API
An API (Application Programming Interface) is a set of rules and protocols that allows different software applications to communicate with each other. It defines the methods and data formats that applications can use to request and exchange information, enabling seamless integration and interaction between disparate systems. APIs can be particularly useful in various contexts, such as integrating job application emails with an application tracking system to streamline the hiring process. In a business application, APIs enable different software components to work together, enhancing overall functionality and efficiency.
What is an API?
Examples of API
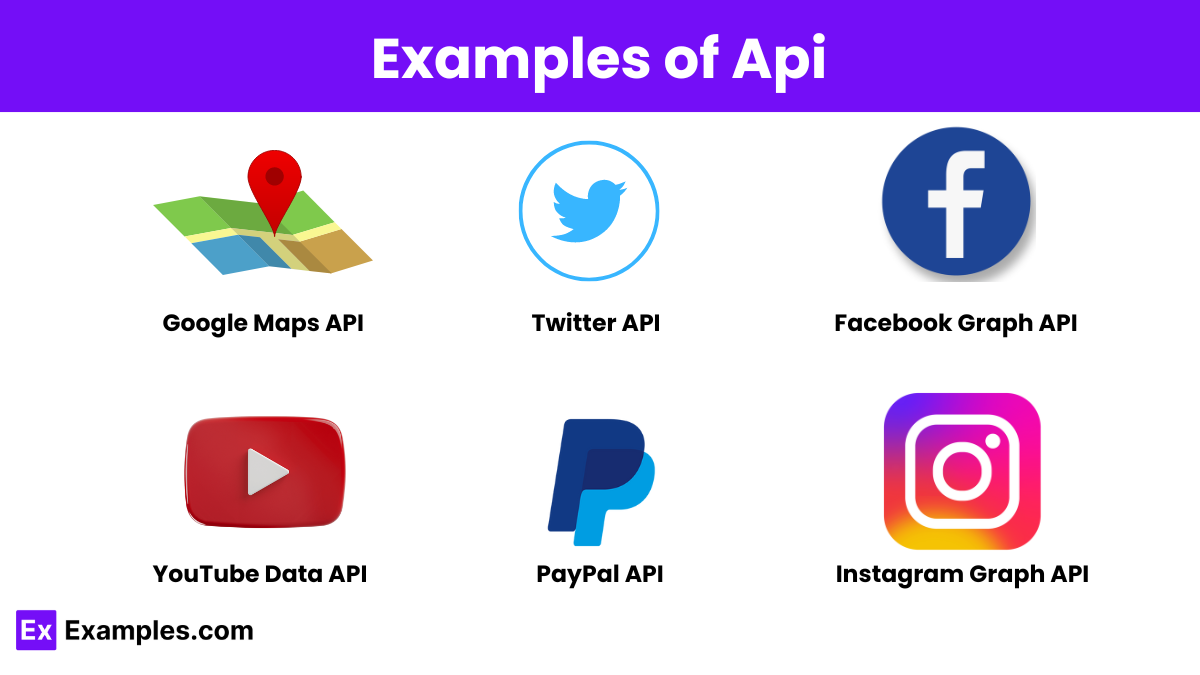
- Google Maps API
- Twitter API
- Facebook Graph API
- Stripe API
- YouTube Data API
- OpenWeatherMap API
- Spotify Web API
- Slack API
- PayPal API
- Amazon Web Services (AWS) API
- Dropbox API
- Twilio API
- Instagram Graph API
- Microsoft Graph API
- LinkedIn API
Types of APIs
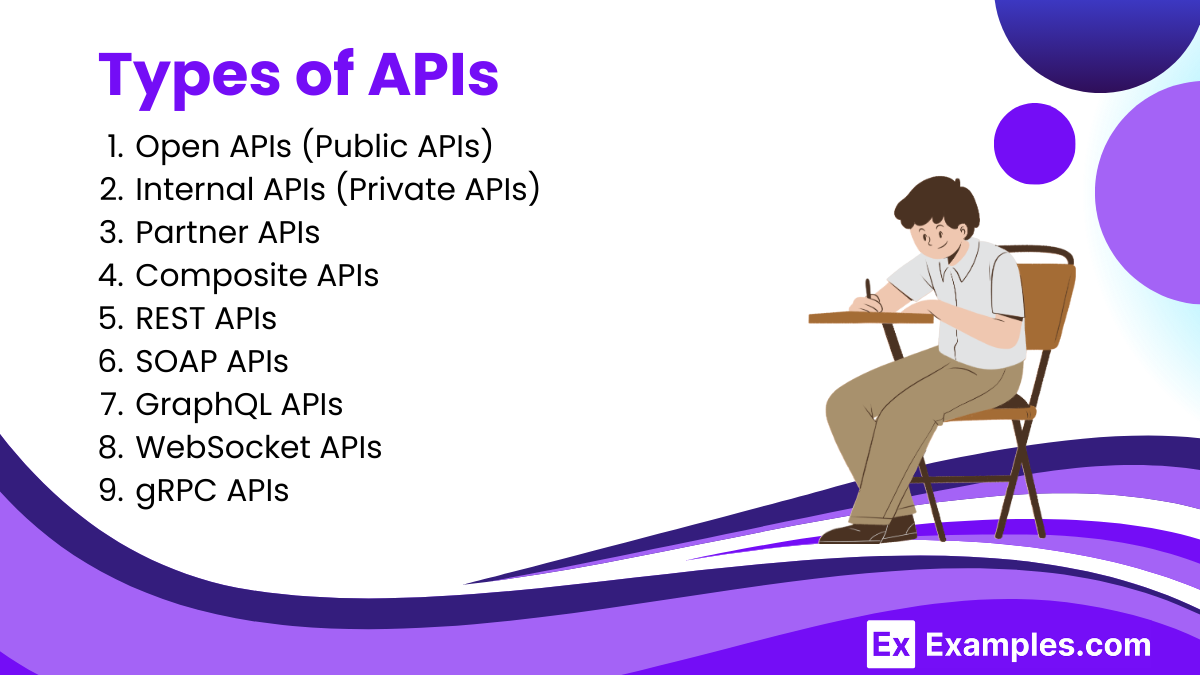
1. Open APIs (Public APIs)
- Description: Available to developers and other users with minimal restrictions. They are intended for external users (developers at other companies, for example).
- Examples: Twitter API, Google Maps API.
- Use Cases: Public-facing services, allowing third-party developers to integrate with the platform.
2. Internal APIs (Private APIs)
- Description: Used within an organization to connect systems and data internally.
- Examples: APIs that connect internal HR systems with payroll systems.
- Use Cases: Enhancing internal processes, improving system integrations within the company.
3. Partner APIs
- Description: Shared with specific business partners. These are more controlled and intended for a specific purpose.
- Examples: APIs shared between a travel agency and airline reservation systems.
- Use Cases: Enabling business partnerships, integrating partner services.
4. Composite APIs
- Description: Combine multiple data or service APIs. They allow developers to access several endpoints in one call.
- Examples: An API that combines data from various microservices in a single response.
- Use Cases: Simplifying complex API interactions, improving efficiency for complex operations.
5. REST APIs
- Description: Use Representational State Transfer architecture, typically interacting over HTTP.
- Examples: Most web APIs, such as those used by Facebook, Amazon, and YouTube.
- Use Cases: Web services, web applications, and mobile apps.
6. SOAP APIs
- Description: Use Simple Object Access Protocol, a protocol for exchanging structured information.
- Examples: Legacy systems, financial services, and payment gateways.
- Use Cases: Enterprise-level web services, transactions requiring high security and compliance.
7. GraphQL APIs
- Description: A query language for APIs that allows clients to request exactly the data they need.
- Examples: APIs used by GitHub, Shopify, and Facebook.
- Use Cases: Applications requiring efficient data retrieval, and scenarios where precise data fetching is critical.
8. WebSocket APIs
- Description: Provide full-duplex communication channels over a single, long-lived connection.
- Examples: Real-time chat applications, live sports updates.
- Use Cases: Real-time applications, bidirectional data transfer.
9. gRPC APIs
- Description: A high-performance, open-source framework for remote procedure calls (RPC).
- Examples: APIs in microservices architecture, and distributed systems.
- Use Cases: Low-latency, high-throughput applications, inter-service communication in microservices.
How do APIs work?
- Client Initiates Request:
- The client application sends a request to the API endpoint using an appropriate HTTP method (e.g., GET, POST).
- The request may include parameters, headers, and a body containing necessary data.
- Server Processes Request:
- The API server receives the request and processes it based on the provided parameters and instructions.
- The server performs the necessary actions, such as querying a database, executing a function, or modifying data.
- Server Sends Response:
- After processing the request, the server sends a response back to the client.
- The response typically includes a status code indicating the success or failure of the request, along with any requested data or error messages.
- Client Receives Response:
- The client receives the response and processes it accordingly.
- If the request was successful, the client uses the returned data to perform further actions or display information to the user.
- If there was an error, the client handles it based on the error code and message.
REST APIs
REST (Representational State Transfer) APIs are a popular architectural style for designing networked applications. They use standard HTTP methods and follow a set of principles to create scalable and stateless interactions between clients and servers.
REST APIs can be used to streamline various processes, such as managing a lawn care application by integrating scheduling and service requests with backend systems. They can also help maintain an application inventory, ensuring that different software applications and services are cataloged and accessible. Additionally, REST APIs can facilitate the submission and processing of a scholarship fund application, providing seamless integration between applicants and scholarship databases.
Web API
A Web API (Application Programming Interface) is a set of rules and protocols for building and interacting with web-based software applications. It defines how different software components should interact over the internet or a network, enabling the exchange of data and functionalities between disparate systems.
API Integrations
API integrations involve connecting different software systems using Application Programming Interfaces (APIs) to enable them to communicate and share data seamlessly. These integrations are essential for creating interconnected, efficient, and scalable software solutions. For instance, integrating a payment application with an e-commerce platform can streamline transactions, improve user experience, and ensure secure data exchange between the payment system and the online store.
Benefits of REST APIs
- Simplicity: REST APIs use standard HTTP methods, making them easy to understand and implement.
- Scalability: Statelessness and separation of client and server enable efficient scaling.
- Flexibility: Can handle multiple data formats (e.g., JSON, XML), making them versatile for various applications.
- Interoperability: Platform-independent and can be used with any programming language that supports HTTP.
- Performance: Lightweight and faster due to less overhead compared to other protocols like SOAP.
- Modularity: Clear structure and endpoints promote modular development and easier maintenance.
- Caching: Supports HTTP caching mechanisms, improving performance and reducing server load.
- Statelessness: Each request is independent, simplifying server design and improving reliability.
API Endpoint and its Importance
An API endpoint is a specific URL where an API interacts with client applications. It represents a distinct function or resource within an API, such as retrieving user data or updating a record. Each endpoint corresponds to a particular method (e.g., GET, POST, PUT, DELETE) that performs a defined action on the resource.
Importance of API Endpoints
- Accessibility: Provides a clear and structured way for clients to access specific functions or data within an API.
- Organization: Helps organize API functions into distinct, manageable routes, making the API easier to use and understand.
- Efficiency: Allows precise interaction with resources, ensuring efficient communication between the client and server.
- Security: Enables implementation of security measures (e.g., authentication, authorization) at specific points in the API.
- Scalability: Facilitates modular and scalable API design, allowing independent development and scaling of different endpoints.
Secure a REST API
Securing a REST API involves implementing various measures to protect data and ensure that only authorized users can access and manipulate resources. Here are key strategies:
1. Authentication
Ensure that only authenticated users can access the API. Use robust authentication mechanisms:
- API Keys: Simple tokens for basic security.
- OAuth 2.0: Standard protocol for authorization.
- JWT (JSON Web Tokens): Secure token-based authentication.
2. Authorization
Control what authenticated users can do:
- Role-Based Access Control (RBAC): Assign permissions based on user roles.
- Scope-Based Access Control: Limit access based on specific API scopes.
3. Encryption
Protect data in transit and at rest:
- HTTPS: Use SSL/TLS to encrypt data sent over the network.
- Encryption: Encrypt sensitive data stored in databases.
4. Rate Limiting and Throttling
Prevent abuse and denial-of-service attacks:
- Rate Limiting: Restrict the number of requests a user can make in a given period.
- Throttling: Gradually slow down or block excessive requests.
5. Input Validation
Prevent injection attacks by validating inputs:
- Sanitization: Clean inputs to remove malicious content.
- Validation: Ensure inputs meet expected formats and types.
6. Error Handling
Avoid exposing sensitive information:
- Generic Messages: Return generic error messages to avoid revealing system details.
- Logging: Log detailed errors internally for troubleshooting.
7. Security Headers
Enhance API security with HTTP headers:
- Content Security Policy (CSP): Prevent cross-site scripting attacks.
- Strict-Transport-Security (HSTS): Ensure all communications use HTTPS.
8. Monitoring and Logging
Track and respond to suspicious activity:
- Monitoring: Continuously monitor API usage.
- Logging: Maintain detailed logs of all API requests and responses.
How to Create an API
Creating an API involves several key steps, from planning to deployment. Here’s a concise guide:
1. Define Objectives and Requirements
Determine the purpose of your API and what functionality it needs to provide. Identify the resources, endpoints, and methods required.
2. Choose the Technology Stack
Select the appropriate programming language, framework, and tools based on your needs and expertise. Common choices include:
- Language: JavaScript (Node.js), Python, Java, Ruby
- Framework: Express (for Node.js), Flask (for Python), Spring Boot (for Java), Rails (for Ruby)
3. Design the API
Create a detailed API design including:
- Endpoints: Define the URLs and the HTTP methods (GET, POST, PUT, DELETE) for each endpoint.
- Data Format: Choose the data format (usually JSON or XML) for requests and responses.
- Authentication: Decide on the authentication method (e.g., API keys, OAuth).
4. Set Up the Environment
Prepare your development environment by installing necessary tools and libraries. Set up a version control system (e.g., Git) for managing your code.
5. Develop the API
Write the code for your API:
- Create Endpoints: Implement the routes and handlers for each endpoint.
- Data Handling: Integrate with a database (e.g., MySQL, MongoDB) to manage data.
- Error Handling: Implement robust error handling and validation.
6. Test the API
Conduct thorough testing to ensure functionality and reliability:
- Unit Testing: Test individual components.
- Integration Testing: Ensure all components work together.
- API Testing Tools: Use tools like Postman or Insomnia to test API endpoints.
7. Document the API
Provide clear documentation for users and developers:
- API Documentation Tools: Use tools like Swagger or Postman to generate interactive documentation.
- Details: Include endpoint descriptions, request/response examples, and authentication methods.
8. Deploy the API
Deploy your API to a server or cloud platform:
- Hosting: Choose a hosting provider (e.g., AWS, Heroku, DigitalOcean).
- CI/CD Pipeline: Set up continuous integration and deployment pipelines for automatic updates and deployment.
9. Monitor and Maintain
Continuously monitor your API for performance and security:
- Monitoring Tools: Use tools like New Relic or Datadog.
- Maintenance: Regularly update and improve your API based on user feedback and new requirements.
API Testing
- Define Test Cases
- Identify the endpoints to be tested, the expected inputs and outputs, and any edge cases.
- Set Up the Environment
- Prepare the testing environment, ensuring that the API server is running and accessible.
- Execute Tests
- Use API testing tools to send requests to the API and validate the responses against the expected results.
- Analyze Results
- Review the test results, identifying any failures or discrepancies and investigating the causes.
- Report and Fix Issues
- Document any issues found during testing and work with developers to resolve them.
- Repeat Testing
- Re-test after fixes are applied to ensure issues are resolved and no new issues are introduced.
How to Write API Documentation
- Overview: Provide a brief introduction to the API, including its purpose and functionality.
- Authentication: Explain the authentication methods used (e.g., API keys, OAuth) and how to obtain access credentials.
- Base URL: Specify the base URL for the API endpoints.
- Endpoints:
- List each endpoint with its HTTP method (GET, POST, PUT, DELETE).
- Provide a brief description of what each endpoint does.
- Include the endpoint URL path.
- Parameters:
- Detail any required and optional parameters for each endpoint.
- Explain the parameter types (e.g., query, path, body) and their formats.
- Request Examples:
- Provide example requests for each endpoint using different HTTP methods.
- Show examples with various parameter combinations.
- Response Examples:
- Include example responses for successful requests.
- Show error responses and status codes for common errors.
- Error Codes: List common error codes with their meanings and possible solutions.
- Rate Limits: Explain any rate limits imposed on API requests and how to handle them.
- Versioning: Document the API versioning strategy and how to specify versions in requests.
- Best Practices: Provide tips and best practices for using the API effectively.
- Code Samples: Include code snippets in various programming languages to demonstrate how to interact with the API.
- FAQs: Address common questions and issues that users might encounter.
- Contact Information: Provide support contact details for users who need help.
How to Use an API?
Using an API involves making requests to the API endpoints and handling the responses. Here’s a step-by-step guide:
1. Understand the API Documentation
- Read the Overview: Understand the purpose and functionality of the API.
- Check Authentication Requirements: Learn how to authenticate with the API (e.g., API keys, OAuth).
2. Obtain API Credentials
- Sign Up: Register for an account with the API provider if required.
- Get API Key or Token: Obtain the necessary credentials for authentication.
3. Familiarize Yourself with Endpoints
- Identify Endpoints: Determine the endpoints you need to interact with.
- Understand HTTP Methods: Know which HTTP methods (GET, POST, PUT, DELETE) to use for each endpoint.
4. Set Up Your Environment
- Choose Tools: Use tools like Postman for testing or write code in a programming language (e.g., Python, JavaScript).
- Install Necessary Libraries: Install libraries like
requests
in Python oraxios
in JavaScript to make HTTP requests.
5. Make API Requests
- Build the Request URL: Combine the base URL with the endpoint and any required parameters.
- Add Headers: Include necessary headers such as
Authorization
,Content-Type
, and any custom headers. - Send the Request: Use the appropriate HTTP method to send the request.
Where Can I find New APIs?
You can find new APIs on various platforms and directories dedicated to API discovery, such as RapidAPI, ProgrammableWeb, and Postman API Network. These platforms provide extensive catalogs of APIs across different categories and industries, complete with documentation, usage examples, and user reviews. Additionally, developer forums, tech blogs, and websites of popular service providers often feature announcements of new APIs, making it easier to stay updated on the latest offerings.
GraphQL
GraphQL is a query language for APIs and a runtime for executing those queries by using a type system you define for your data. Developed by Facebook in 2012 and released as an open-source project in 2015, GraphQL provides a more efficient, powerful, and flexible alternative to traditional REST APIs.
Amazon API Services
Amazon API Services, provided by Amazon Web Services (AWS), encompass a wide range of APIs that allow developers to interact with AWS infrastructure and services programmatically. These APIs enable the automation of tasks, integration with AWS services, and the creation of robust, scalable applications.
What is a webhook?
A webhook is a way for an application to provide real-time information to other applications by sending HTTP POST requests when certain events occur.
How do APIs benefit businesses?
APIs benefit businesses by enabling automation, improving data sharing, enhancing user experiences, and fostering innovation through third-party integrations.
What is OAuth?
OAuth is an open standard for access delegation, allowing users to grant third-party applications limited access to their resources without sharing credentials.
What are API rate limits?
API rate limits restrict the number of requests a client can make to an API within a specified time period to prevent abuse and ensure fair usage.
What is versioning in APIs?
Versioning in APIs allows developers to introduce changes or improvements without disrupting existing users, typically by including a version number in the URL.
What is an SDK?
An SDK (Software Development Kit) is a collection of tools, libraries, and documentation that helps developers create applications for specific platforms or frameworks.
How are APIs used in web development?
APIs allow web applications to request data from servers, enabling dynamic content, third-party service integration, and enhanced functionality.
What is SOAP?
SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in web services, using XML.
What is an API endpoint?
An API endpoint is a specific URL where an API can access the resources it needs to perform its function.
What is JSON in APIs?
JSON (JavaScript Object Notation) is a lightweight data-interchange format often used to structure data in API requests and responses.