JSON – Examples, Usage, Comparison, Difference
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It is primarily used to transmit data between a server and web application. JSON data is organized in key-value pairs and is often used for APIs and web services. A JSON schema is used to define the structure, content, and types of data for JSON objects, ensuring data consistency and validation.
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It uses key-value pairs and arrays to represent structured data, commonly used for web APIs.
JSON Examples
Simple JSON Object
{
“name”: “John Doe”,
“age”: 30,
“city”: “New York”
}
{
“student”: {
“name”: “Jane Smith”,
“age”: 22,
“subjects”: {
“major”: “Computer Science”,
“minor”: “Mathematics”
}
}
}
Nested JSON Object
{
“student”: {
“name”: “Jane Smith”,
“age”: 22,
“subjects”: {
“major”: “Computer Science”,
“minor”: “Mathematics”
}
}
}
JSON Array
{
“employees”: [
{
“name”: “Alice Johnson”,
“position”: “Developer”
},
{
“name”: “Bob Brown”,
“position”: “Designer”
}
]
}
JSON with Mixed Data Types
{
“id”: 123,
“isActive”: true,
“balance”: 2500.75,
“contacts”: [
{
“type”: “email”,
“value”: “***************“
},
{
“type”: “phone”,
“value”: “+123456789”
}
]
}
JSON Employee Example
{
“employee”: {
“id”: 101,
“firstName”: “John”,
“lastName”: “Doe”,
“age”: 35,
“position”: “Software Engineer”,
“department”: “Development”,
“email”: “***************“,
“phone”: “+123456789”,
“address”: {
“street”: “123 Main St”,
“city”: “San Francisco”,
“state”: “CA”,
“zip”: “94105”
},
“skills”: [“JavaScript”, “Python”, “Java”],
“projects”: [
{
“name”: “Project Alpha”,
“role”: “Lead Developer”,
“duration”: “6 months”
},
{
“name”: “Project Beta”,
“role”: “Software Engineer”,
“duration”: “1 year”
}
]
}
}
Why Use JSON?
- Lightweight Data Format: JSON’s lightweight nature ensures quick data transfer, essential for API performance.
- Easy to Read and Write: Human-readable format makes it easy for developers to understand and work with JSON data.
- Language Independent: JSON’s compatibility with various programming languages enhances its utility in API development.
- Structured Data: Key-value pairs and arrays in JSON provide a clear structure, making data management straightforward.
- Supports Complex Data Structures: JSON can represent nested objects and arrays, accommodating complex data models in APIs.
Using JSON in API Calls
- Simplifies Data Exchange: JSON simplifies data exchange between a client and server. Its lightweight format ensures quick and efficient data transfer, crucial for robust communication protocols.
- Enhances Readability: JSON’s structure, based on key-value pairs, is human-readable. This readability helps developers quickly understand and debug data sent and received through APIs.
- Language Compatibility: JSON is language-independent, which means it can be easily parsed and generated by various programming languages. This makes it a universal format for API calls in diverse development environments.
- Structured Data Handling: JSON’s ability to represent structured data, including nested objects and arrays, allows for the transmission of complex data models in API calls. This ensures that data integrity and structure are maintained during the exchange.
- Supports Asynchronous Operations: JSON is commonly used with asynchronous operations, such as AJAX (Asynchronous JavaScript and XML). This enhances the efficiency of web applications by allowing non-blocking data exchange, improving user experience and application performance.
Using JSON Files in JavaScript
- Loading JSON Data: Retrieve the original data from a JSON file using methods like the
fetch
API orXMLHttpRequest
. - Parsing JSON Data: Convert JSON strings into JavaScript objects with the
JSON.parse()
method, making it easier to handle the data. - Accessing and Modifying Data: Once converted to a JavaScript object, access and modify properties like any other JavaScript object.
- Converting to JSON: Use the
JSON.stringify()
method to convert modified JavaScript objects back to JSON strings for sending or storing. - Using JSON in Web Applications: Combine JSON data with JavaScript DOM manipulation to dynamically update web content without reloading the page.
Comparison to JSON, YAML & XML
- Readability:
- JSON: Easy to read and write, especially for those familiar with JavaScript.
- YAML: More human-readable due to its minimal syntax.
- XML: Verbose with more complex syntax, making it harder to read.
- Data Format:
- JSON: Uses key-value pairs and arrays.
- YAML: Similar structure to JSON but supports additional features like comments.
- XML: Uses nested tags, providing a hierarchical structure.
- Parsing and Serialization:
- JSON: Native support in most programming languages, easy to parse.
- YAML: Requires third-party libraries for parsing in many languages.
- XML: Well-supported but requires more complex parsing logic.
- Use in Business Cases:
- JSON: Ideal for web APIs and data interchange due to its simplicity and efficiency. Widely used in modern web applications, making it suitable for many business cases.
- YAML: Often used in configuration files and data serialization where human readability is crucial.
- XML: Preferred in legacy systems and applications requiring strict data validation and complex document structures.
- Schema Validation:
- JSON: Supports schema validation through JSON Schema, ensuring data integrity.
- YAML: Lacks native schema validation, requiring additional tools.
- XML: Strong schema validation using DTD or XSD, making it reliable for strict data validation.
JSON Python
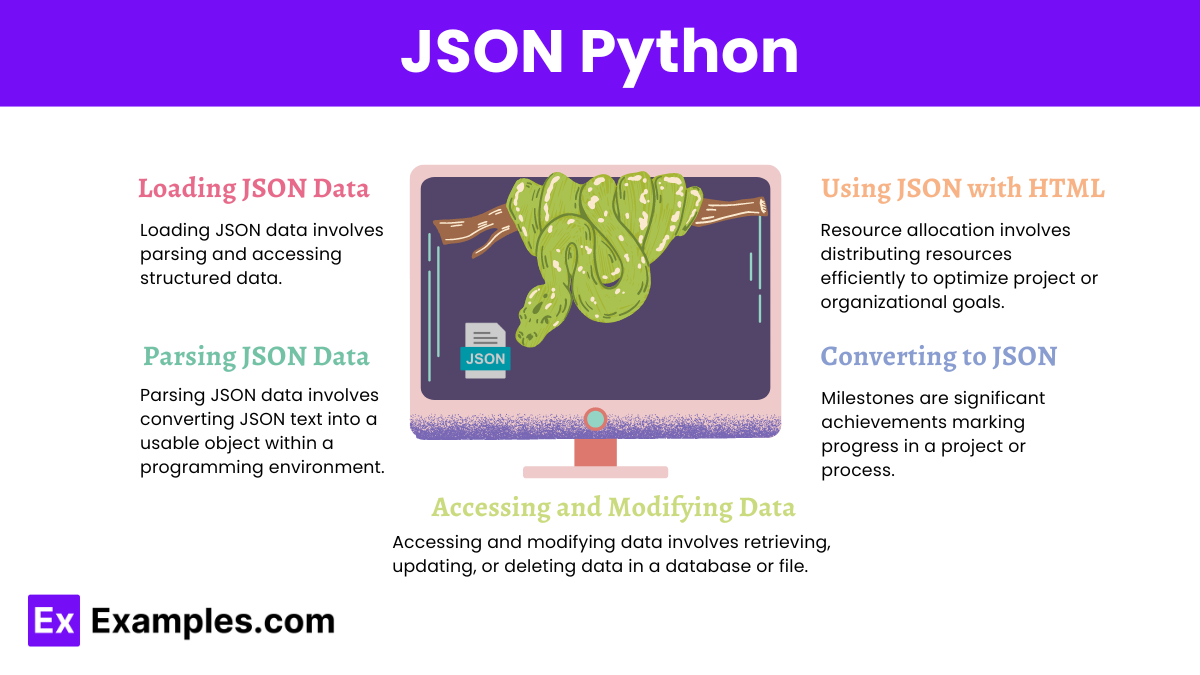
- Loading JSON Data:
- Use Python’s built-in
json
module to load JSON data from a file or string.
- Use Python’s built-in
- Parsing JSON Data:
- Convert JSON strings into Python dictionaries using
json.loads()
.
- Convert JSON strings into Python dictionaries using
- Accessing and Modifying Data:
- Once parsed, access and modify JSON data like any other Python dictionary.
- Converting to JSON:
- Use
json.dumps()
to convert Python dictionaries back into JSON strings.
- Use
- Using JSON with HTML:
- JSON data can be embedded within HTML pages to dynamically update content using JavaScript.
Differences Between JavaScript and JSON
Aspect | JavaScript | JSON |
---|---|---|
Definition | Programming language used to create dynamic web content | Data format for storing and transporting data |
Purpose | To build interactive websites and web applications | To exchange data between server and client |
Syntax | Complex, includes variables, functions, loops, etc. | Simple, key-value pairs in a structured format |
Data Types | Supports various data types (objects, arrays, strings) | Supports a subset of JavaScript data types |
Usage | Used for scripting web pages, server-side development | Used for configuration files, data interchange in APIs |
Executable | Code that can be executed by a JavaScript engine | Plain text that cannot be executed |
Flexibility | Highly flexible, allowing for complex logic and control | Limited to data representation, no logic |
Comments | Supports comments for documentation | Does not support comments |
Parsing and Stringifying | Not required to parse or stringify | Requires parsing (JSON.parse() ) and stringifying (JSON.stringify() ) |
Format | Human-readable but can be complex | Human-readable and lightweight |
What is JSON used for?
JSON is used for transmitting data between a server and a web application.
Is JSON language-dependent?
No, JSON is language-independent.
Can you use JSON with HTML?
Yes, JSON can be used with HTML to dynamically update web content.
Can JSON be used for configuration files?
Yes, JSON is often used for configuration files.
What is a JSON schema?
A JSON schema defines the structure, content, and types of data for JSON objects.
Can JSON contain arrays?
Yes, JSON can represent nested objects.
Does JSON support comments?
No, JSON does not support comments.
How do you handle JSON in Python?
How do you handle JSON in Python?