In AP Computer Science A, understanding where variables can be used in program code is crucial for managing data and ensuring proper functionality. Variables can have different scopes that determine their accessibility. These include local variables, which are limited to a specific method or block; instance variables, which belong to an object; and static variables, which are shared among all instances of a class. Properly managing variable scope is essential for avoiding errors, maintaining clean code, and ensuring variables are used in the correct context.
Learning Objectives
In studying “Where Variables Can be Used in Program Code” for AP Computer Science A, you should learn how to identify and differentiate between local, instance, static, and parameter variables. You should understand the scope and lifetime of each variable type, how they are accessed, and where they are visible in the program. Additionally, grasp the concept of variable shadowing and recognize the importance of scope management to avoid errors such as variable re-declaration or access issues. Mastering these concepts ensures efficient and error-free coding practices.
Where Variables Can be Used in Program Code
In Java, variables are an essential component that store data values. Where variables can be used in the program is determined by their scope, which is the region in the code where a variable is accessible.
1. Local Variables
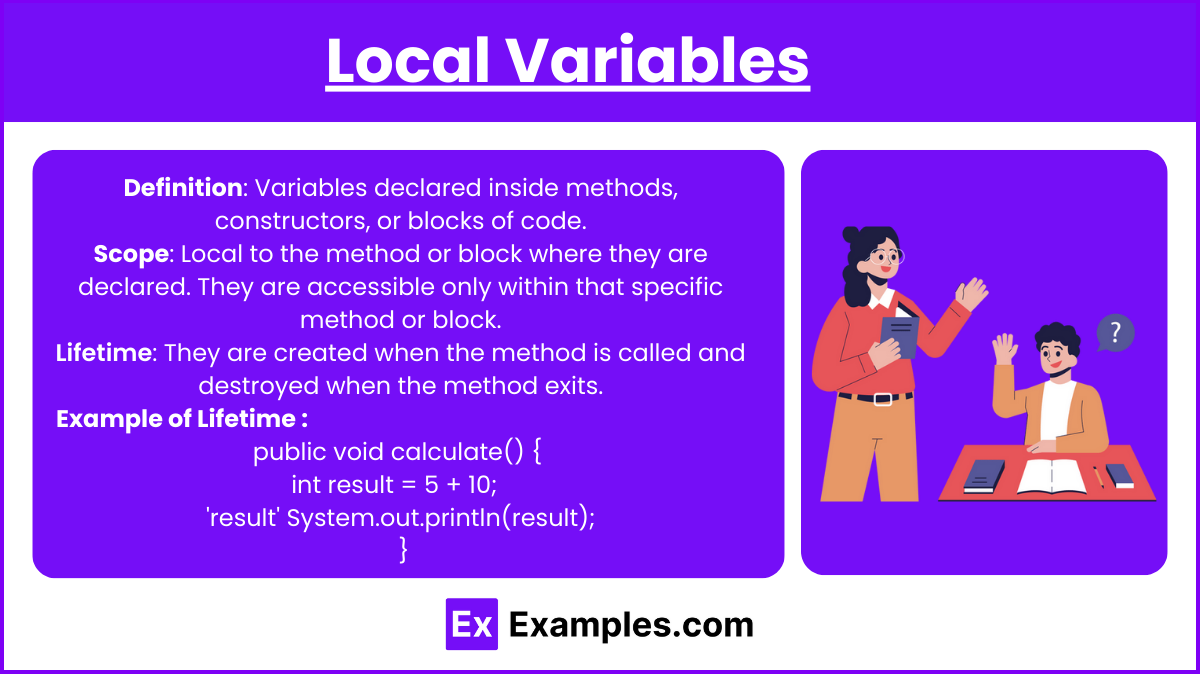
- Definition: Variables declared inside methods, constructors, or blocks of code. Local variables are variables that are declared within a method, constructor, or block of code (e.g., within a for loop or if statement). They are only accessible within the structure they are declared in and are used to temporarily hold data during the execution of that method or block. They are typically used for intermediate computations or to store method arguments.
- Scope: Local to the method or block where they are declared. They are accessible only within that specific method or block.The scope of a local variable is confined to the method or block where it is declared. This means it cannot be accessed outside of that specific method or block. If the method calls another method, the local variable cannot be passed automatically; it would have to be explicitly passed as an argument if needed in other methods.
- Lifetime: They are created when the method is called and destroyed when the method exits.Local variables are created when the method, block, or constructor in which they are defined is invoked, and they are destroyed when that method, block, or constructor exits. This means that their lifetime is tied to the execution of the specific method or block they are in.
- Example of Lifetime:
public void calculate() {
int result = 5 + 10; // Local variable 'result'
System.out.println(result); // 'result' exists here
} // 'result' is destroyed after this method finishes
2. Instance Variables (Non-static Fields)
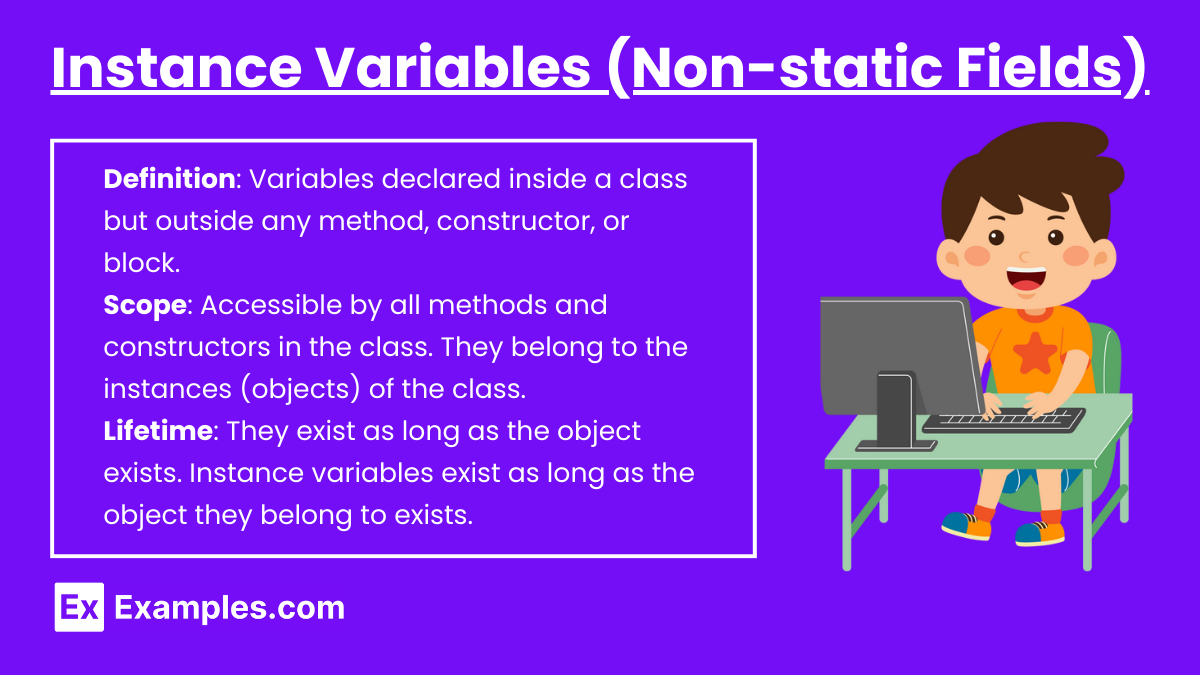
- Definition: Variables declared inside a class but outside any method, constructor, or block. Instance variables are variables that are declared within a class but outside any method, constructor, or block. These variables are also known as non-static fields because they are associated with an instance of the class, not the class itself. This means that each object (instance) of the class has its own copy of the instance variables.
- Scope: Accessible by all methods and constructors in the class. They belong to the instances (objects) of the class. The scope of instance variables is the entire class in which they are declared. They can be accessed by all methods, constructors, and blocks within the class. Unlike local variables, they are not restricted to a single method or block.
- Lifetime: They exist as long as the object exists. Instance variables exist as long as the object they belong to exists. They are created when an object is instantiated using the new keyword and destroyed when the object is garbage collected. Their values are preserved as long as the object is accessible within the program.
- Example of Lifetime:
public class MyClass {
int age; // instance variable
public MyClass(int age) {
this.age = age; // initialized when the object is created
}
}
MyClass obj = new MyClass(30); // instance variable 'age' exists
// 'age' is destroyed when 'obj' is garbage collected
3. Static Variables (Class Variables)
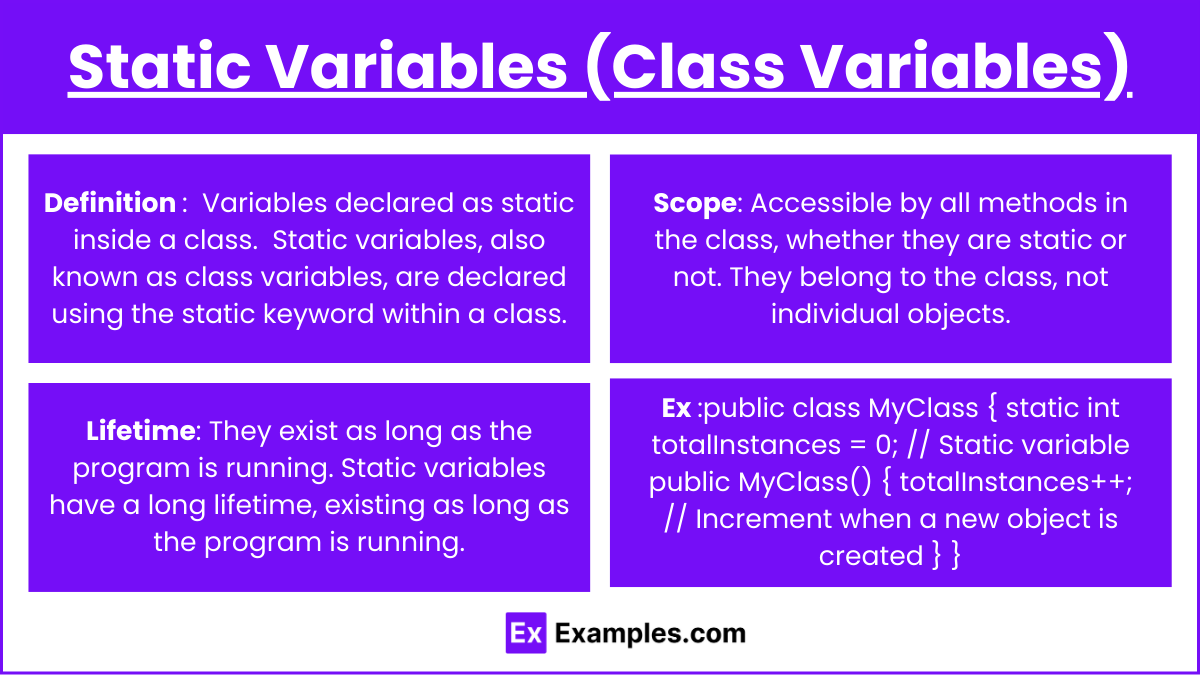
- Definition: Variables declared as static inside a class. They are shared by all objects of the class. Static variables, also known as class variables, are declared using the static keyword within a class. Unlike instance variables, which are tied to specific objects (instances of a class), static variables are shared among all instances of the class.
- Scope: Accessible by all methods in the class, whether they are static or not. They belong to the class, not individual objects. Static variables have a broader scope than instance or local variables. They are accessible by all methods in the class, whether the methods are static or not.
- Lifetime: They exist as long as the program is running. Static variables have a long lifetime, existing as long as the program is running. They are created when the class is loaded into memory, and they are destroyed when the program terminates.
- Example of Lifetime:
public class MyClass {
static int totalInstances = 0; // Static variable
public MyClass() {
totalInstances++; // Increment when a new object is created
}
}
MyClass obj1 = new MyClass(); // totalInstances = 1
MyClass obj2 = new MyClass(); // totalInstances = 2
4. Parameter Variables
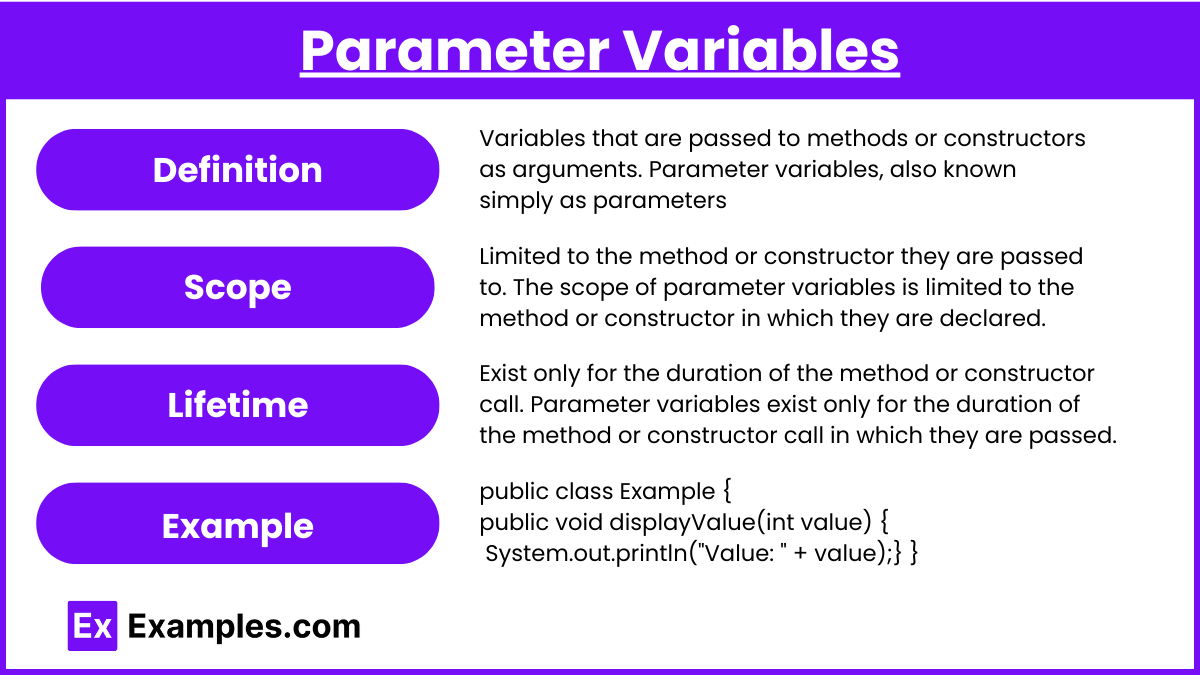
- Definition: Variables that are passed to methods or constructors as arguments. Parameter variables, also known simply as parameters, are variables that are passed to methods or constructors when they are called. They allow the caller to pass values into the method or constructor, which can then be used within the body of the method.
- Scope: Limited to the method or constructor they are passed to. The scope of parameter variables is limited to the method or constructor in which they are declared. Once the method or constructor finishes executing, the parameter variables go out of scope and are no longer accessible.
- Lifetime: Exist only for the duration of the method or constructor call. Parameter variables exist only for the duration of the method or constructor call in which they are passed. When the method is called, parameter variables are created, and once the method completes execution, these variables are destroyed.
- Example of Lifetime:
public class Example {
public void displayValue(int value) { // 'value' is a parameter variable
System.out.println("Value: " + value); // 'value' exists during the method call
} // 'value' is destroyed after the method ends
}
Example ex = new Example();
ex.displayValue(100); // Prints: Value: 100
5. Block Scope (Loop Variables)
- Definition: Variables declared within a block of code like for, while, or if blocks.
- Scope: Limited to the block in which they are declared.
- Lifetime: Exist only for the duration of the block.
- Example of Lifetime:
public void loopExample() {
for (int i = 0; i < 5; i++) { // 'i' is block-scoped to this for loop
System.out.println("Iteration: " + i);
}
// System.out.println(i); // ERROR: i cannot be accessed outside the for loop
}
Examples
Example 1: Local Variables Inside a Method
Local variables are declared within a method and can only be accessed inside that method. For example, in a calculateTotal() method, a variable int total = 0; may be declared and used only within that method. Once the method finishes executing, the variable total is no longer accessible. This ensures the variable is local to the method, avoiding unintended interference with other parts of the program.
Example 2: Instance Variables in a Class
Instance variables are declared outside any method but within the class body. These variables belong to an instance of the class and can be accessed by any method in that class. For example, if a class Car has an instance variable int speed;, all methods in the Car class can access speed as long as they are working with an instance of Car. This type of variable allows data to persist between method calls on an object.
Example 3: Static Variables for Class-Level Access
Static variables are shared by all instances of a class. They are declared using the static keyword inside the class but outside any method. For instance, a static variable static int count; in the class Employee can be accessed by any object of Employee, and any change to count will be reflected in all objects. Static variables are useful when a value is shared across all instances, such as maintaining a record count of how many objects have been created.
Example 4: Parameter Variables Passed to Methods
Variables can be passed to methods as parameters. For instance, a method void printName(String name) accepts name as a parameter. The variable name is local to that method, meaning it can only be used within the printName method. This is an example of how variables can be passed into a method, used within that method, but are not accessible outside of it.
Example 5: Variables Inside a Loop (Block Scope)
Variables can be declared within a loop or conditional block and are only accessible within that block. For example, in a for loop for (int i = 0; i < 10; i++), the variable i is local to the loop block. Outside the loop, the variable i is no longer valid. Block-scoped variables ensure that loop control variables or other block-specific data are contained within the loop, preventing them from affecting other parts of the program.
Multiple Choice Questions
Question 1
Where can a local variable be used in Java?
a) Throughout the class
b) Inside the method where it was declared
c) Inside any method in the class
d) Anywhere in the program
Answer: b) Inside the method where it was declared
Explanation: A local variable is declared within a method, and its scope is limited to that specific method. This means it can only be accessed and used within the method where it was declared. Once the method finishes executing, the local variable is destroyed, making it unavailable outside the method.
Question 2
What is true about instance variables in Java?
a) They can be used in any class
b) They are shared across all instances of a class
c) They are specific to each object and can be accessed by any method of that class
d) They are declared inside methods
Answer: c) They are specific to each object and can be accessed by any method of that class
Explanation: Instance variables are variables declared in the class but outside any methods. They belong to each instance (object) of the class, meaning each object has its own copy of the instance variables. These variables can be accessed by any method within the class, allowing object-specific data to persist across method calls.
Question 3
What is the scope of a static variable in Java?
a) Limited to the method in which it was declared
b) Limited to the object in which it was created
c) Shared among all objects of the class and can be accessed by static methods
d) Available only in the main method
Answer: c) Shared among all objects of the class and can be accessed by static methods
Explanation: A static variable is declared with the static keyword, meaning it belongs to the class rather than any particular object. All objects of that class share the same static variable. Static variables can be accessed by both static and non-static methods of the class, but static methods must only work with static variables, as they don’t have access to instance-specific data.