Python Code Examples
Python code is a versatile tool for programming, widely used in various fields such as web development, data analysis, artificial intelligence, and scientific computing. Its simple syntax and readability make it accessible to beginners, while its extensive libraries and frameworks cater to advanced applications. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This flexibility, combined with a large, supportive community, makes Python a powerful and popular choice for developers worldwide.
What is Python Code?
Python code is a high-level programming language known for its simplicity and readability. It’s widely used for web development, data analysis, artificial intelligence, and more. Python’s extensive libraries and frameworks, along with support for various programming paradigms, make it highly versatile and powerful.
Python Code Examples
Hello World
print("Hello, World!")
Basic Arithmetic
a = 5
b = 3
sum = a + b
print("Sum:", sum)
If-Else Statement
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is 5 or less")
For Loop
for i in range(5):
print("Iteration:", i)
Function Definition
def greet(name):
return "Hello, " + name
print(greet("Alice"))
List Comprehension
squares = [x**2 for x in range(10)]
print(squares)
Dictionary
student = {
"name": "John",
"age": 21,
"courses": ["Math", "Computer Science"]
}
print(student)
Reading a File
with open('example.txt', 'r') as file:
content = file.read()
print(content)
Python Code Examples for Beginners
While Loop
# Printing numbers from 1 to 5 using a while loop
count = 1
while count <= 5:
print(“Count:”, count) count += 1
Variable Assignment
# Assigning values to variables
x = 10
y = 20
z = x + y
print("The value of z is:", z)
Finding the Length of a String:
# Finding the length of a string
message = "Hello, World!"
length = len(message)
print("The length of the message is:", length)
What can Python do?
- Web Development: Python is used by web developers to build server-side web applications. Frameworks like Django and Flask streamline the development process.
- Software Development: Python supports software developers in building, testing, and deploying applications. It is often used for scripting and automating tasks.
- Data Analysis: Python is a favorite among data analysts for processing, analyzing, and visualizing data. Libraries like Pandas and Matplotlib facilitate these tasks.
- Machine Learning: Python is widely used in machine learning and artificial intelligence, with powerful libraries such as TensorFlow and Scikit-Learn providing essential tools for building models.
- Automation: Python can automate repetitive tasks, saving time and reducing errors. Scripts can be written to handle file operations, data entry, and more.
- Game Development: Python is also used in game development. Libraries like Pygame provide tools to create games and multimedia applications.
- Networking: Python is used to handle networking tasks, including building network servers and clients, working with protocols, and managing network resources.
- Email Handling: Python can be used to automate email tasks such as sending, receiving, and managing web development email signatures, using libraries like smtplib and email.
Web development
- Web Scraping: Python can be used to extract data from websites using libraries like BeautifulSoup and Scrapy, often processing HTML content to gather the needed information.
- API Development: Python allows developers to create and consume APIs (Application Programming Interfaces) using frameworks like Flask and FastAPI, facilitating communication between different software systems.
- Scientific Computing: Python is extensively used in scientific research and computing with libraries like SciPy and NumPy, enabling complex calculations and simulations.
- Desktop Applications: Python can be used to develop cross-platform desktop applications using frameworks like PyQt and Tkinter, providing graphical user interfaces (GUIs).
- Internet of Things (IoT): Python is popular in IoT development, enabling the programming of devices and sensors to collect and process data, often using microcontrollers like Raspberry Pi.
- Cloud Computing: Python is widely used in cloud computing for automating cloud infrastructure, managing cloud resources, and building scalable applications on platforms like AWS, Google Cloud, and Azure.
- Cybersecurity: Python is utilized in cybersecurity for writing scripts to test security vulnerabilities, creating tools for penetration testing, and automating security tasks.
- Digital Marketing: Python helps in digital marketing by automating tasks such as web scraping for competitor analysis, email marketing campaigns, and analyzing customer data to drive marketing strategies.
Why We Use Python Code?
- Readability: Python’s syntax is clear and straightforward, making it easy to read and write. This readability enhances productivity and reduces the learning curve for new programmers.
- Versatility: Python can be used for various applications, including web development, data analysis, machine learning, and more. Its versatility makes it a one-stop solution for many programming needs.
- Extensive Libraries: Python boasts a vast collection of libraries and frameworks, such as Pandas for data analysis, Django for web development, and TensorFlow for machine learning, which significantly speed up development.
- Community Support: Python has a large, active community that contributes to its extensive documentation and offers support through forums, tutorials, and online courses, making it easier to find help and resources.
- Portability: Python is cross-platform, meaning it can run on various operating systems like Windows, macOS, and Linux without requiring changes to the codebase.
- Ease of Learning: Python is an excellent language for beginners due to its simple and intuitive syntax, which includes the use of conditional statements like
if
,elif
, andelse
for decision-making in code. - Integration Capabilities: Python easily integrates with other languages and technologies, allowing developers to use Python alongside C/C++, Java, or .NET, and connect to various databases and web services.
- Automation: Python is ideal for automating repetitive tasks and workflows, including file management, web scraping, and test automation, making it a powerful tool for increasing efficiency.
Python Syntax Compared to other Programming Languages
- Readability and Simplicity:
- Python: Uses indentation to define code blocks, which enhances readability and reduces the need for braces or keywords like
end
. - Other Languages: Languages like C++ and Java use braces
{}
to define code blocks, which can make the code appear more cluttered.
- Python: Uses indentation to define code blocks, which enhances readability and reduces the need for braces or keywords like
- Variable Declaration:
- Python: Variables are dynamically typed and do not require explicit declaration of data types. For example,
x = 10
. - Other Languages: Languages like Java require explicit type declaration, such as
int x = 10;
.
- Python: Variables are dynamically typed and do not require explicit declaration of data types. For example,
- Conditional Statements:
- Python: Uses
if
,elif
, andelse
for conditional statements, with a clear and concise syntax. - Other Languages: Languages like JavaScript use
if
,else if
, andelse
, often with braces{}
to define the scope of conditions.
- Python: Uses
- Loop Structures:
- Python: Employs simple syntax for loops, such as
for i in range(5):
andwhile condition:
. - Other Languages: Languages like C++ use more complex loop structures with initializations and conditions inside the loop definition, such as
for(int i = 0; i < 5; i++)
.
- Python: Employs simple syntax for loops, such as
- Data Visualization:
- Python: Has powerful libraries like Matplotlib and Seaborn for data visualization, which offer straightforward syntax for creating complex plots and charts.
- Other Languages: Languages like R are also strong in data visualization but often require more verbose syntax, while JavaScript uses libraries like D3.js, which can be more complex to set up and use compared to Python’s simplicity.
How to Write Python Code?
- Set Up the Environment: Install Python from the official website and choose an Integrated Development Environment (IDE) like PyCharm, VS Code, or Jupyter Notebook.
- Write the Code: Open your IDE or text editor, create a new file with a
.py
extension, and start writing your Python code. - Save the File: Save your Python file with a descriptive name, ensuring it ends with the
.py
extension, e.g.,my_script.py
. - Run the Code: Execute your script by running the command
python my_script.py
in your terminal or command prompt, or by clicking the run button in your IDE. - Debug and Test: Review the output for any errors, debug your code if necessary, and test it thoroughly to ensure it works as expected.
Automation or Scripting
- Readability and Simplicity: Python’s clean syntax enhances readability, making it easy to write and understand scripts.
- Versatility: Python’s extensive libraries support various automation tasks, from web scraping to data manipulation and visualization.
- Skills Development: Learning Python for automation boosts programming skills, enhancing problem-solving abilities and efficiency in handling repetitive tasks.
- Data Visualization: Python libraries like Matplotlib and Seaborn simplify data visualization, enabling automated creation of charts and graphs.
- Cross-Platform: Python scripts can run on multiple operating systems, ensuring compatibility and ease of deployment across different environments.
- Community Support: Python’s large community offers abundant resources, tutorials, and forums, aiding in quick problem resolution and learning.
Data Analysis and Machine Learning
- Data Manipulation: Python’s Pandas library offers powerful data manipulation capabilities, essential for preparing datasets for analysis and machine learning.
- Data Cleaning: Python simplifies data cleaning processes, allowing for efficient handling of missing values and data inconsistencies.
- Exploratory Data Analysis: Python’s libraries like Matplotlib and Seaborn facilitate exploratory data analysis through easy-to-create visualizations, enhancing insights.
- Machine Learning Models: Python’s Scikit-Learn library provides tools for building and evaluating machine learning models, from simple regressions to complex algorithms.
- Technical Skills Development: Mastering Python for data analysis and machine learning enhances technical skills, making professionals proficient in handling real-world data challenges.
- Big Data Integration: Python can integrate with big data technologies like Hadoop and Spark, enabling scalable data processing and advanced machine learning applications.
Uses of Python Code
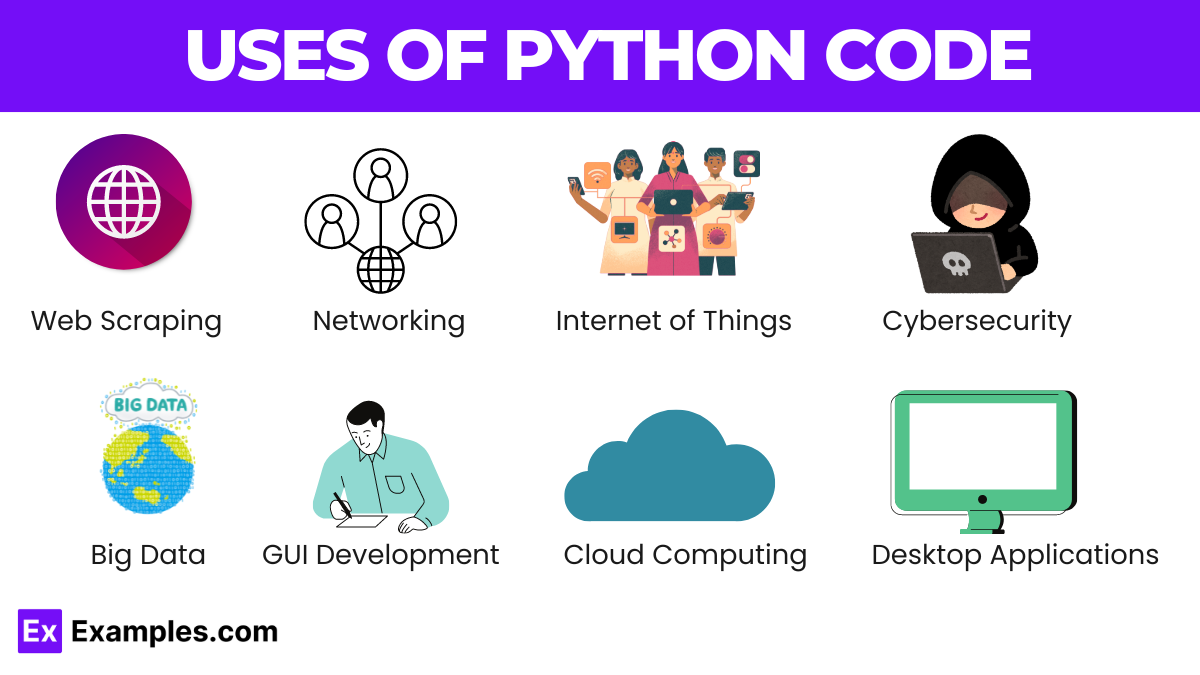
- Web Scraping: Extracting data from websites using BeautifulSoup and Scrapy.
- Game Development: Developing games and multimedia applications with Pygame.
- Networking: Handling network operations and protocols.
- Desktop Applications: Building cross-platform desktop applications with Tkinter and PyQt.
- IoT (Internet of Things): Programming devices and sensors for IoT projects.
- Cybersecurity: Writing scripts for penetration testing and security automation.
- Scientific Computing: Performing complex calculations and simulations with SciPy and NumPy.
- Big Data: Processing and analyzing large datasets with tools like PySpark.
- GUI Development: Creating graphical user interfaces with Tkinter.
- Cloud Computing: Automating cloud services and infrastructure management.
- Digital Marketing: Automating marketing tasks and analyzing customer data.
What is Python?
Python is a high-level, interpreted programming language known for its readability and simplicity.
How do you print something in Python?
Use the print()
function, e.g., print("Hello, World!")
.
How do you create a variable in Python?
Assign a value to a variable using =
, e.g., x = 10
.
What is a list in Python?
A list is an ordered collection of items, e.g., my_list = [1, 2, 3]
.
How do you add an item to a list?
Use the append()
method, e.g., my_list.append(4)
.
How do you access a value in a dictionary?
Use the key inside square brackets, e.g., my_dict["name"]
.
What is a function in Python?
A function is a block of reusable code defined with the def
keyword, e.g., def my_function():
.
What is a module in Python?
A module is a file containing Python code, which can be imported, e.g., import math
.
How do you install a package in Python?
Use the pip
command, e.g., pip install package_name
.
How do you create an object in Python?
Instantiate a class, e.g., my_object = MyClass()
.
Python Code Examples
Python code is a versatile tool for programming, widely used in various fields such as web development, data analysis, artificial intelligence, and scientific computing. Its simple syntax and readability make it accessible to beginners, while its extensive libraries and frameworks cater to advanced applications. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This flexibility, combined with a large, supportive community, makes Python a powerful and popular choice for developers worldwide.
What is Python Code?
Python code is a high-level programming language known for its simplicity and readability. It’s widely used for web development, data analysis, artificial intelligence, and more. Python’s extensive libraries and frameworks, along with support for various programming paradigms, make it highly versatile and powerful.
Python Code Examples
Hello World
print("Hello, World!")
Basic Arithmetic
a = 5
b = 3
sum = a + b
print("Sum:", sum)
If-Else Statement
x = 10
if x > 5:
print("x is greater than 5")
else:
print("x is 5 or less")
For Loop
for i in range(5):
print("Iteration:", i)
Function Definition
def greet(name):
return "Hello, " + name
print(greet("Alice"))
List Comprehension
squares = [x**2 for x in range(10)]
print(squares)
Dictionary
student = {
"name": "John",
"age": 21,
"courses": ["Math", "Computer Science"]
}
print(student)
Reading a File
with open('example.txt', 'r') as file:
content = file.read()
print(content)
Python Code Examples for Beginners
While Loop
# Printing numbers from 1 to 5 using a while loop
count = 1
while count <= 5:
print(“Count:”, count) count += 1
Variable Assignment
# Assigning values to variables
x = 10
y = 20
z = x + y
print("The value of z is:", z)
Finding the Length of a String:
# Finding the length of a string
message = "Hello, World!"
length = len(message)
print("The length of the message is:", length)
What can Python do?
Web Development: Python is used by web developers to build server-side web applications. Frameworks like Django and Flask streamline the development process.
Software Development: Python supports software developers in building, testing, and deploying applications. It is often used for scripting and automating tasks.
Data Analysis: Python is a favorite among data analysts for processing, analyzing, and visualizing data. Libraries like Pandas and Matplotlib facilitate these tasks.
Machine Learning: Python is widely used in machine learning and artificial intelligence, with powerful libraries such as TensorFlow and Scikit-Learn providing essential tools for building models.
Automation: Python can automate repetitive tasks, saving time and reducing errors. Scripts can be written to handle file operations, data entry, and more.
Game Development: Python is also used in game development. Libraries like Pygame provide tools to create games and multimedia applications.
Networking: Python is used to handle networking tasks, including building network servers and clients, working with protocols, and managing network resources.
Email Handling: Python can be used to automate email tasks such as sending, receiving, and managing web development email signatures, using libraries like smtplib and email.
Web development
Web Scraping: Python can be used to extract data from websites using libraries like BeautifulSoup and Scrapy, often processing HTML content to gather the needed information.
API Development: Python allows developers to create and consume APIs (Application Programming Interfaces) using frameworks like Flask and FastAPI, facilitating communication between different software systems.
Scientific Computing: Python is extensively used in scientific research and computing with libraries like SciPy and NumPy, enabling complex calculations and simulations.
Desktop Applications: Python can be used to develop cross-platform desktop applications using frameworks like PyQt and Tkinter, providing graphical user interfaces (GUIs).
Internet of Things (IoT): Python is popular in IoT development, enabling the programming of devices and sensors to collect and process data, often using microcontrollers like Raspberry Pi.
Cloud Computing: Python is widely used in cloud computing for automating cloud infrastructure, managing cloud resources, and building scalable applications on platforms like AWS, Google Cloud, and Azure.
Cybersecurity: Python is utilized in cybersecurity for writing scripts to test security vulnerabilities, creating tools for penetration testing, and automating security tasks.
Digital Marketing: Python helps in digital marketing by automating tasks such as web scraping for competitor analysis, email marketing campaigns, and analyzing customer data to drive marketing strategies.
Why We Use Python Code?
Readability: Python’s syntax is clear and straightforward, making it easy to read and write. This readability enhances productivity and reduces the learning curve for new programmers.
Versatility: Python can be used for various applications, including web development, data analysis, machine learning, and more. Its versatility makes it a one-stop solution for many programming needs.
Extensive Libraries: Python boasts a vast collection of libraries and frameworks, such as Pandas for data analysis, Django for web development, and TensorFlow for machine learning, which significantly speed up development.
Community Support: Python has a large, active community that contributes to its extensive documentation and offers support through forums, tutorials, and online courses, making it easier to find help and resources.
Portability: Python is cross-platform, meaning it can run on various operating systems like Windows, macOS, and Linux without requiring changes to the codebase.
Ease of Learning: Python is an excellent language for beginners due to its simple and intuitive syntax, which includes the use of conditional statements like
if
,elif
, andelse
for decision-making in code.Integration Capabilities: Python easily integrates with other languages and technologies, allowing developers to use Python alongside C/C++, Java, or .NET, and connect to various databases and web services.
Automation: Python is ideal for automating repetitive tasks and workflows, including file management, web scraping, and test automation, making it a powerful tool for increasing efficiency.
Python Syntax Compared to other Programming Languages
Readability and Simplicity:
Python: Uses indentation to define code blocks, which enhances readability and reduces the need for braces or keywords like
end
.Other Languages: Languages like C++ and Java use braces
{}
to define code blocks, which can make the code appear more cluttered.
Variable Declaration:
Python: Variables are dynamically typed and do not require explicit declaration of data types. For example,
x = 10
.Other Languages: Languages like Java require explicit type declaration, such as
int x = 10;
.
Conditional Statements:
Python: Uses
if
,elif
, andelse
for conditional statements, with a clear and concise syntax.Other Languages: Languages like JavaScript use
if
,else if
, andelse
, often with braces{}
to define the scope of conditions.
Loop Structures:
Python: Employs simple syntax for loops, such as
for i in range(5):
andwhile condition:
.Other Languages: Languages like C++ use more complex loop structures with initializations and conditions inside the loop definition, such as
for(int i = 0; i < 5; i++)
.
Data Visualization:
Python: Has powerful libraries like Matplotlib and Seaborn for data visualization, which offer straightforward syntax for creating complex plots and charts.
Other Languages: Languages like R are also strong in data visualization but often require more verbose syntax, while JavaScript uses libraries like D3.js, which can be more complex to set up and use compared to Python’s simplicity.
How to Write Python Code?
Set Up the Environment: Install Python from the official website and choose an Integrated Development Environment (IDE) like PyCharm, VS Code, or Jupyter Notebook.
Write the Code: Open your IDE or text editor, create a new file with a
.py
extension, and start writing your Python code.Save the File: Save your Python file with a descriptive name, ensuring it ends with the
.py
extension, e.g.,my_script.py
.Run the Code: Execute your script by running the command
python my_script.py
in your terminal or command prompt, or by clicking the run button in your IDE.Debug and Test: Review the output for any errors, debug your code if necessary, and test it thoroughly to ensure it works as expected.
Automation or Scripting
Readability and Simplicity: Python’s clean syntax enhances readability, making it easy to write and understand scripts.
Versatility: Python’s extensive libraries support various automation tasks, from web scraping to data manipulation and visualization.
Skills Development: Learning Python for automation boosts programming skills, enhancing problem-solving abilities and efficiency in handling repetitive tasks.
Data Visualization: Python libraries like Matplotlib and Seaborn simplify data visualization, enabling automated creation of charts and graphs.
Cross-Platform: Python scripts can run on multiple operating systems, ensuring compatibility and ease of deployment across different environments.
Community Support: Python’s large community offers abundant resources, tutorials, and forums, aiding in quick problem resolution and learning.
Data Analysis and Machine Learning
Data Manipulation: Python’s Pandas library offers powerful data manipulation capabilities, essential for preparing datasets for analysis and machine learning.
Data Cleaning: Python simplifies data cleaning processes, allowing for efficient handling of missing values and data inconsistencies.
Exploratory Data Analysis: Python’s libraries like Matplotlib and Seaborn facilitate exploratory data analysis through easy-to-create visualizations, enhancing insights.
Machine Learning Models: Python’s Scikit-Learn library provides tools for building and evaluating machine learning models, from simple regressions to complex algorithms.
Technical Skills Development: Mastering Python for data analysis and machine learning enhances technical skills, making professionals proficient in handling real-world data challenges.
Big Data Integration: Python can integrate with big data technologies like Hadoop and Spark, enabling scalable data processing and advanced machine learning applications.
Uses of Python Code
Web Scraping: Extracting data from websites using BeautifulSoup and Scrapy.
Game Development: Developing games and multimedia applications with Pygame.
Networking: Handling network operations and protocols.
Desktop Applications: Building cross-platform desktop applications with Tkinter and PyQt.
IoT (Internet of Things): Programming devices and sensors for IoT projects.
Cybersecurity: Writing scripts for penetration testing and security automation.
Scientific Computing: Performing complex calculations and simulations with SciPy and NumPy.
Big Data: Processing and analyzing large datasets with tools like PySpark.
GUI Development: Creating graphical user interfaces with Tkinter.
Cloud Computing: Automating cloud services and infrastructure management.
Digital Marketing: Automating marketing tasks and analyzing customer data.
What is Python?
Python is a high-level, interpreted programming language known for its readability and simplicity.
How do you print something in Python?
Use the print()
function, e.g., print("Hello, World!")
.
How do you create a variable in Python?
Assign a value to a variable using =
, e.g., x = 10
.
What is a list in Python?
A list is an ordered collection of items, e.g., my_list = [1, 2, 3]
.
How do you add an item to a list?
Use the append()
method, e.g., my_list.append(4)
.
How do you access a value in a dictionary?
Use the key inside square brackets, e.g., my_dict["name"]
.
What is a function in Python?
A function is a block of reusable code defined with the def
keyword, e.g., def my_function():
.
What is a module in Python?
A module is a file containing Python code, which can be imported, e.g., import math
.
How do you install a package in Python?
Use the pip
command, e.g., pip install package_name
.
How do you create an object in Python?
Instantiate a class, e.g., my_object = MyClass()
.