Developing algorithms is a core concept in AP Computer Science Principles, focusing on creating systematic, step-by-step solutions to problems. An algorithm is a logical sequence of instructions that a computer follows to perform tasks or solve complex challenges efficiently. In this course, students learn to design algorithms using methods like iteration, recursion, and conditionals, while considering factors like time and space complexity. Understanding algorithm development is essential for tackling real-world problems in programming, making it a fundamental skill for achieving success on the exam.
Learning Objectives
When learning about “Developing Algorithms” for the AP Computer Science Principles Exam, focus on understanding how to design, analyze, and implement step-by-step procedures for solving problems. You should learn to use pseudocode to outline solutions, analyze time and space complexity for efficiency, and apply common algorithm strategies like divide and conquer or dynamic programming. Additionally, you should practice developing algorithms for searching, sorting, and recursion, while ensuring your solutions handle edge cases and optimize performance using appropriate data structures.
Basic Algorithm Design
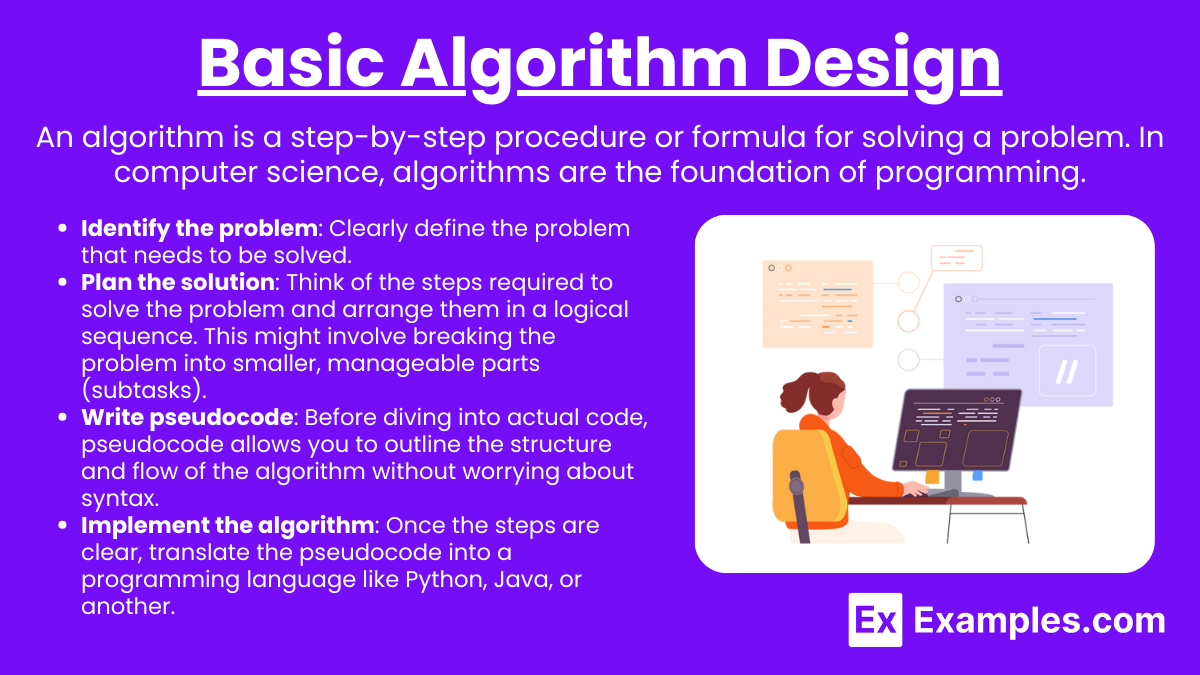
An algorithm is a step-by-step procedure or formula for solving a problem. In computer science, algorithms are the foundation of programming. They are used to outline instructions that the computer can follow to perform tasks, calculate results, or solve complex problems.
- Identify the problem: Clearly define the problem that needs to be solved.
- Plan the solution: Think of the steps required to solve the problem and arrange them in a logical sequence. This might involve breaking the problem into smaller, manageable parts (subtasks).
- Write pseudocode: Before diving into actual code, pseudocode allows you to outline the structure and flow of the algorithm without worrying about syntax. It provides a clear, language-agnostic view of the logic.
- Implement the algorithm: Once the steps are clear, translate the pseudocode into a programming language like Python, Java, or another.
Algorithm Types
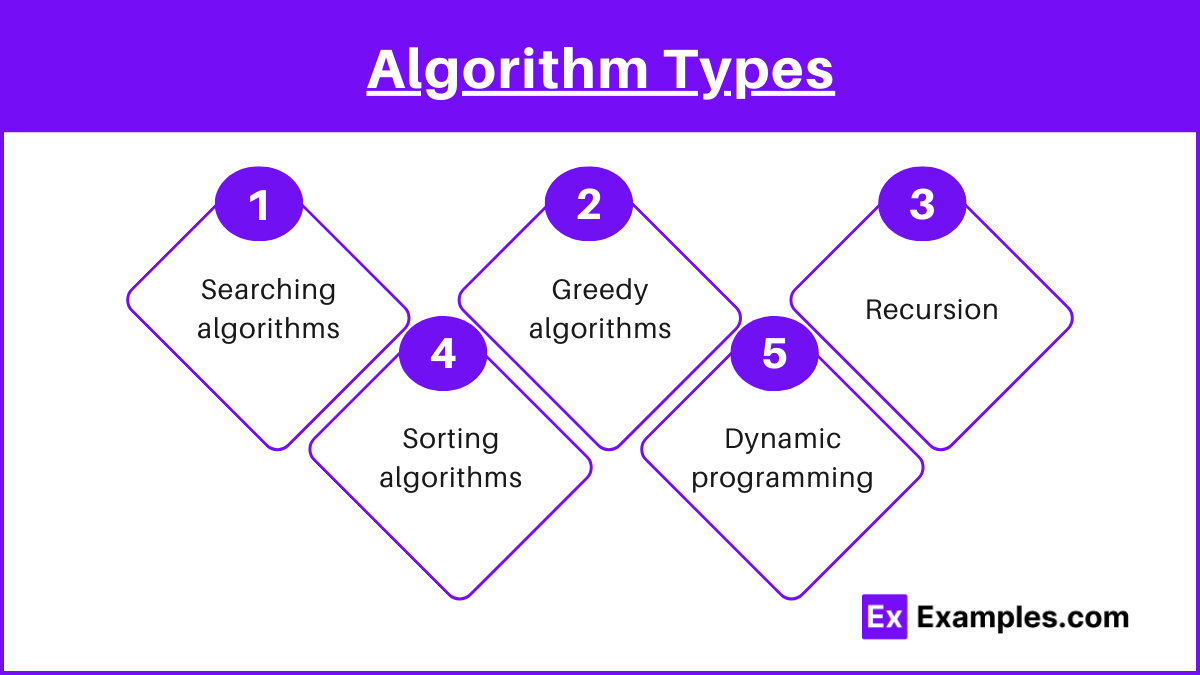
- Searching algorithms: These help find an element in a data structure (e.g., Linear Search, Binary Search).
- Sorting algorithms: These arrange data in a particular order (e.g., Bubble Sort, Merge Sort, Quick Sort).
- Greedy algorithms: These make the optimal choice at each step, hoping to find the global optimum (e.g., Prim’s algorithm, Dijkstra’s algorithm).
- Dynamic programming: This breaks down problems into simpler sub-problems and stores the results for future use (e.g., Fibonacci sequence, Knapsack problem).
- Recursion: A function that calls itself to solve smaller instances of the problem (e.g., Tower of Hanoi, factorial calculation).
Steps to Develop an Algorithm
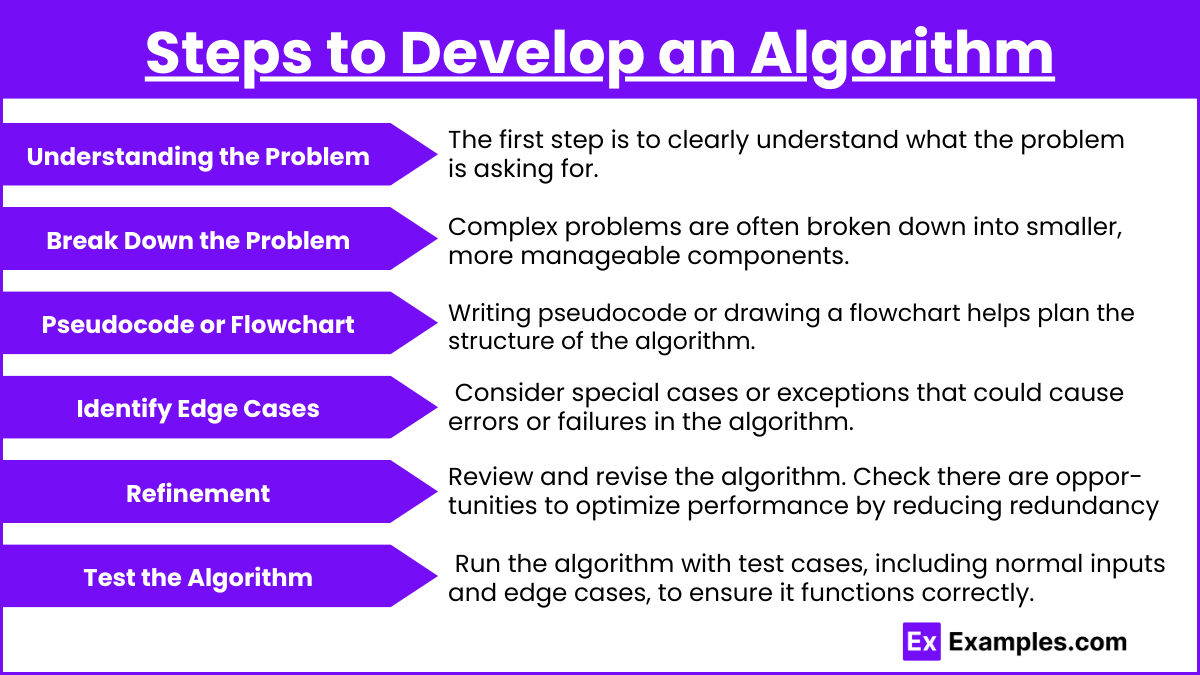
- Understanding the Problem: The first step is to clearly understand what the problem is asking for. Identify what inputs will be needed and what outputs are expected.
- Break Down the Problem: Complex problems are often broken down into smaller, more manageable components. This process is known as decomposition.
- Pseudocode or Flowchart: Writing pseudocode or drawing a flowchart helps plan the structure of the algorithm. It allows you to focus on the logic of the algorithm without getting bogged down by specific programming syntax.
- Identify Edge Cases: Consider special cases or exceptions that could cause errors or failures in the algorithm.
- Refinement: Review and revise the algorithm. Check if there are opportunities to optimize performance by reducing redundancy, time complexity, or space complexity.
- Test the Algorithm: Run the algorithm with test cases, including normal inputs and edge cases, to ensure it functions correctly.
Algorithm Design Techniques
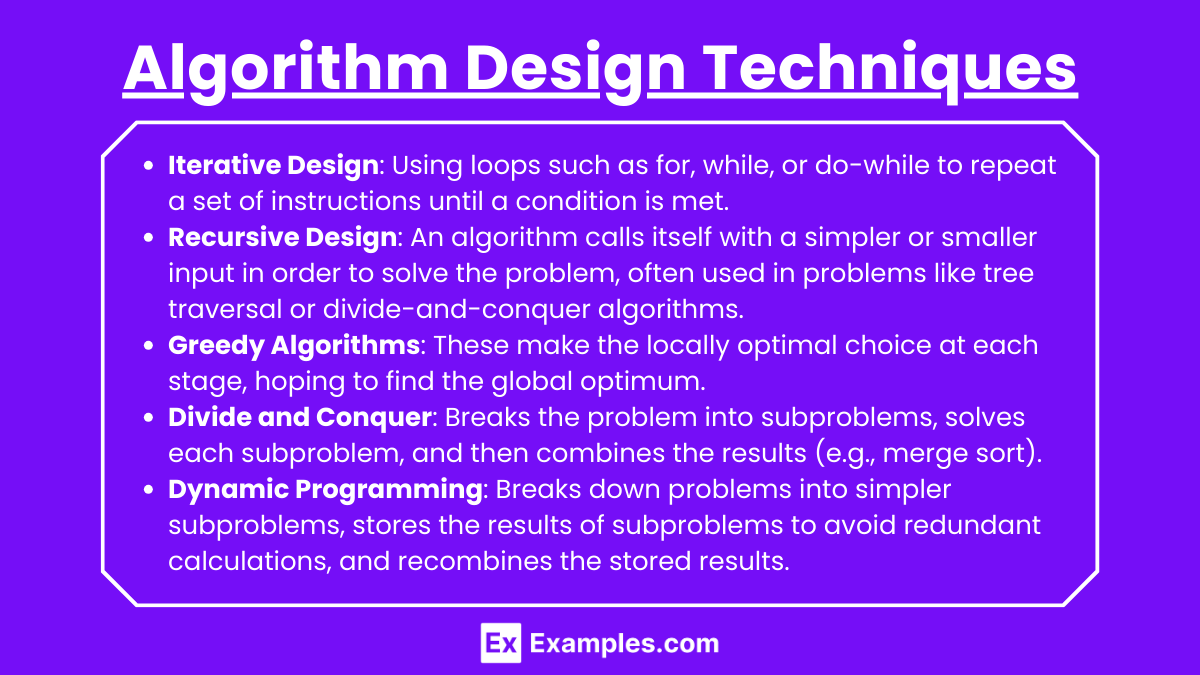
- Iterative Design: Using loops such as for, while, or do-while to repeat a set of instructions until a condition is met.
- Recursive Design: An algorithm calls itself with a simpler or smaller input in order to solve the problem, often used in problems like tree traversal or divide-and-conquer algorithms.
- Greedy Algorithms: These make the locally optimal choice at each stage, hoping to find the global optimum (e.g., selecting the largest item first).
- Divide and Conquer: Breaks the problem into subproblems, solves each subproblem, and then combines the results (e.g., merge sort).
- Dynamic Programming: Breaks down problems into simpler subproblems, stores the results of subproblems to avoid redundant calculations, and recombines the stored results.
Testing and Debugging Algorithms
- Dry Run: Manually walking through the algorithm with sample inputs to understand its flow and identify any errors.
- Edge Cases: Test the algorithm with special cases such as minimum or maximum input values, empty input, or invalid data.
- Algorithm Trace: Keeping track of variable values and the path through the algorithm as it runs helps in debugging issues.
Examples
Example 1: Sorting a List of Numbers Using Bubble Sort
The Bubble Sort algorithm works by repeatedly stepping through the list of numbers, comparing adjacent elements, and swapping them if they are in the wrong order. This process continues until no more swaps are needed, meaning the list is sorted. It’s a simple algorithm with a time complexity of O(n²), making it inefficient for large datasets, but it is a great starting point for understanding algorithm development. The steps are clearly defined, and it involves a nested loop structure to manage the repeated comparisons and swaps.
Example 2: Finding the Maximum Value in an Array
To develop an algorithm that finds the maximum value in an array, you start by assuming the first element is the largest. Then, you iterate through the rest of the array, comparing each element with the current maximum. If a larger element is found, you update the maximum value. By the end of the iteration, the algorithm returns the largest value. This algorithm is simple, efficient, and has a time complexity of O(n), where n is the number of elements in the array. It showcases how a problem can be solved by breaking it down into iterative steps.
Example 3 : Searching a Sorted List Using Binary Search
Binary Search is an efficient algorithm for searching a sorted list. The algorithm works by dividing the search space in half at each step. First, it compares the middle element of the list to the target value. If the middle element is the target, it returns the index. If the target is smaller, it searches the left half; if larger, it searches the right half. This process continues until the target is found or the search space is empty. With a time complexity of O(log n), Binary Search is much faster than linear search algorithms, making it an excellent example of a divide-and-conquer approach.
Example 4: Solving the Fibonacci Sequence Using Recursion
The Fibonacci sequence is a classic example of developing an algorithm using recursion. In this algorithm, each number in the sequence is the sum of the two preceding ones, starting from 0 and 1. To solve it recursively, the algorithm defines the base case (Fibonacci(0) = 0, Fibonacci(1) = 1) and recursively calls itself to calculate the next numbers. Although this recursive solution is elegant, it has a time complexity of O(2ⁿ) due to redundant calculations, making it a good example to demonstrate optimization techniques like memoization or dynamic programming.
Example 5: Calculating Factorials Using Iteration
The factorial of a number n (denoted as n!) is the product of all positive integers from 1 to n. To develop an algorithm for calculating a factorial iteratively, you can start with a result of 1 and multiply it by each number from 2 to n. This algorithm is efficient, with a time complexity of O(n). Unlike its recursive counterpart, the iterative approach avoids the overhead of recursive calls, demonstrating how algorithm development can involve choosing between iterative and recursive methods for efficiency.
Multiple Choice Questions
Question 1
Which of the following best describes an algorithm?
A) A piece of hardware that executes code
B) A sequence of instructions to solve a specific problem
C) A programming language used to create software
D) A data structure used for storing elements
Answer: B) A sequence of instructions to solve a specific problem
Explanation: An algorithm is defined as a step-by-step sequence of instructions designed to perform a specific task or solve a problem. Option B is the correct definition. Option A refers to hardware, which is unrelated to algorithms. Option C refers to programming languages, which are used to write algorithms but are not the algorithms themselves. Option D refers to a data structure, which is often used in algorithms but is not an algorithm on its own.
Question 2
Which of the following algorithms uses the “divide and conquer” strategy?
A) Linear Search
B) Binary Search
C) Bubble Sort
D) Selection Sort
Answer: B) Binary Search
Explanation: Binary Search uses the “divide and conquer” strategy by repeatedly dividing the search space in half until the target element is found. This results in a much faster search process compared to linear search, with a time complexity of O(log n). The other options (A, C, D) are not divide and conquer algorithms. Linear Search checks each element one by one (O(n)), while Bubble Sort and Selection Sort are sorting algorithms that do not involve dividing the problem into sub-problems.
Question 3
What is the time complexity of the Binary Search algorithm?
A) O(n)
B) O(log n)
C) O(n²)
D) O(1)
Answer: B) O(log n)
Explanation: The Binary Search algorithm has a time complexity of O(log n) because it reduces the search space by half at each step, leading to a logarithmic growth pattern. Option A (O(n)) refers to linear search, which checks each element one by one. Option C (O(n²)) is common for algorithms like Bubble Sort. Option D (O(1)) represents constant time, which would only apply if the answer were found immediately without needing any iterations.