Pseudocode
Pseudocode is a simplified, language-agnostic way to outline a program’s logic. It uses plain English to describe the steps a computer program must take, bridging the gap between human thinking and computer code. By focusing on the logic rather than syntax, pseudocode helps programmers plan and communicate their ideas effectively. It’s especially useful for brainstorming and debugging, making it an essential tool in software development and algorithm design.
What is PseudoCode?
Pseudocode is a plain-language description of a computer program’s logic. It uses everyday language to outline the steps and structure of the code without focusing on syntax. This approach helps programmers plan, communicate, and debug their ideas effectively before actual coding begins.
Pseudocode Examples
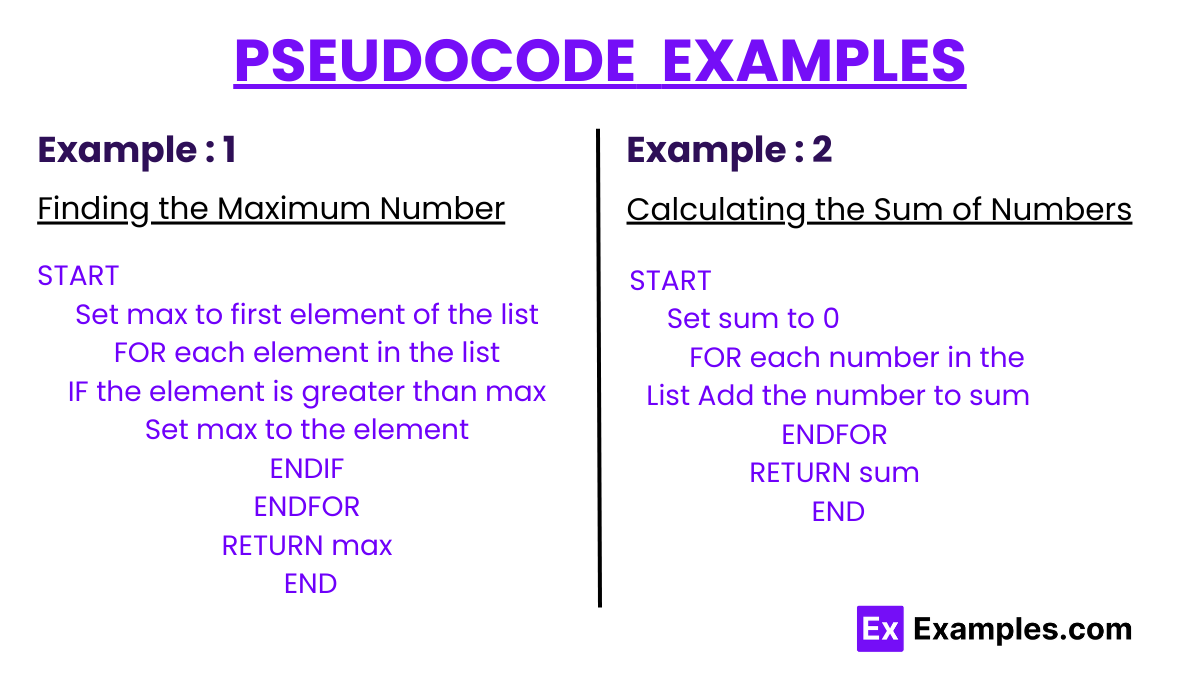
1. Calculate the Sum of Two Numbers
BEGIN
INPUT number1
INPUT number2
sum = number1 + number2
PRINT sum
END
2. Find the Largest Number in a List
BEGIN
INPUT list_of_numbers
largest = list_of_numbers[0]
FOR each number IN list_of_numbers
IF number > largest THEN
largest = number
ENDIF
ENDFOR
PRINT largest
END
3. Check if a Number is Prime
BEGIN
INPUT number
IF number <= 1 THEN
PRINT “Not Prime”
ELSE
is_prime = TRUE
FOR i FROM 2 TO (number – 1)
IF number MOD i == 0 THEN
is_prime = FALSE
BREAK
ENDIF
ENDFOR
IF is_prime THEN
PRINT “Prime”
ELSE
PRINT “Not Prime”
ENDIF
ENDIF
END
4. Bubble Sort Algorithm
BEGIN
INPUT list_of_numbers
n = LENGTH(list_of_numbers)
FOR i FROM 0 TO n-1
FOR j FROM 0 TO n-i-2
IF list_of_numbers[j] > list_of_numbers[j+1] THEN
SWAP list_of_numbers[j] WITH list_of_numbers[j+1]
ENDIF
ENDFOR
ENDFOR
PRINT list_of_numbers
END
5. Factorial of a Number Using Recursion
FUNCTION factorial(n)
IF n == 0 THEN
RETURN 1
ELSE
RETURN n * factorial(n – 1)
ENDIF
END FUNCTION
BEGIN
INPUT number
result = factorial(number)
PRINT result
END
Pseudocode in C
Pseudocode in C helps outline the logic of a C program without focusing on specific syntax. It uses simple, plain-language instructions to represent the flow and structure of the code, aiding in planning and communication. For instance, instead of writing for (int i = 0; i < n; i++), pseudocode might say “FOR each element from 0 to n.” This approach ensures that the logical sequence is clear and easy to follow, bridging the gap between idea and implementation.
How to write a Pseudo-Code?
Define the Objective : Clearly state what problem or task the program will address, ensuring a focused goal to guide the pseudocode development.
Break Down the Problem : Divide the main problem into smaller, manageable tasks, making it easier to plan and structure the pseudocode.
Write Steps in Plain Language : Use simple, clear language to describe each step, avoiding specific programming syntax to ensure understanding.
Use Standard Structures : Incorporate common programming constructs like loops and conditionals, using terms such as IF, ELSE, FOR, WHILE, and END for clarity.
Maintain Logical Flow : Arrange steps in a logical sequence, ensuring the process flows naturally from start to finish for better comprehension.
Keep It Simple and Concise : Focus on essential logic, avoiding unnecessary details to keep the pseudocode straightforward and easy to follow.
Review and Refine : Re-examine the pseudocode to ensure clarity and completeness, making adjustments for improved understanding and accuracy.
What is the need for Pseudocode
Clarifies Logic : Pseudocode helps in clarifying the logic of an algorithm by outlining steps in plain language, making complex processes easier to understand.
Enhances Communication : It serves as a communication tool among team members, enabling developers, designers, and stakeholders to discuss and agree on the logic before coding begins.
Simplifies Debugging : By focusing on logic without worrying about syntax, pseudocode makes it easier to identify and fix errors early in the development process.
Facilitates Planning : Pseudocode allows for thorough planning of a program’s structure and flow, ensuring that all aspects are considered and organized logically before implementation.
Aids in Learning : For beginners, pseudocode provides a way to grasp programming concepts without getting bogged down by language-specific syntax, making it an effective educational tool.
Pseudocode Algorithm
A pseudocode algorithm is a simplified, language-agnostic representation of a program’s logic, designed to describe an algorithm’s steps clearly and concisely. It uses plain language and standard programming constructs like loops, conditionals, and functions to outline the flow and structure of the code without focusing on syntax. This approach facilitates understanding and communication among developers and stakeholders, aids in debugging and planning, and serves as an educational tool for beginners. By prioritizing logical clarity over syntactical precision, pseudocode algorithms help bridge the gap between conceptual ideas and actual programming implementation.
Benifits of PseudoCode
- Simplifies Complex Concepts:
Pseudocode breaks down complex algorithms into simple, understandable steps, making it easier to grasp the overall logic. - Enhances Communication:
It acts as a universal language among programmers, designers, and stakeholders, facilitating better collaboration and understanding. - Improves Problem Solving:
By focusing on the logical flow rather than syntax, pseudocode helps in planning and troubleshooting algorithms effectively. - Aids in Debugging:
Early identification of logical errors is possible with pseudocode, reducing the time spent on debugging during the actual coding phase. - Educational Tool:
Pseudocode is an excellent learning aid for beginners, helping them understand programming logic without the complexity of syntax. - Language-Agnostic:
Being independent of any programming language, pseudocode can be easily adapted and translated into various coding languages.
Can pseudocode be used for complex algorithms?
Yes, pseudocode is effective for both simple and complex algorithms.
Is there a standard format for pseudocode?
While there is no strict standard, using consistent, clear language and logical flow is important.
How does pseudocode help beginners?
Pseudocode helps beginners understand programming concepts without the complexity of syntax.
Can pseudocode replace detailed design documents?
Pseudocode can complement detailed design documents but is not a replacement for them.
How detailed should pseudocode be?
Pseudocode should be detailed enough to outline the logic clearly but not overly complex.
Does pseudocode include variable declarations?
Pseudocode may include simple variable declarations if they aid in understanding the logic.
What is the main purpose of pseudocode?
The main purpose is to simplify and clarify the algorithm’s logic before actual coding begins.
Can pseudocode be used for all programming languages?
Yes, because it is language-agnostic, pseudocode can be translated into any programming language.
How does pseudocode facilitate planning?
It allows thorough planning of the program’s structure and flow, ensuring logical organization.
What is the difference between pseudocode and flowcharts?
Pseudocode uses plain language to describe logic, while flowcharts use diagrams to represent flow.
Is pseudocode used in professional development?
Yes, pseudocode is widely used in professional development for planning and communication.