REST API – 20+ Examples, Types, Challenges, Advantages, Uses
REST API (Representational State Transfer Application Programming Interface) is a set of rules and conventions for building and interacting with web services. REST API use standard HTTP methods (such as GET, POST, PUT, DELETE) to enable communication between client and server applications. REST APIs often utilize JSON (JavaScript Object Notation) as a lightweight format for data interchange, making it easy to read and write data between client and server. For example, a Python code snippet can use a REST API to fetch and process data in JSON format. In diverse contexts like slam poetry, social surveys, and other interactive platforms, REST APIs can facilitate seamless data exchange and integration.
What is a REST API?
A REST API (Representational State Transfer Application Programming Interface) is a method of allowing communication between a client and a server over the internet. It uses standard HTTP methods like GET, POST, PUT, and DELETE to perform operations on data. REST APIs are designed to be stateless, meaning each request from a client to a server must contain all the information needed to understand and process the request.
Examples of REST API
- Fetching user data from a social media platform
- Retrieving weather information from a weather service
- Getting stock market data from a financial service
- Accessing map and location data from a mapping service
- Fetching product details from an e-commerce site
- Retrieving flight information from an airline’s API
- Getting movie information from a film database
- Accessing news articles from a news service
- Fetching book details from a library’s catalog
- Retrieving music tracks and playlists from a music streaming service
- Accessing health and fitness data from a wellness app
- Getting sports scores and stats from a sports service
- Retrieving transaction data from a banking API
- Accessing job listings from a job board
- Fetching recipe information from a cooking site
Types of REST API
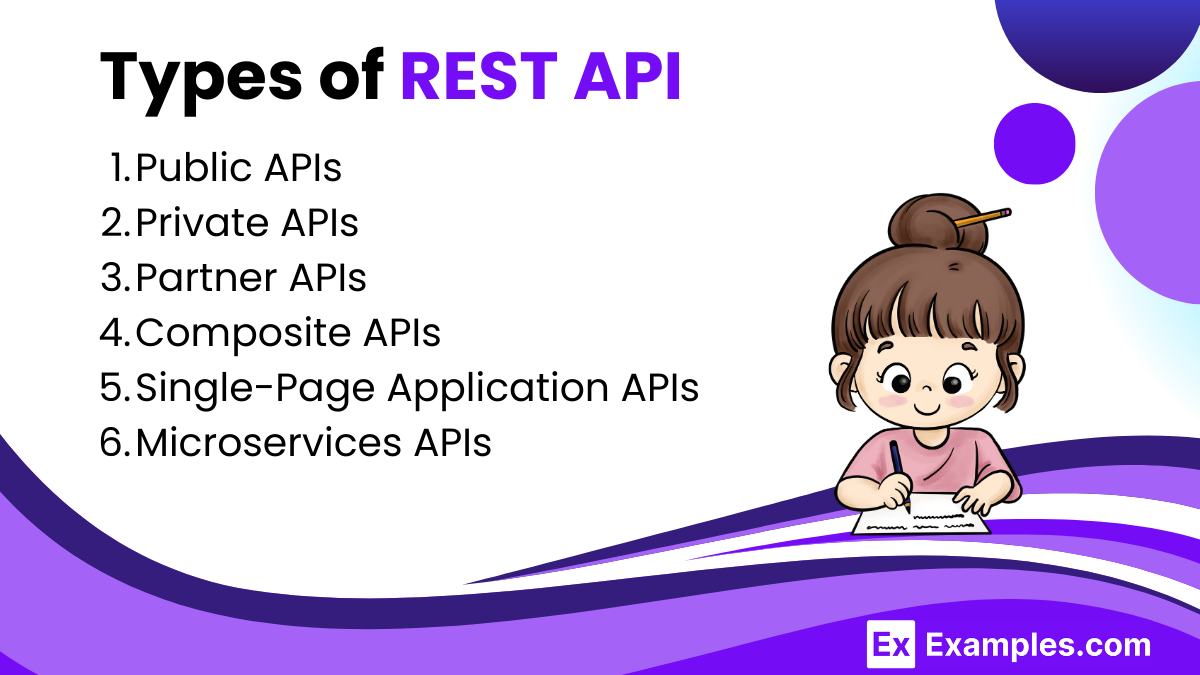
1. Public APIs
- Description: Accessible by any developer or user without restrictions.
- Examples: OpenWeatherMap API, Google Maps API.
2. Private APIs
- Description: Restricted to specific users or developers within an organization.
- Examples: Internal APIs for a company’s internal applications.
3. Partner APIs
- Description: Shared with specific third-party partners or collaborators.
- Examples: APIs shared between a company and its business partners.
4. Composite APIs
- Description: Combine multiple API calls into a single request to reduce server round trips.
- Examples: An API that retrieves user information and their related posts in one call.
5. Single-Page Application (SPA) APIs
- Description: Designed specifically for single-page applications to interact dynamically with back-end services.
- Examples: APIs used in modern web applications like React, Angular, or Vue.js apps.
6. Microservices APIs
- Description: REST APIs designed for microservices architecture, where each service has its own API.
- Examples: APIs for individual services like user management, payment processing, and product catalog in an e-commerce platform.
History of REST API
The REST API (Representational State Transfer Application Programming Interface) was introduced in 2000 by Roy Fielding in his doctoral dissertation at the University of California, Irvine. Fielding defined REST as an architectural style designed to exploit the existing protocols of the Web, particularly HTTP. This style emphasizes stateless communication, uniform interfaces, and the use of standard HTTP methods (GET, POST, PUT, DELETE) to facilitate interactions between clients and servers. REST’s simplicity, scalability, and ease of integration quickly made it a preferred choice for web services, influencing the design of modern APIs and becoming a foundational technology in web development.
How REST API Work
1. Client-Server Architecture
- Description: REST API operates on a client-server architecture, where the client requests resources, and the server provides them.
- Example: A web browser (client) sends a request to a web server, which returns the requested web page.
2. Stateless Communication
- Description: Each request from the client to the server must contain all the information needed to understand and process the request. The server does not store any state between requests.
- Example: When a client requests user data, it must include authentication details in each request, as the server does not remember previous requests.
3. Resources and URIs
- Description: Resources are the objects or data entities that are accessed via REST API. Each resource is identified by a Uniform Resource Identifier (URI).
- Example:
/users/123
might be the URI for accessing the user with ID 123.
4. HTTP Methods
- Description: REST APIs use standard HTTP methods to perform operations on resources.
- GET: Retrieve a resource.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Remove a resource.
- Example:
GET /users
retrieves a list of users, whilePOST /users
creates a new user.
5. HTTP Status Codes
- Description: The server responds to requests with standard HTTP status codes to indicate the result of the request.
- 200 OK: Request was successful.
- 201 Created: Resource was successfully created.
- 400 Bad Request: The request was invalid.
- 404 Not Found: Resource not found.
- 500 Internal Server Error: Server encountered an error.
- Example: A
GET /users/123
request might return a200 OK
status code if the user exists, or a404 Not Found
if the user does not exist.
6. Request and Response Bodies
- Description: The request body contains data sent to the server, typically in JSON or XML format. The response body contains data returned by the server.
- Example: A
POST /users
request might include a JSON body with user details, and the server response might include a JSON body with the created user information.
7. Headers
- Description: Headers provide metadata about the request or response, such as content type and authentication tokens.
- Example: The
Content-Type
header specifies the format of the data (application/json
), and theAuthorization
header includes an authentication token.
REST API Design Principles
1. Use Meaningful Resource URIs
- Description: URIs should represent resources and be easy to understand.
- Example:
/users/123
for accessing user with ID 123.
2. Utilize HTTP Methods Correctly
- Description: Use standard HTTP methods to perform operations on resources.
- GET: Retrieve a resource.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Remove a resource.
- PATCH: Partially update a resource.
3. Statelessness
- Description: Each request from a client must contain all the information needed by the server to fulfill that request.
- Example: Include authentication tokens in every request instead of relying on server session state.
4. Use HTTP Status Codes Appropriately
- Description: Return appropriate HTTP status codes based on the outcome of the client’s request.
- 200 OK: The request was successful.
- 201 Created: A resource was successfully created.
- 204 No Content: The request was successful, but there is no representation to return.
- 400 Bad Request: The request could not be understood or was missing required parameters.
- 401 Unauthorized: Authentication failed or user does not have permissions for the desired action.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: An error occurred on the server.
5. Consistent Data Formatting
- Description: Use consistent data formats for requests and responses, typically JSON or XML.
- Example:
{ "id": 123, "name": "John Doe" }
.
6. Versioning
- Description: Implement versioning to manage changes and updates to the API without breaking existing clients.
- Example: Include the version number in the URI, such as
/v1/users/123
.
7. Security
- Description: Secure the API by using HTTPS, validating inputs, and implementing authentication and authorization mechanisms.
- Example: Use OAuth for secure user authentication.
8. Pagination, Filtering, and Sorting
- Description: Provide mechanisms to paginate, filter, and sort large sets of data.
- Example:
/users?page=2&limit=10&sort=asc&filter=active
.
9. Hypermedia as the Engine of Application State (HATEOAS)
- Description: Include hypermedia links in responses to provide clients with the information needed to navigate the API.
- Example:
{ "id": 123, "name": "John Doe", "links": { "self": "/users/123", "friends": "/users/123/friends" } }
.
10. Documentation
- Description: Provide clear and comprehensive documentation for the API.
- Example: Use tools like Swagger or OpenAPI to create interactive API documentation.
REST API Design and Architecture Constraints
- Client-Server Architecture: Separates the client and server, enhancing scalability and simplifying interactions.
- Statelessness: Each request from the client contains all necessary information, improving reliability and scalability, though it may require more complexity on the client side.
- Cacheability: Responses must indicate whether they can be cached, improving performance and reducing server load.
- Uniform Interface: Standardizes communication between clients and servers, simplifying and decoupling the architecture.
- Layered System: The architecture is composed of hierarchical layers, enhancing scalability and improving security.
- Code on Demand (Optional): Servers can send executable code to clients, simplifying client implementation by reducing the need for certain functionalities.
RESTful API Authentication Methods
- Basic Authentication: Uses a username and password encoded in Base64. Simple but not secure without HTTPS, as credentials are sent in every request.
- Token-Based Authentication: Clients receive a token after providing valid credentials, which they include in subsequent requests. More secure as tokens can be expired and regenerated without exposing credentials.
- OAuth (Open Authorization): A token-based method allowing third-party apps to access user resources without exposing credentials. Highly secure, supports scopes for fine-grained access control, and commonly used for user authorization.
- JWT (JSON Web Tokens): Tokens are issued by the server, encoded in a compact JSON format, and include claims about the user. Secure and stateless, as the server doesn’t need to store session information.
- API Key: Clients include a unique key provided by the server in their requests. Simple to implement, but less secure if keys are not managed properly.
- HMAC (Hash-based Message Authentication Code): Uses a shared secret key to produce a hash value that verifies the integrity and authenticity of the message. Provides data integrity and authenticity, suitable for environments requiring message verification.
REST vs. SOAP
Aspect | REST | SOAP |
---|---|---|
Protocol | REST is an architectural style, not a protocol. | SOAP is a protocol. |
Data Format | Primarily JSON, XML, but also supports other formats like HTML and plain text. | XML only. |
Communication | Uses standard HTTP methods (GET, POST, PUT, DELETE). | Uses HTTP, SMTP, TCP, and other protocols, but primarily relies on HTTP. |
Message Security | Relies on underlying protocols like HTTPS for security. | Built-in security protocols like WS-Security. |
Complexity | Simpler, less verbose, easier to use. | More complex, with a strict set of rules and a heavier XML format. |
Performance | Generally faster due to less overhead. | Slower due to the complexity and size of the XML messages. |
State | Stateless. Each request from client to server must contain all the information needed to understand and process the request. | Can be stateless or stateful. |
Service Interface | Uses URLs to expose resources. | Uses service interfaces and contracts defined by WSDL. |
Error Handling | HTTP status codes (e.g., 200 OK, 404 Not Found). | Custom XML-based error messages. |
Standards Compliance | Fewer standards, more flexible. | Strict standards, including WS-Security, WS-AtomicTransaction, etc. |
Transaction Management | Limited support for transactions. | Built-in support for ACID-compliant transactions. |
Caching | Fully supports caching. | Does not natively support caching. |
Tooling and Support | Extensive support in web frameworks and libraries. | Extensive support in enterprise environments and tools. |
Use Cases | Web services where performance and scalability are important. | Enterprise-level services requiring high security and transaction compliance. |
REST API Challenges
- Security: Ensuring secure communication, data protection, and authentication can be complex, especially with sensitive information.
- Rate Limiting: Managing and enforcing rate limits to prevent abuse while maintaining performance for all users.
- Versioning: Handling API versioning to ensure backward compatibility without disrupting existing clients.
- Error Handling: Providing clear, consistent, and useful error messages to help clients understand and fix issues.
- Documentation: Creating and maintaining comprehensive, up-to-date documentation to help developers use the API effectively.
- Scalability: Designing APIs to handle increasing loads and large volumes of requests without degradation in performance.
- Consistency: Ensuring uniformity in API design, naming conventions, and behavior across different endpoints.
- State Management: Maintaining statelessness while managing client state effectively, such as through tokens or cookies.
- Caching: Implementing effective caching strategies to improve performance without serving stale data.
- Testing: Comprehensive testing to cover various scenarios, including edge cases, to ensure robustness and reliability.
Advantages and Disadvantages of REST API
Aspect | Advantages | Disadvantages |
---|---|---|
Simplicity | Easy to understand and use with standard HTTP methods. | Can be less structured, leading to inconsistencies in API design. |
Scalability | Stateless nature allows for scalable and distributed systems. | Requires careful management of stateless interactions, which can be complex. |
Flexibility | Supports multiple data formats (JSON, XML, HTML, etc.). | Lack of standardization can lead to variations in API implementations. |
Performance | Can be optimized with caching mechanisms. | Overhead of using HTTP can impact performance compared to other protocols like gRPC. |
Statelessness | Each request contains all information needed, simplifying server design. | Statelessness can lead to larger payloads, as all information must be included in each request. |
HTTP Support | Leverages existing web infrastructure, including firewalls, routers, and proxies. | Limited to the capabilities and constraints of HTTP. |
Interoperability | Language and platform agnostic, allowing integration with various systems. | Differences in implementation can cause interoperability issues. |
Caching | Easily implement caching strategies to improve performance and reduce load. | Incorrect caching can lead to serving stale or outdated data. |
Error Handling | Uses standard HTTP status codes for error handling, providing clear feedback. | May require additional mechanisms to convey detailed error information. |
Community and Tools | Wide adoption and extensive support with numerous libraries and tools available. | Popularity means a large number of APIs with varying quality and adherence to best practices. |
Documentation | Often supported by tools like Swagger/OpenAPI for automatic documentation generation. | Comprehensive documentation requires ongoing maintenance and effort. |
HATEOAS | Hypermedia as the Engine of Application State can guide clients in API usage. | Implementing HATEOAS can add complexity and is often not fully utilized. |
Uses of REST API
- Web Service Integration: REST APIs enable seamless integration between different web services. For example, a website can use a REST API to fetch data from a remote server, such as retrieving product information from an e-commerce platform or weather data from a meteorological service.
- Mobile Application Development: REST APIs are widely used in mobile app development to connect applications with backend servers. They allow mobile apps to fetch, update, and sync data with remote databases, ensuring that users have access to the latest information.
- Microservices Architecture: In microservices architecture, REST APIs facilitate communication between different microservices. Each microservice exposes a REST API, enabling them to interact with one another and perform specific functions within a larger system.
- Third-Party Integrations: REST APIs enable third-party integrations by allowing external developers to access and interact with a service’s functionality. Examples include integrating payment gateways, social media logins, and email marketing services into a website or application.
- Data Access and Manipulation: REST APIs provide a standardized way to access and manipulate data stored in databases. They allow applications to perform CRUD operations on data, such as creating new records, reading existing data, updating information, and deleting entries.
- Webhooks and Real-Time Notifications: REST APIs are used to create webhooks, which are HTTP callbacks that notify other systems of events in real time. For instance, a payment processing service might use webhooks to notify an e-commerce platform when a transaction is completed.
- IoT (Internet of Things): REST APIs play a crucial role in IoT applications by enabling devices to communicate with cloud services. They allow IoT devices to send data to remote servers for processing and receive commands to control device behavior.
- Single-Page Applications (SPAs): REST APIs are essential for SPAs, which dynamically load content without refreshing the entire page. They enable the front-end application to request and display data from the server as users interact with the app.
What is a resource in REST API?
A resource is any piece of data that can be identified and manipulated, such as a user, order, or product.
What does stateless mean in REST API?
Stateless means each request from a client contains all the information needed to process it, without relying on stored context.
How do you authenticate in a REST API?
You authenticate in a REST API using methods like API keys, OAuth tokens, or basic authentication.
What is a RESTful service?
A RESTful service is a web service that adheres to the principles and constraints of REST architecture.
What is an endpoint in REST API?
An endpoint is a specific URL where an API resource can be accessed, such as /users or /orders.
What is the difference between REST and SOAP?
REST uses standard HTTP and is simpler and more flexible, while SOAP uses XML and includes built-in error handling and security features.
How do you handle errors in REST API?
Handle errors using standard HTTP status codes like 404 for not found, 500 for server error, and providing descriptive error messages.
What is JSON and how is it used in REST API?
JSON (JavaScript Object Notation) is a lightweight data format used to represent and exchange data in REST API responses.
How do you version a REST API?
Version a REST API by including the version number in the URL, such as /v1/users, or in request headers.
What is HATEOAS in REST API?
HATEOAS (Hypermedia As The Engine Of Application State) is a constraint of REST where clients interact with applications entirely through hypermedia.