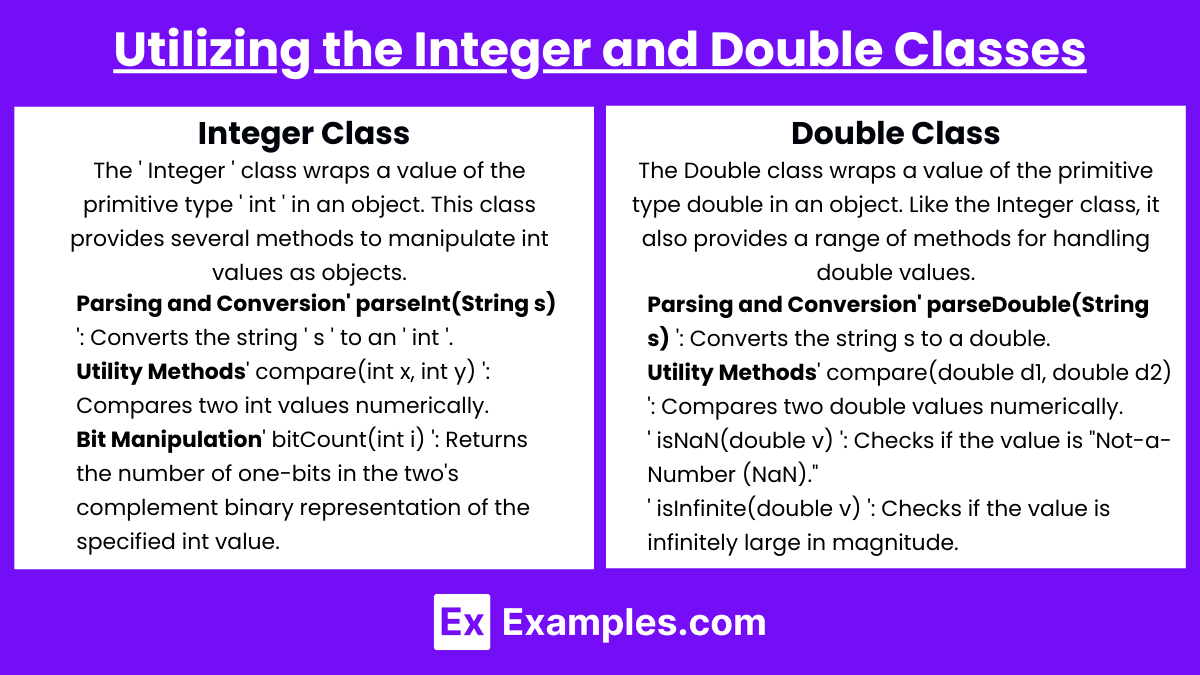
1. Integer Class
The ‘ Integer ‘ class wraps a value of the primitive type ‘ int ‘ in an object. This class provides several methods to manipulate int values as objects.
- Parsing and Conversion
- ‘ parseInt(String s) ‘: Converts the string ‘ s ‘ to an ‘ int ‘.
- ‘ valueOf(int i) ‘: Returns an ‘ Integer ‘ instance representing ‘ i ‘.
- ‘ valueOf(String s) ‘: Converts the string s to an Integer.
- Utility Methods
- ‘ compare(int x, int y) ‘: Compares two int values numerically.
- ‘ min(int a, int b) ‘: Returns the smaller of two int values.
- ‘ max(int a, int b) ‘: Returns the larger of two int values.
- Bit Manipulation
- ‘ bitCount(int i) ‘: Returns the number of one-bits in the two’s complement binary representation of the specified int value.
2. Double Class
The Double class wraps a value of the primitive type double in an object. Like the Integer class, it also provides a range of methods for handling double values.
- Parsing and Conversion
- ‘ parseDouble(String s) ‘: Converts the string s to a double.
- ‘ valueOf(double d) ‘: Returns a Double instance representing d.
- ‘ valueOf(String s) ‘: Converts the string s to a Double.
- Utility Methods
- ‘ compare(double d1, double d2) ‘: Compares two double values numerically.
- ‘ isNaN(double v) ‘: Checks if the value is “Not-a-Number (NaN).”
- ‘ isInfinite(double v) ‘: Checks if the value is infinitely large in magnitude.
3. Autoboxing and Unboxing
Java allows automatic conversion between the primitive types and their corresponding object wrapper classes. This feature is known as autoboxing (for converting primitives to objects) and unboxing (for converting objects back to primitives).
Example of Autoboxing
Integer myInt = 5; // autoboxing int to Integer
Example of Unboxing
int myPrimitiveInt = myInt; // unboxing Integer to int
Examples
Example 1: Converting Strings to Numbers
One common task in programming is converting strings to numbers, especially when receiving input from a user or reading a file. The ‘ Integer ‘ and ‘ Double ‘ classes provide parsing methods for this purpose. For example, if you have a string that represents an integer, you can use ‘ Integer.parseInt(“123”) ‘ to convert it to the primitive int type. Similarly, for a string representing a floating-point number, ‘ Double.parseDouble(“3.14”) ‘ converts it into a ‘ double ‘.
Example 2: Handling Null Values with Wrapper Classes
The wrapper classes ‘ Integer ‘ and ‘ Double ‘ can be particularly useful when you need to deal with possible null values which primitive types can’t handle. For example, in a scenario where user input might not be mandatory, and you want to store either an integer value or no value at all, ‘ Integer ‘ can be used. You can store ‘ null ‘ in an Integer object, something that’s not possible with the primitive int type.
Example 3: Using Utility Methods for Mathematical Operations
The ‘ Integer ‘ and ‘ Double ‘ classes include utility methods such as ‘ min() ‘, ‘ max() ‘, and ‘ abs() ‘, which can be extremely handy. For example, to find the maximum or minimum of two values without writing additional code to check which one is larger or smaller, you can simply use ‘ Integer.max(5, 10) ‘ or ‘ Double.min(5.5, 10.5) ‘.
Example 4: Autoboxing in Collections
Java allows objects of wrapper classes to be stored directly in collections, which only accept objects, not primitive types. This feature of autoboxing helps in seamlessly converting primitives to objects. For instance, when adding elements to an ‘ ArrayList ‘, you can add ‘ int ‘ or ‘ double ‘ directly, and Java automatically converts these primitives into ‘ Integer ‘ or ‘ Double ‘ objects, respectively. This is demonstrated by:
ArrayList<Integer> list = new ArrayList<>();
list.add(3); // Autoboxing converts int to Integer automatically
Example 5: Comparing Numeric Objects
When dealing with numeric data, there are often requirements to compare values not just for equality, but also to determine which is smaller or larger. The ‘ Integer ‘ and ‘ Double ‘ classes provide methods such as ‘ compareTo() ‘, which can be used to compare two objects. For example, ‘ new Integer(5).compareTo(new Integer(10)) ‘ returns a negative value indicating that 5 is less than 10. This method is useful in sorting and other operations where order is important.
Multiple Choice Questions
Question 1
What is the result of the following Java code snippet?
System.out.println(Integer.parseInt("123") + Integer.parseInt("456"));
A) 579
B) “579”
C) An error occurs
D) None of the above
Correct Answer: A) 579
Explanation:
The ‘ Integer.parseInt(String) ‘ method is used to convert a string into its equivalent integer value. In this code, ‘ “123” ‘ is converted to 123 and ‘ “456” ‘ to ‘ 456 ‘. These numbers are then added together, resulting in ‘ 579 ‘. The ‘ System.out.println() ‘ method prints this integer value to the console. No error occurs, and the output is not a string, hence option A is correct.
Question 2
Which line of code correctly creates a ‘ Double ‘ object representing the value 10.5?
A) ‘ Double d = 10.5; ‘
B) ‘ Double d = new Double(10.5); ‘
C) ‘ Double d = Double.parseDouble(“10.5”); ‘
D) ‘ Double d = Double.valueOf(“10.5”); ‘
Correct Answer: D) Double.valueOf(“10.5”);
Explanation:
Option A uses autoboxing to create a ‘ Double ‘ object, but it isn’t the typical method for explicitly creating a ‘ Double ‘ object from a string. Option B uses the constructor of the ‘ Double ‘ class, which is valid but deprecated as of Java 9. Option C is incorrect because ‘ Double.parseDouble(String) ‘ returns a primitive ‘ double ‘, not a ‘ Double ‘ object. Option D is correct because ‘ Double.valueOf(String) ‘ correctly parses the string ‘ “10.5” ‘ and returns a ‘ Double ‘ object.
Question 3
Consider the following Java code:
Integer a = 100;
Integer b = 100;
Integer c = 150;
Integer d = 150;
System.out.println((a == b) + ", " + (c == d));
What is the output of this code?
A) true, true
B) false, false
C) true, false
D) false, true
Correct Answer: C) true, false
Explanation:
Java caches ‘ Integer ‘ objects for values between -128 and 127. Therefore, when ‘ a ‘ and ‘ b ‘ are both assigned the value ‘ 100 ‘, they refer to the same cached ‘ Integer ‘ object, making ‘ (a == b) ‘ true because they are the same object. However, ‘ c ‘ and ‘ d ‘, being assigned the value ‘ 150 ‘, do not fall within this cache range. Consequently, ‘ c ‘ and ‘ d ‘ are different ‘ Integer ‘ objects, making ‘ (c == d) ‘ false because they are not the same object. This demonstrates an interesting aspect of ‘ Integer ‘ class behavior related to object caching in Java.